I’m currently working with a nonprofit called SaverLife. Saverlife’s mission is to inspire, inform, and reward the millions of Americans who need help saving money. They give working people the methods and motivation to take control of their financial future,through engaging technologies and strategic partnerships.
我目前正在与一家名为SaverLife的非营利组织合作。 Saverlife的使命是激励,告知和奖励数百万需要帮助以节省金钱的美国人。 他们通过采用技术和战略合作伙伴关系,为劳动者提供了控制财务未来的方法和动力。
SaverLife wants to help their users by better predicting their budgets and upcoming expenses. My team and I are tasked with creating a web application that displays past financial data in the form of visualizations, which are mostly graphs. The graphs clearly show both past spending and transactional history. There is also a feature that helps the user better budget their money using predictive models.
SaverLife希望通过更好地预测他们的预算和即将发生的费用来帮助他们的用户。 我和我的团队的任务是创建一个Web应用程序,以可视化的形式显示过去的财务数据,这些可视化主要是图形。 这些图清楚地显示了过去的支出和交易历史。 还有一个功能可以帮助用户使用预测模型更好地预算资金。
The web application is entirely built using Javascript utilizing React.js library on the front-end and the Node.js platform on the back-end. There are two teams working on this project, a team of web developers and another team of data scientists. I am working as a back-end web developer on the web team.
该Web应用程序完全是使用Javascript构建的,该Javascript使用前端的React.js库和后端的Node.js平台。 有两个团队从事此项目,一个Web开发人员团队和另一个数据科学家团队。 我正在作为Web团队的后端Web开发人员。
My main concern going into this project was how well the two teams would work together cross-functionally since the web application is reliant on receiving the user’s transactional data from the data science API.
我进入该项目的主要关注点在于,由于Web应用程序依赖于从数据科学API接收用户的交易数据,因此两个团队在跨功能的协作中表现得如何。
The specific tasks for the web application were first broken down between the front-end and back-end. I focused in on particular user stories and how the back-end would fulfill those tasks.
该Web应用程序的特定任务首先在前端和后端之间分解。 我专注于特定的用户故事以及后端如何完成这些任务。
For example one user story is “I want to be able to see my past spending habits”. For the back-end that would mean creating a “/spending” endpoint that sends a POST request to the “/spending” Data science API endpoint. The data science API then responds by returning the spending data to the original request made by the front-end.
例如,一个用户故事是“我希望能够看到我过去的消费习惯”。 对于后端,这意味着创建一个“ / spending”端点,该端点将POST请求发送到“ / spending” Data science API端点。 然后,数据科学API会通过将支出数据返回到前端发出的原始请求进行响应。
设置后端端点 (Setting up the Back-end endpoint)
Rendering a visualization on the front-end was one of the web teams minimum requirements. So as a member of the back-end I prioritized setting up a back-end endpoint that the front-end could call to request visualization data. Specifically this visual data would come in as a JSON string that would be parsed on the front-end and plugged into a Plotly.js component.
在Web团队中,最低要求之一就是在前端呈现可视化效果。 因此,作为后端的成员,我优先设置了一个后端端点,前端可以调用该端点来请求可视化数据。 具体来说,这些可视数据将作为JSON字符串输入,该字符串将在前端进行解析并插入Plotly.js组件中。
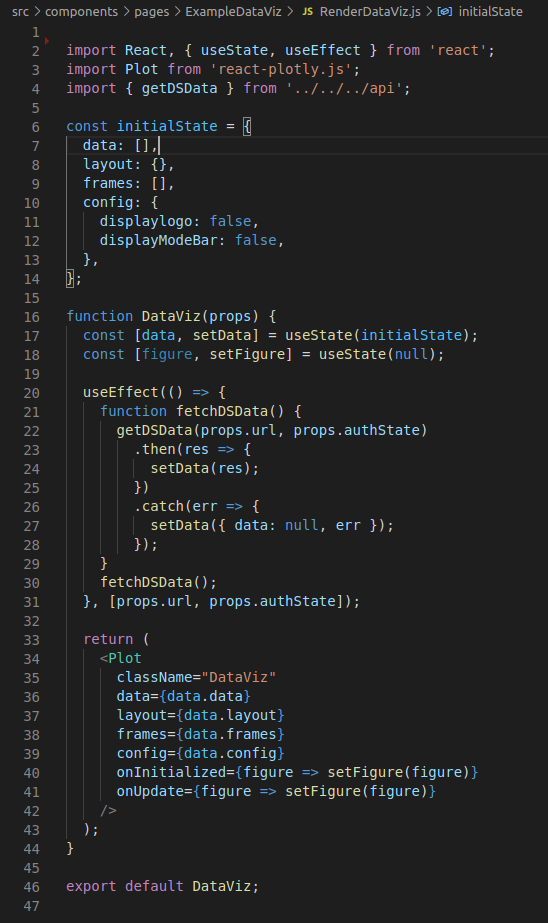
The back-end endpoint is going to request the Plotly.js graph data from the data science API. There are two main reasons behind having the back-end request the data from the data science API instead of the front-end. The first is authentication and authorization routes are built in through the back-end. The second is the back-end could cache the graph data for easier access by the front-end and less server load for the data science API.
后端端点将从数据科学API请求Plotly.js图形数据。 使后端从数据科学API而不是前端请求数据的背后有两个主要原因。 首先是身份验证和授权路由是通过后端内置的。 第二个是后端可以缓存图形数据,以便前端更轻松地访问,并减少数据科学API的服务器负载。
The main issue I ran into early on in the project was not having a data science API endpoint to work with. The data science team needed time to gain access to user data they needed to develop their API endpoints. My solution to this problem was creating a dummy data science endpoint to test my own back-endpoints.The dummy endpoint was just an endpoint that responded with mock graph data in the form of a JSON string when called .
我在项目早期就遇到的主要问题是没有可使用的数据科学API端点。 数据科学团队需要时间来访问开发API端点所需的用户数据。 我对这个问题的解决方案是创建一个虚拟数据科学端点来测试我自己的后端。虚拟端点只是一个以JSON字符串形式响应模拟图数据的端点。
Most of the back-end endpoints make requests to the data science API and then send the response from that request to the front-end. The back-end endpoint does this through a function that makes an HTTP request to the data science API. After the function return with a response it is sent to the front-end which made the initial request to the back-end endpoint.
大多数后端端点都向数据科学API发出请求,然后将来自该请求的响应发送到前端。 后端端点通过向数据科学API发出HTTP请求的功能来执行此操作。 函数返回响应后,将其发送到前端,后端向后端端点发出了初始请求。

The next step, Back-end Data Persistence
下一步,后端数据持久化
Now that the project has working endpoints the next step is creating tables and columns in a database to store data being received from the front-end and the data science team. Graph data and other predictive data from the data science API needs to be stored to be persistent across user log ins. Also the user will be inputting things like their budget goals which should also be saved long term. Redis caching is also something I am looking into in order to cache frequently used data. This would be a benefit to all teams by increasing response time for the front-end and decreasing API calls to the data science team. I look forward to implementing these features soon as I continue to work on this project.
现在项目已经有了工作端点,下一步就是在数据库中创建表和列,以存储从前端和数据科学团队接收到的数据。 需要存储来自数据科学API的图形数据和其他预测性数据,以在用户登录期间保持持久性。 用户还将输入诸如预算目标之类的东西,这些东西也应长期保存。 我也正在研究Redis缓存,以便缓存常用数据。 通过增加前端的响应时间并减少对数据科学团队的API调用,这将对所有团队都有利。 我期待在继续从事该项目时尽快实现这些功能。
翻译自: https://medium.com/@mbidnyk1/connecting-apis-and-web-apps-using-node-js-d2de251d0571