Javascript is one of the most popular programming languages in the world. A lot of people also have a notion that it’s really easy to learn Javascript, but over last few years it has gained popularity exponentially, and with frameworks like Node JS, people have started to take it seriously in terms of server side development as well. Anyone can learn the basics of the language, but it takes time and effort to truly understand the more advanced areas of the language.
Javascript是世界上最受欢迎的编程语言之一。 许多人还认为,学习Javascript真的很容易,但是在过去的几年中,它已经成倍增长,并且使用Node JS之类的框架,人们也开始认真对待它在服务器端开发方面。 任何人都可以学习该语言的基础知识,但是要真正理解该语言的更高级领域需要花费时间和精力。
In this article we will discuss a little bit about the basics of core javascript, along with some weird things happening in javascript.
在本文中,我们将讨论一些有关javascript核心的基本知识,以及javascript中发生的一些奇怪的事情。
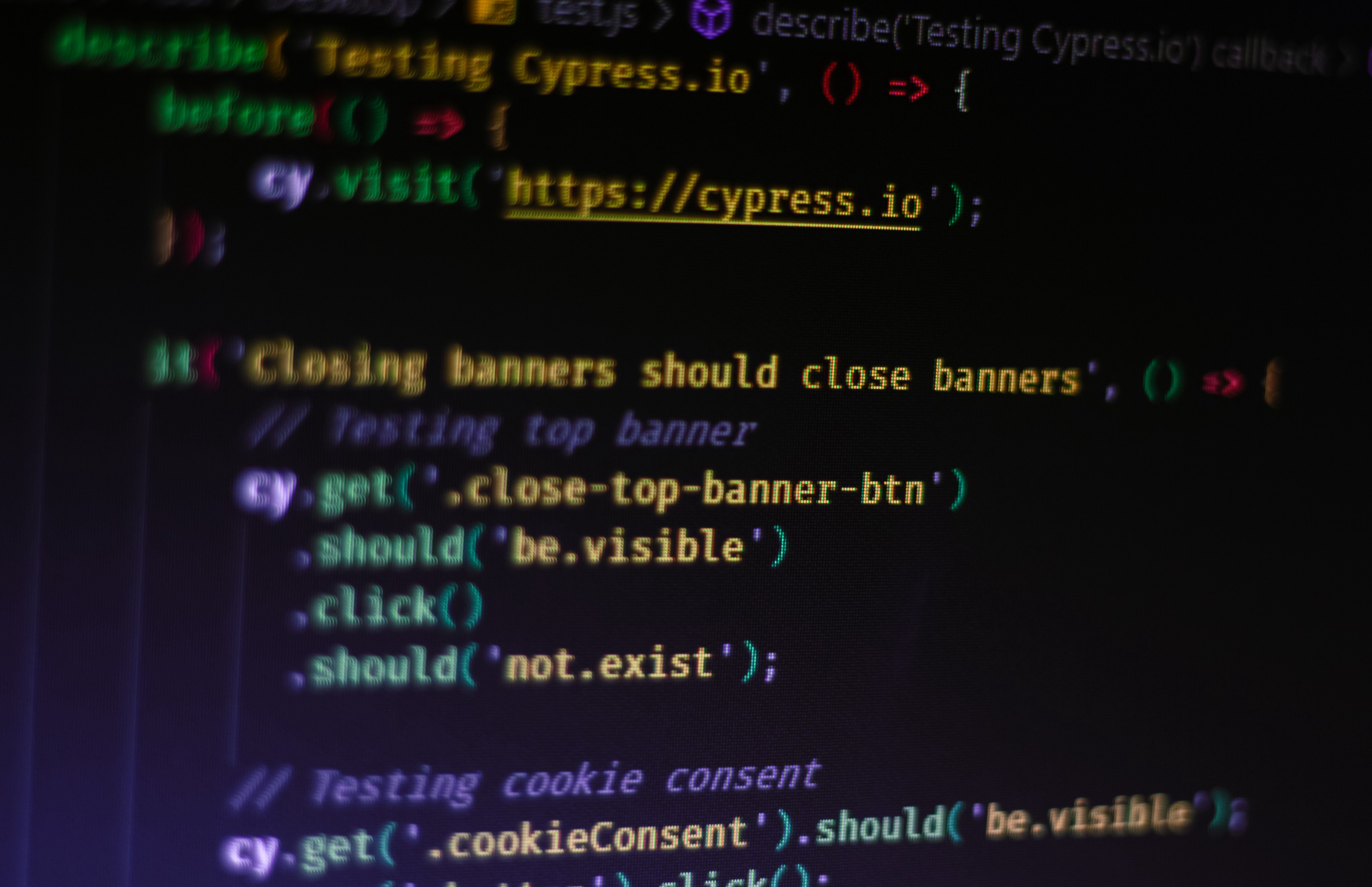
标记的模板文字 (Tagged template literals)
You may already know that ES6 introduced the creation of Template Literals by using the `` quotes (backtick character) located on the tilde key.
您可能已经知道ES6通过使用波浪号键上的``引号( 反引号字符)引入了模板文字的创建。
To use Template Literals you have to use the backtick quotes instead of single or double quotes (which would not work with template literals.)
要使用模板文字,您必须使用反引号引起来的单 引号或双引号 ( 不适用于模板文字)。

But a lot of us are not aware about the magic we can do with string literals. It is possible to combine template literals with a custom-written function created specifically to work in the context of a string literal.
但是我们很多人都不知道我们可以使用字符串文字实现魔术。 可以将模板文字与自定义编写的函数结合使用,该函数专门用于在字符串文字的上下文中工作。
It may sound a little confusing, but try to recollect if you have worked with Styled components library. That’s what we are going to achieve. Take a look at below example.
这听起来可能有些混乱,但是如果您使用过样式化的组件库,请尝试重新收集。 这就是我们要实现的目标。 看下面的例子。

Strange !! Is it? Not exactly. Now the next question that comes up is, can we use dynamic values inside it. Good News ! Yes, you can add dynamic values in the custom function, and the same concept is being used by styled components. In the below code, i have kept the function name as h1 since i am creating a custom h1 tag, you can use any random name for the function.
奇怪! 是吗? 不完全是。 现在出现的下一个问题是,我们可以在其中使用动态值吗? 好消息 ! 是的,您可以在自定义函数中添加动态值,并且样式化组件正在使用相同的概念。 在下面的代码中,自从创建自定义h1标签以来,我一直将函数名称保留为h1,您可以为函数使用任何随机名称。
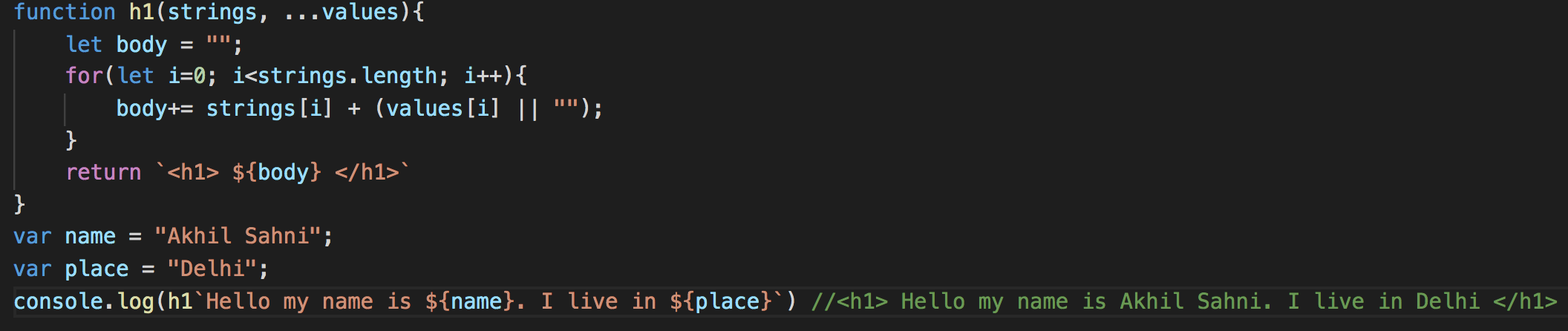
奇怪的NaN (Strange NaN)
We all have once in a while encountered NaN in our code. But a lot of us are not aware about how weird NaN can be at times. It is always a challenge to check the equality for values of NaN. Why I say so is because
我们所有人在我们的代码中偶尔都会遇到NaN。 但是我们很多人都不知道NaN有时会多么奇怪。 检查NaN值的相等性始终是一个挑战。 我之所以这么说是因为
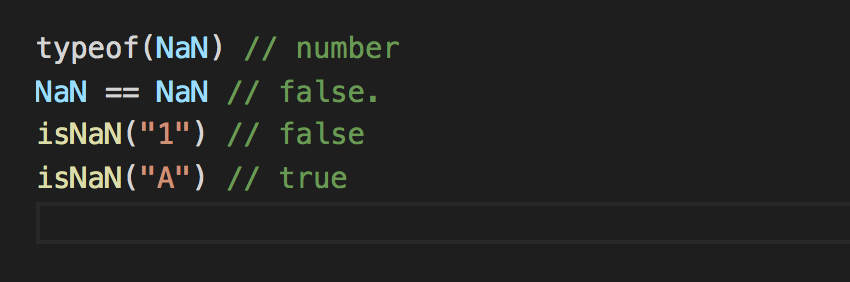
Confused, here comes the biggest catch, NaN is the only value in javascript which will result in below result.
困惑,这是最大的收获,NaN是javascript中唯一的值,它将导致以下结果。
var a = NaN;
var a = NaN;
a !== a → will return true.
!== a→将返回true。
So the best way to check for NaN is using the above condition for isNaN and check the equality at the same time.
因此,检查NaN的最佳方法是将上述条件用于isNaN并同时检查相等性。
功能性论点 (Functional arguments)
Sometimes in functions we use arguments, which is an array-like variable that contains all the arguments passed to the function. But the catch here is, that it’s not an array but an array like object. It has length property but not other array properties. Take a look at below example, where arguments.slice call will throw an error.
有时在函数中,我们使用参数,这是一个类似于数组的变量,包含传递给函数的所有参数。 但是这里要注意的是,它不是数组,而是类似对象的数组。 它具有length属性,但没有其他数组属性。 看下面的示例,其中arguments.slice调用将引发错误。
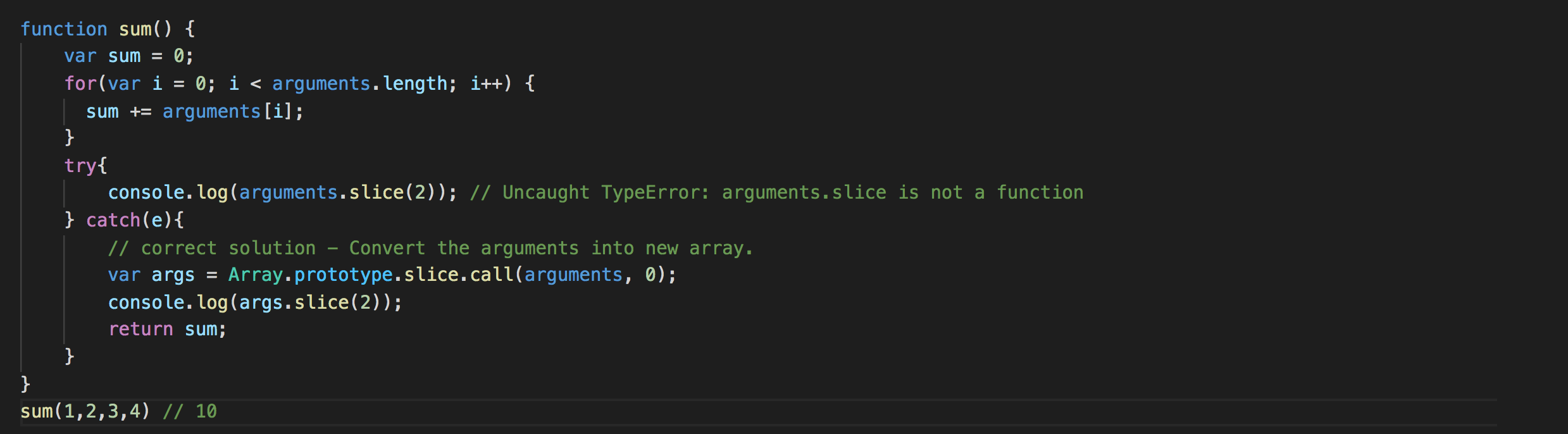
了解这一点 (Understanding this)
This is my all time favourite topic in javascript. In any function, this is a reserved keyword that refers to the owner of the function. For example: when calling a function defined on an object, it will be the object itself:
这是我一直以来最喜欢的javascript主题。 在任何函数中, 这都是一个保留关键字,它指向函数的所有者 。 例如:调用在对象上定义的函数时,它将是对象本身:

Let’s make it a little more complex. Let’s say, we have another function inside checkThis function. What will be the value of this inside inner function, any guesses??
让我们稍微复杂一点。 假设我们在checkThis函数中有另一个函数。 这个内部函数的值是多少?

So, how do we handle this? You might have seen at places using word “self”. This is where we make use of another cool javascript concept called closures.
那么,我们该如何处理呢? 您可能在使用“自我”一词的地方看到过。 这是我们利用另一个很酷的javascript概念(称为闭包)的地方。
var self = this;
var self = this;
With this declaration, you can use the value of this inside the inner function as well.
通过此声明,您也可以在内部函数中使用this的值。
Being able to change the owner of a function can lead to some confusion what this currently refers to but there are only three rules for what it can be:
能够改变一个函数的所有者可能会导致一些混乱这是什么目前是指,但只有三种它可以是什么的规则:
The object, when the function is called on the object (using the . or [] operator)
在对象上调用函数时(使用。或[]运算符)的对象
The new object when a function is called with the new operator
使用new运算符调用函数时的新对象
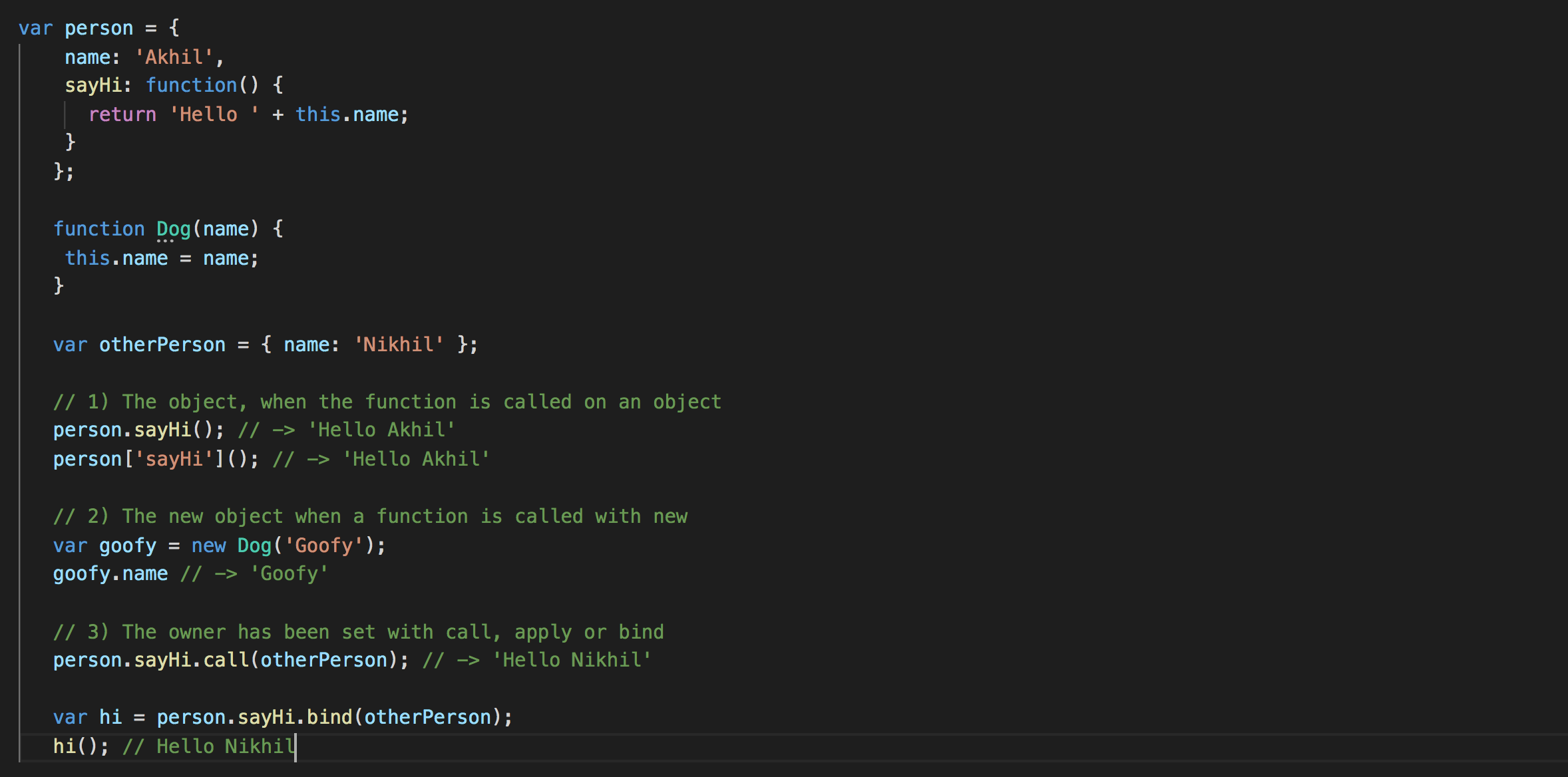
结论 (Conclusion)
This is a definitely incomplete list of some JavaScript weirdnesses and things you might trip over especially when just starting out with JavaScript. In a follow up post I want to talk about inheritance( prototypical and constructor) in JavaScript.
这绝对不是一些JavaScript怪异和您可能会绊倒的事情的完整列表,尤其是在刚开始使用JavaScript时。 在后续文章中,我想谈谈JavaScript中的继承(原型和构造函数)。
翻译自: https://medium.com/swlh/weird-parts-of-javascript-7cb30677044f