前端架构 从入门到微前端
I am starting a series of articles on architecture of projects, especially Front-End. The posts will have an emphasis on explaining everything “in English”, without so much code. Perhaps, in another moment, the practical part will come up in a series of videos. I will post the texts based on a list of several subjects, from folders and files to versioning, continuous integration and DevOps, not necessarily in that order — and, in the end, I will get everything together in an ordered list. For now, I decided to start with Tests. Leave your suggestions in the comments. :)
我开始撰写有关项目体系结构的系列文章,尤其是Front-End 。 这些帖子将重点说明没有太多代码的所有“英语”内容。 也许再过一会,实用的部分将出现在一系列视频中。 我将根据几个主题的列表发布文本,从文件夹和文件到版本控制,持续集成和DevOps,而不必按此顺序进行-最后,我将所有内容整理在一个有序列表中。 现在,我决定从Tests开始。 将您的建议留在评论中。 :)
Before starting, it is important to remember the existence of a universe of Formatters and Linters, but I prefer to leave them for an article on standards, where we can talk about the choices that are the reason for which linters exists: reinforce patterns, either by convention, security or whatever technical reasons.
在开始之前,重要的是要记住存在Formatters和Linters的世界,但是我更喜欢将它们留在一篇有关标准的文章中,在这里我们可以讨论构成linters的原因的选择:加强模式,或者根据惯例,安全性或任何技术原因。
We will talk about:
我们将谈论:
- Unit 单元
- Integration积分
- End-to-end端到端
- Regression回归
Each of these will be structured with:
每一个都将具有以下结构:
- What it is 这是什么
- When to use it什么时候使用
- Pros优点
- Cons缺点
- Tools工具类
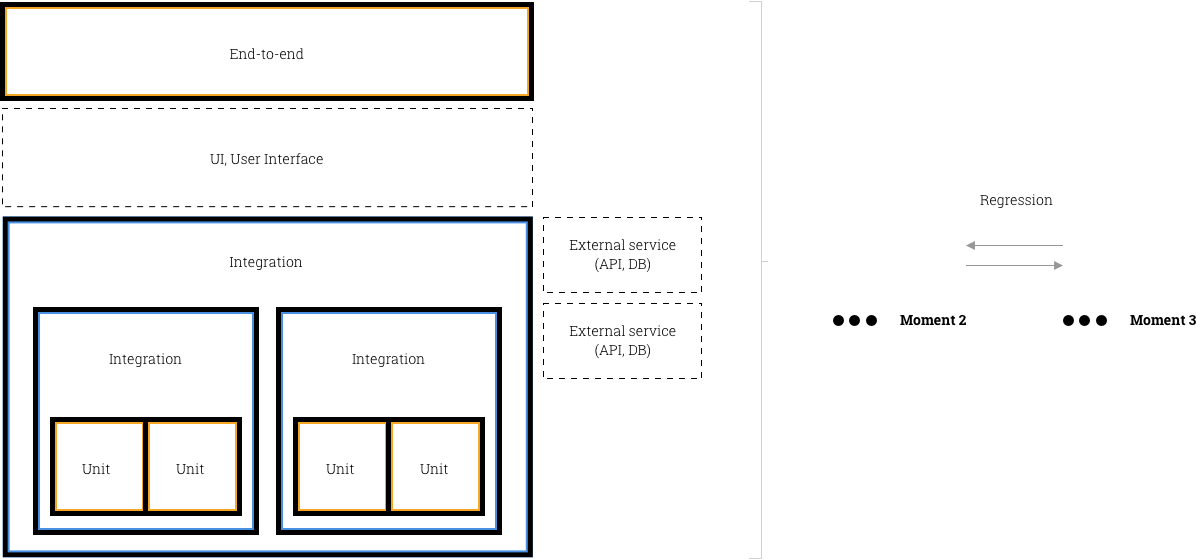
单元(Unit)
这是什么(What it is)
As the name says, it refers to the units, and, as the word suggest, it means that it is something that cannot be divided, since it is the smallest possible part. When referring to software, it means that they are functions that only do one thing. Unit tests are responsible for attesting that such functions (or units) are behaving properly. As we are talking about Front-End, these functions are written in Javascript or any other language that compiles to it.
顾名思义,它指的是单位,而顾名思义,则表示它是不可分割的,因为它是最小的部分。 当提到软件时,这意味着它们是只能做一件事的功能。 单元测试负责证明此类功能(或单元)的行为正常。 当我们谈论前端时,这些函数是用Javascript或编译成它的任何其他语言编写的。
Whether using the TDD process or not, it is difficult for a developer to take into account all possible scenarios, especially when programming such functions. Writing unit tests can help you to prepare your code for situations that are not “optimal”. In addition, they can make sure that during the life of the project, the “little guys” (unit tests) will complain before you notice such errors, in case something goes out of the established format or architecture,. By writing them, you are creating an army of robots with a life of their own, whose only mission is to observe their functions and ensure that they are correct.
无论是否使用TDD流程,开发人员都难以考虑所有可能的情况,尤其是在对此类功能进行编程时。 编写单元测试可以帮助您为非“最佳”情况准备代码。 此外,他们可以确保在项目生命周期中,“小家伙”(单元测试)会在您发现此类错误之前进行投诉,以防万一某些内容超出了既定的格式或体系结构。 通过编写它们,您将创建一支拥有自己生命的机器人大军,其唯一任务是观察其功能并确保其正确。
An example, in the case of a function that formats dates, would be to guarantee the outputs, for practically all types of input — which happens when someone uses a date in different formats, invalid dates, and so on, including how the function handles errors and exceptions.
例如,对于格式化日期的函数,将保证几乎所有类型的输入的输出-当某人使用不同格式的日期,无效日期等(包括该函数如何处理)时会发生这种情况错误和异常。
什么时候使用 (When to use it)
- The project has a considerable amount of logic该项目具有大量逻辑
- There are many functions that are reused in many places有很多功能可以在许多地方重用
- You have a business rule or a code that is crucial to the success of how the business flow goes您拥有对于成功实现业务流程至关重要的业务规则或代码
- It is a product or project with a long life它是使用寿命长的产品或项目
- It is an open-source project with contributors这是一个有贡献者的开源项目
Note: How can you tell when it’s a logic code or not? Logic, most of the time, is pure Javascript — that is, if you are accessing the DOM, changing classes or animating, this is already the consequence of logic, or, in other words, it is a side effect of a decision. Usually, logical functions are composed of conditionals (if-else, switch) and loops.
注意:您怎么知道什么时候是逻辑代码? 逻辑在大多数情况下都是纯Javascript,也就是说,如果您访问DOM,更改类或设置动画,这已经是逻辑的结果,或者换句话说,这是决策的副作用。 通常,逻辑功能由条件(if-else,switch)和循环组成。
优点 (Pros)
Bugs are found faster
发现错误的速度更快
Run-time errors are treated preventively
运行时错误得到预防
More confidence in deploys
对部署更有信心
It makes it easier the use of refactor (rewriting functions while keeping the same inputs and outputs)
它使重构的使用更加容易(重写函数,同时保持相同的输入和输出)
缺点 (Cons)
- More time spent in developing开发上花费了更多时间
- More time spent with the project在项目上花费更多的时间
It is difficult to rewrite. Unlike the refactor, if a function or module has been poorly designed and needs to be redone, the test will need to be rewritten as well
很难重写。 与重构不同,如果功能或模块的设计不良,并且需要重做,则测试也需要重写
工具类(Tools)
覆盖范围(Coverage)
This is a controversial point. When using unit tests as a synonym for more stability and security, it is very common to compromise or require some “coverage”, usually measured in percentage. At first, the more of your Javascript files, functions, lines, conditions — in short, the more operation is covered by tests, the better. But there is “too much testing”. A happy medium would be to manually choose critical files, with a business rule or logic, and measure their coverage, instead of doing that with all files.
这是一个有争议的观点。 当使用单元测试作为更多稳定性和安全性的代名词时,折衷或要求一定的“覆盖率”是很常见的,通常以百分比来衡量。 首先,您的Javascript文件,函数,行,条件越多—简而言之,测试覆盖的操作越多,效果越好。 但是有“太多的测试”。 一个不错的方法是手动选择具有业务规则或逻辑的关键文件,并衡量其覆盖范围,而不是对所有文件都这样做。
积分(Integration)
它是什么(What is it)
There are a lot of gifs on the internet exemplifying the difference between unit and integration tests. It basically means testing units together. While in unit tests it is often necessary to mock (simulate data locally, removing the need for external integrations) parameters, data and dependencies, in the integration test the “real units” are used as much as possible.
互联网上有很多gif图像,说明了单元测试和集成测试之间的区别。 从根本上讲,这意味着一起测试单元。 在单元测试中,通常需要模拟(本地模拟数据,无需外部集成)参数,数据和相关性,而在集成测试中,尽可能使用“真实单元”。
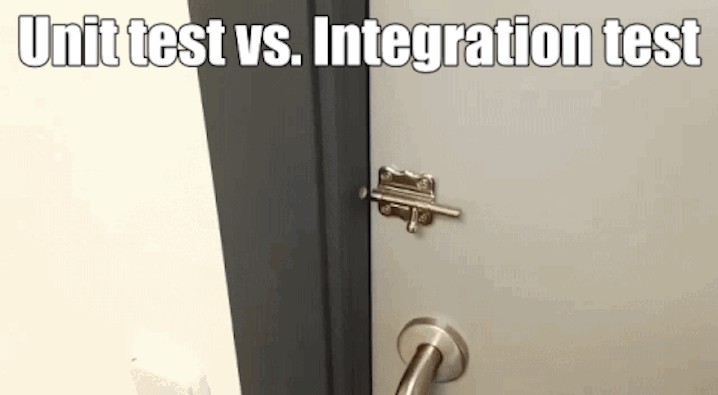
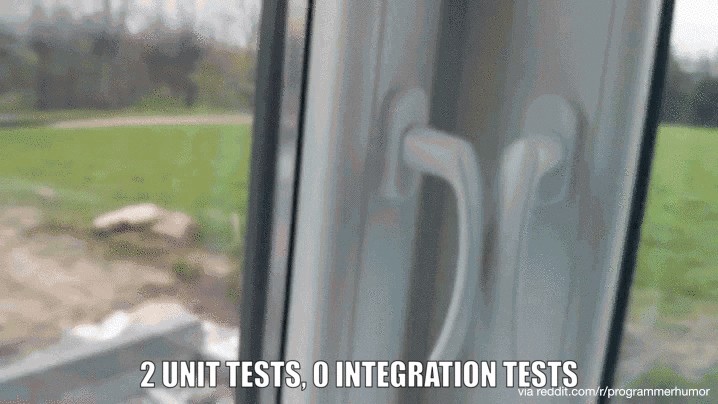
Here’s an example, in the case of dates. You would probably have at least one function to format dates and another to format time (hours of the day). And here’s the question: would a simple integration test be sufficient to verify what would happen, for example, if the date and time formats are different — even if the date and time formatting works in isolation? Will the output be the date in the format of a specific country and will the time be in the format of another one — or is that an exception?
这是一个有关日期的示例。 您可能至少具有一个用于格式化日期的函数,以及另一个用于格式化时间(一天中的小时)的函数。 问题是:简单的集成测试是否足以验证发生的事情,例如,日期和时间格式是否不同-即使日期和时间格式是单独工作的? 输出将是特定国家/地区格式的日期,还是时间将是另一个国家/地区的格式-还是例外?
什么时候使用 (When to use it)
The word “integration” in this context means a lot. Basically, these tests aim to answer the question that still remains: how do two units tested together work together? However, in this case, unity can have several meanings:
在本文中,“整合”一词意义重大。 基本上,这些测试旨在回答仍然存在的问题:两个测试单元如何一起工作? 但是,在这种情况下,统一可以具有多种含义:
To test two or more functions, when one is used by the other and the unit test was solved by the mock
为了测试两个或多个函数,当一个函数被另一个函数使用并且单元测试由模拟解决时
To test functions or modules that use external dependencies (like NPM packages) and the unit test was solved by mock
测试使用外部依赖项的功能或模块(例如NPM软件包),并通过模拟解决了单元测试
To connect APIs or external services to tests that were previously mocked
将API或外部服务连接到先前模拟的测试
On microservice architectures, to test functions or modules from different repositories that work together
在微服务架构上,测试可一起工作的不同存储库中的功能或模块
优点(Pros)
- It provides you even more security in the application, mainly as a validation before deploying它为您提供了更多的应用程序安全性,主要是作为部署之前的验证
- It quickly warns you of any failures as a result of a refactor, either from your own code or from the others, which otherwise might only be caught in production它会Swift警告您因重构而导致的任何失败,无论是您自己的代码还是其他代码,否则可能只会在生产中出现
缺点(Cons)
- Depending on the stack and the architecture of the project, it might take some considerable setup time, specially when provisioning instances in the cloud, including setup and teardown services根据项目的堆栈和体系结构,可能会花费一些可观的设置时间,尤其是在云中设置实例(包括设置和拆卸服务)时
- It can be slow, increasing the build time considerably它可能很慢,大大增加了构建时间
工具类(Tools)
The same as for unit tests, but depending on the architecture of the project you may need to run a browser to access some specific functionalities.
与单元测试相同,但是根据项目的体系结构,您可能需要运行浏览器才能访问某些特定功能。
端到端 (End-to-end)
它是什么(What is it)
It is what the name says: “from end to end”. We are talking about “applications”, which have data, logic and interface, so “end-to-end” tests must encompass all three. They are focused on the interface as a way to automatically test behaviors, interactions and flows, whether they contain data and logic or not.
顾名思义,它是“从头到尾”。 我们谈论的是具有数据,逻辑和接口的“应用程序”,因此“端到端”测试必须包含所有这三个。 它们专注于界面,可以自动测试行为,交互和流,无论它们是否包含数据和逻辑。
An example: through this option, it would be to possible to automate an interface test in which the user accesses a calendar and chooses dates, and in another column he/she would be able to choose the hours of the day, attesting that the correct options are shown, that the success and error messages appear and so on.
一个示例:通过该选项,可以自动化界面测试,使用户可以访问日历并选择日期,在另一列中,他/她将可以选择一天中的小时,以证明正确显示选项,显示成功和错误消息,依此类推。
什么时候使用 (When to use it)
Whenever you and your team find yourselves performing the same interface test dozens of times and there is time to schedule automated tests. The most important question that defines whether this is the case or not is: how long will these tests continue to be done, or how long will this project take? If the answer is at least a few months, it is certainly the case.
每当您和您的团队发现自己执行相同的接口测试数十次时,就有时间安排自动测试。 定义是否是这种情况的最重要的问题是:这些测试将继续执行多长时间,或者该项目需要多长时间? 如果答案至少是几个月,那就一定是这样。
优点 (Pros)
- It shortens considerably the time you spend doing manual tests它大大缩短了您进行手动测试的时间
- If configured correctly, it makes it easier to find cross-browser divergences如果配置正确,则可以更轻松地发现跨浏览器的差异
缺点(Cons)
- It demands considerable time to schedule tests安排测试需要大量时间
Drivers, browsers headless and different browsers have an unstable behavior when it comes to automated tests, leading to many exceptions that must be skipped and retested manually
驱动程序,无头浏览器和其他浏览器在进行自动测试时会出现不稳定的行为,从而导致许多异常必须手动跳过并重新测试
工具类(Tools)
Connect with: Browserstack
连接: Browserstack
Connect with: AWS Device Farm
连接: AWS设备场
回归(Regression)
它是什么(What is it)
All integration and end-to-end (unit) tests have much more value when monitored over time. It is possible to configure them to use a log that clearly shows what has been successful or failed. If you are on a well-structured project, it is possible that there are dedicated QA professionals — and, in this case, they are probably doing exploratory manual tests as well, and writing down the test results over time.
随着时间的推移,所有集成和端到端(单元)测试的价值都将大大提高。 可以将它们配置为使用清楚显示成功或失败内容的日志。 如果您是一个结构良好的项目,则可能有专门的QA专业人员-在这种情况下,他们可能也在进行探索性的手动测试,并随着时间的推移写下测试结果。
While the uses mentioned above focus primarily on the layer of data and the logic of an application, there is another use, perhaps one of the most useful for large-scale projects. For example, to observe automatically the interface differences during the lifetime of a product or project, using past references. This is extremely useful in a project with hundreds or thousands of pages, since it would take several days or weeks to certify that everything is okay if you check it manually. So it is valid to use regression testing.
尽管上述用途主要集中在数据层和应用程序逻辑上,但还有另一种用途,也许是对大型项目最有用的用途。 例如,使用过去的引用自动观察产品或项目生命周期中的界面差异。 这在具有成百上千个页面的项目中非常有用,因为如果您手动检查所有内容,则需要几天或几周的时间来证明一切正常。 因此,使用回归测试是有效的。
什么时候使用 (When to use it)
In complex software where there is a well-used design system, that is, a component system with some consistent design rules. In such systems, it is possible to infer through automated scripts that changes that are poorly made in design are likely to be a break in the design system. In addition, it is possible to have too many pages to remember, especially when considering all multiple releases and timelines.
在具有良好使用的设计系统的复杂软件中,即具有一些一致设计规则的组件系统。 在这样的系统中,有可能通过自动脚本推断出设计不善的更改很可能会破坏设计系统。 此外,可能有太多页面需要记住,尤其是在考虑所有多个发行版和时间表时。
优点 (Pros)
- It can help you to ensure that new releases conform to the previous ones that were successful.它可以帮助您确保新版本符合以前成功的版本。
缺点 (Cons)
- It requires end-to-end testing to be effective.它要求端到端测试有效。
- It requires considerable setup time. 这需要大量的设置时间。
- Depending on your compatibility matrix, it may not remove the need for excessive manual testing. 根据您的兼容性矩阵,它可能不会消除对过多手动测试的需求。
- There is no perfect solution yet, since it’s problematic to deal with responsiveness, threshold proportionality and screenshots of all possible interactions. 目前还没有完美的解决方案,因为处理响应性,阈值比例和所有可能的交互的屏幕截图是有问题的。
工具类 (Tools)
Note: In addition to the tests mentioned above, there are also specific test tools for you to use with a framework, such as Protractor from Angular and Enzyme for React.
注意:除了上述测试外,还有一些特定的测试工具可用于框架,例如Angular的Protractor和React的Enzyme。
Tests are a whole different world and there are many new challenges. It is a very old and popular subject in software development, mainly in the Back-End, but it is recent in the Front-End debate, especially in Brazil. More and more companies request this knowledge, even though it is still very rare! Thus, it is a good way for you to stand out in the job market.
品鉴是一个完全不同的世界,并且存在许多新的挑战。 它是软件开发中非常古老且流行的主题,主要是在后端,但是在前端辩论中却是最近的,尤其是在巴西。 尽管仍然很少,但是越来越多的公司要求获得这种知识! 因此,这是您在就业市场上脱颖而出的好方法。
If you are interested and don’t know where to start, send me a message on Linkedin, I will be happy to help you with content references.
如果您有兴趣并且不知道从哪里开始,请在Linkedin上给我发送消息,我将很乐意为您提供内容参考。
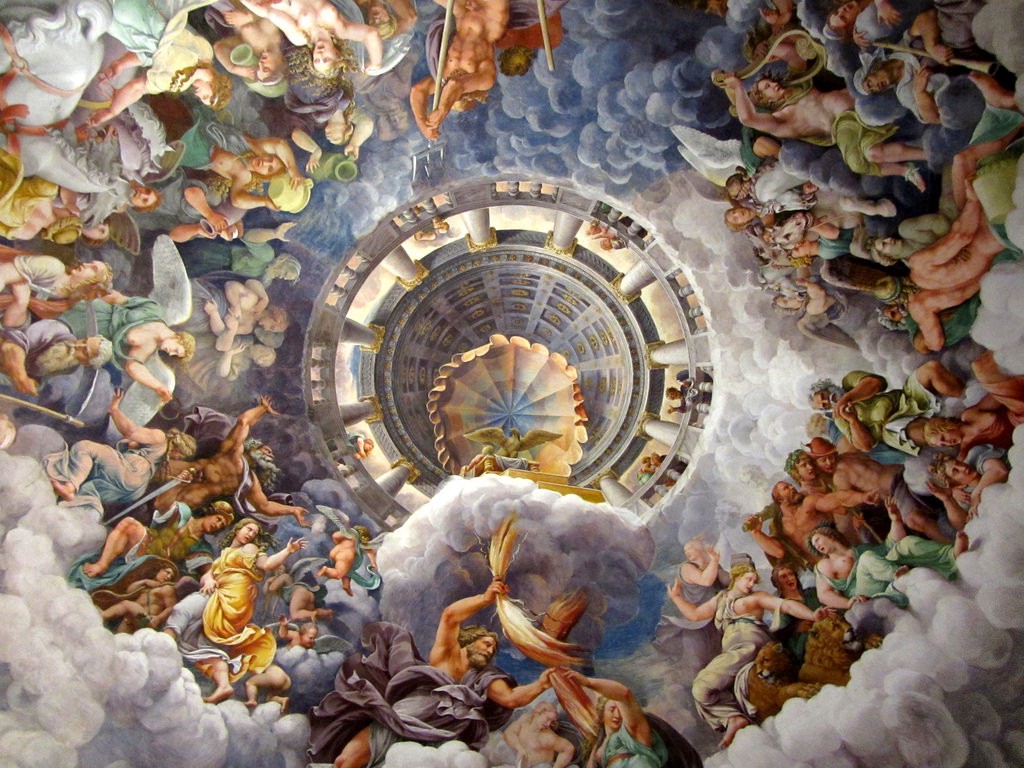
翻译自: https://medium.com/swlh/front-end-architecture-tests-edad10f4a8a4
前端架构 从入门到微前端