算法排序算法
Welcome Back to Algo Corner! This is my little corner of the internet to teach you and me about different algorithm concepts and break down how algorithms work in a fundamental manner. This will all be in Javascript with ES6 formatting.
欢迎回到Algo Corner! 这是我在互联网上的一个小角落,它可以教您和我有关不同算法的概念,并分解算法的基本工作方式。 所有这些都将使用ES6格式的Javascript。
DISCLAIMER: I AM NO EXPERT AND STILL LEARNING. If you find an error in my algorithm or math please let me know and let it be a teachable moment rather than a sarcastic or self-righteous one.
免责声明:我不是专家,而且仍在学习。 如果您发现我的算法或数学错误,请告诉我,并且这应该是一个可教的时刻,而不是讽刺或自以为是的时刻。
Today, I will be covering the first of the three “easier” sorting methods that can get you acclimated with sorting algorithms, Bubble Sort.
今天,我将介绍三种“更轻松”的排序方法中的第一种,它可以使您适应排序算法Bubble Bubble。
什么是气泡排序? (What is Bubble Sort?)
The best way to describe Bubble Sort is to iterate an array, and compare every iteration to every other element in the array. If our current number is larger than the next element in the array, we swap. If not we move on to the next element in the array and check again.
描述冒泡排序的最好方法是迭代数组,并将每次迭代与数组中的每个其他元素进行比较。 如果我们当前的数字大于数组中的下一个元素,则交换。 如果不是,我们继续到数组中的下一个元素,然后再次检查。
This might sound like a lot and it is! This sorting method is almost never used due to the inefficiency of its Big O. However, it is a good intro algorithm to get our feet wet, and understand sorting at a base level.
这听起来可能很多,而且确实如此! 由于Big O的效率低下,几乎从未使用过这种排序方法。但是,这是一个很好的介绍性算法,可以使我们精打细算,并了解基本级别的排序。
问题 (Problem)
Write a function that takes in an array of integers and returns a sorted version of that array. Use the Bubble Sort algorithm to sort the array.
编写一个接受整数数组并返回该数组的排序版本的函数。 使用冒泡排序算法对数组进行排序。
Sample
样品
array = [8, 5, 2, 9, 5, 6, 3]**BUBBLE SORT MAGIC**array = [2, 3, 5, 5, 6, 8, 9]
Now I know that seems simple, but we are not really concerned with the end product, but the process. Take a look at the solution walk through below to get into the meat of it.
现在我知道这似乎很简单,但是我们并不是真正关心最终产品,而是过程。 看一看下面介绍的解决方案以了解其中的内容。
解 (Solution)
const bubbleSort = (array) => {
for (let i = 0; i < array.length; i++) {
for (let j = 0; j < array.length; j++) {
if (array[j] > array[j + 1]) {
[array[j], array[j + 1]] = [array[j + 1], array[j]];
}
}
}
return array;
}
Key Elements of Solution
解决方案的关键要素
ES6 Swap: Prior to this handy dandy function, we needed to create a swap helper function that did some sleight of hand with reassignments. But now, to swap two items in an array all we need to do is set two arrays equal to each other, pass them items you want to swap into the first array, and switch the two values in the second array.
ES6交换:在此便捷的花花哨功能之前,我们需要创建一个交换助手功能,该功能可以轻松完成重新分配。 但是现在,要交换数组中的两个项目,我们需要做的是将两个数组设置为彼此相等,将要交换的项目传递给它们,将它们交换到第一个数组中,然后在第二个数组中切换两个值。
arr = [thing1, thing2, thing3, thing4]
[thing1, thing2] = [thing2, thing1]
// New Array after swap
arr = [thing2, thing1, thing3, thing4]
It looks so much neater and really cleans up the code.
它看起来非常整洁,可以真正清理代码。
代码中发生了什么? (What is happening in the code?)
We are running two for loops(uh oh)! Our first number is the number we will compare every other element to. If there is a larger number than the first number, that number will take over moving to the end. If not, our original number will make it all the way to the end. The best way to see what happens is to map it out! We will go over the outer for loop iteration, and if you want just follow the pattern for the rest of the numbers in the array.
我们正在运行两个for循环(呃哦)! 我们的第一个数字是我们将与其他所有元素进行比较的数字。 如果数字比第一个数字大,则该数字将移至末尾。 如果没有,我们的原始号码将一直使用到最后。 查看发生情况的最好方法是将其绘制出来! 我们将遍历外部for循环迭代,并且如果您只想遵循数组中其余数字的模式。
[8,5,2,9,5,6,3]First Loop j= 0 Value = 8
[8,5,2,9,5,6,3]第一个循环j = 0值= 8
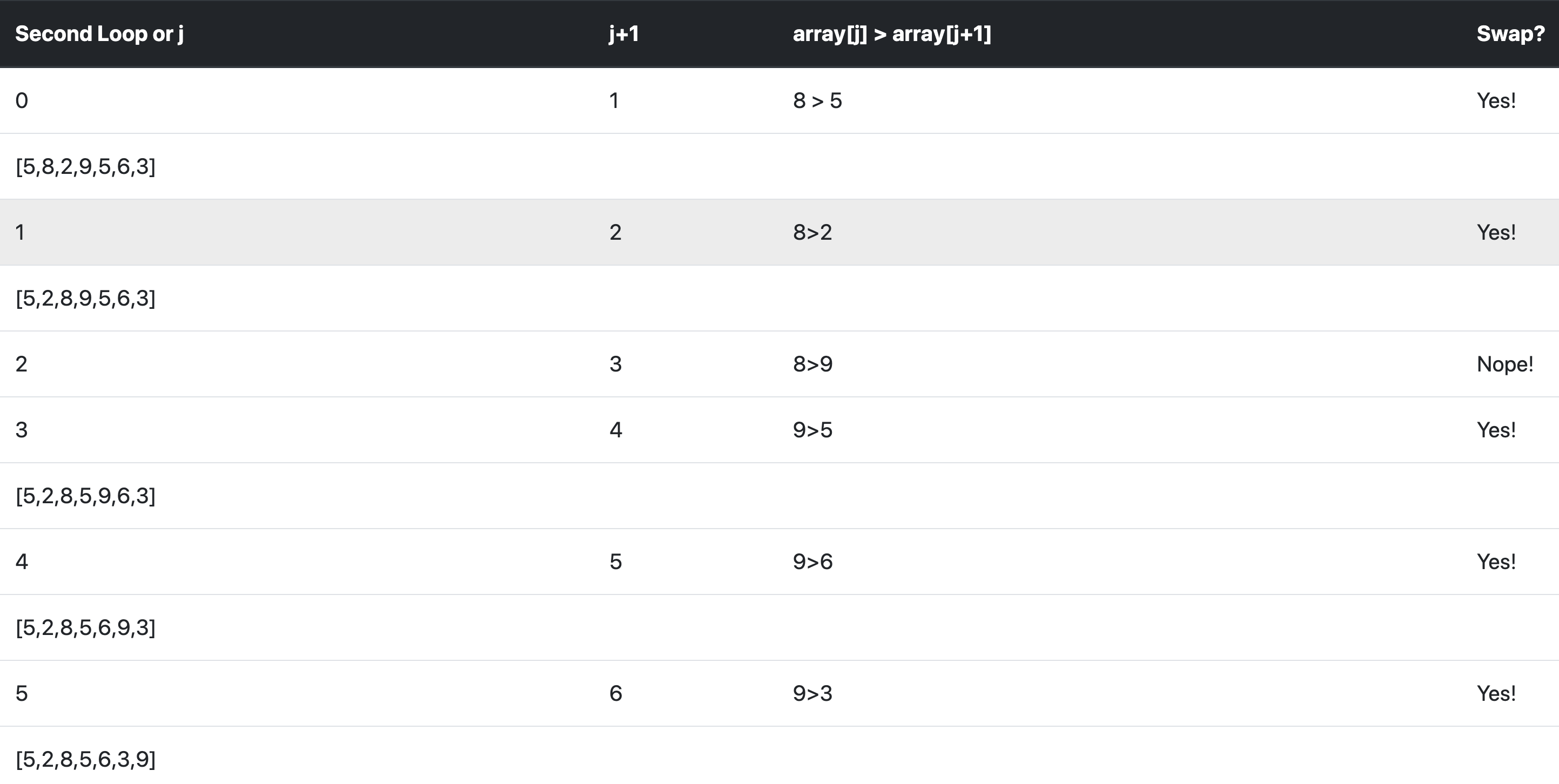
Hence, the term bubble sort, because we are bubbling the largest number to the top! Not much to really cover here. Please write it out! You will see the pattern. It is always better to understand the principle of the sort, than the actual code. The algorithm is code agnostic!
因此,我们将术语“冒泡排序”称为冒泡排序,因为我们正在将最大的数量冒顶! 这里没有太多要讨论的内容。 请写出来! 您将看到该模式。 理解排序的原理总是比实际的代码更好。 该算法与代码无关!
Important Note: I am going to re-stress that you would never use this in production! There are far better sorts. We are using this as a spring board because (a) it is easy to grasp and (b) we love bubbles!
重要说明:我要强调一点,您永远不会在生产中使用此功能! 有更好的选择。 我们将其用作跳板,因为(a)易于掌握,并且(b)我们喜欢气泡!
时间/空间复杂度 (Time/Space Complexity)
Our time complexity is O(n²) because of the nested loops. We can have a best case scenario of O(n), but that is only if the list is sorted already.
由于嵌套循环,我们的时间复杂度为O(n²) 。 我们可以有一个O(n)的最佳情况,但这仅在列表已排序的情况下。
Our space complexity is O(1) because we are not using any additional space. We are mutating the array and swapping indexes. We are not creating any new indexes, or any new data structures to cache or parse data for us.
我们的空间复杂度为O(1),因为我们没有使用任何额外的空间。 我们正在变异数组并交换索引。 我们不会创建任何新索引或任何新数据结构来为我们缓存或解析数据。
下次在Algo Corner (Next Time on Algo Corner)
We will be continuing our sorting series with Insertion Sort! The sort that puts itself in the middle of everything.
我们将通过插入排序继续我们的排序系列! 将自己置于所有事物中间的那种。
翻译自: https://medium.com/@depakborhara/algo-corner-bubble-sort-9684eb745c52
算法排序算法