nodejs react
Real-time web chat application.
实时网络聊天应用程序。
In this article, I’ll be showing you how to build a web chat application using React, Express, socket.io, and Node.js.
在本文中,我将向您展示如何使用React,Express,socket.io和Node.js构建Web聊天应用程序。
The motive behind this application:
此应用程序背后的动机:
Join. Chat. Leave. No Records.
加入。 聊天 离开。 没有记录。
Let’s start with the definition of a chat application.
让我们从聊天应用程序的定义开始。
Chat App is a software that enables the messages to be sent and received between different clients ( or users ).
聊天应用程序是一种软件,它使消息能够在不同的客户端(或用户)之间发送和接收。
Before diving into the chat application, we must understand the basic concepts and frameworks behind the same which are React, socket.io, express.js, and Node.js.
在进入聊天应用程序之前,我们必须了解其背后的基本概念和框架,即React,socket.io,express.js和Node.js。
What is React?
什么是React?
React is an open-source JavaScript library for building user interfaces or UI components. It is maintained by Facebook and a community of individual developers and companies. React can be used as a base in the development of single-page or mobile applications.
React是一个开放源代码JavaScript库,用于构建用户界面或UI组件。 它由Facebook以及由个人开发人员和公司组成的社区维护。 React可以用作开发单页或移动应用程序的基础。
What is socket.io?
什么是socket.io?
Socket.IO is a JavaScript library for realtime web applications. It enables realtime, bi-directional communication between web clients and servers. It has two parts: a client-side library that runs in the browser, and a server-side library for Node.js.
Socket.IO是一个用于实时Web应用程序JavaScript库。 它支持Web客户端和服务器之间的实时双向通信。 它包含两个部分:在浏览器中运行的客户端库和用于Node.js的服务器端库。
What is Express or Express.js?
什么是Express或Express.js?
Express.js, or simply Express, is a web application framework for Node.js, released as free and open-source software under the MIT License. It is designed for building web applications and APIs. It has been called the de facto standard server framework for Node.js.
Express.js(或简称Express)是Node.js的Web应用程序框架,根据MIT许可证作为免费和开源软件发布。 它旨在用于构建Web应用程序和API。 它已被称为Node.js的事实上的标准服务器框架。
What is Node.js?
什么是Node.js?
Node.js is an open-source, cross-platform, JavaScript runtime environment that executes JavaScript code outside a web browser.
Node.js是一个开放源代码,跨平台JavaScript运行时环境,可在Web浏览器之外执行JavaScript代码。
The chat application is divided into two parts:
聊天应用程序分为两个部分 :
Client: This will be responsible for the front end of the application.
客户 :这将负责应用程序的前端。
Server: This will be responsible for the back end portion of the application.
服务器 :这将负责应用程序的后端部分。
Now, that we have a basic idea about the different frameworks and libraries used in this application, let’s start with the development phase.
现在,我们对此应用程序中使用的不同框架和库有了基本的了解,让我们从开发阶段开始。
Quick Note: I have used visual studio for this application, but you can use any editor of your choice
快速说明:我已经为该应用程序使用了Visual Studio,但是您可以使用任何选择的编辑器
开发阶段 (Development Phase)
Create a directory (let’s say “chat-app”) in which we’ll be storing both our client and server directories.
创建一个目录(例如“ chat-app”),在该目录中我们将存储我们的客户端和服务器目录。
In the terminal run the react command:
在终端中运行react命令:
npx create-react-app client
&
和
Create a folder “server” and navigate into it and run this command in the terminal:
创建一个文件夹“服务器”并导航到其中并在终端中运行以下命令:
npm init
After this, your “chat-app” directory should like somewhat like this:
之后,您的“ chat-app”目录应如下所示:
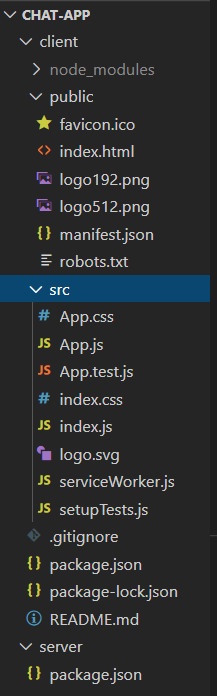
There are some external dependencies which we will be using in this app, so let us install them one-by-one.
我们将在此应用程序中使用一些外部依赖项 ,因此让我们一一安装它们。
In the client terminal, run ( or install ):
在客户端中,运行(或安装):
npm install react-emoji, react-router, react-scroll-to-bottom, socket.io-client
In the server terminal, run ( or install ):
在服务器终端中,运行(或安装):
npm install cors, express, nodemon, socket.io
Quick Note: cors : ( Cross-origin resource ) sharing is a mechanism that allows restricted resources on a web page to be requested from another domain outside the domain from which the first resource was served. A web page may freely embed cross-origin images, stylesheets, scripts, iframes, and videos.
快速说明: cors :(跨域资源)共享是一种机制,它允许从提供第一个资源的域之外的另一个域请求网页上的受限资源。 网页可以自由地嵌入跨域图像,样式表,脚本,iframe和视频。
让我们深入研究应用程序的功能。 (Let’s dive into the functionalities of our application.)
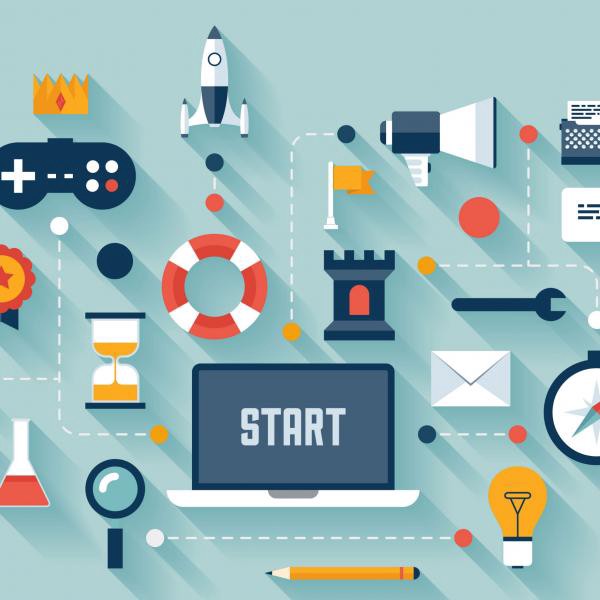
Quick Note: I’ll be explaining only the important functionalities of our chat app.
快速说明:我将仅解释聊天应用程序的重要功能 。
服务器端代码 (Server-Side Code)
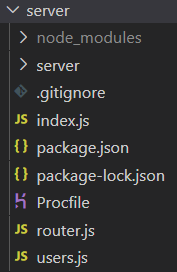
- server/router.js 服务器/router.js
On the server-side, we only need one route to connect to the client-side and the rest will be taken care of by the client-side.
在服务器端,我们只需要一条路由即可连接到客户端 ,其余的将由客户端来处理 。
server/index.js
服务器/ index.js
Here, “io.on(‘connect’, (socket) =>{}” is used for incoming socket connections. Once there is a new connection, then we’ll be attaching different event listeners to the stream.
这里, “ io.on('connect',(socket)=> {}” )用于传入的套接字连接。一旦有了新的连接,我们将在流上附加不同的事件侦听器 。
For example,
例如,
socket.emit(‘message’) is used to greet the user whenever a new user joins the chat room.
每当新用户加入聊天室时,都使用socket.emit('message')向该用户打招呼。
socket.emit(‘sendMessage’) is used to send messages from one client to another client(user).
socket.emit('sendMessage')用于将消息从一个客户端发送到另一个客户端(用户)。
socket.emit(‘disconnect’) is called when a user quits the chat room.
当用户退出聊天室时,将调用socket.emit('disconnect') 。
server/users.js
服务器/users.js
The server adds the new connection to the room (adding a user to the room) and appends the list of users list with the new connection name and assigns a unique id to the user.
服务器将新的连接添加到会议室( 将用户添加到会议室 ),并在用户列表中附加新的连接名称,并为用户分配唯一的ID 。
The server will also check for parameters like whether the new user name is different from all the users in that particular room, if not then the user is asked to enter the room with a different name.
服务器还将检查参数,例如新用户名是否不同于该特定房间中的所有用户,如果不是,则要求用户使用其他名称进入房间。
When a connection leaves the room then the user’s list is updated and the name of that connection(user) is removed. But this does not affect the others chatting the group.
当连接离开会议室时,将更新用户列表,并删除该连接( 用户 )的名称。 但这不影响其他与小组聊天的人。
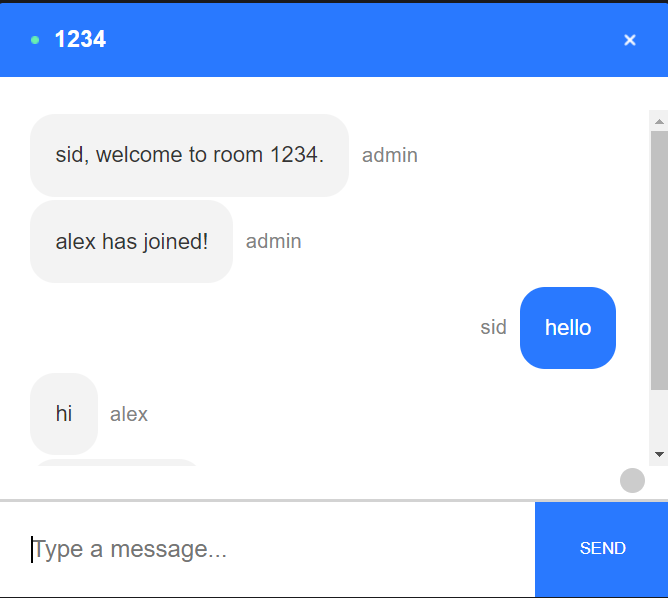
Whenever a user connects to a room, a predefined message greets the user in the room.
每当用户连接到房间时,预定义的消息就会在房间中向用户打招呼。
socket.io basically handles all the functionalities, and help us chat in real-time.
socket.io基本上可以处理所有功能,并帮助我们进行实时聊天。
客户端代码 (Client-Side Code)
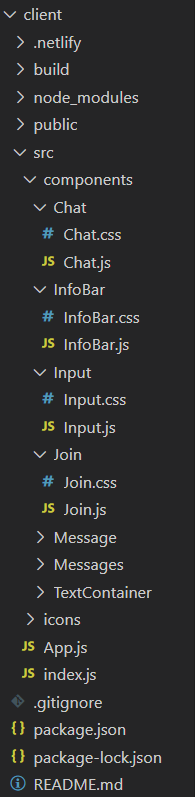
client/src/App.js
客户端/ src / App.js
Two different routes are required in this app, one for joining the chat group and the other being the chat group itself.
此应用程序需要两种不同的路由 ,一种是加入聊天组 ,另一种是聊天组本身 。
client/src/components/Join/Join.js
客户/源代码/组件/连接/Join.js
In this, event.preventDefault() check for any error like if the username already exists or not. If there is no such error then the user is connected to the room he/she wishes to enter ( to={`/chat?name=${name}&room=${room}`} ).
在这种情况下, event.preventDefault()检查是否存在任何错误,例如用户名是否已存在。 如果没有这样的错误,则用户连接到他/她希望进入的房间( to = {`/ chat?name = $ {name}&room = $ {room}`} )。
client/src/components/Chat/Chat.js
客户端/src/components/Chat/Chat.js
This piece of code handles all the incoming messages from different users and enables us to effectively chat in real-time. This also serves as the connection point between client and server. It helps us emitting (displaying) the various message from the users on the screen using rendering.
这段代码处理来自不同用户的所有传入消息,并使我们能够有效地进行实时聊天。 这也用作客户端和服务器之间的连接点 。 它有助于我们使用渲染在屏幕上从用户发出(显示)各种消息。
The rest of the files mainly include the UI of the app and I’m not gonna bore you with that as you can visit my repository regarding it.
其余文件主要包括应用程序的用户界面,我不会对此感到厌烦,因为您可以访问有关它的存储库 。
该应用程序的功能: (Features of the App:)
Multiple users can connect to the same room and chat.
多个用户可以连接到同一个房间并聊天。
The app is based on Snapchat as when you leave the room the messages automatically get deleted.
该应用程序基于Snapchat,因为当您离开会议室时,邮件会自动被删除。
The app allows you to send text messages and emojis ;)
该应用程序允许您发送短信和表情符号 ;)
No duplicate users can join the same room which means no two users with the same name can join the same room.
没有重复的用户可以加入同一个会议室,这意味着没有两个具有相同名称的用户可以加入同一个会议室。
Multiple rooms can function simultaneously.
多个房间可以同时运行。
The app is purely based on JS so none of your chat data is saved anywhere. User privacy is important.
该应用程序完全基于JS,因此您的聊天数据都不会保存在任何地方。 用户隐私很重要 。
结论 (Conclusion)
In this article, I have explained the important modules and their use in building the real-time web chat application. You must be wondering about the final product, if yes then visit the link.
在本文中,我解释了重要的模块及其在构建实时Web聊天应用程序中的用途。 您一定想知道最终产品,如果可以,请访问链接 。
If you require the code then visit my repository on Github(do give a star if you like it).
如果您需要的代码,然后访问我的[R epository在Github上(如果你喜欢这样给一颗星)。
Thanks for reading this article. I hope it’s helpful to you all!
感谢您阅读本文。 希望对您有帮助!
翻译自: https://medium.com/swlh/build-a-chat-app-using-react-express-socket-io-nodejs-32b922c9dd4f
nodejs react