go执行shell命令
In this tutorial, I will tell you how to create a containerised Go application which is capable of executing shell commands. Once you get used to the workflow you could change application according to your preference and get the relevant output.
在本教程中,我将告诉您如何创建一个容器化的Go应用程序,该应用程序能够执行Shell命令。 一旦适应了工作流程,就可以根据自己的喜好更改应用程序并获得相关的输出。
情境 (Scenario)
I will create a Go application which can execute shell commands in it and then I containerise the application using Docker. If you’re not familiar with Golang, you can use the code which I’ve provided below and also you’re not supposed to stick with the same process and the code I’m describing here. Alright, let’s get started.
我将创建一个可以在其中执行shell命令的Go应用程序,然后使用Docker对该应用程序进行容器化。 如果您不熟悉Golang,则可以使用下面提供的代码,并且不要遵循与我在此处描述的相同的过程。 好吧,让我们开始吧。
安装所需的软件 (Installing the required software)
Before creating the Go application, let’s set up the environment for development.
在创建Go应用程序之前,让我们设置开发环境。
Mainly, you need to get Docker installed on your computer and if you’ve already installed Docker in your computer, you can directly proceed to the next step. Or else use the link below to download it to your computer.
主要是,您需要在计算机上安装Docker,如果您已经在计算机上安装了Docker,则可以直接继续进行下一步。 或者使用下面的链接将其下载到您的计算机。
Then you need to have a text editor to create all the configuration files for this project. I prefer VS Code, so for this tutorial I’m going to use it. But, you can use any of your favourite text editor and if you need to download VS Code, use the link below.
然后,您需要一个文本编辑器来创建此项目的所有配置文件。 我更喜欢VS Code,因此在本教程中,我将使用它。 但是,您可以使用任何喜欢的文本编辑器,并且如果需要下载VS Code,请使用下面的链接。
创建Go应用程序 (Create the Go application)
If you’re good at Golang programming language, then writing a Go program wouldn’t be so hard for you. In usual scenarios, before writing a Go app, you need to install Go on your computer.
如果您精通Golang编程语言,那么编写Go程序对您来说并不难。 在通常情况下,编写Go应用程序之前,您需要在计算机上安装Go 。
But in this tutorial, we’re supposed to create a Go application inside a Docker container; so that we can use the official Golang docker image instead of installing Golang manually.
但是在本教程中,我们应该在Docker容器中创建一个Go应用程序。 这样我们就可以使用官方的Golang docker映像,而不是手动安装Golang。
Let’s create our Go app and for the demonstration purposes, I will create a basic Go app which shows the response on the browser. You can change this later to get some different outputs.
让我们创建Go应用程序,为演示起见,我将创建一个基本的Go应用程序,该应用程序在浏览器上显示响应。 您可以稍后更改它以获取一些不同的输出。
package main
import (
"fmt"
"os/exec"
"bytes"
"log"
"strings"
"net/http"
)
func main(){
http.HandleFunc("/", index_handler)
http.ListenAndServe(":8000", nil)
}
func index_handler(w http.ResponseWriter, r *http.Request){
cmd := exec.Command("ls")
cmd.Stdin = strings.NewReader("")
var out bytes.Buffer
cmd.Stdout = &out
err := cmd.Run()
if err != nil{
log.Fatal(err)
}
fmt.Fprintf(w, "Directories: \n%s",out.String())
}
Here, ls
command will list the available files and directories inside our application and display them on the browser when we request via the port we assigned. [Note: you can use different shell commands such as pwd
, echo
to get different outputs. Also, you can try out different environment variables such as PATH
by changing this Go file.]
在这里, ls
命令将列出应用程序中的可用文件和目录,并在我们通过分配的端口进行请求时在浏览器中显示它们。 [ 注意 :您可以使用不同的shell命令(例如 pwd
, echo
来获取不同的输出。 另外,您可以 通过更改此Go文件 来尝试使用其他环境变量,例如 PATH
。]
创建一个Dockerfile (Creating a Dockerfile)
To create the Dockerfile, first you need to choose a Golang Docker image with a tag. Go and see the below link and try to select one the tags you prefer.
要创建Dockerfile,首先需要选择带有标签的Golang Docker映像。 请转到下面的链接,然后尝试选择您喜欢的标签之一。
Using a Golang docker image from the above link, we can create our Dockerfile. In Dockerfile you can change commands according to your scenario and here, I’m giving you a basic configuration file with all the essential commands to build our particular docker image.
使用上面链接中的Golang Docker映像,我们可以创建Dockerfile。 在Dockerfile中,您可以根据自己的情况更改命令,在这里,我为您提供了一个基本配置文件,其中包含用于构建特定Docker映像的所有基本命令。
FROM golang:alpine
WORKDIR /go/src/app
COPY . /go/src/app
RUN go build -o /go/usr/app .
EXPOSE 8000
CMD ["/go/usr/app"]
Now both our Go application and Dockerfile are ready. Let’s build our image and run the container using that image to verify whether we can see the response on the browser.
现在我们的Go应用程序和Dockerfile都已准备就绪。 让我们构建图像并使用该图像运行容器,以验证是否可以在浏览器上看到响应。
建立Docker映像 (Building a docker image)
When all our files are ready, we can go for building our image of the Go application. Open your terminal and change directory to your Go application and run the below command to build your docker image.
当我们所有的文件准备就绪时,我们就可以构建Go应用程序的映像了。 打开终端并将目录更改为Go应用程序,然后运行以下命令以构建docker映像。
$ docker build . -t go-app
docker build
is the command we use to build a docker image and the .
and -t
flag represent the current directory and the name of your image. In my case, go-app
is the docker image name and using -t
flag you can even add a tag to your docker image as below.
docker build
是用于构建docker映像和.
和-t
标志表示当前目录和图像名称。 在我的情况下, go-app
是docker映像名称,并且使用-t
标志,您甚至可以如下所示将标签添加到docker映像。
$ docker build . -t go-app:1.0
Once executed all the steps you mentioned in Dockerfile successfully, you can run docker images
on your terminal to verify whether your docker image is created successfully. If you’ve added a tag for your image when building it, you’ll also see that tag in docker images
output.
一旦成功执行了您在Dockerfile中提到的所有步骤,就可以在终端上运行Docker docker images
,以验证是否成功创建了Docker映像。 如果在构建图像时为图像添加了标签,则还将在docker images
输出中看到该标签。
If your go-app:1.0
is in the list, you can proceed to the next step which is creating a docker container using that image.
如果您的go-app:1.0
在列表中,则可以继续执行下一步,即使用该映像创建docker容器。
创建一个Docker容器 (Creating a Docker container)
You can create a docker container using the command below on your terminal.
您可以在终端上使用以下命令创建docker容器。
$ docker run -p 8080:8000 --name go-c1 go-app:1.0
docker run
is the command we use to create a container from a docker image. In the above command, we used -p
flag to export ports for our container. In -p 8080:8000
command, the port 8080
is our local port and the port 8000
is the one which our container runs.
docker run
是用于从docker映像创建容器的命令。 在上面的命令中,我们使用了-p
标志来导出容器的端口。 在-p 8080:8000
命令中,端口8080
是我们的本地端口,端口8000
是我们的容器运行的端口。
Also, you can use the --name
flag to set a name for the container and in this case, go-c1
is the name of the container and at the end of the container name, you need to specify the docker image name which you used to create the docker container.
另外,您可以使用--name
标志设置容器的名称,在这种情况下, go-c1
是容器的名称,并且在容器名称的末尾,您需要指定docker映像名称用于创建docker容器。
Once you successfully executed the docker run
command, you can list all your running containers using docker ps
command. [Note: use another tab of your terminal to run docker ps
command since the above command will not release the terminal until you cancel out the process]
成功执行docker run
命令后,您可以使用docker ps
命令列出所有正在运行的容器。 [ 注意 :使用终端的另一个选项卡运行 docker ps
命令,因为上述命令 只有在 取消该过程后才会释放终端]
If you see your container in docker ps
command, then you’re almost done with your development.
如果您在docker ps
命令中看到了您的容器,那么您的开发就差不多完成了。
在浏览器上查看响应 (See the response on browser)
Once you completed all the above steps, you can open the browser and navigate to localhost:port
. If you’re still unsure about the port which you need to append after localhost
, you can use docker port
command to verify it as below.
完成上述所有步骤后,您可以打开浏览器并导航到localhost:port
。 如果仍然不确定需要在localhost
之后添加的端口,则可以使用docker port
命令进行如下验证。
$ docker port <container-id>
Get the container id from docker ps
command and copy-past as below. Then you will get the port which runs your docker container locally.
从docker ps
命令获取容器ID,如下所示进行复制docker ps
。 然后,您将获得在本地运行docker容器的端口。
$ docker port 329ea194a282//output
8000/tcp -> 0.0.0.0:8080
Here you can see the application runs on port 8080
locally so that go to the browser and navigate to localhost:8080
to see the response.
在这里,您可以看到该应用程序在本地8080
端口上运行,因此请转到浏览器并导航到localhost:8080
以查看响应。
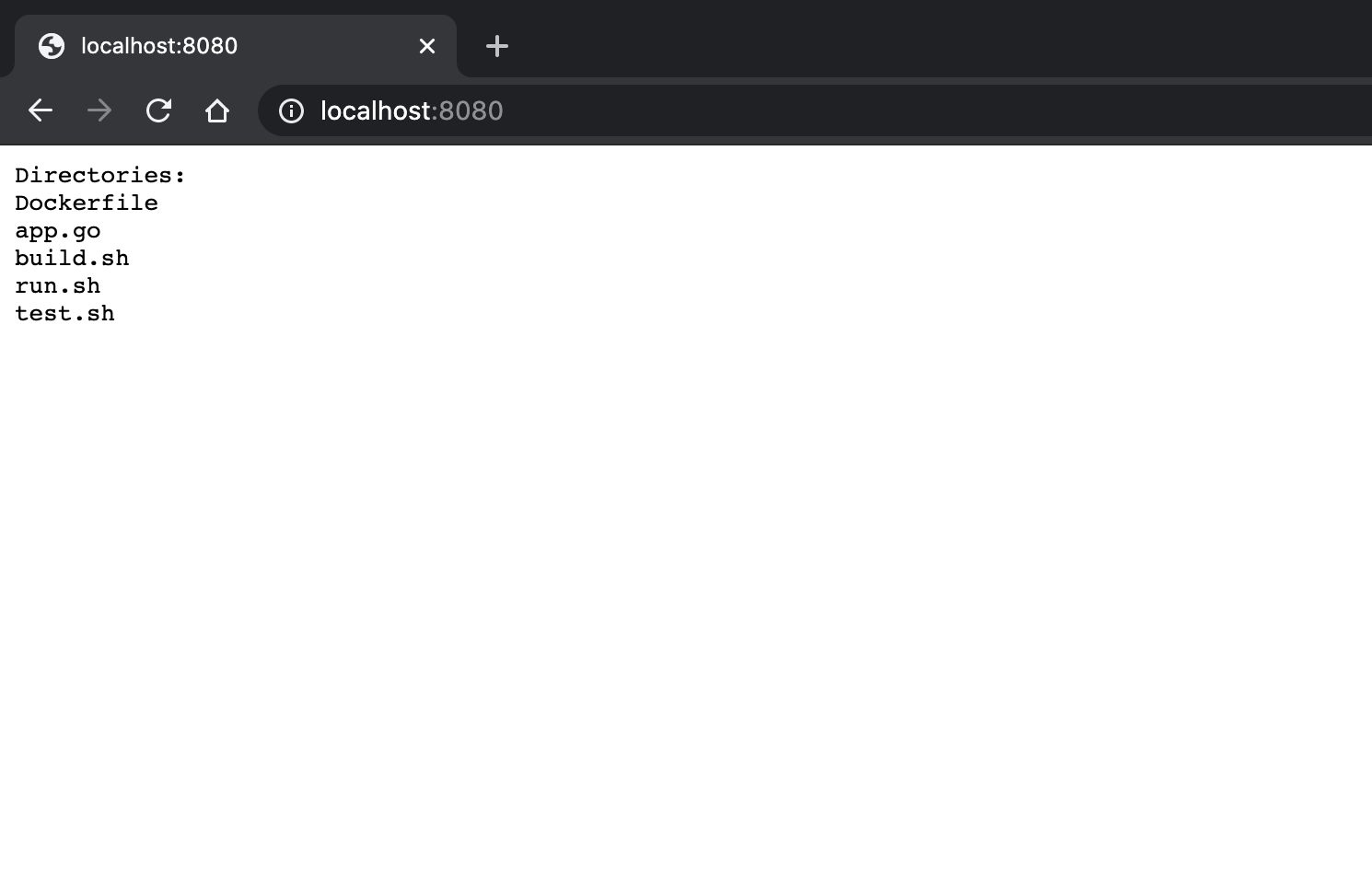
结论 (Conclusion)
Congratulations! 🎉
恭喜你! 🎉
You have created your Go application which can run shell commands inside a docker container. You can change your Go app according to your scenario and try out different outputs accordingly.
Ÿ欧已经创建了可泊坞窗容器内运行shell命令你去申请。 您可以根据自己的情况更改Go应用,然后相应地尝试不同的输出。
相关故事 (Related Stories)
Thanks for reading. I hope you found the information useful in this article. If you have any questions, feel free to leave a response below.
谢谢阅读。 希望您在本文中找到有用的信息。 如有任何疑问,请在下面留下您的答复。
go执行shell命令