If you are building an app that revolves around maps and locations, you might need screens where you mark a particular location/coordinate. While it’s easy for iOS, things can get slightly messy for Android.
如果要构建围绕地图和位置旋转的应用程序,则可能需要在屏幕上标记特定的位置/坐标。 虽然对于iOS而言很容易,但对于Android而言,情况可能会有些混乱。
I have compiled an example on how you can mark a location with a custom marker such that it works perfectly for both iOS and Android platforms.
我整理了一个示例,说明如何使用自定义标记标记位置,以使其完美适用于iOS和Android平台。
主屏幕 (Main Screen)
First, create a main screen where you will place your MapContainer
or any other buttons.
首先,创建一个主屏幕,您将在其中放置MapContainer
或任何其他按钮。
MapContainer (MapContainer)
Next, create another component, which will be your map container. This will contain a few sub-components.
接下来,创建另一个组件,它将成为您的地图容器。 这将包含一些子组件。
MapboxGL.MapView (MapboxGL.MapView)
The map is created and held inside the MapView. Note that the Id
field needs to be a string.
将创建地图并将其保存在MapView中。 请注意, Id
字段必须为字符串。
MapboxGL.Camera (MapboxGL.Camera)
With a Camera, you basically zoom into the coordinate and display a zoomed version of your map on the screen. The zoomLevel
and animationDuration
can be adjusted as per the requirement.
使用相机,您基本上可以放大坐标并在屏幕上显示地图的缩放版本。 可以根据需要调整zoomLevel
和animationDuration
。
MapboxGL.MarkerView (MapboxGL.MarkerView)
The MarkerView
is where we will place our custom marker. Now this is something that can cause problems on Android. In order to avoid that, keep these things in mind:
MarkerView
是放置自定义标记的地方。 现在,这可能会在Android上引起问题。 为了避免这种情况,请记住以下几点:
Make sure your
AnnotationContent
is wrapped under a view.确保您的
AnnotationContent
包装在视图下。Make sure your
MarkerView
itself is wrapped under a view.确保
MarkerView
本身包装在视图下。- The styling will often differ in Android. Try writing and testing a code that seems appropriate on both platforms. 在Android中,样式通常会有所不同。 尝试编写和测试在两个平台上都合适的代码。
注释内容 (AnnotationContent)
This is what will be displayed as a marker. Even if it’s a single component (for example just an icon or just text), make sure it’s wrapped in a <View>
. The <Icon>
I have used it from FontAwesome
and works perfectly but you can also use custom images (eg: an svg image) by adding an <Image>
component.
这将显示为标记。 即使是单个组件(例如,仅是图标或文本),也请确保将其包装在<View>
。 我已经从FontAwesome
使用了<Icon>
它可以完美工作,但是您也可以通过添加<Image>
组件来使用自定义图像(例如svg图像)。
地址文本框 (AddressTextBox)
Now this is something optional. If you want to place an additional item on top of your map, you can do something like this. Note that according to MapboxGL’s team, it’s not possible to render an additional <View>
inside the <MapView>
. In my case, it worked in iOS, but not in Android.
现在这是可选的。 如果要在地图上放置其他项目,可以执行以下操作。 请注意,根据MapboxGL的团队,不可能在<MapView>
内渲染其他<View>
<MapView>
。 就我而言,它可以在iOS中使用,但不能在Android中使用。
Hence, you have to place it outside the <MapView>
and give its parent container a position: ‘absolute’
.
因此,您必须将其放置在<MapView>
之外,并为其父容器指定一个position: 'absolute'
。
The result will look somewhat like this. The text box at the top comes from the AddressTextBox
while the marker icon and the smaller text box come from AnnotationContent
结果将看起来像这样。 顶部的文本框来自AddressTextBox
而标记图标和较小的文本框来自AnnotationContent
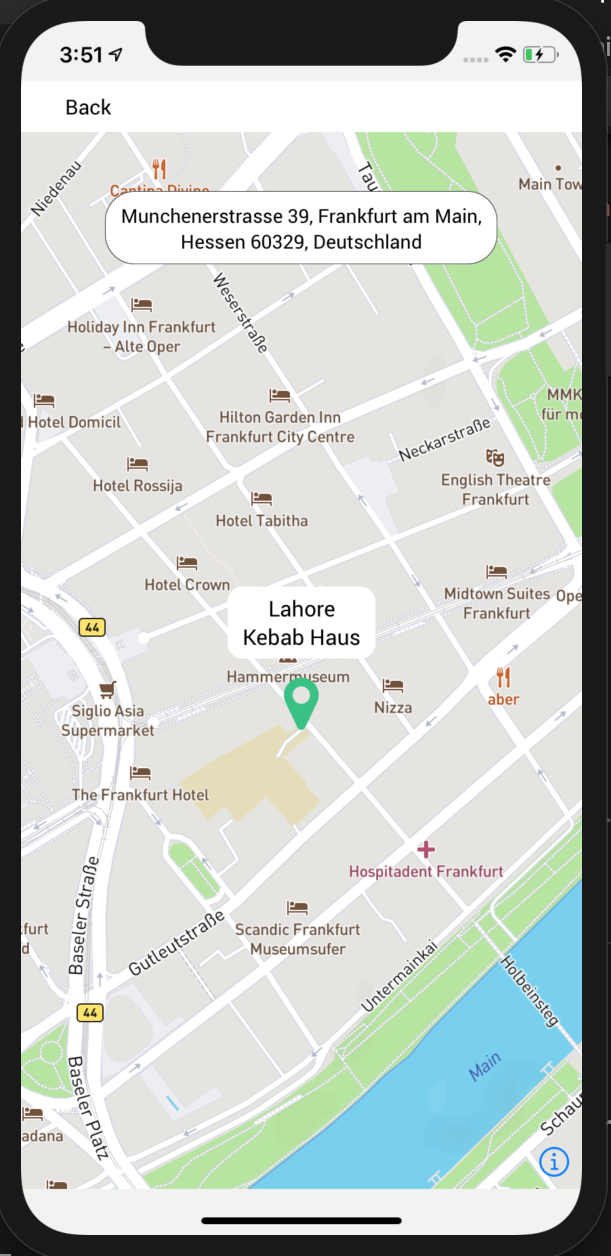
MarkerView与PointAnnotation与ShapeSource (MarkerView vs PointAnnotation vs ShapeSource)
According to Miklós Fazekas from MpaboxGL’s team, on iOS, PointAnnotation is more or less the same as MarkerView. However, on Android, it’s emulated with ShapeSource + SybolLayer. In Android, the child React Native View is captured into a static image and then displayed. So, for instance, you cannot place a button as a PointAnnotation’s child on Android.
根据MpaboxGL团队的MiklósFazekas的说法,在iOS上,PointAnnotation与MarkerView大致相同。 但是,在Android上,它是通过ShapeSource + SybolLayer模拟的。 在Android中,子React Native View被捕获为静态图像,然后显示出来。 因此,例如,您不能在Android上将按钮作为PointAnnotation的子级放置。
One good thing about PointAnnotations is that they also also support callouts. A <MapboxGL.Callout>
component will allow you to click on an annotation and see a text box pop up.
PointAnnotations的优点之一是它们还支持标注。 <MapboxGL.Callout>
组件将允许您单击注释,并看到一个弹出的文本框。
如何查找位置的坐标 (How to Find Coordinates for a Location)
If for some reason, you’re unable to use Mapbox here, you can also use Google Maps. Simply open a location on Google Maps, right click on it and select “What’s here”. You’ll see the exact coordinates for that location. You can copy the coordinates and use them in your code.
如果由于某种原因您无法在此处使用Mapbox,也可以使用Google Maps。 只需在Google地图上打开一个位置,右键单击它,然后选择“这里是什么”即可。 您会看到该位置的确切坐标。 您可以复制坐标并在代码中使用它们。
Note that coordinates need to be in form of an array. For example:
请注意,坐标必须采用数组形式。 例如:
centerCoordinate={[8.5574, 50.7891]}
centerCoordinate={[8.5574, 50.7891]}
Hope this helps!
希望这可以帮助!
普通英语JavaScript (JavaScript In Plain English)
Did you know that we have three publications and a YouTube channel? Find links to everything at plainenglish.io!
您知道我们有三个出版物和一个YouTube频道吗? 在plainenglish.io上找到所有内容的链接!