1.自定义注解
<?php
namespace App\Annotation;
use Hyperf\Di\Annotation\AbstractAnnotation;
class ValidateParams extends AbstractAnnotation
{
}
2.创建aop切面
<?php
namespace App\Aspect;
use App\Annotation\ValidateParams;
use App\common\response\SystemCode;
use App\Exception\ValidateException;
use Hyperf\Di\Annotation\Aspect;
use Hyperf\Di\Annotation\Inject;
use Hyperf\Di\Aop\AbstractAspect;
use Hyperf\Di\Aop\ProceedingJoinPoint;
use Hyperf\HttpServer\Router\Dispatched;
use Hyperf\Logger\LoggerFactory;
use Hyperf\HttpServer\Contract\RequestInterface;
use Hyperf\Validation\Contract\ValidatorFactoryInterface;
use Psr\Container\ContainerInterface;
class ValidateAspect extends AbstractAspect
{
protected $container;
protected $request;
protected $logger;
protected $validationFactory;
public function __construct(ContainerInterface $container, RequestInterface $request, LoggerFactory $logger)
{
$this->container = $container;
$this->request = $request;
$this->logger = $logger->get('log', 'default');
}
public $annotations = [
ValidateParams::class,
];
public function process(ProceedingJoinPoint $proceedingJoinPoint)
{
$this->logger->info(date('Y-m-d H:i:s', time()),["ValidateParams注解调用前执行..."]);
list($LongController, $method) = $this->request->getAttribute(Dispatched::class)->handler->callback;
$controller = substr(substr(strrchr($LongController,'\\'),1),0,-10);
$res = $this->paramsValidate($controller,$method,$this->request);
if($res["code"] === -1){
throw new ValidateException($res["data"],SystemCode::SYSTEM_ERROR_PARAM_NULL);
}
$result = $proceedingJoinPoint->process();
$this->logger->info(date('Y-m-d H:i:s', time()),["ValidateParams注解调用后执行..."]);
return $result;
}
private function paramsValidate($controller,$method,$request){
$nameSpace = '\App\Vilidate\\'.$controller;
$ValidateObj = (new $nameSpace)->$method;
$validator = $this->validationFactory->make(
$request->all(),$ValidateObj["rule"],$ValidateObj["msg"]
);
if ($validator->fails()){
$errorMessage = $validator->errors()->first();
return ["code"=>-1,"msg"=>"校验不通过!","data"=>$errorMessage];
}
return ["code"=>0,"msg"=>"校验通过!","data"=>[]];
}
}
3.添加校验规则
<?php
namespace App\Vilidate;
class Test
{
public $index = [
'rule'=>[
],
'msg'=>[
]
];
public $exceptionTest = [
'rule'=>[
'title' => 'required|max:5',
'body' => 'required',
],
'msg'=>[
'title.required' => 'title参数不能为空!',
'title.max' => 'title最大长度超限',
'body.required' => 'body参数不能为空!',
]
];
public $eventTest = [
'rule'=>[
'title' => 'required|max:5',
'body' => 'required',
],
'msg'=>[
'title.required' => 'title参数不能为空!',
'title.max' => 'title最大长度超限',
'body.required' => 'body参数不能为空!',
]
];
public $getUser = [
'rule'=>[
'userId' => 'required|min:0|numeric',
],
'msg'=>[
'userId.required' => 'userId参数不能为空!',
'userId.numeric' => 'userId格式必须为数字!',
'userId.min' => 'userId最小长度超限',
]
];
public $validateTest = [
'rule'=>[
'userId' => 'required|min:0|numeric',
],
'msg'=>[
'userId.required' => 'userId参数不能为空!',
'userId.numeric' => 'userId格式必须为数字!',
'userId.min' => 'userId最小长度超限',
]
];
}
4.使用
public function validateTest(RequestInterface $request)
{
$params = $request->all();
$userId = $params["userId"];
return RespResult::result(SystemCode::SYSTEM_SUCCESS, SystemMessage::SYSTEM_SUCCESS, $this->userModel->getUser($userId));
}
5.检验是否可以拦截
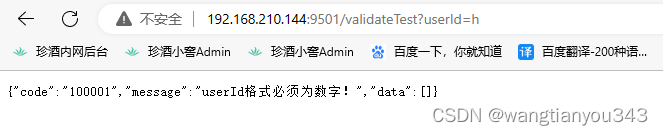
注:用到的注解,一定要use引进来哈