odoo 自定义视图
In the Android application, Activity
or Fragment
would be the base component for the single page. You can put other UI controls into a single layout xml and inflate it in anActivity
or a Fragment
. One of the problems is that if the UI is complicated, the Activity
or Fragment
might also include complicated UI logic. In general case, a single page is a composite of many basic UI controls like TextView
, Button
, ImageView
, etc. You need to implement the presentation logic in theActivity
or the Fragment
even in the Controller, Presenter or ViewModel.
在Android应用程序中,“ Activity
或“ Fragment
将是单个页面的基本组件。 您可以将其他UI控件放入单个布局xml中,然后将其填充到Activity
或Fragment
。 问题之一是,如果UI复杂,则Activity
或Fragment
也可能包含复杂的UI逻辑。 通常,单个页面是由许多基本UI控件(如TextView
, Button
, ImageView
等)组成的。您需要在Activity
或Fragment
甚至在Controller , Presenter或ViewModel中实现表示逻辑。
一个简单的例子 (A simple example)
Assume that we want to implement a simple digest UI like this
假设我们要实现一个简单的摘要UI,例如
This widget includes 3 TextViews in a ViewGroup. So we can declare the UI layout in xml like this.
此小部件在ViewGroup中包含3个TextView。 因此,我们可以像这样在xml中声明UI布局。
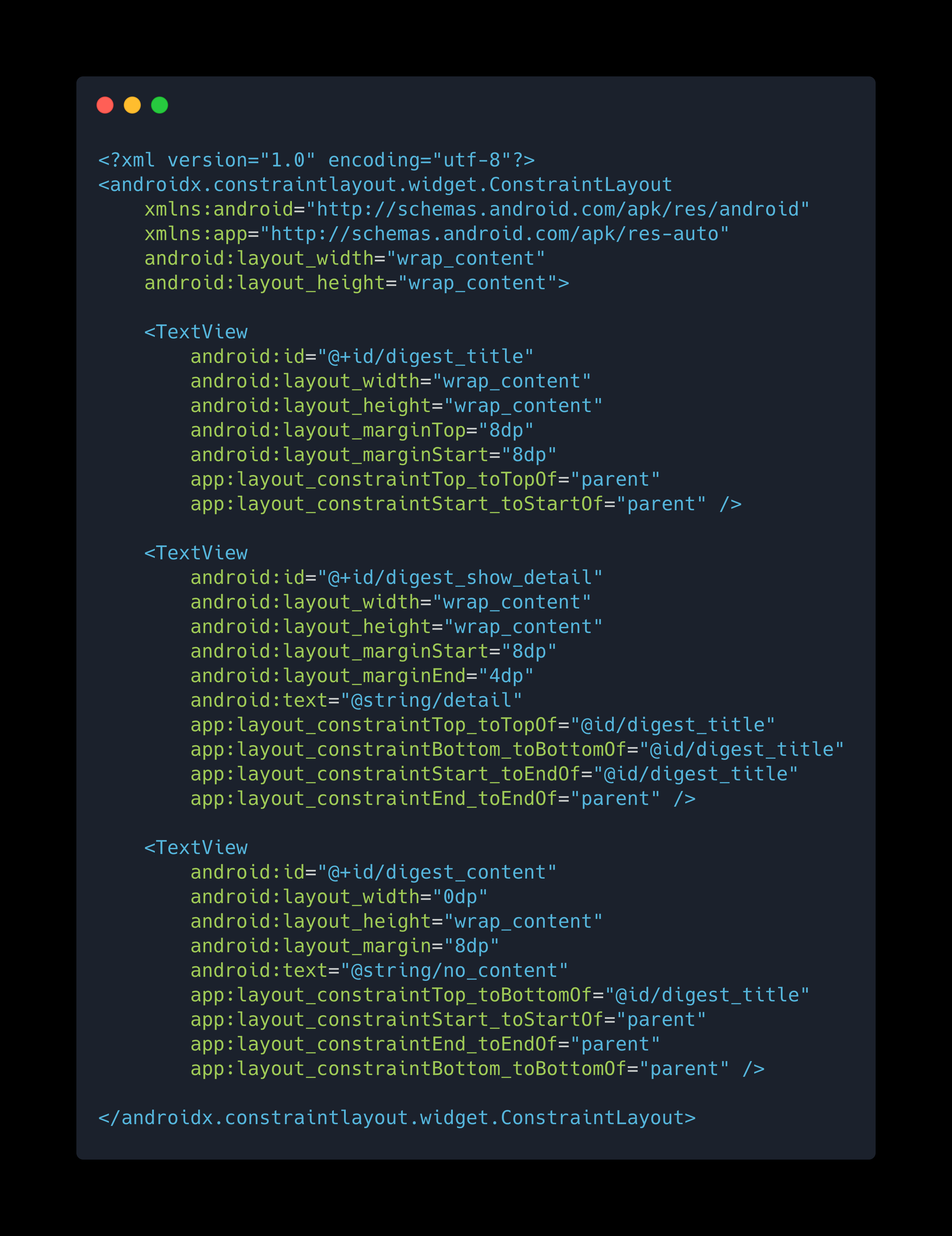
then we can add the UI setup function in Fragment
然后我们可以在Fragment
添加UI设置功能
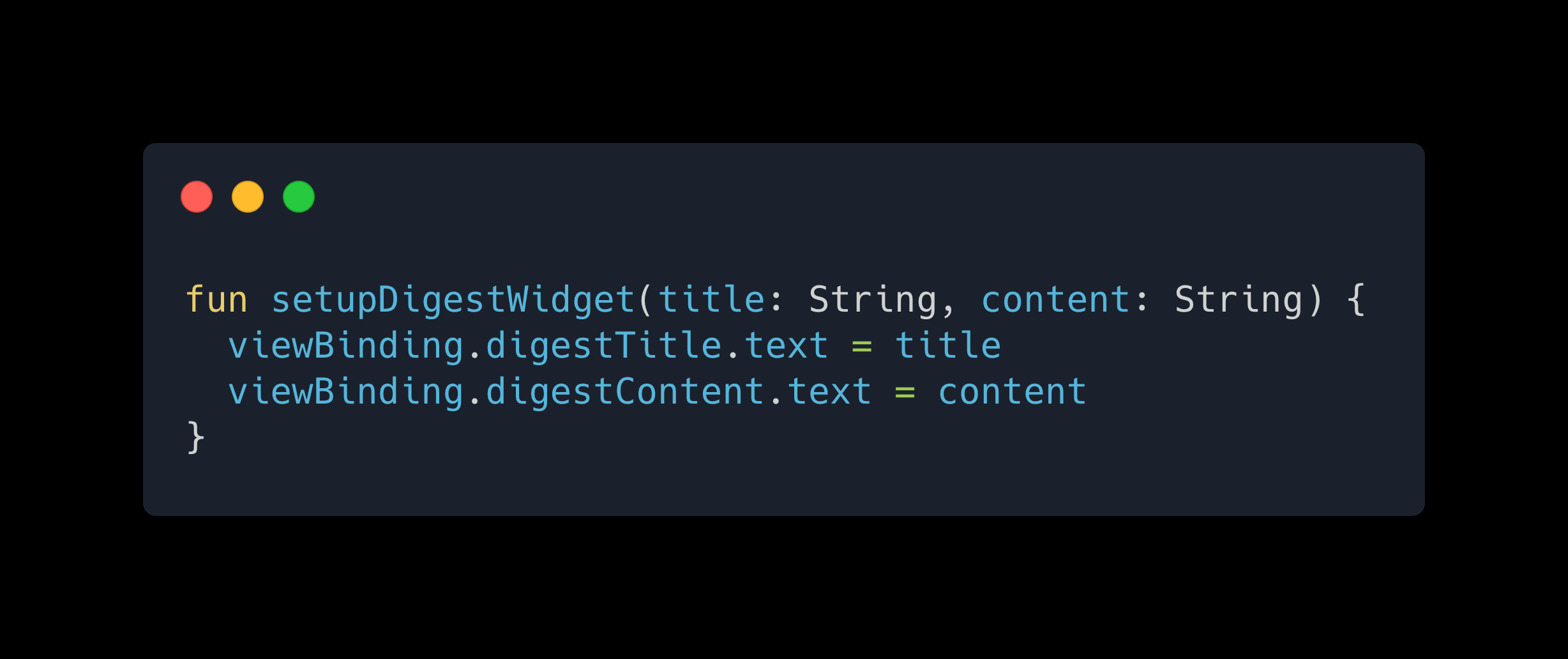
and thanks new ViewBinding, we don’t need to use findViewById
by ourselves. And then we want to add the right arrow icon to the right of Detail
TextView and assign the same color and adjust the dimension. So we add one more function to do it.
还要感谢new ViewBinding ,我们不需要自己使用findViewById
。 然后,我们想在Detail
TextView的右侧添加右箭头图标,并分配相同的颜色并调整尺寸。 因此,我们增加了一个功能。
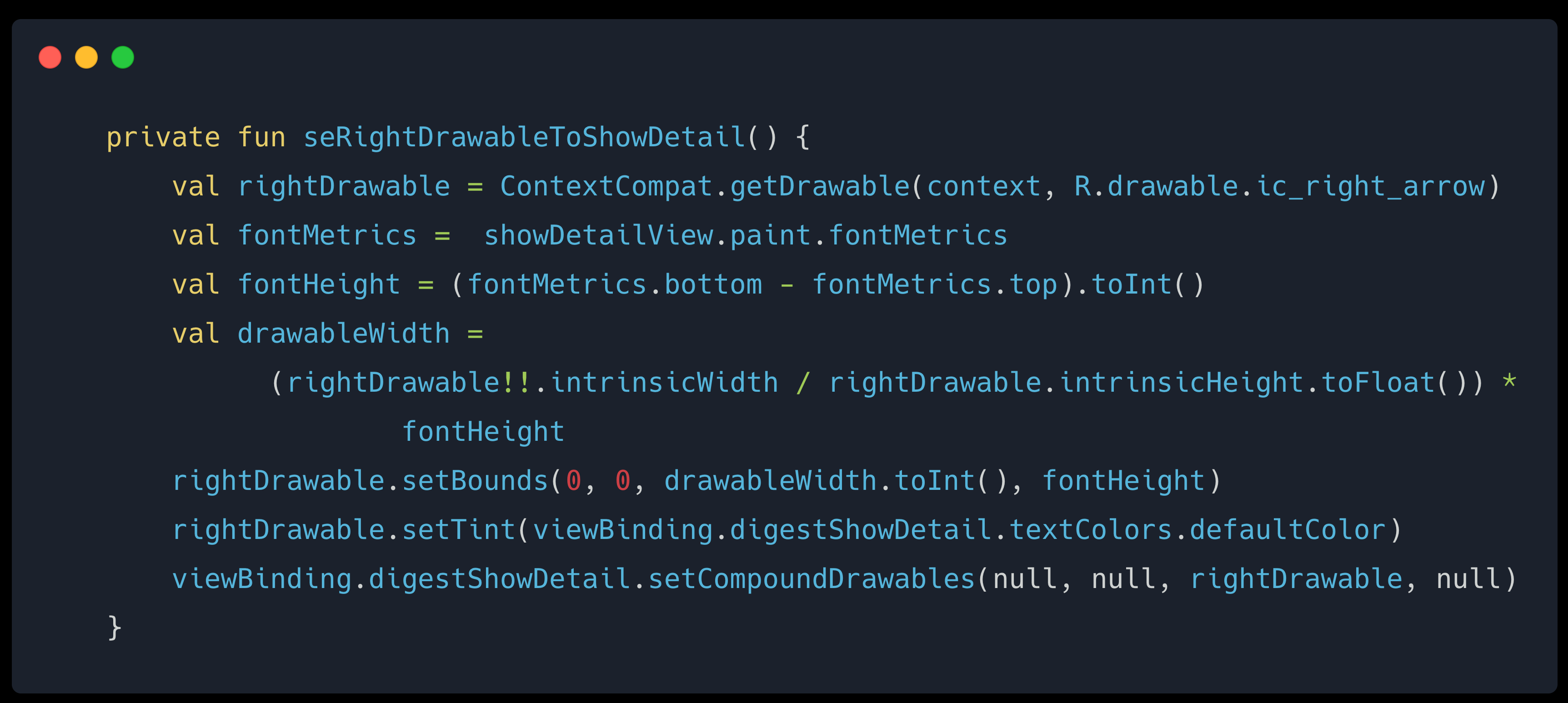
As you can see, this is not unusual in the Android development. If the UI includes many widgets, the fragment will become a fatter because it includes lots of presentation logics. Besides, if we want to reuse the UI widget in another fragment, it would be difficult.
如您所见,这在Android开发中并不罕见。 如果UI包含许多小部件,则该片段将变得更加胖,因为它包含许多表示逻辑。 此外,如果我们想在另一个片段中重用UI小部件,将很困难。
Could we have a better solution? One of them is that we can create a custom view group and use the layout file with a<merge>
tag. So we can move presentation logics into the custom view group.
我们能有更好的解决方案吗? 其中之一是,我们可以创建一个自定义视图组,并使用带有<merge>
标签的布局文件。 因此,我们可以将表示逻辑移动到自定义视图组中。
<merge>技巧 (The <merge> tricks)
By the official document, the <merge>
is usually used with <include>
tag. The purpose of <merge>
is to eliminate redundant view groups in the view hierarchy. Actually, we can also create a custom view group and inflate the layout xml with <merge>
. Let’s see how to do it.
在官方文档中, <merge>
通常与<include>
标记一起使用。 <merge>
的目的是消除视图层次结构中的冗余视图组。 实际上,我们还可以创建一个自定义视图组,并使用<merge>
填充布局xml。 让我们来看看如何做。
First, We change the root tag of original xml to <merge>
like below.
首先,我们将原始xml的根标签更改为<merge>
如下所示。
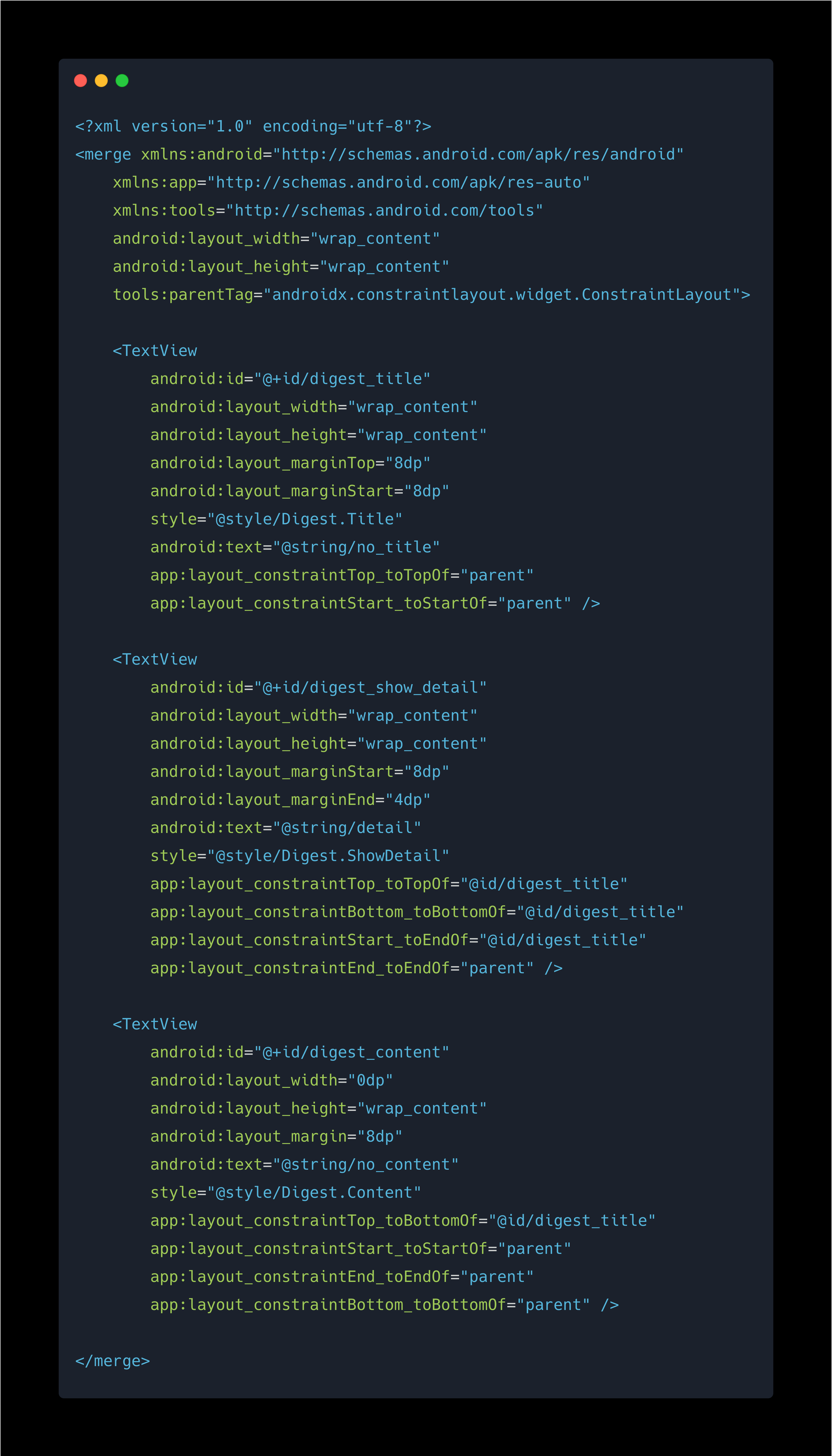
Then we create a class to extend ConstraintLayout
然后我们创建一个类来扩展ConstraintLayout
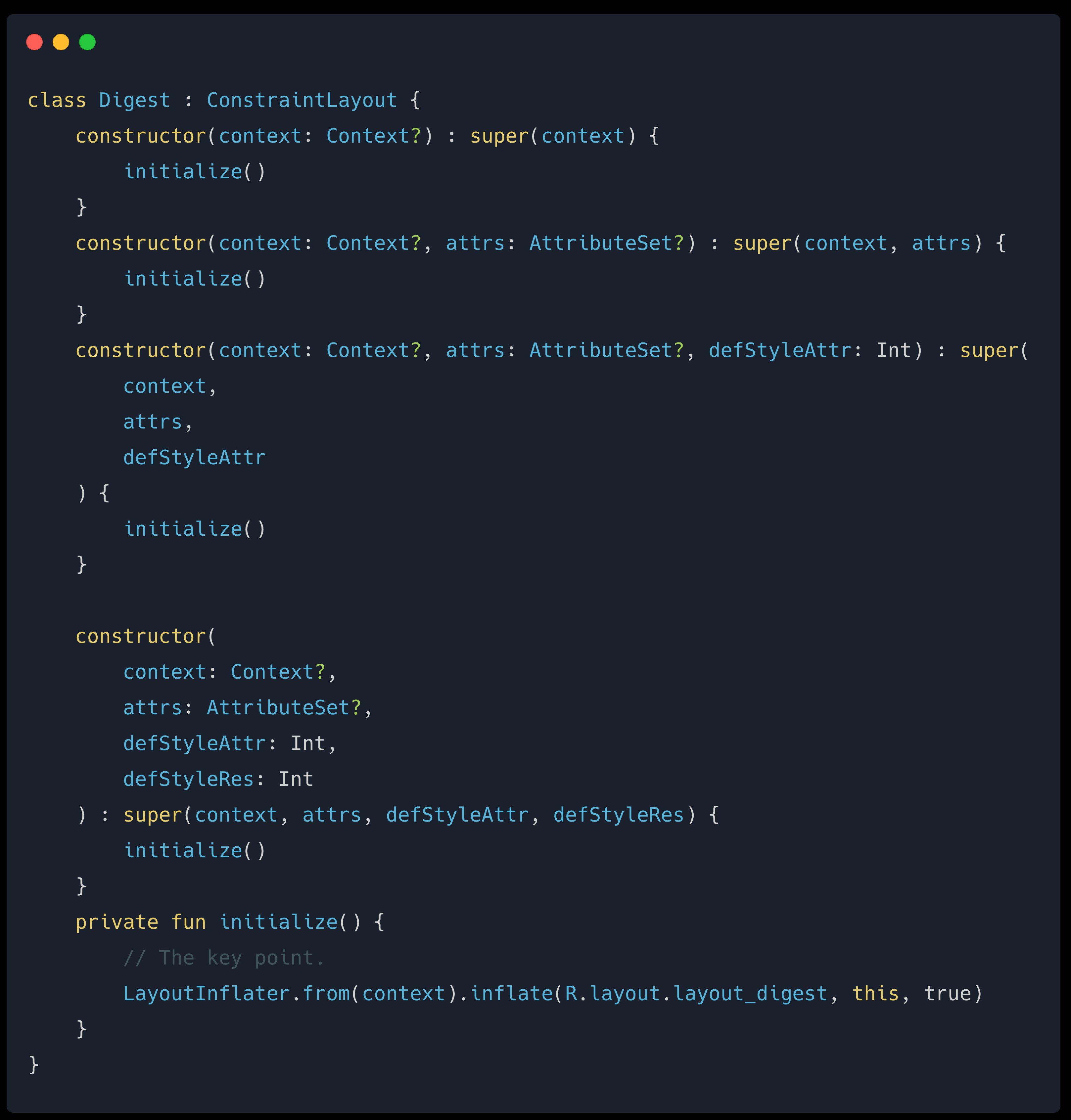
The key point is that we inflate the xml and attach to the root ViewGroup.
关键是我们将xml膨胀并附加到根ViewGroup。
LayoutInflater
.from(context)
.inflate(R.layout.layout_digest, this, true)
and then we have a compound UI widget and we can also use it another xml layout directly!
然后我们有一个复合UI小部件,我们还可以直接使用它另一个XML布局!
<Digest
android:id="@+id/digest"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Next, we move the presented functions into the new class and create 2 String properties
接下来,我们将呈现的函数移到新类中并创建2个String属性
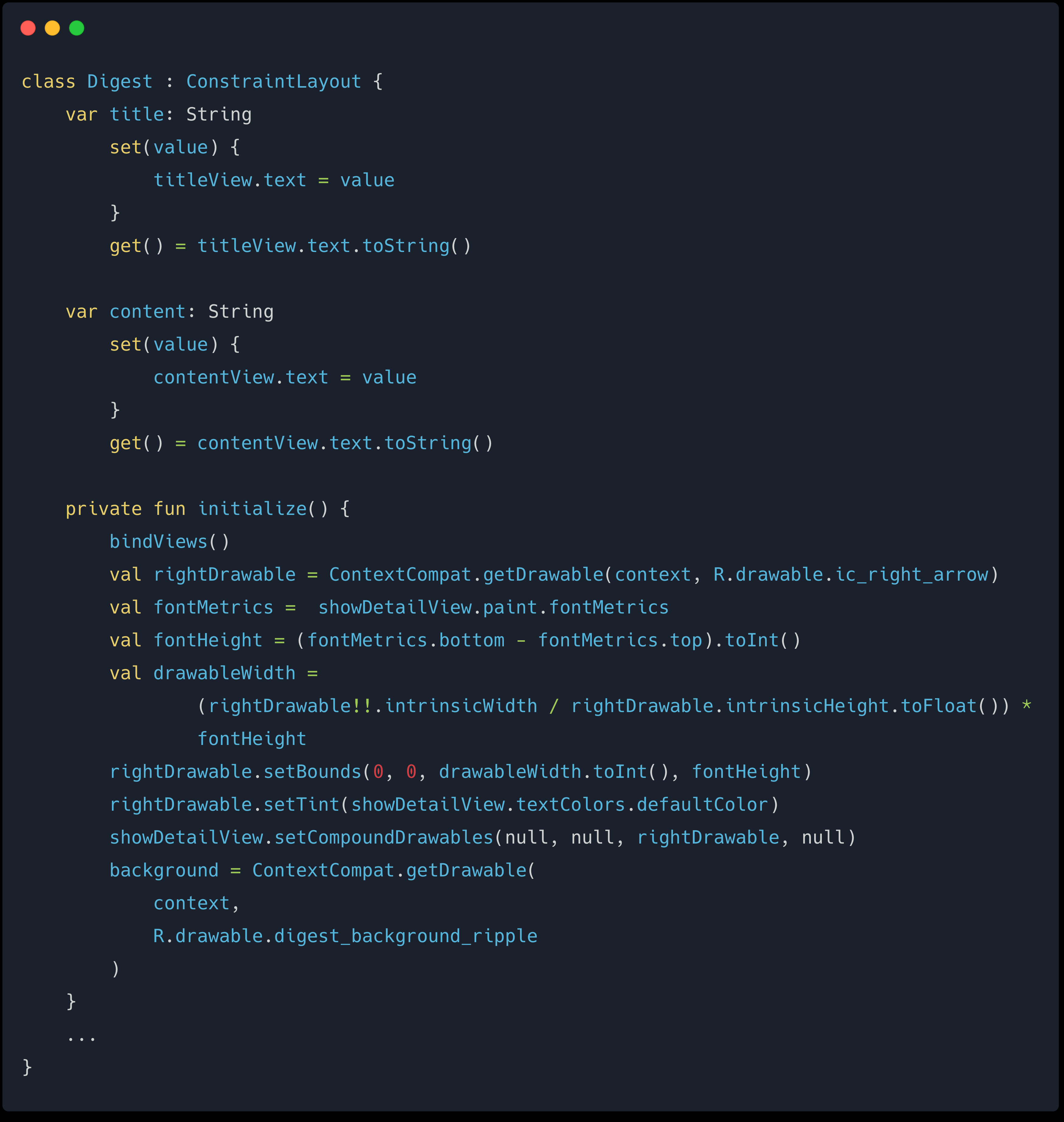
Finally, we can move all presented code into the custom ViewGroup and use it in the Fragment
or Activity
directly.
最后,我们可以将所有呈现的代码移到自定义ViewGroup中,然后直接在Fragment
或Activity
使用它。
// In Fragment
viewBinding.digest.title = "This is title"
viewBinding.digest.content = "This is content"
利弊 (Pros and Cons)
By using <merge>
in the xml can help us to extract the presented code from Fragment
or Activity
. We can more focus on business logic. Of course it is not a perfect solution. Here is the Pros and Cons as I know.
通过在xml中使用<merge>
可以帮助我们从Fragment
或Activity
提取呈现的代码。 我们可以更加关注业务逻辑。 当然,这不是一个完美的解决方案。 据我所知,这是优点和缺点。
优点 (Pros)
We can move the presentation logic which belongs a “View” to the appropriate place
我们可以将属于“视图”的表示逻辑移动到适当的位置
Reduce the complexity of
Fragment
orActivity
降低
Fragment
或Activity
的复杂性- The UI can be reused in another place.UI可以在其他地方重复使用。
If you don’t like to use
Fragment
, you can use this trick to replaceFragment
.如果您不喜欢使用
Fragment
,可以使用此技巧来替换Fragment
。
缺点 (Cons)
- You may create many custom widgets您可以创建许多自定义小部件
Some properties assigned in xml may not work. It depends on how you initialize the
ViewGroup
nowxml中分配的某些属性可能不起作用。 这取决于您现在如何初始化
ViewGroup
That’s it! If you have any feedback, please don’t hesitate to leave your comments.
而已! 如果您有任何反馈意见,请随时发表评论。
odoo 自定义视图