重点(Top highlight)
There’s an interesting discussion on the Internet about how Web Components will replace modern front-end libraries, and that popular tools like React and Vue will slowly become obsolete like MooTools and jQuery.
互联网上有一个有趣的讨论,关于Web组件将如何取代现代的前端库,而流行的工具(如React和Vue)将像MooTools和jQuery一样逐渐过时。
Maybe you want to use React for your web application projects, but fear that it’s going to be replaced by Web Components. This article will help you alleviate that fear by learning the difference between Web Components and React.
也许您想在Web应用程序项目中使用React,但是担心它会被Web Components取代。 本文将通过学习Web Components和React之间的区别来帮助您减轻这种恐惧。
Web组件的目的 (The purpose of Web Components)
Web Components is a set of different technologies that are used together to help developers write UI elements that are semantic, reusable, and properly isolated.
Web组件是一组不同的技术,可以一起使用,以帮助开发人员编写语义,可重用和正确隔离的UI元素。
A custom element is a way of defining an HTML element by using the browser’s JavaScript API. A custom element has its own semantic tag and lifecycle methods, similar to a React component
自定义元素是一种使用浏览器JavaScript API定义HTML元素的方法。 自定义元素具有自己的语义标签和生命周期方法,类似于React组件
Shadow DOM is a way of isolating DOM elements and scoping CSS locally to prevent breaking changes from affecting other elements.
Shadow DOM是一种隔离DOM元素并在本地范围内定义CSS的方法,以防止重大更改影响其他元素。
HTML template is a way of writing invisible HTML elements that acts as a template that you can operate on using JavaScript’s query selector.
HTML模板是一种编写不可见HTML元素的方法,该元素充当可以使用JavaScript的查询选择器进行操作的模板。
Let’s learn how each of these technology actually works with code examples.
让我们学习一下每种技术如何与代码示例实际结合使用。
自定义元素示例 (A custom element example)
Web Component’s custom elements are created by using the browser’s JavaScript API. A custom element is defined using JavaScript’s class keyword that extends HTMLElement
. Once defined, the custom element can be reused as many times as you need in your application:
Web组件的自定义元素是通过使用浏览器JavaScript API创建的。 使用扩展HTMLElement
JavaScript的class关键字定义自定义元素。 定义后,可以根据需要在应用程序中重复使用custom元素:
A custom element name must include a dash or the browser will complain that you have an invalid element name. You need to name a button as <primary-button>
or <button-component>
instead of just <button>
.
自定义元素名称必须包含破折号,否则浏览器会抱怨您的元素名称无效。 您需要将按钮命名为<primary-button>
或<button-component>
而不仅仅是<button>
。
Custom elements also have lifecycle callbacks for running code during specific times of its existence. These lifecycle callbacks work in a similar way to React’s lifecycle methods.
自定义元素还具有生命周期回调,用于在代码存在的特定时间运行代码。 这些生命周期回调的工作方式与React的生命周期方法类似。
As of today, you need to write custom elements
截止到今天,您需要编写自定义元素
影子DOM示例(The shadow DOM example)
Shadow DOM allows you to write HTML elements that were scoped from the actual DOM tree. It’s attached to the parent element, but won’t be considered as its child element by the browser. Here is an example:
Shadow DOM允许您编写实际DOM树范围内HTML元素。 它附加在父元素上,但浏览器不会将其视为子元素。 这是一个例子:
Any code you write inside the shadow DOM will be encapsulated from the code outside of it. One of the benefits of using shadow DOM is that any CSS code you write will be local and won’t affect any element outside of it.
您在影子DOM中编写的任何代码都将从其外部代码中封装。 使用影子DOM的好处之一是,您编写的任何CSS代码都将是本地的,并且不会影响其中的任何元素。
When you inspect the shadow element, you’ll see it marked with #shadow-root:
当您检查阴影元素时,您会看到它标有#shadow-root:
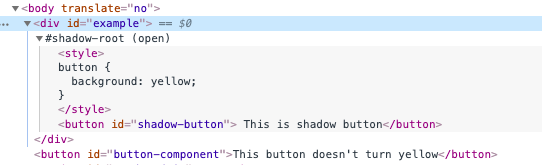
The browser will return null
when you try to select the shadow button with document.getElementById(‘shadow-button’)
. You need to select the parent element and grab its shadowRoot
object first:
当您尝试使用document.getElementById('shadow-button')
选择阴影按钮时,浏览器将返回null
。 您需要选择父元素并首先获取其shadowRoot
对象:
const el = document.getElementById('example').shadowRoot
el.getElementById('shadow-button')
HTML模板示例(HTML template example)
HTML template allows you to write invisible HTML elements that you can iterate through with JavaScript in order to display dynamic data. To write one, you need to wrap the elements inside a <template>
tag:
HTML模板允许您编写不可见HTML元素,可以使用JavaScript对其进行迭代以显示动态数据。 要编写一个,需要将元素包装在<template>
标记内:
<template id="people-template">
<li>
<span class="name"></span> —
<span class="age"></span>
</li>
</template><ul id="people"></ul>
Then you can iterate through the template above with JavaScript selector and append it into the <ul>
element :
然后,您可以使用JavaScript选择器遍历上面的模板,并将其附加到<ul>
元素中:
const fragment = document.getElementById('people-template');
const people = [
{ name: 'Daniel', age: 22 },
{ name: 'Jessie', age: 29 },
{ name: 'Andy', age: 32 }
];people.forEach(person => {
const instance = document.importNode(fragment.content, true);
instance.querySelector('.name').innerHTML = person.name;
instance.querySelector('.age').innerHTML = person.age;
document.getElementById('people').appendChild(instance);
});
By using the template, you can fetch data from an API server and use the template to serve the data in a structured way.
通过使用模板,您可以从API服务器获取数据,并使用模板以结构化的方式提供数据。
Now that you know what and how Web Components work, it’s time to answer the question.
现在您知道了Web组件的工作方式以及工作方式,现在该回答这个问题了。
Web组件会取代React吗? (Will Web Components replace React?)
A modern front-end application needs more than just components. The great thing about React is that it keeps your components in sync with your data.
现代的前端应用程序不仅需要组件。 React的伟大之处在于它使您的组件与数据保持同步。
When compared with Web Components, React has the following advantages:
与Web组件相比,React具有以下优点:
- Allows you to change the underlying data model with state 允许您更改带有状态的基础数据模型
- Trigger UI changes based on the state根据状态触发用户界面更改
- Writing components using functions and hooks使用函数和钩子编写组件
- A ready to use unidirectional data flow即用型单向数据流
- A greater ecosystem of third-party libraries and guides更大的第三方图书馆和指南生态系统
React’s eco-system is incredibly vast — so much so that it is now possible to use React for everything: Dynamic SPAs, Static pages, Native Android/iOS apps, Windows/Mac desktop apps, CLI apps, etc.
React的生态系统异常庞大,以至于现在可以将React用于所有内容:动态SPA,静态页面,本机Android / iOS应用程序,Windows / Mac桌面应用程序,CLI应用程序等。
That means two things:
这意味着两件事:
- You can learn once and build for multiple platforms 您可以学习一次并为多个平台构建
- You can build once and reuse across different apps您可以构建一次并在不同的应用程序之间重用
Sharing components (and reusing them across apps) is now easier than ever with tools like Bit (Github) that allow you to track components independently and share them, from any project, to a single component hub.
现在,使用Bit ( Github )之类的工具可以比以往更加轻松地共享组件(并在应用程序之间重用),该工具可让您独立跟踪组件并将它们从任何项目共享到单个组件中心。
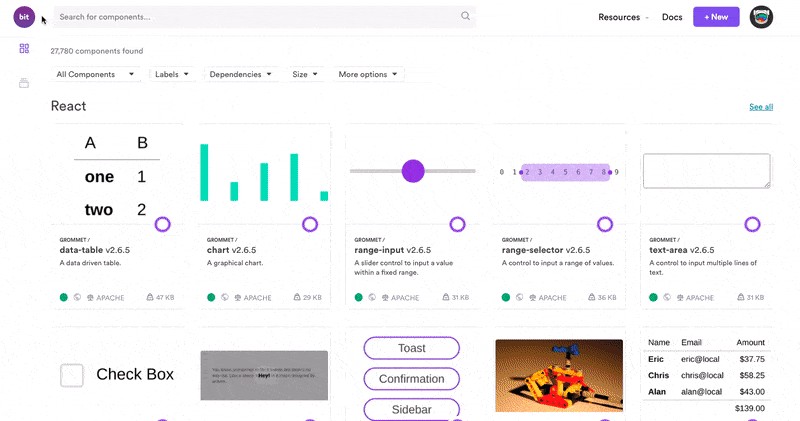
React’s underlying data model with state and props allows its components to change the rendered elements on the screen without imperatively manipulating the DOM or changing attributes manually. When you change the state, React will automatically re-render the components to trigger UI changes.
React带有状态和属性的底层数据模型允许其组件更改屏幕上的呈现元素,而无需强制性地操作DOM或手动更改属性。 当您更改状态时,React将自动重新渲染组件以触发UI更改。
React also allows you to write components using functions and hooks. When it first came out, hooks are a big improvement to React because it reduces the complexity of managing a component’s lifecycle. It also enables you to write stateful components using functions, completely removing the need to use classes, which confuses both people and machines.
React还允许您使用函数和钩子编写组件。 钩子首次发布时,它是对React的一项重大改进,因为它降低了管理组件生命周期的复杂性。 它还使您能够使用函数来编写有状态的组件,从而完全消除了使用类的需求,而这会混淆人和机器。
Furthermore, functional components in React are easier to maintain because of the way useEffect
hook gather related logic under one function. For example, when using class components in React, you need to duplicate your code to run on mount and subsequent updates:
此外,由于useEffect
挂钩在一个功能下收集相关逻辑的方式,React中的功能组件更易于维护。 例如,在React中使用类组件时,您需要复制代码以在mount和后续更新中运行:
class Example extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}componentDidMount() {
document.title = `You clicked ${this.state.count} times`;
}componentDidUpdate() {
document.title = `You clicked ${this.state.count} times`;
}render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Click me
</button>
</div>
);
}
}
But with hooks, you can have a useEffect
function that runs on mount and subsequent updates in a single function:
但是,有了钩子,您可以拥有一个useEffect
函数,该函数可以在单个函数中的安装和后续更新中运行:
import React, { useState, useEffect } from 'react';function Example() {
const [count, setCount] = useState(0);useEffect(() => {
document.title = `You clicked ${count} times`;
});return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
When using class components, you need to group unrelated code under the same lifecycle method because that’s the only place where you can run the code reasonably, such as fetching data and setting up listener on componentDidMount:
使用类组件时,需要将不相关的代码归为同一生命周期方法,因为这是可以合理地运行代码的唯一位置,例如,在componentDidMount:
上获取数据和设置侦听器componentDidMount:
componentDidMount() {
// Putting data fetching and listener in the same function
axios.post();DataSource.addChangeListener(this.handleChange);
}
With useEffect
hook, you can separate this unrelated code but run them on the same lifecycle:
使用useEffect
挂钩,您可以分离不相关的代码,但可以在相同的生命周期中运行它们:
// separating functions to minimize confusionuseEffect(() => {
axios.post();
});useEffect(() => {
DataSource.addChangeListener(this.handleChange);
});
As a library for building complete web applications, React also offers a unidirectional data flow pattern, where data is passed down from parent components to children components with props. Web Components currently requires you to write your own data binding pattern.
作为用于构建完整Web应用程序的库,React还提供了单向数据流模式,其中数据通过props从父组件传递到子组件。 Web组件当前需要您编写自己的数据绑定模式。
Finally, React has a big collection of third party libraries to help developers with the most common application features like:
最后,React有大量第三方库来帮助开发人员提供最常见的应用程序功能,例如:
Of course, Web Components may replace React when there is enough support for all of these development needs, but I’m certain by then Web Components won’t be what we know today. Just like React, it will have supporting libraries and even visual builders to allow developers to build applications easier.
当然,当所有这些开发需求都有足够的支持时,Web Components可能会取代React,但是我敢肯定,届时Web Components将不再是我们今天所知道的。 就像React一样,它将具有支持库甚至可视化生成器,以使开发人员可以更轻松地构建应用程序。
Even then, don’t forget that React is also used outside of web development. It’s more likely that Web Components will one day become an alternative option for developing web applications, rather than replace the need for libraries entirely.
即使这样,也不要忘记React也用于Web开发之外。 有一天,Web组件更有可能成为开发Web应用程序的替代选择,而不是完全取代对库的需求。
结论 (Conclusion)
Web Components is a set of different technology being used together to help you write reusable elements that are encapsulated from the rest of your code. On the other hand, React is a JavaScript library meant to help you write user interface in a declarative way.
Web组件是一起使用的一组不同技术,可帮助您编写从其余代码中封装的可重用元素。 另一方面,React是一个JavaScript库,旨在帮助您以声明的方式编写用户界面。
A scalable modern front-end development requires many other things besides components. That’s why you don’t have to be afraid to use React and its ecosystem of tools and libraries. It surely won’t be replaced by Web Components anytime soon.
可扩展的现代前端开发除组件外还需要许多其他功能。 这就是为什么您不必害怕使用React及其工具和库的生态系统的原因。 它肯定不会很快被Web Components取代。
学到更多 (Learn More)
翻译自: https://blog.bitsrc.io/web-component-why-you-should-stick-to-react-c56d879a30e1