vscode编写react
A couple of years ago I was assigned a task to implement an application feature that allows users to switch between application icons. The application was built using React-Native so my solution was to write an open-source library called react-native-change-icon
.
几年前,我被分配了一项任务,以实现一个应用程序功能,该功能允许用户在应用程序图标之间切换。 该应用程序是使用React-Native构建的,因此我的解决方案是编写一个名为react-native-change-icon
的开源库。
写作 (Writing)
I began by using the react-native-create-library
tool to create a template that provided me with the basic file and directory setup which includes the required Java classes for Android and Objective-C modules for iOS. Now you might think that I must be mad skilled at writing code in Java and Objective-C but that far away from the truth. I had some experience with Java before but I never used it for Android, and it was the first time that I was using Objective-C, that too for writing something for iOS applications.
我首先使用react-native-create-library
工具创建一个模板,该模板为我提供了基本的文件和目录设置,其中包括Android所需的Java类和iOS的Objective-C模块。 现在您可能会认为我必须非常狂热于用Java和Objective-C编写代码,但事实与事实相去甚远。 我曾经有过Java的经验,但是我从未在Android上使用过Java,这也是我第一次使用Objective-C,也为iOS应用程序编写了东西。
I started searching for tutorials and documentation for both Android and iOS to find ways to implement the task. For iOS, I was able to find a blog that provided a straightaway implementation of the method setAlternateIconName
in Objective-C and the setup that I needed to create in order to use this. For Android, I did not find any direct way to implement this but I found an article in which a similar functionality was created by manipulating the <activity-alias>
element.
我开始搜索Android和iOS的教程和文档,以找到实现任务的方法。 对于iOS,我能够找到一个博客,该博客提供了Objective-C中setAlternateIconName
方法的直接实现以及我需要创建的设置才能使用它。 对于Android,我没有找到任何直接的方法来实现这一点,但是我发现了一篇文章,其中通过操纵<activity-alias>
元素创建了类似的功能。
的iOS (iOS)
The implementation for iOS was pretty straightforward. First, we need to add and link multiple icons in the Xcode project of iOS app. Then, we mention these icons in the Info.plist
file to be used as alternate icons. Then, to switch the icon, we need to pass the name of the icon we need to activate in the setAlternateIconName
method.
iOS的实现非常简单。 首先,我们需要在iOS应用的Xcode项目中添加并链接多个图标。 然后,我们在Info.plist
文件中提到这些图标,以用作备用图标。 然后,要切换图标,我们需要在setAlternateIconName
方法中传递需要激活的图标的名称。
安卓系统 (Android)
The implementation for Android was a bit tricky. First, we need to add all the icon assets in the mipmap directories. Next step would be to create an <activity-alias>
element for each of the icons that's there in the application's assets. As we’ll be using <activity-alias>
elements to display the application icon on the device launcher, we can remove the activity MAIN and category LAUNCHER intent filters from the <activity>
element. The functionality will work by enabling one <activity-alias>
at a time and when switching the icon, we’ll disable the previous activity and enable the new one. We’ll create a custom function that can do this using the Android activity package manager. As I said the implementation is tricky but you’ll get a clearer picture by watching the code implementation.
Android的实现有些棘手。 首先,我们需要在mipmap目录中添加所有图标资产。 下一步将是为应用程序资产中存在的每个图标创建一个<activity-alias>
元素。 由于我们将使用<activity-alias>
元素在设备启动器上显示应用程序图标,因此我们可以从<activity>
元素中删除活动MAIN和类别LAUNCHER Intent过滤器。 该功能将通过一次启用一个<activity-alias>
起作用,并且在切换图标时,我们将禁用上一个活动并启用新活动。 我们将创建一个自定义函数,可以使用Android活动包管理器执行此操作。 正如我所说的,实现是棘手的,但是通过观看代码实现,您将获得更清晰的画面。
包 (Package)
The native implementation was finalised but now I had figure out how to give this functionality to the React-Native context. For this, React-Native has provided an API called NativeModules. It provides native packages that can be used to expose your native classes and methods to the JavaScript context through the React-Native bridge. The API would have been easy to use if there was proper documentation at the time I was writing this library as they have now. With this API, you can call native methods with arguments, get callback values or return promises with values. I used these APIs to export the changeIcon
method from the Android Java module and the iOS Objective-C module.
本机实现已完成,但现在我已经弄清楚了如何将此功能提供给React-Native上下文。 为此,React-Native提供了一个名为NativeModules的API。 它提供了本机包,可用于通过React-Native桥向JavaScript上下文公开本机类和方法。 如果我像现在这样编写该库的时候有适当的文档,那么该API将会易于使用。 使用此API,您可以使用参数调用本机方法,获取回调值或使用值返回承诺。 我使用这些API从Android Java模块和iOS Objective-C模块导出changeIcon
方法。
With this, the package implementation was complete and I published this package on NPM.
至此,该软件包的实现已完成,我将该软件包发布在NPM上。
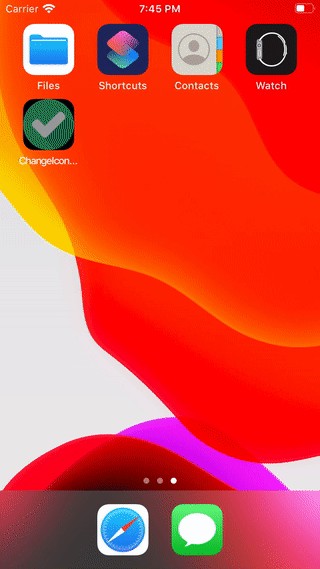
包装寿命(Life of the package)
Since the time of its initial release, the package received a number of issues and pull requests from the open-source community. These issues and pull requests helped in continuous updating of the package to work with the latest React-Native, iOS and Android versions.
自从最初发布以来,该软件包就收到了许多问题,并收到了来自开源社区的请求。 这些问题和请求请求有助于持续更新软件包以使其与最新的React-Native,iOS和Android版本一起使用。
改写 (Re-Writing)
Recently I came across a new CLI tool that generates templates for new libraries which is @react-native-community/bob
. This package allows you to generate templates in multiple native languages that work with Android and iOS. You can use Kotlin or Java for Android and Objective-C or Swift for iOS. With this package, I generated a template for the change icon package written in Kotlin for Android and Swift for iOS. Now all I had to do was convert the code that I already wrote in Java and Objective-C to Kotlin and Swift.
最近,我遇到了一个新的CLI工具,该工具为@react-native-community/bob
新库生成模板。 该软件包可让您以多种本机语言生成适用于Android和iOS的模板。 您可以使用Android的Kotlin或Java,以及iOS的Objective-C或Swift。 借助此程序包,我为用Android的Kotlin和iOS的Swift编写的更改图标包生成了模板。 现在,我要做的就是将我已经用Java和Objective-C编写的代码转换为Kotlin和Swift。
The template generated was very seamless to work with. It included a setup which I didn’t have previously because I didn’t think I actually needed it. To all the people who are creating a new react-native library, I would strongly suggest you go with this tool. It takes all the pressure of your mind to create all the basic and recommended setup for an NPM package. You are able to just focus on your native implementation.
生成的模板可以无缝使用。 它包含了我以前没有的设置,因为我认为我实际上并不需要它。 对于所有正在创建新的本机React库的人,我强烈建议您使用此工具。 为NPM软件包创建所有基本和推荐的设置都需要您的所有压力。 您可以只专注于本机实现。
There is really good documentation for working with Kotlin and Swift. I was able to move all my code to these languages pretty easily. From the point of view of a package user, nothing was changed, the steps to install and use the package remained the same.
关于Kotlin和Swift的工作确实有很好的文档。 我能够轻松地将所有代码移至这些语言。 从软件包用户的角度来看,什么都没有改变,安装和使用软件包的步骤保持不变。
结论 (Conclusion)
As a package owner, I would suggest to always keep good documentation for your package. Provide step-by-step instructions to install and use the package. For react-native packages, the installation and usage might be a little tricky so in my opinion, you should always create an example application that uses your package, it makes a lot easier for people to understand how to use the package. While creating a release, write a good changelog that exclusively mentions the breaking changes. Maintaining the package is one of the most important aspect for owning a package.
作为软件包所有者,我建议始终为您的软件包保留良好的文档。 提供安装和使用软件包的分步说明。 对于本机软件包,安装和使用可能会有些棘手,因此,我认为,您应始终创建一个使用该软件包的示例应用程序,这使人们更容易理解如何使用该软件包。 在创建发行版时,编写一个良好的变更日志,专门提及重大变更。 维护软件包是拥有软件包最重要的方面之一。
Thank you for reading this!
谢谢您阅读此篇!
vscode编写react