mvc应用程序生命周期
MVC设计模式的背景📚(Background on the MVC Design Pattern📚)
Tightly coupled code can be easy to write but is a huge pain in the A** when trying to scale…
紧密耦合的代码易于编写,但是在尝试扩展时却给A **带来了巨大的痛苦……
- it is hard to work in parallel with other devs in tightly coupled code since there are lots of inter-dependencies. 由于存在许多相互依赖关系,因此很难与其他开发人员在紧密耦合的代码中并行工作。
- it can be difficult to test since the responsibilities of different sections of code are not well separated or defined. 由于代码的不同部分的职责没有很好地分开或定义,因此很难进行测试。
- it makes it harder to change one part of the code without needing to change the rest of the code. 这使得更改一部分代码而不更改其余代码变得更加困难。
The MVC (Model View Controller) is a commonly used design pattern in Applications that have a UI. The main goal of the MVC is to decouple the front-end from the business logic and data store.
MVC(模型视图控制器)是具有UI的应用程序中常用的设计模式。 MVC的主要目标是使前端与业务逻辑和数据存储脱钩。
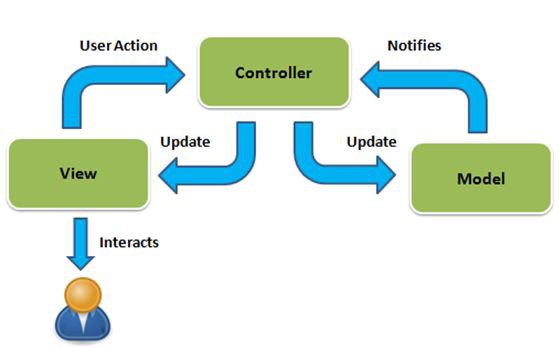
The above diagram is a good example of the workflow with an MVC.
上图是带有MVC的工作流的一个很好的示例。
- User does something on the View (UI) 用户在视图(UI)上执行某些操作
- The View tells the Controller about the action taken视图告诉控制器所采取的措施
- The Controller updates the model控制器更新模型
- The model supplies the new data that is a result of the action该模型提供了作为操作结果的新数据
- The controller updates the view控制器更新视图
⚠️NOTE⚠️: Sometimes in the MVC architecture there is another layer called Service acting between the Model and Controller. This Service layer will handle calling and modifying the Model in the database. In our example, the data will be stored locally so we will not use the Service layer.
注意:有时,在MVC体系结构中,在模型和控制器之间还有另一个称为服务的层。 该服务层将处理在数据库中调用和修改模型。 在我们的示例中,数据将存储在本地,因此我们将不使用服务层。
MVC的具体示例 (Concrete Example of an MVC 💻)
CODING TIME!
编码时间!
In our example, we will have a simple MVC using a dog as our data model.
在我们的示例中,我们将使用狗作为数据模型的简单MVC。
Here is the Model POJO (Plain Old Java Object). We keep it simple with two fields each with a getter and setter and a constructor which takes both the fields.
这是Model POJO(普通的旧Java对象)。 我们通过两个字段使每个字段都具有一个getter和setter以及一个构造函数来简化操作。
模型: (Model:)
Here is the View (instead of a full on html page we will just print out the dog’s name and breed)
这是视图(不是完整的html页面,我们只会打印出狗的名字和品种)
视图: (View:)
This will simply print out the dog’s name and breed.
这将简单地打印出狗的名字和品种。
{name} is a {breed}
Finally, here is the controller, which acts as a translator between the Model and View.
最后,这是控制器,它充当模型和视图之间的转换器。
控制器: (Controller:)
As you can See, our Controller has dependencies on the View and Model and we inject those via the constructor. The methods in the controller delegate to either the model or the view. (There could also be some business logic that takes place for more complicated projects)
如您所见,我们的Controller依赖于View和Model,我们通过构造函数注入它们。 控制器中的方法委托给模型或视图。 (对于更复杂的项目,也可能会发生一些业务逻辑)
Now we will run a program that simulates the webpage loading, the user modifying the data, and then the web page updating.
现在,我们将运行一个程序,该程序模拟网页加载,用户修改数据然后更新网页。
We will get the following output
我们将获得以下输出
Ralph is a Corgi
Spot is a Yorkie
Ta-Da! You have made an MVC to interact with Ralph and the Rest of his friends 😀.
塔达! 您已经制作了一个MVC,可以与Ralph和他的其他朋友interact互动。
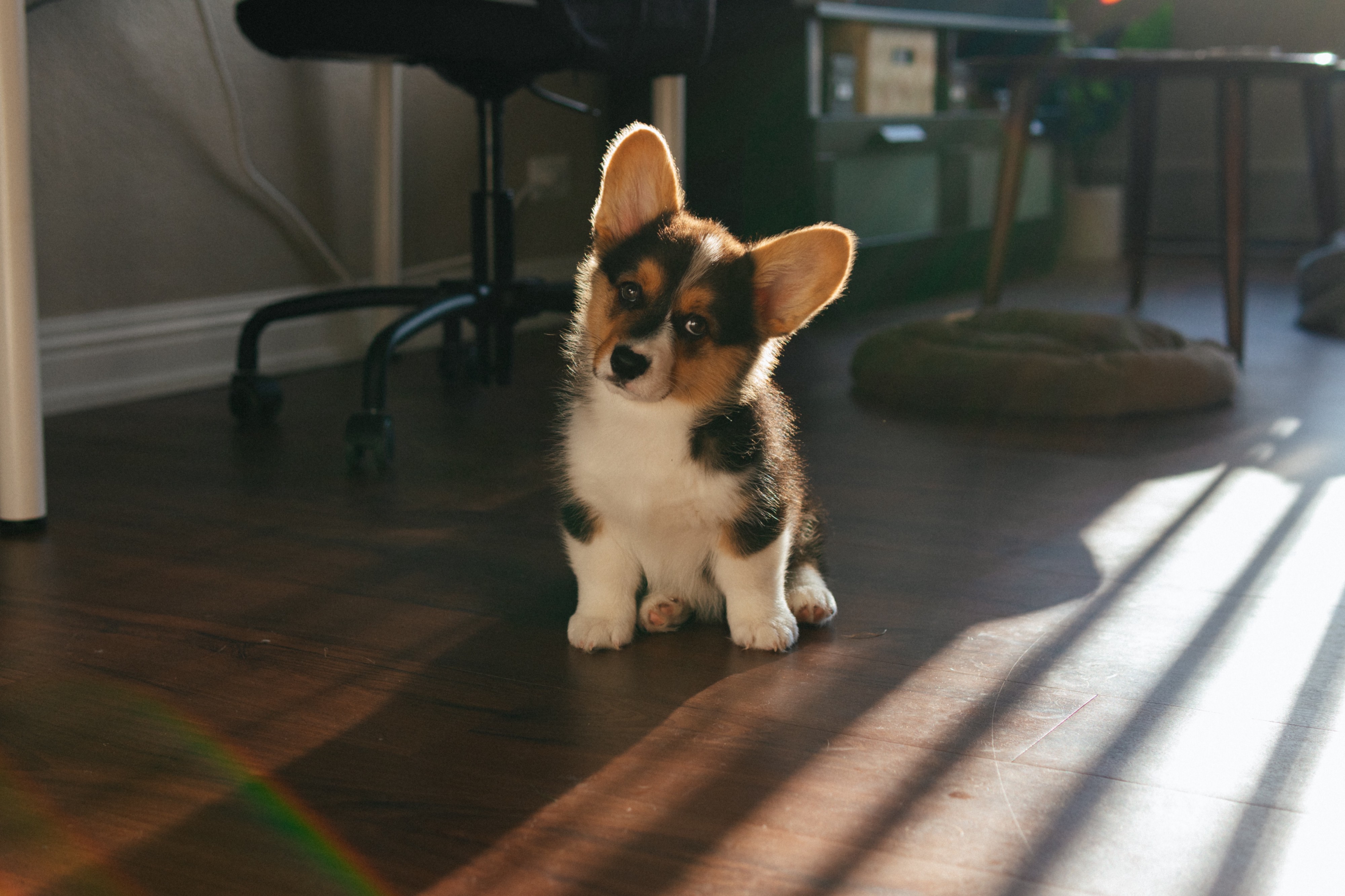
By using this design pattern, we can make the View very simple and decouple a lot of the business logic and data querying away from the frontend.
通过使用这种设计模式,我们可以使View非常简单,并使许多业务逻辑和数据查询与前端分离。
为什么要使用MVC? 🤔 (Why Use MVC? 🤔)
So you know how to implement the MVC design pattern, but why would you use it? It is easier to just call the model from the View, why should you use the controller. I am not going to say you should ALWAYS use MVC because it won’t necessarily be right for every project.
因此,您知道如何实现MVC设计模式,但是为什么要使用它呢? 仅从View调用模型比较容易,为什么要使用控制器。 我不会说您应该始终使用MVC,因为它不一定适用于每个项目。
I WILL give you a list of the pros and cons of using MVC…
我会给你使用MVC的优点和缺点的列表...
优点:👍 (PROS: 👍)
Simultaneous Development — by separating the code into these three sections, we could have developers work on the Model, View, and Controller independently with minimal impact on one another.
同步开发-通过将代码分为这三个部分,我们可以使开发人员独立地进行Model,View和Controller的工作,而彼此之间的影响最小。
Easily Modified — by separating the responsibilities of each section, we make modification easier since we only have to worry about that section.
轻松修改-通过分开每个部分的职责,我们使修改变得更容易,因为我们只需要担心该部分。
Better Testing — with better defined boundaries, we can easily test each section individually.
更好的测试-具有更好定义的边界,我们可以轻松地分别测试每个部分。
缺点:👎 (CONS: 👎)
Complicated to write — MVC architecture has a learning curve and will take longer to write than just hacking an app together.
编写很复杂-MVC架构具有学习曲线,并且比将应用程序一起黑客入侵需要更长的时间。
Boilerplate Code — for a small project, the MVC requires a lot of boilerplate code, for example in the dog example, we could achieve the same end result with just a Model and a view and keep the code relatively clean.
样板代码—对于一个小型项目,MVC需要大量样板代码,例如在dog示例中,我们可以仅使用模型和视图来达到相同的最终结果,并使代码保持相对干净。
In short, if you are the only person working on a small web project, MVC might not be for you. If you are writing a bigger application with a team of developers, MVC can cut down development time and improve code testability and readability.
简而言之,如果您是从事小型Web项目的唯一人员,那么MVC可能不适合您。 如果您要与一组开发人员一起编写更大的应用程序,则MVC可以减少开发时间并提高代码的可测试性和可读性。
翻译自: https://medium.com/dev-genius/make-an-awesome-application-using-the-mvc-design-pattern-9f064b78df48
mvc应用程序生命周期