让不同的div区块从宽度(width)、高度(height)、边框(border)、文字大小(font-size)、不透明度(opacity)等多个维度实现JS运动框架构建。
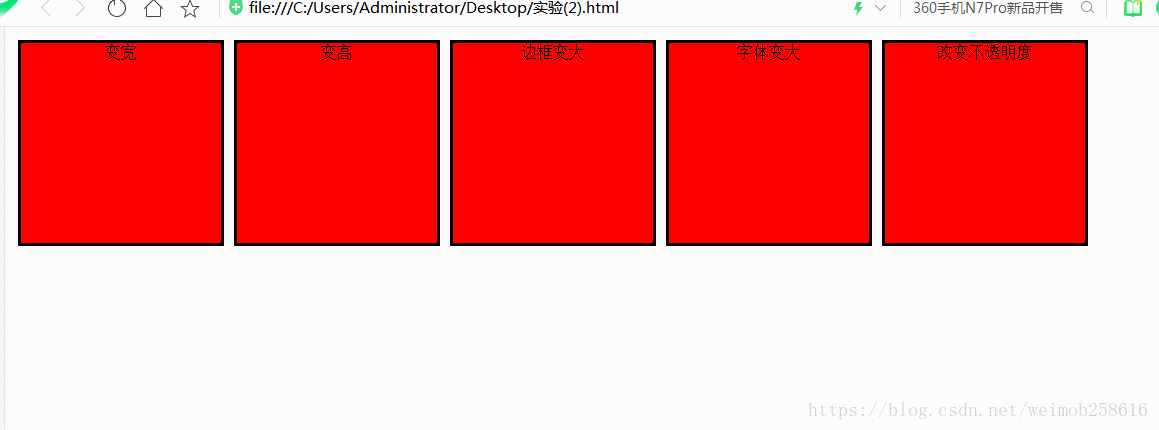
HTML代码部分
<div class='div1'>变宽</div>
<div class="div1">变高</div>
<div class="div1">边框变大</div>
<div class="div1">字体变大</div>
<div class="div1">改变不透明度</div>
CSS样式表
.div{float:left;width:200px;height:200px;background:red;padding:0;opacity:1;margin:5px;border:3px;}
针对宽度(width)、高度(height)、边框(border)、文字大小(font-size)构建的运动框架。
window.onload=function()
{
var oDiv=document.getElementsByClassName('div1');
oDiv[0].onmouseover=function(){
startMove(this,'width',400)
}
oDiv[0].onmouseout=function(){
startMove(this,'width',200)
}
oDiv[1].onmouseover=function(){
startMove(this,'height',400)
}
oDiv[1].onmouseout=function(){
startMove(this,'height',200)
}
oDiv[2].onmouseover=function(){
startMove(this,'borderWidth',10)
}
oDiv[2].onmouseout=function(){
startMove(this,'borderWidth',3)
}
oDiv[3].onmouseover=function(){
startMove(this,'fontSize',32)
}
oDiv[3].onmouseout=function(){
startMove(this,'fontSize',16)
}
}
function getStyle(obj,name)
{
if(getComputedStyle(obj,null)[name])
{
return getComputedStyle(obj,null)[name];
//fireFox、chrome
}
else
{
return obj.currentStyle[name];
//IE
}
}
function startMove(obj,attr,iTarget)
{
clearInterval(obj.timer);
obj.timer=setInterval(function(){
var cur=0;
cur=parseInt(getStyle(obj,attr));
var speed=(iTarget-cur)/30;
speed=speed>0?Math.ceil(speed):Math.floor(speed);
if(cur==iTarget)
{
clearInterval(obj.timer);
}
else
{
obj.style[attr]=cur+speed+'px';
}
},30)
}
不透明度(opacity)属性值不带“px”,需要单独设置
window.onload=function()
{
var oDiv=document.getElementsByClassName('div1');
oDiv[0].onmouseover=function(){
startMove(this,'width',400)
}
oDiv[0].onmouseout=function(){
startMove(this,'width',200)
}
oDiv[1].onmouseover=function(){
startMove(this,'height',400)
}
oDiv[1].onmouseout=function(){
startMove(this,'height',200)
}
oDiv[2].onmouseover=function(){
startMove(this,'borderWidth',10)
}
oDiv[2].onmouseout=function(){
startMove(this,'borderWidth',3)
}
oDiv[3].onmouseover=function(){
startMove(this,'fontSize',32)
}
oDiv[3].onmouseout=function(){
startMove(this,'fontSize',16)
}
oDiv[4].onmouseover=function(){
startMove(this,'opacity',30)
}
oDiv[4].onmouseout=function(){
startMove(this,'opacity',100)
}
}
function getStyle(obj,name)
{
if(getComputedStyle(obj,null)[name])
{
return getComputedStyle(obj,null)[name];
//fireFox、chrome
}
else
{
return obj.currentStyle[name];
//IE
}
}
function startMove(obj,attr,iTarget)
{
clearInterval(obj.timer);
obj.timer=setInterval(function(){
var cur=0;
if(attr=="opacity")
{
cur=Math.round(parseFloat(getStyle(obj,attr))*100);
}
else
{
cur=parseInt(getStyle(obj,attr));
}
var speed=(iTarget-cur)/30;
speed=speed>0?Math.ceil(speed):Math.floor(speed);
if(cur==iTarget)
{
clearInterval(obj.timer);
}
else
{
if(attr=="opacity")
{
cur+=speed;
obj.style.fliter='alpha(opacity:'+cur+')';
obj.style.opacity=cur/100;
}
else
{
obj.style[attr]=cur+speed+'px';
}
}
},30)
}
[1]函数getStyle(obj,name)获取的值如width、height、borderSize等为带有单位的字符串,并非数字。
function getStyle(obj,name){
if(getComputedStyle(obj,null)[name])
{
return getComputedStyle(obj,null)[name];
}
else
{
return obj.CurrentStyle[name];
}
}
完整的源代码
<!doctype html>
<html>
<head>
<title>多物体多属性维度的运动示例</title>
<meta charset="utf-8">
<style>
.div1{float:left;width:200px;height:200px;background:red;padding:0;opacity:1;filter:alpha(opacity=100);margin:5px;border:3px solid black;text-align:center;}
</style>
<script>
window.onload=function()
{
var oDiv=document.getElementsByClassName('div1');
oDiv[0].onmouseover=function(){
startMove(this,'width',400)
}
oDiv[0].onmouseout=function(){
startMove(this,'width',200)
}
oDiv[1].onmouseover=function(){
startMove(this,'height',400)
}
oDiv[1].onmouseout=function(){
startMove(this,'height',200)
}
oDiv[2].onmouseover=function(){
startMove(this,'borderWidth',10)
}
oDiv[2].onmouseout=function(){
startMove(this,'borderWidth',3)
}
oDiv[3].onmouseover=function(){
startMove(this,'fontSize',32)
}
oDiv[3].onmouseout=function(){
startMove(this,'fontSize',16)
}
oDiv[4].onmouseover=function(){
startMove(this,'opacity',30)
}
oDiv[4].onmouseout=function(){
startMove(this,'opacity',100)
}
}
function getStyle(obj,name)
{
if(getComputedStyle(obj,null)[name])
{
return getComputedStyle(obj,null)[name];
//fireFox、chrome
}
else
{
return obj.currentStyle[name];
//IE
}
}
function startMove(obj,attr,iTarget)
{
clearInterval(obj.timer);
obj.timer=setInterval(function(){
var cur=0;
if(attr=="opacity")
{
cur=Math.round(parseFloat(getStyle(obj,attr))*100);
}
else
{
cur=parseInt(getStyle(obj,attr));
}
var speed=(iTarget-cur)/30;
speed=speed>0?Math.ceil(speed):Math.floor(speed);
if(cur==iTarget)
{
clearInterval(obj.timer);
}
else
{
if(attr=="opacity")
{
cur+=speed;
obj.style.fliter='alpha(opacity:'+cur+')';
obj.style.opacity=cur/100;
}
else
{
obj.style[attr]=cur+speed+'px';
}
}
},30)
}
</script>
</head>
<body>
<div class='div1'>变宽</div>
<div class="div1">变高</div>
<div class="div1">边框变大</div>
<div class="div1">字体变大</div>
<div class="div1">改变不透明度</div>
</body>
</html>