js 子节点父节点兄弟节点
Node.js is great for reusing code — and the backbone of reusing code is NPM packages.
Node.js非常适合重用代码-重用代码的基础是NPM软件包。
NPM packages save us tons of time and effort. Need a date library? There’s a package for it. Need a utility library? No problem, just install the package. Whenever you need to solve a problem with code, the chances are there’s a package tailored to your needs.
NPM软件包节省了我们大量的时间和精力。 需要日期库吗? 有一个包装。 需要实用程序库吗? 没问题,只需安装软件包即可。 每当您需要解决代码问题时,都有机会根据您的需求量身定制一个软件包。
Here’s a list of packages I think every Node.js developer should know. Treat these NPM packages as time savers and magic fairy helpers.
这是我认为每个Node.js开发人员都应该知道的软件包列表。 将这些NPM软件包视为节省时间和魔术仙子的助手。
沙哑 (husky)
Husky makes it straightforward to implement git hooks. Work with a team and want to enforce coding standards across the team? No problem! Husky lets you require everyone to automatically lint and tests their code before committing or pushing to the repository.
Husky使实现git挂钩变得简单明了。 与团队合作,并希望在整个团队中实施编码标准? 没问题! 赫斯基(Husky)使您要求所有人在提交或推送到存储库之前自动完成测试并测试其代码。
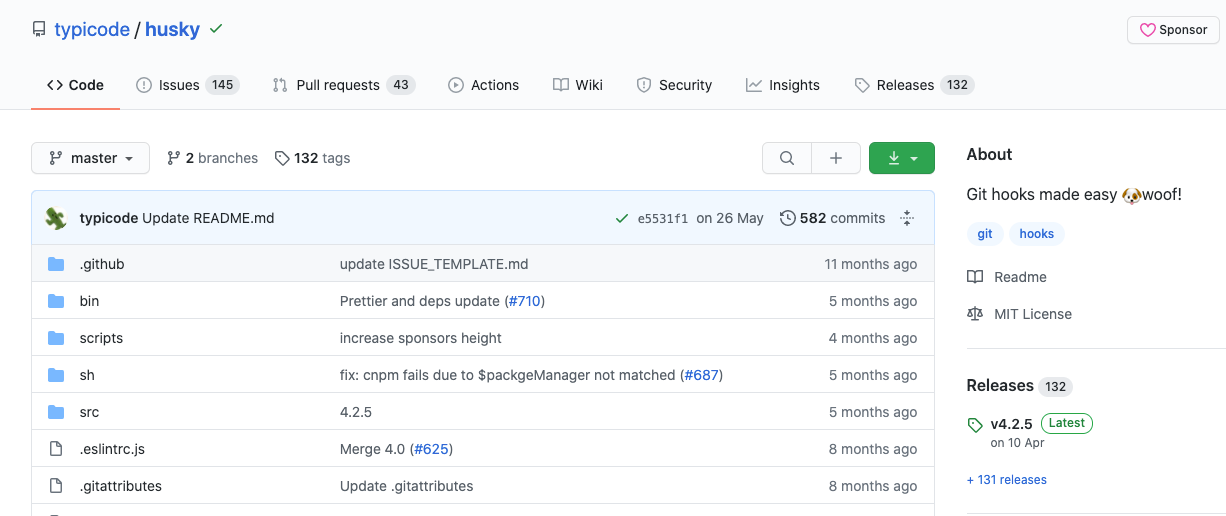
如何安装 (How to install)
yarn add husky
用法 (Usage)
Here’s an example of how you can implement husky hooks:
这是一个如何实现沙哑的钩子的示例:
The pre-commit
hooks will run before you commit to the repository.
pre-commit
挂钩将在您提交到存储库之前运行。
The pre-push
hook runs before you push the code to the repository.
在将代码推送到存储库之前,将运行pre-push
挂钩。
Dotenv (dotenv)
Dotenv is a zero-dependency module that loads environment variables from a
.env
file intoprocess.env
. Storing configuration in the environment separate from code is based on The Twelve-Factor App methodology.Dotenv是一个零依赖模块,可将环境变量从
.env
文件加载到process.env
。 将配置与代码分开存储在环境中是基于“十二因子应用程序”方法的。
如何安装 (How to install)
yarn add dotenv
用法 (Usage)
As early as possible in your application, require and configure dotenv:
尽早在您的应用程序中,要求并配置dotenv:
require('dotenv').config()
Create a .env
file in the root directory of your project. Add environment-specific variables on new lines in the form of NAME=VALUE
. For example:
在项目的根目录中创建一个.env
文件。 在新行上以NAME=VALUE
的形式添加特定于环境的变量。 例如:
DB_HOST=localhostDB_USER=rootDB_PASS=s1mpl3
process.env
now has the keys and values you defined in your .env
file:
process.env
现在具有您在.env
文件中定义的键和值:
const db = require('db')db.connect({ host: process.env.DB_HOST, username: process.env.DB_USER, password: process.env.DB_PASS})
日期-fns (date-fns)
Date-fns is like lodash, but for dates. It includes many utility functions that make it easier to work with dates.
Date-fns就像lodash,但用于日期。 它包含许多实用程序功能,这些功能使处理日期变得更加容易。
date-fns provides the most comprehensive, yet simple and consistent toolsetfor manipulating JavaScript dates in a browser & Node.js.
date-fns提供最全面,最简单且一致的工具集,用于在浏览器和Node.js中处理JavaScript日期。

如何安装 (How to install)
yarn add date-fns
用法 (Usage)
Here’s a quick sample of the date-fns library:
这是date-fns库的快速示例:
import { compareAsc, format } from 'date-fns'
format(new Date(2014, 1, 11), 'yyyy-MM-dd')
//=> '2014-02-11'
const dates = [
new Date(1995, 6, 2),
new Date(1987, 1, 11),
new Date(1989, 6, 10),
]
dates.sort(compareAsc)
//=> [
// Wed Feb 11 1987 00:00:00,
// Mon Jul 10 1989 00:00:00,
// Sun Jul 02 1995 00:00:00
// ]
Check out the documentation for further examples and use cases.
查看文档以获取更多示例和用例。
本扬 (Bunyan)
Bunyan is an easy-to-grasp and performant JSON logging library for Node.
Bunyan是Node的易于掌握且性能卓越的JSON日志记录库。

如何安装 (How to install)
yarn add bunyan
Tip: The bunyan
CLI tool is written to be compatible (within reason) with all versions of Bunyan logs. Therefore you might want to yarn add global bunyan
to get the Bunyan CLI on your PATH, then use local Bunyan installs for node.js library usage of Bunyan in your apps.
提示: bunyan
CLI工具被编写为与所有版本的Bunyan日志兼容(在一定程度上)。 因此,您可能希望yarn add global bunyan
以在您的PATH上获取Bunyan CLI,然后将本地Bunyan安装用于应用程序中Bunyan的node.js库使用。
用法 (Usage)
Bunyan is a simple and fast JSON logging library for node.js services.
Bunyan是用于node.js服务的简单快速的JSON日志记录库。
// hi.jsconst bunyan = require('bunyan');const log = bunyan.createLogger({name: "myapp"});log.info("hi");
Here’s what’s being returned to the console if you run node hi.js
.
如果您运行node hi.js
这就是返回控制台的node hi.js
。

拉姆达 (Ramda)
Rambda is a practical, functional, utility library for JavaScript programmers. Ramda emphasizes a purer functional style.
Rambda是一个面向JavaScript程序员的实用,实用的实用程序库。 Ramda强调纯正的功能风格。
Immutability and side-effect free functions are at the heart of Ramda’s design philosophy. This can help you get the job done with simple, elegant code.
不变性和无副作用的功能是Ramda设计理念的核心。 这可以帮助您使用简单,优雅的代码完成工作。
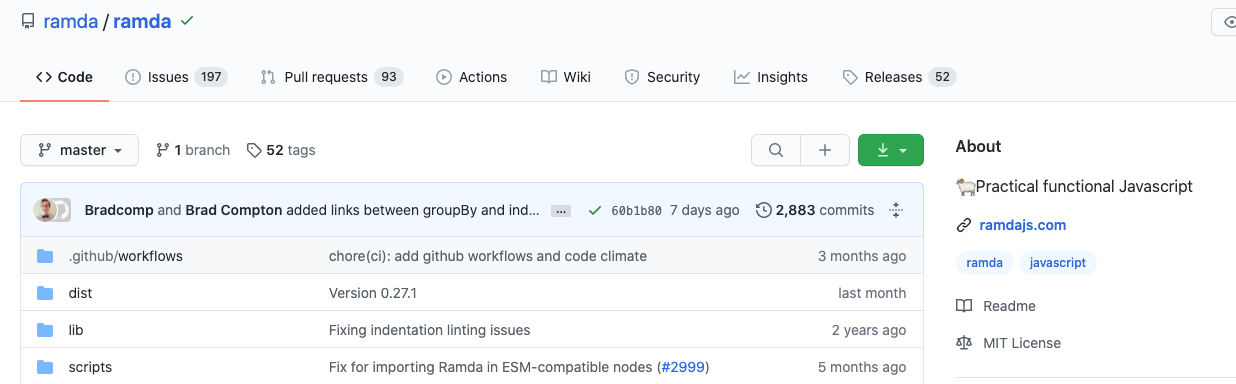
如何安装 (How to install)
$ yarn add ramda
用法 (Usage)
import * as R from 'ramda'const greet = R.replace('{name}', R.__, 'Hello, {name}!'); greet('Alice'); //=> 'Hello, Alice!'
调试 (debug)
Debug is a tiny JavaScript debugging utility modeled after Node.js core’s debugging technique.
Debug是一个小巧JavaScript调试实用程序,其模仿Node.js核心的调试技术。

如何安装 (How to install)
$ yarn add debug
用法 (Usage)
debug
exposes a function — simply pass this function the name of your module and it will return a decorated version of console.error
for you to pass debug statements to.
debug
公开了一个函数—只需将此函数传递给模块名称即可,它将返回经过修饰的console.error
版本,以便您将debug语句传递给该函数。

如何安装 (How to install)
yarn add debug
用法 (Usage)
This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
这将允许您为模块的不同部分以及整个模块切换调试输出。

平面 (flat)
flat takes a nested Javascript object and flattens it. You can also unflatten an object with delimited keys.
flat接受一个嵌套的Javascript对象并将其展平。 您也可以使用分隔键取消展平对象。

安装 (Installation)
$ yarn add flat
用法 (Usage)
const flatten = require('flat')
flatten({
key1: {
keyA: 'valueI'
},
key2: {
keyB: 'valueII'
},
key3: { a: { b: { c: 2 } } }
})
// {
// 'key1.keyA': 'valueI',
// 'key2.keyB': 'valueII',
// 'key3.a.b.c': 2
// }
JSON5 (JSON5)
The JSON5 Data Interchange Format (JSON5) is a superset of JSON that aims to alleviate some of the limitations of JSON by expanding its syntax to include some productions from ECMAScript 5.1.
JSON5数据交换格式(JSON5)是JSON的超集,旨在通过扩展其语法以包含ECMAScript 5.1的某些产品来减轻JSON的某些限制。
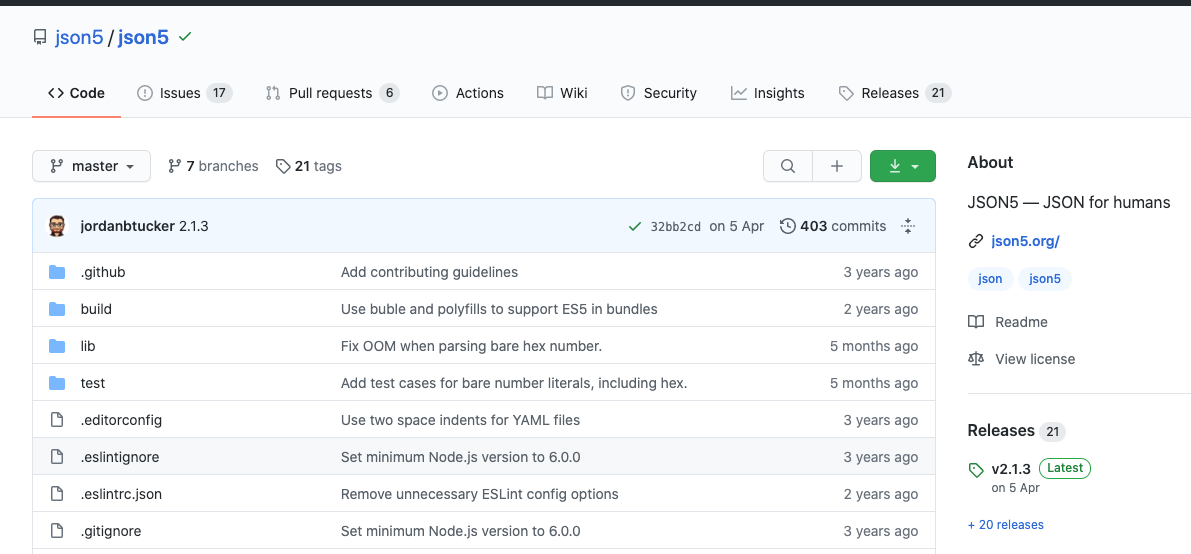
如何安装 (How to install)
yarn add json5
const JSON5 = require('json5')
用法 (Usage)
Notice the file extension. JSON5 is an extension and superset of JSON.
注意文件扩展名。 JSON5是JSON的扩展和超集。
ESLint (ESLint)
ESLint is a wonderful tool for avoiding bugs and forcing coding standards for development teams. ESLint is a tool for identifying and reporting on patterns found in ECMAScript/JavaScript code.
ESLint是一个很好的工具,可以避免错误并强制开发团队使用编码标准。 ESLint是用于识别和报告ECMAScript / JavaScript代码中的模式的工具。

如何安装和使用 (How to install and use)
$ yarn add eslint
You should then set up a configuration file:
然后,您应该设置一个配置文件:
$ ./node_modules/.bin/eslint --init
After that, you can run ESLint on any file or directory like this:
之后,您可以在任何文件或目录上运行ESLint,如下所示:
$ ./node_modules/.bin/eslint yourfile.js
For further explanations, please refer to the official documentation. There are lots of examples of getting started and configuration.
有关更多说明,请参阅官方文档 。 有许多入门和配置示例。
下午2 (PM2)
PM2 is a production process manager for Node.js applications with a built-in load balancer. It allows you to keep applications alive forever, to reload them without the downtime, and to facilitate common system admin tasks.
PM2是具有内置负载平衡器的Node.js应用程序的生产过程管理器。 它使您可以使应用程序永远保持活动状态,可以在不停机的情况下重新加载它们,并简化常见的系统管理任务。

安装PM2 (Installing PM2)
$ yarn add global pm2
开始申请 (Start an application)
You can start any application (Node.js, Python, Ruby, binaries in $PATH…) like so:
您可以像这样启动任何应用程序(Node.js,Python,Ruby,$ PATH中的二进制文件……):
$ pm2 start app.js
Your app is now daemonized, monitored, and kept alive forever. More about Process Management.
现在,您的应用程序将被守护,监控并永远保持活动状态。 有关过程管理的更多信息 。
管理应用程式 (Managing Applications)
Once applications are started you can manage them easily. Here’s how you can list all running applications:
一旦启动应用程序,您就可以轻松管理它们。 这是列出所有正在运行的应用程序的方法:
$ pm2 ls

Check out the official documentation for the full list of features and possibilities.
请查阅官方文档 ,以获取功能和可能性的完整列表。
头盔 (Helmet)
The Helmet library helps you with securing your Express apps by setting various HTTP headers. “It’s not a silver bullet, but it can help!”
头盔库可通过设置各种HTTP标头来帮助您保护Express应用程序的安全。 “ 这不是灵丹妙药 ,但可以帮上忙!”

如何安装 (How to install)
yarn add helmet
用法 (Usage)
Helmet is Connect-style middleware, which is compatible with frameworks like Express. (If you need support for Koa, see koa-helmet
.)
头盔是Connect风格的中间件,它与Express等框架兼容。 (如果需要对Koa的支持,请参阅koa-helmet
。)
const express = require("express");const helmet = require("helmet");const app = express();
app.use(helmet());
The top-level helmet
function is a wrapper around 11 smaller middleware. In other words, these two things are equivalent:
顶级helmet
功能是11种较小的中间件的包装。 换句话说,这两件事是等效的:
// This...
app.use(helmet());// ...is equivalent to this:
app.use(helmet.contentSecurityPolicy());
app.use(helmet.dnsPrefetchControl());
app.use(helmet.expectCt());
app.use(helmet.frameguard());
app.use(helmet.hidePoweredBy());
app.use(helmet.hsts());
app.use(helmet.ieNoOpen());
app.use(helmet.noSniff());
app.use(helmet.permittedCrossDomainPolicies());
app.use(helmet.referrerPolicy());
app.use(helmet.xssFilter());
压缩 (compression)
The compression library is a Node.js compression middleware.
压缩库是Node.js压缩中间件。
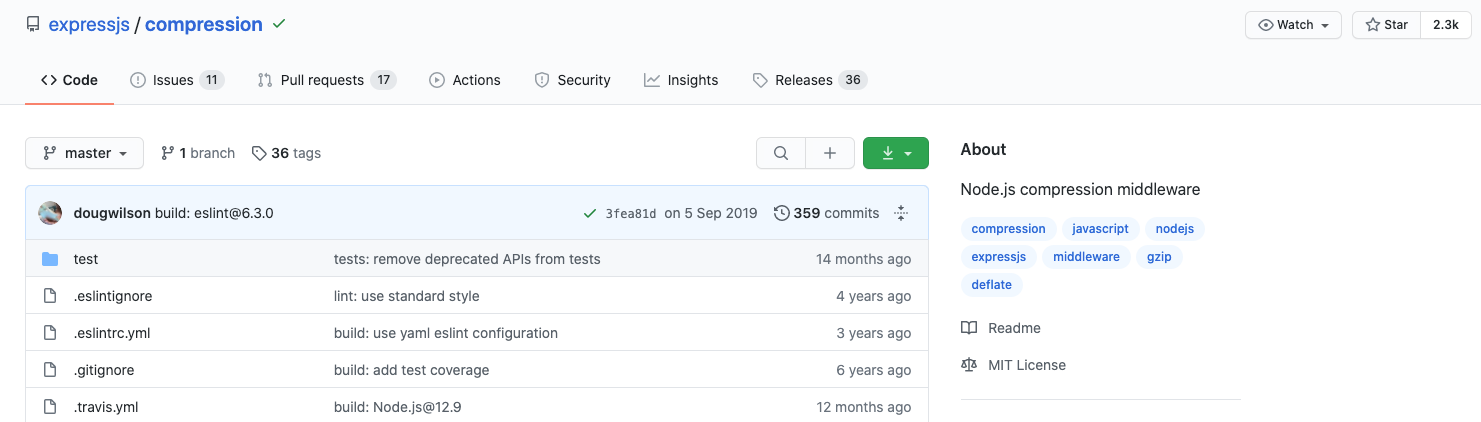
如何安装 (How to install)
$ yarn add compression
用法 (Usage)
When using this module with express or connect, simply call compression with express middleware. Requests that pass through the middleware will be compressed.
当将此模块与Express或Connect一起使用时,只需使用Express中间件调用压缩。 通过中间件的请求将被压缩。
const compression = require('compression')
const express = require('express')const app = express()// compress all responses
app.use(compression())// ...
js 子节点父节点兄弟节点