javascript 事件
Hello coders! Today, I’ll be explaining a useful and powerful event handling concept in JavaScript called Event Delegation. But first, a little background knowledge is needed.
您好编码员! 今天,我将在JavaScript中解释一个有用且功能强大的事件处理概念,称为事件委托。 但是首先,需要一些背景知识。
背景知识:事件捕获和冒泡 (Background Knowledge: Event Capturing & Bubbling)
An ‘onclick’ attribute is usually attached to a <button> element in HTML. This attribute allows the button to run an event (function) when someone clicks on it. Similar to when you add an addEventListener()
to a button from a script.
通常,将“ onclick”属性附加到HTML中的<button>元素。 此属性允许按钮在有人单击时运行事件(功能)。 与从脚本向按钮添加addEventListener()
时类似。
For ‘onclick’ event handlers, 2 phases occur: Capturing and Bubbling
对于“ onclick”事件处理程序,发生两个阶段: 捕获和冒泡
Event Capturing: browser checks from the topmost element <html> for an onclick handler on it. If it does, it will run it before moving down to the next HTML element and repeat the process until it reaches the target (clicked) element. AKA Top to Target.
事件捕获: 浏览器从最上方的元素<html>检查其上的onclick处理程序。 如果是这样,它将在 向下移动 到下一个HTML元素 之前运行它, 并重复该过程直到到达目标(单击)元素。 也就是目标。
On the other hand,
另一方面,
Event Bubbling: browser checks from the target (clicked) element for an onclick handler on it. If it does, it will run it before moving up to the next HTML element and repeat the process until it reaches the topmost element <html>. AKA Target to Top.
事件冒泡: 浏览器从目标(单击)元素上检查其上的onclick处理程序。 如果是的话,它会 向上移动 到下一个HTML元素 之前运行它 ,直到它到达最上方的元素<HTML>重复该过程。 又名目标至上。
Here’s the visual explanation of the 2 phases
这是两个阶段的直观说明
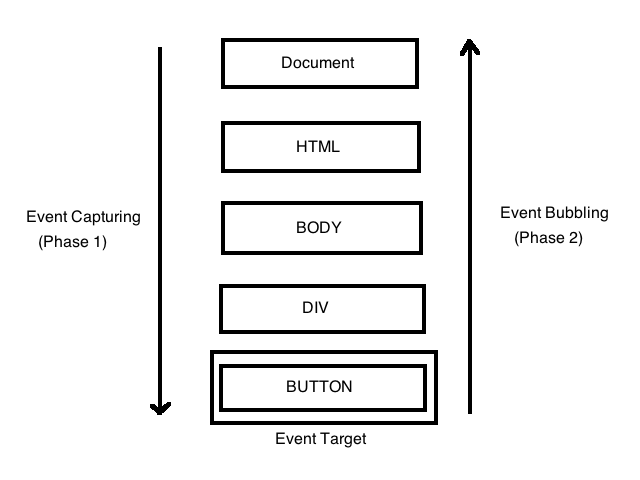
Note: In modern browsers, by default, all event handlers are registered for the bubbling phase. Source: developer.mozilla.org/en-US/docs/Learn/Java..
注意:在现代浏览器中,默认情况下,所有事件处理程序都在冒泡阶段注册。 资料来源: developer.mozilla.org/en-US/docs/Learn/Java ..
For this reason, using too much ‘onclick’ handlers can sometimes get messy, especially when you have an event that runs in an element with a parent element who has an ‘onlick’ attribute too. The parent element’s ‘onclick’ will be triggered even if you don’t want it to.
因此,使用过多的“ onclick”处理程序有时会变得混乱,尤其是当您的事件在具有父元素的元素中也具有“ onlick”属性的元素中运行时。 即使您不希望其父元素的“ onclick”也会被触发。
A solution to this issue is:
该问题的解决方案是:
Use
addEventListener()
instead使用
addEventListener()
代替Add
event.stopPropagation()
in the event function在事件函数中添加
event.stopPropagation()
Though it might seem that these 2 phases are troublesome, they are not entirely bad. In fact, as a developer, Event Capturing and Bubbling can be used to implement a more efficient way to code event handlers. Yes, this efficient event handling concept is known as Event Delegation.
尽管这两个阶段似乎很麻烦,但并不完全坏。 实际上,作为开发人员,可以使用事件捕获和冒泡来实现更有效的方式来编写事件处理程序。 是的,这种有效的事件处理概念被称为事件委托 。
活动委托 (Event Delegation)
A powerful and efficient event handling pattern to use when a lot of elements in a parent will run the same event.
一种强大且高效的事件处理模式,当父级中的许多元素将运行同一事件时使用。
Let’s take a look at an example. Say you have a “to-do list” app like this:
让我们看一个例子。 假设您有一个类似“待办事项”的应用:
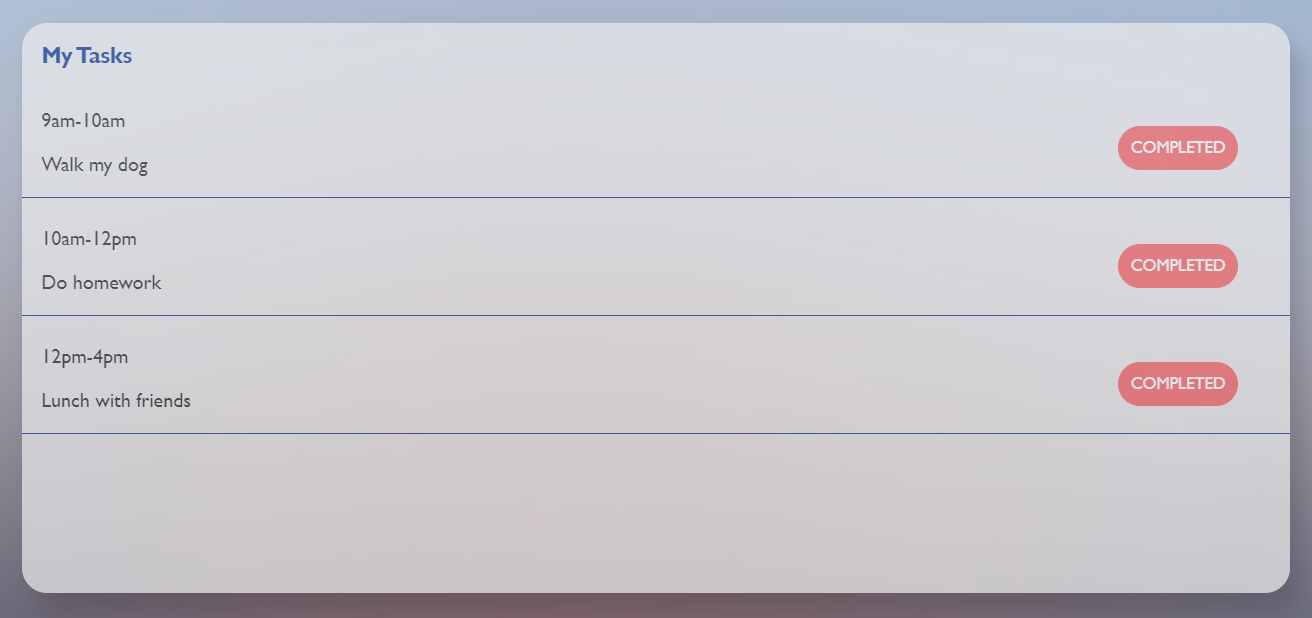
Instead of adding an event handler in each “COMPLETED” button to remove the task when the button is clicked, we can just place one event handler in the parent element.
无需在每个“完成”按钮中添加事件处理程序以在单击按钮时删除任务,我们只需在父元素中放置一个事件处理程序即可。
Here’s the HTML:
这是HTML:
<div id="task-box">
<h2>My Tasks</h2>
<div id="task">
<button class="done">COMPLETED</button>
<p>9am-10am</p>
<p>Walk my dog</p>
</div>
<div id="task">
<button class="done">COMPLETED</button>
<p>10am-12pm</p>
<p>Do homework</p>
</div><div id="task">
<button class="done">COMPLETED</button>
<p>12pm-4pm</p>
<p>Lunch with friends</p>
</div>
</div>
So instead of an ‘onclick’ to each <button>
element. We have an 'onclick' assigned to <div id="task-box">
element that will check if button was clicked and runs a removeTask() function to delete that button and its task from the to-do list. Let's code that:
因此,而不是每个<button>
元素的“ onclick”。 我们为<div id="task-box">
元素分配了一个'onclick'元素,该元素将检查按钮是否被单击,并运行removeTask()函数以从任务列表中删除该按钮及其任务。 让我们编写代码:
document.getElementById("task-box").onclick = function(event) {
if (event.target && event.target.matches("button.done")) {
removeTask(event.target.parentNode);
}
});
And the result worked perfectly:
结果非常完美:

说明 (Explanation)
When the button is clicked, the Bubbling phase checks if the button (target element) has an ‘onclick’. It does not, so it moves up to <div id = “task”> and checks again. No it doesn’t have an ‘onclick’ too, so it moves up again to <div id=”task-box”>. Aha! An ‘onclick’ is found! So it will run the function, which checks the event.target (the clicked element). If it is a button with class = “done”, then we shall remove the <div id = “task”> (parentNode of event.target) by calling the function removeTask().
单击按钮时,冒泡阶段将检查按钮(目标元素)是否具有“ onclick”。 它没有,所以它向上移动到<div id =“ task”>并再次检查。 不,它也没有“ onclick”功能,因此它再次向上移动到<div id =“ task-box”>。 啊哈! 找到一个“ onclick”! 因此它将运行该函数,该函数将检查event.target(单击的元素)。 如果它是class =“ done”的按钮,那么我们将通过调用函数removeTask()删除<div id =“ task”>(event.target的parentNode)。
就是这样! (And that’s it!)
I hope this simple example helps you visualize how Event Delegation works and how it uses Event Bubbling/Capturing to implement its concept. It is very helpful in situations like this to-do list, when elements are constantly being removed and added. It will be a hassle to add an ‘onclick’ to each button.
我希望这个简单的示例可以帮助您直观地了解事件委托的工作原理以及它如何使用事件冒泡/捕获来实现其概念。 在不断删除和添加元素的情况下,这在待办事项列表中非常有用。 向每个按钮添加“ onclick”会很麻烦。
There are so many wonderful uses to implementing Event Delegation. I hope you can apply it to your projects. Thank you for reading and if you find it helpful, please leave a ‘thumbs up’ to let me know~ If you have any questions regarding Event Capturing/Bubbling and Event Delegation, please do not hesitate to ask in the comments. Till next time, cheers!
实现事件委托有很多奇妙的用途。 我希望您可以将其应用于您的项目。 感谢您的阅读,如果对您有帮助,请留下“竖起大拇指”让我知道〜如果您对事件捕获/冒泡和事件委派有任何疑问,请随时在评论中提问。 直到下一次,加油!
翻译自: https://medium.com/javascript-in-plain-english/javascript-event-delegation-explained-4786685bb21
javascript 事件