react 生命挂钩
“What the heck are hooks?” question pops up on your mind after covering all react basic concepts. If you are a React developer and haven’t learned about React hooks yet, it is the perfect time to start learning now and start using.
“钩到底是什么?” 涵盖所有React基本概念后,您会突然想到这个问题。 如果您是React开发人员,但尚未了解React钩子,那么现在就是开始学习并开始使用的绝佳时机。
The main hooks are introduced in react 1) useEffect()
and 2) useState()
3) useReducer()
4) useFetch
. This article is on useEffect hook
在React1) useEffect()
和2) useState()
3) useReducer()
4) useFetch
中引入了主钩子。 本文在useEffect挂钩上
P.S: If you are new to React, I would recommend learning Hooks first, and then learn the older ways of doing things.
PS:如果您不熟悉React,建议您先学习Hook,然后再学习较旧的做事方法。
React挂钩的前提条件 (Pre-requisites for React Hooks)
Node version
6
or above节点版本
6
或更高版本NPM version
5.2
or aboveNPM
5.2
或更高版本React and React-DOM version >
16.8
React和React-DOM版本>
16.8
React Hooks ...它试图解决什么? (React Hooks… What is it trying to solve?)
Hooks were introduced in React version 16.8
and now used by many teams that use React.
挂钩是在React版本16.8
中引入的,现在被使用React的许多团队使用。
Hooks solves the problem of code reuse across components. They are written without classes (functional component). This does not mean that React is getting rid of classes, but hooks is just an alternate approach.
Hooks解决了跨组件的代码重用问题。 它们编写时没有类(功能组件)。 这并不意味着React摆脱了类,但是钩子只是一种替代方法。
In React, you can soon end up with complex components with stateful logic. It is not easy to break these components because within the class you are dependent on the React Lifecycle Methods. That’s where React Hooks come in handy. They provide you a way to split a component, into smaller functions. Instead of splitting code based on the Lifecycle methods, you can now organize and split your code into smaller units based on functionality.
在React中,您很快就可以拥有带有状态逻辑的复杂组件。 打破这些组件并不容易,因为在该类中,您依赖于React Lifecycle Methods。 那就是React Hooks派上用场的地方。 它们为您提供了一种将组件拆分为较小功能的方法。 现在,您可以根据功能将代码组织和拆分为较小的单元,而不是根据生命周期方法拆分代码。
This is a huge win for React developers. We have always been trained to write React classes that adhere to the confusing lifecycle methods. Things are going to get better with the introduction of hooks to React.
对于React开发人员来说,这是一个巨大的胜利。 我们一直受过训练,编写遵循混乱的生命周期方法的React类。 引入React的挂钩将使情况变得更好。
‘Hooks are functions that let you “hook into” React state and lifecycle features from function component. They do not work within a class. They let you use React without a class.’ — React Official Blog post.
“挂钩是使您能够“挂钩”功能组件中的React状态和生命周期功能的函数。 他们不在班级里工作。 他们让您无需上课就能使用React。 — React官方博客文章。
如何使用useEffect()挂钩? (How to use useEffect() hook?)
useEffect hook essentially is to allow side effects within the functional component. In class components, you may be familiar with lifecycle methods. The lifecycle methods, componentDidMount, componentDidUpdate and componentWillUnmount, are all handled by the useEffect hook in functional components.
useEffect挂钩本质上是为了允许功能组件内出现副作用。 在类组件中,您可能熟悉生命周期方法。 生命周期方法componentDidMount,componentDidUpdate和componentWillUnmount均由功能组件中的useEffect挂钩处理。
Before the introduction of this hook, there was no way to perform these side-effects in a functional component. Now the useEffect hook, can provide the same functionality as the three lifecycle methods mentioned above. Let’s look at some examples to learn this better.
在引入此挂钩之前,还没有办法在功能组件中执行这些副作用。 现在, useEffect挂钩可以提供与上述三种生命周期方法相同的功能。 让我们看一些例子来更好地学习。
A story Before Hooks: you can follow that here . Let’s skip this for now.
一个在钩子之前的故事:您可以在此处关注。 让我们暂时跳过这个。
具有useEffect Hook的功能组件 (Functional Component with useEffect Hook)
import React, { useState, useEffect } from "react";
const UseEffectExample = () => {
const [button, setButton] = useState("");
//useEffect hook
useEffect(() => {
console.log("useEffect has been called!", button);
});
const onYesPress = () => {
setButton("Yes");
};
const onNoPress = () => {
setButton("No");
};
return (
<div>
<button onClick={() => this.onYesPress()}>Yes</button>
<button onClick={() => this.onNoPress()}>No</button>
</div>
);
};
export default UseEffectExample;
Note: The first thing we need to do to get the useEffect to work is, import the useEffect from React.
注意:要使useEffect起作用,我们需要做的第一件事是从React导入useEffect 。
import React, { useEffect } from "react";
Notice here that the useEffect hook has access to the state. When you run this code, you will initially see that the useEffect is called which could be similar to the componentDidMount. After that every time the state of the button changes, the useEffect hook is called.
请注意, useEffect挂钩可以访问状态。 运行此代码时,最初会看到调用了useEffect ,它可能类似于componentDidMount 。 之后,每次按钮状态更改时,都会调用useEffect挂钩。
// Console logs
useEffect has been called! ""
useEffect has been called! Yes
useEffect has been called! No
I hope you are with me so far. Let’s look into some more details about the useEffect hook.
我希望你到目前为止与我在一起。 让我们研究有关useEffect挂钩的更多详细信息。
传递空数组以使用效果钩 (Passing Empty Array to useEffect Hook)
You can optionally pass an empty array to the useEffect hook, which will tell React to run the effect only when the component mounts.
您可以选择将一个空数组传递给useEffect钩子,该钩子将告诉React仅在组件安装时运行效果。
Here is the modified useEffect hook from the previous example, which will occur at mount time.
这是上一个示例中经过修改的useEffect挂钩,它将在安装时发生。
//useEffect hook
useEffect(() => {
console.log("useEffect has been called!", button);
}, []);
When you run this on the console you will only see the useEffect being called once at mount.
在控制台上运行此命令时,只会看到在挂载时调用一次useEffect 。
// Console log
useEffect has been called! ""
使用多次使用效果挂钩的单独关注点 (Separate Concerns using Multiple useEffect Hooks)
An interesting feature of the useEffect hook is that, you can separate them into multiple hooks, based on the logic. With lifecycle methods, this was not possible. Often, unrelated logic was combined within the same lifecycle method, because there could only be one of each lifecycle method within the class component.
useEffect挂钩的一个有趣功能是,您可以根据逻辑将它们分成多个挂钩。 使用生命周期方法,这是不可能的。 通常,不相关的逻辑被合并在同一个生命周期方法中,因为类组件中每个生命周期方法只能有一个。
If you have multiple states in your functional component, you can have multiple useEffect hooks. Let’s extend the previous example, by adding another state within the component, that display the titles of blog posts. Now this is unrelated to the yes and no button we had. We can create multiple useEffect hooks, to separate the concerns as shown below:
如果功能组件中有多个状态,则可以具有多个useEffect挂钩。 让我们扩展前面的示例,方法是在组件内添加另一个状态,以显示博客文章的标题。 现在,这与我们拥有的是和否按钮无关。 我们可以创建多个useEffect挂钩,以分离关注点,如下所示:
import React, { useState, useEffect } from "react";
import { default as UUID } from "uuid";const UseEffectExample = () => {
const [button, setButton] = useState(""); const [blogPosts, setBlogPosts] = useState([
{ title: "Learn useState Hook", id: 1 },
{ title: "Learn useEffect Hook", id: 2 }
]); useEffect(() => {
console.log("useEffect has been called!", button);
}, [button]); useEffect(() => {
console.log("useEffect has been called!", blogPosts);
}, [blogPosts]); const onYesPress = () => {
setButton("Yes");
}; const onNoPress = () => {
setButton("No");
};
const onAddPosts = () => {
setBlogPosts([...blogPosts, { title: "My new post", id: UUID.v4() }]);
}; return (
<div>
<button onClick={() => this.onYesPress()}>Yes</button>
<button onClick={() => this.onNoPress()}>No</button>
<ul>
{blogPosts.map(blogPost => {
return <li key={blogPost.id}>{blogPost.title}</li>;
})}
</ul>
<button onClick={() => onAddPosts()}>Add Posts</button>
</div>
);
};export default UseEffectExample;
In the example above, we have a button state and a blog post state within the component. We have separated the unrelated logic into two different effect hooks. With the lifecycle methods, this would have not been possible. Hooks let us split the code based on what it is doing rather than a lifecycle method name. React will apply every effect used by the component, in the order they were specified.
在上面的示例中,组件中有一个按钮状态和一个博客帖子状态。 我们将无关的逻辑分为两个不同的效果挂钩。 使用生命周期方法,这是不可能的。 挂钩让我们根据代码的工作方式(而不是生命周期方法名称)来拆分代码。 React将按照指定的顺序应用组件使用的所有效果。
When we run this code, when the component is mounted both the useEffect hooks are run as follows:
当我们运行此代码时,在安装组件时,两个useEffect挂钩均按以下方式运行:
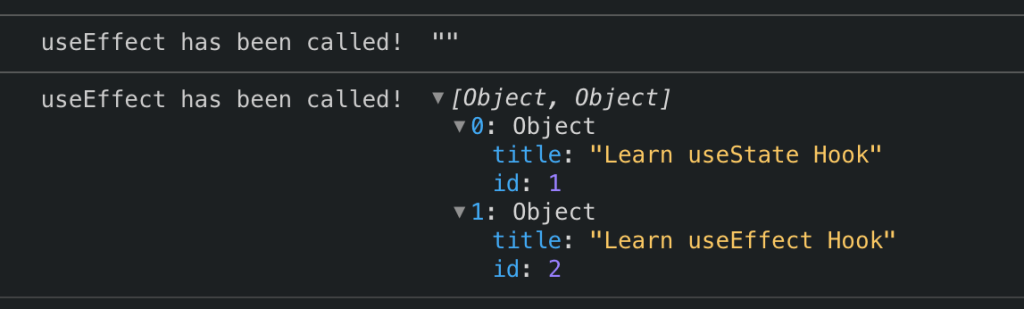
You can see how the effects have been separated for each state. This is done by passing the state within an array to the useEffect hook.
您可以看到每种状态的效果是如何分离的。 这是通过将数组内的状态传递给useEffect挂钩来完成的。
Now if the Yes/No button is pressed, you should see this on the console.
现在,如果按下“是/否”按钮,您应该在控制台上看到它。

Notice here, that the useEffect for the blogPosts has not been invoked here. This tells React which effect to apply, without bundling them all within a call. Now if we clicked on adding a blog post button, we would see its effect take place.
请注意,此处未调用blogPosts的useEffect。 这告诉React应用哪种效果,而不会在调用中将它们全部捆绑在一起。 现在,如果单击添加博客帖子按钮,我们将看到其效果发生了。
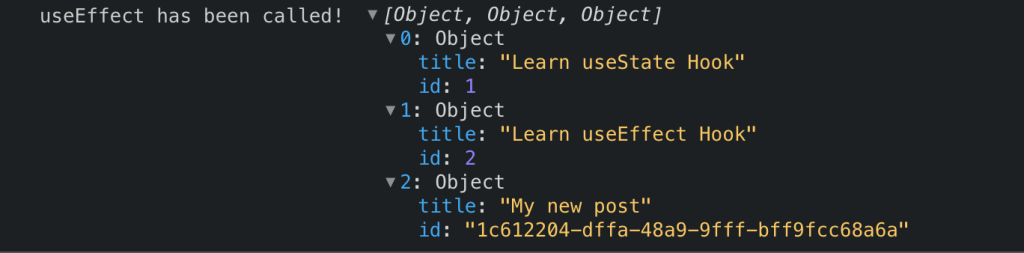
You get the idea! Now get more..
你明白了! 现在得到更多..
The rules of Hooks clearly state:
胡克规则明确规定:
Don’t call Hooks inside loops, conditions, or nested functions. Instead, always use Hooks at the top level of your React function.
不要在循环,条件或嵌套函数中调用Hook。 相反,请始终在您的React函数的顶层使用Hooks。
Hooks need to be called in the same order each time the component renders. There are several reasons why this is the case which is beautifully articulated in. You definitely cannot do this:
每次渲染组件时,都需要以相同的顺序调用挂钩。 出现这种情况的原因很多,其中有几个原因。您绝对不能这样做:
<button onClick={() => useFetch({ skip: n + 1 * 10, take: 10 })}>
{n + 1}
</button>
优化? (Optimization?)
If you are used to the class components with lifecycle methods, you would have tried to optimize when the componentDidUpdate is called by passing the prevProps or prevState and compare it with the current state. Only if they don’t match the componentDidUpdate will happen. Now with useEffect hook, you can achieve the same optimization by simply passing the state in an array as a parameter as we have seen in the example above. This will ensure that the hook is run when the state passed to the effect changes.
如果您使用的类组件与生命周期方法,你会试图优化时componentDidUpdate通过使prevProps或prevState并将其与当前状态比较调用。 仅当它们不匹配时, componentDidUpdate才会发生。 现在,通过useEffect挂钩,您可以通过简单地将数组中的状态作为参数传递来实现相同的优化,如上例所示。 这将确保在传递给效果的状态发生更改时,挂钩可以运行。
结论 (Conclusion)
Congratulations, you have stayed with me so far! Hooks is a fairly newer concept in React, and the official React documentation does not recommend that you rewrite all your components using Hooks. Instead, you can start writing your newer components using Hooks.
恭喜,您到目前为止已经和我在一起! Hooks是React中一个相对较新的概念,并且官方的React文档不建议您使用Hooks重写所有组件。 相反,您可以使用Hooks开始编写更新的组件。
For more reading:
欲了解更多信息:
https://medium.com/@Harry_1408/react-hook-a-complete-understanding-of-usereducer-ff68cc2c37a4
https://medium.com/@Harry_1408/react-hook-a-complete-understanding-of-usereducer-ff68cc2c37a4
https://medium.com/@Harry_1408/react-hook-a-complete-understanding-of-usestate-933a992e0c4f
https://medium.com/@Harry_1408/react-hook-a-complete-understanding-of-usestate-933a992e0c4f
https://medium.com/@Harry_1408/react-story-before-react-hooks-5b7c65b04075
https://medium.com/@Harry_1408/react-story-before-react-hooks-5b7c65b04075
Have a Grate Learning!
学习炉排!
Stay Safe ❤
保持安全❤
翻译自: https://medium.com/@Harry_1408/react-hook-a-guide-to-learning-useeffect-11687e777aeb
react 生命挂钩