react 生命挂钩
I wanted the navigation bar of my portfolio website to disappear upon scrolling down, and reappear upon scrolling up.
我希望投资组合网站的导航栏在向下滚动时消失,而在向上滚动时重新出现。

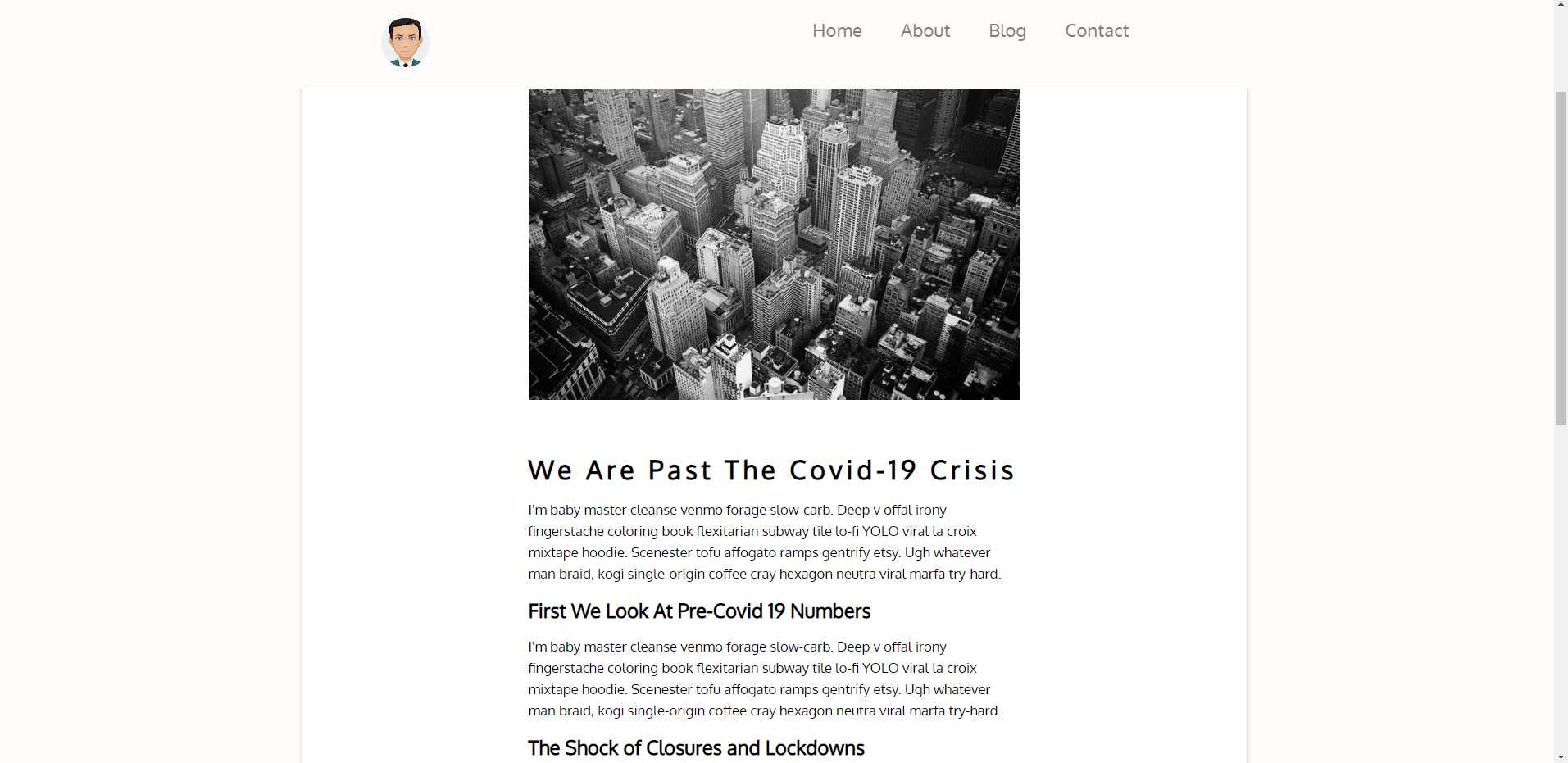
To achieve the above functionality I used React Hooks to write a customized hook that would determine the users scrolling direction.
为了实现上述功能,我使用React Hooks编写了一个定制的钩子,该钩子将确定用户的滚动方向。
The navigation bar component used the returned direction value from the hook to apply the appropriate Css style to itself.
导航栏组件使用钩子返回的方向值将适当的Css样式应用于自身。
If the user was at the top of the page, the navigation bar was displayed at the top with no special Css styling applied to it.
如果用户位于页面顶部,则导航栏显示在顶部,而没有应用特殊CSS样式。
useScrollDirection挂钩背后的逻辑 (The Logic Behind My useScrollDirection Hook)
To determine the amount scrolled by the user I used window.scrollY
. The scrollY property on the browser window object returned the number of pixels the document was scrolled vertically.
为了确定用户的滚动量,我使用window.scrollY
。 浏览器窗口对象上的scrollY属性返回垂直滚动文档的像素数。
Initially window.scrollY
is 0 and the more the user scrolls down the larger it gets.
最初window.scrollY
为0,并且用户向下滚动的次数越多,获取的内容就越大。
Within the custom hook I had two states:
在自定义钩子中,我有两种状态:
- The scrolled direction, initially null 滚动方向,最初为null
- The previous scrolled amount from the top of the page, initially 0 从页面顶部开始的前一个滚动量,最初为0
const [scrollDirection, setScrollDirection] = useState(null)
const [prevOffset, setPrevOffset] = useState(0)
I also subscribed an event listener to the window scrolling event. I set up the subscription with the help of the effect hook.
我还为窗口滚动事件订阅了事件侦听器。 我在效果挂钩的帮助下设置了订阅。
useEffect(() => {
window.addEventListener("scroll", toggleScrollDirection) return () => {
window.removeEventListener("scroll", toggleScrollDirection)
}
})
Every time the user scrolled, toggleScrollDirection
was invoked.
每次用户滚动时, toggleScrollDirection
调用toggleScrollDirection
。
Inside the function, the amount scrolled by the user was captured by a variable
在函数内部,用户滚动的量由变量捕获
let scrollY = window.scrollY;
And then for every time scrolling happened the following conditions were checked in order to set the appropriate scrolling direction state value.
然后,每次发生滚动时,都要检查以下条件,以设置适当的滚动方向状态值。
if (scrollY === 0) {
setScrollDirection(null)
}if (scrollY > prevOffset){
setScrollDirection("down")
} else if (scrollY < prevOffset) {
setScrollDirection("up")
}setPrevOffset(scrollY);
If the user was at the top of the page, scrollY would be zero, and so we set the set the scrolling direction as null.
如果用户位于页面顶部,则scrollY将为零,因此我们将滚动方向设置为null。
If the user was scrolling down, then the scrollY value would be greater than the last scrollY value stored in the prevOffset
state.
如果用户向下滚动,则scrollY值将大于存储在prevOffset
状态的最后一个scrollY值。
The exact opposite was true when the user scrolled up. In both cases the scrollDirection
state would be set appropriately.
当用户向上滚动时,情况恰好相反。 在这两种情况下, scrollDirection
正确设置scrollDirection
状态。
Finally, the prevOffset
would be set as this last scrollY value, to be referenced the next time the user scrolled.
最后,将prevOffset
设置为该最后的scrollY值,以供用户下次滚动时参考。
The entire Hook Code was
整个钩子代码是
const useScrollDirection = () => {
const [scrollDirection, setScrollDirection] = useState(null)
const [prevOffset, setPrevOffset] = useState(0) const toggleScrollDirection = () => {
let scrollY = window.scrollY
if (scrollY === 0) {
setScrollDirection(null)
}
if (scrollY > prevOffset) {
setScrollDirection("down")
} else if (scrollY < prevOffset) {
setScrollDirection("up")
}
setPrevOffset(scrollY)
}
useEffect(() => {
window.addEventListener("scroll", toggleScrollDirection)
return () => {
window.removeEventListener("scroll", toggleScrollDirection)
}
}) return scrollDirection
}
导航栏组件如何使用此挂钩切换导航栏外观 (How The Navigation Bar Component Used This Hook to Toggle Navigation Bar Appearance)
Inside the Navbar component we captured the scroll direction value returned from the useScrollDirection hook.
在Navbar组件内部,我们捕获了从useScrollDirection挂钩返回的滚动方向值。
const scrollDirection = useScrollDirection()
Then according to scrollDirection
, we would apply appropriate css styles to the navigation bar.
然后根据scrollDirection
,我们将适当CSS样式应用于导航栏。
The styling of the navigation bar can be done in many different ways so I leave that out of this article.
导航栏的样式可以通过许多不同的方式完成,因此在本文中不再赘述。
This is my very first article published on Medium. Forgive me for the subpar writing. I hope to improve as I write more! I hope you enjoyed it and found it useful!
这是我在Medium上发表的第一篇文章。 原谅我的写作不佳。 我希望随着我写的更多而有所改善! 希望您喜欢它并发现它有用!
普通英语JavaScript (JavaScript In Plain English)
Enjoyed this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!
喜欢这篇文章吗? 如果是这样,请订阅我们的YouTube频道解码,以获得更多类似的内容!
翻译自: https://medium.com/@kairosh.sanjar/custom-scrolling-direction-react-hook-f55558206ab6
react 生命挂钩