Redis is a very powerful distributed caching engine and offers very low latency key-value pair caching. If used in the right business context, Redis can significantly boost application performance. In this article we will do a walk through of how to use Redis Cache from the perspective of a .NET Core Serverless HTTP function. I intend to demonstrate the simplicity and elegance of Redis caching through the following topics:
Redis是一个非常强大的分布式缓存引擎,并提供了非常低延迟的键值对缓存。 如果在正确的业务环境中使用,Redis可以大大提高应用程序性能。 在本文中,我们将从.NET Core无服务器HTTP函数的角度出发,逐步介绍如何使用Redis缓存。 我打算通过以下主题展示Redis缓存的简洁性和优雅性:
- Installing Redis locally and on Azure 在本地和Azure上安装Redis
- Using the command line tools of Redis 使用Redis的命令行工具
- Scripting the installation of Azure Redis via PowerShell and ARM templates 通过PowerShell和ARM模板编写Azure Redis安装脚本
- Writing some sample C# code to demonstrate the key-value pair caching aspect of Redis using a sample Azure HTTP trigger function 编写一些示例C#代码以使用示例Azure HTTP触发函数演示Redis的键值对缓存方面
- Benchmarking the latency and throughput of Redis using simple C# client code 使用简单的C#客户端代码对Redis的延迟和吞吐量进行基准测试
什么是分布式缓存? (What is a distributed cache?)
What is a cache? Think of it as a repository of frequently used data which can be accessed very speedily, thereby improving application performance. If we go back to the early days of .NET Framework 1.0, ASP.NET provided a means of caching objects in the memory of the worker process. The cached data was confined to the local server. If your objects supported binary serialization then using the in-proc Cache was fairly easy. It was all fine until you faced the need for scaling out your web servers to more than one instance. How do you keep the in-proc cache on 2 or more web servers in sync?
什么是缓存? 可以将其视为经常使用的数据的存储库,可以快速访问这些数据,从而提高应用程序性能。 如果我们回到.NET Framework 1.0的早期,ASP.NET提供了一种在工作进程的内存中缓存对象的方法。 缓存的数据仅限于本地服务器。 如果您的对象支持二进制序列化,那么使用进程内缓存非常简单。 一切都很好,直到您需要将Web服务器扩展到多个实例为止。 如何使2个或更多Web服务器上的进程内缓存保持同步?
In the scenario of a load balanced farm with more than one web servers, if Web server 1 made an update to a record and invalidated its cache, there is no way for Web server 2 to get that knowledge This is where distributed cache products like Memcached and Redis change the game by taking the cache out of the local web server and placing it on an external server(s). Notice the plural. Yes — the distributed cache could itself scale out horizontally.
在具有多个Web服务器的负载平衡服务器场的情况下,如果Web服务器1更新了一条记录并使它的缓存无效,则Web服务器2将无法获得该知识。这就是分布式缓存产品(如Memcached) Redis通过将缓存移出本地Web服务器并将其放置在外部服务器上来更改游戏。 注意复数。 是的-分布式缓存本身可以水平扩展。
分布式缓存有帮助的方案 (Scenarios where a distributed cache helps)
新闻网站的登录页面 (Landing page of a news web site)
If you were to hit bbc.co.uk, one cannot help but notice how fast the page gets loaded. I am not privy to the knowledge if BBC is using Redis or any other caching technology or it could just be a case of a super-fast CMS database. However, the site is an example of what a good page load response time should be and can be fairly easily implemented by caching. If you see the results from the Chrome Devtools output, the entire home page loaded in about 1.5 seconds!
如果您要访问bbc.co.uk ,那么您不禁会注意到页面加载的速度。 我不了解BBC是否正在使用Redis或任何其他缓存技术,或者仅是超快速CMS数据库的情况。 但是,该站点是一个很好的页面加载响应时间的示例,并且可以通过缓存轻松实现。 如果您从Chrome Devtools输出中看到结果,则整个主页将在约1.5秒内加载!
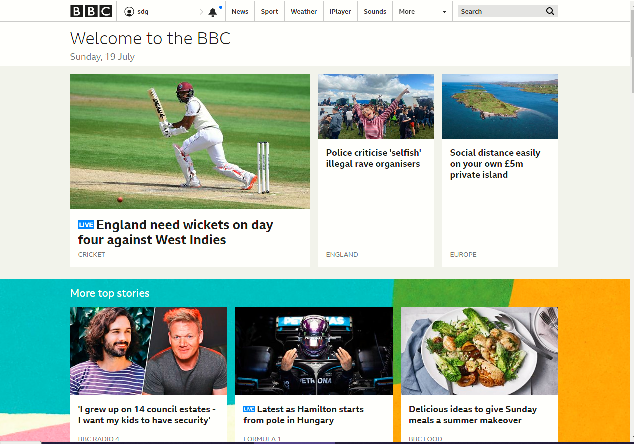
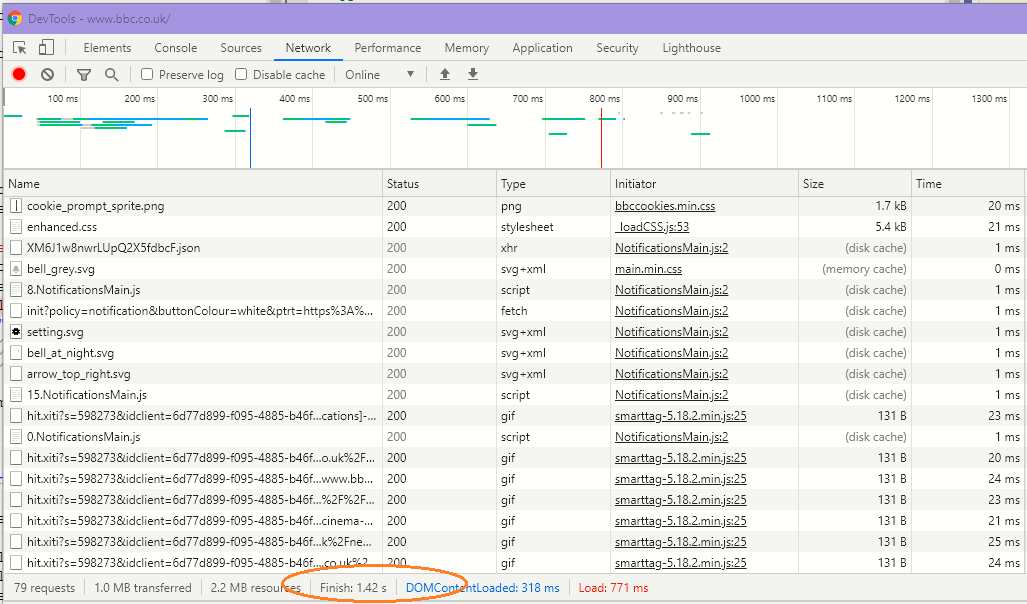
电子商务门户的购物车 (Shopping cart of an eCommerce portal)
Using a cache for storing transient data such as user session and shopping cart can save expensive database round trips.
使用高速缓存存储瞬态数据(例如用户会话和购物车)可以节省昂贵的数据库往返行程。
任何具有大量静态数据或不经常更改数据的应用程序 (Any application which has lots of static data or infrequently changing data)
Any line of business application where there is infrequently changing static data held in the database. Example — Relational tables which store lookup data like countries, item codes, and static customer information. For most practical purposes there is a lot of mileage to gain if round trips to the database can be minimized.
数据库中不经常更改静态数据的任何业务应用程序。 示例—关系表,用于存储查找数据,例如国家/地区,商品代码和静态客户信息。 对于大多数实际目的,如果可以最大程度地减少数据库往返次数,那么将会有很多收获。
像Facebook和Netfix这样的公司如何使用分布式缓存? (How do companies like Facebook and Netfix use distributed cache?)
This is an old videoby Mark Zuckerberg where he talks about how memcached helped improve the performance.
这是马克·扎克伯格(Mark Zuckerberg)的老录像,他在其中谈到了内存缓存如何帮助提高性能。
and this one from Netflix:
而这是来自Netflix的:
微软世界中缓存的历史 (A bief history of caching in the Microsoft world)
ASP.NET Cache: When Microsoft introduced ASP.NET , a light weight in-memory cache came along with the framework.
ASP.NET缓存 :当Microsoft引入ASP.NET时,框架附带了轻量级的内存中缓存。
Session state server: An integral part of .NET Framework. This was a stand-alone daemon that let multiple web servers store their session data in the process memory of this executable. You could re-configure your web.config and have all session state stored in a central server, independent of any single web server in a load balanced farm.
会话状态服务器 :.NET Framework的组成部分。 这是一个独立的守护程序,使多个Web服务器将其会话数据存储在此可执行文件的进程内存中。 您可以重新配置web.config,并将所有会话状态存储在中央服务器中,而与负载平衡场中的任何单个Web服务器无关。
Microsoft Appfabric: Microsoft’s attempt to go for a full blown distributed cache. Microsoft has ended support for this product
Microsoft Appfabric :Microsoft尝试使用完整的分布式缓存。 Microsoft已终止对此产品的支持
- Third party products like NCache, Memcached and Redis 第三方产品,例如NCache,Memcached和Redis
什么是Redis? (What is Redis?)
Redis is an open source distributed in-memory key-value pair database and message broker. The acronym stands for Remote Dictionary Server. Put simplistically, Redis is a daemon running on a Linux box and listening on incoming connections on a well published port such as 6379. Redis goes a step ahead of in-memory key-value databases by backing up transient data to a file which helps in rapid recovery in case of failures. It is more sophisticated than traditional in-memory key-value pair databases because it offers support for complex data types,custom server side scripting, partitioning and pub-sub capabilities. Example: A simple distributed cache product like Memcached supports key-value pairs ony.
Redis是一个开源的分布式内存键值对数据库和消息代理。 首字母缩写词代表远程词典服务器。 简而言之,Redis是一个运行在Linux机器上的守护程序,它监听发布良好的端口(例如6379)上的传入连接。Redis通过将瞬态数据备份到文件中而在内存键值数据库方面领先一步。发生故障时Swift恢复。 它比传统的内存中键/值对数据库更加复杂,因为它提供了对复杂数据类型,自定义服务器端脚本,分区和发布订阅功能的支持。 示例:像Memcached这样的简单分布式缓存产品仅支持键/值对。
如何调试和开发与Redis交互的应用程序? (How do I debug and develop my application which interacts with Redis?)
While coding around Redis you have the the following options:
在围绕Redis进行编码时,您可以使用以下选项:
- Install Redis locally 在本地安装Redis
- Abstract away Redis by using an in-memory abstraction (IDistributedCache) 通过使用内存中抽象(IDistributedCache)将Redis抽象化
of interacting with directly with the Redis client or through an abstraction layer. Redis a client-server product which listens on a specific port for incoming requests
直接与Redis客户端进行交互或通过抽象层进行交互的过程。 Redis客户端-服务器产品,该产品在特定端口上侦听传入的请求
选项-我何时需要在本地安装Redis? (Option — When would I need to install Redis locally?)
The following scenarios come to my mind:
我想到以下情形:
- You are using the advanced features of Redis such as pub-sub and need to develop/debug locally to test the behavior 您正在使用Redis的高级功能(例如pub-sub),并且需要在本地进行开发/调试以测试行为
- You are using simple key-value caching, you intend on doing a smoke test of your ASP.NET Core Web app or ASP.NET Azure trigger function and want to debug locally with an actual Redis implementation 您正在使用简单的键值缓存,打算对ASP.NET Core Web应用程序或ASP.NET Azure触发功能进行烟雾测试,并希望使用实际的Redis实现在本地进行调试
选项-仅使用内存抽象(IDistributedCache)何时足够? (Option — When is it enough to just use the in-memory abstraction (IDistributedCache)?)
If your scope is limited to key-value pair caching then the interface IDistributedCache is your first point of call. The interface IDistributedCache is implemented in the assembly Microsoft.Extensions.Caching.Abstractions.dll. I have presented some of the methods below:
如果您的范围仅限于键值对缓存,则接口IDistributedCache是您的第一个调用点。 接口IDistributedCache在程序集Microsoft.Extensions.Caching.Abstractions.dll中实现 。 我介绍了以下一些方法:
public interface IDistributedCache
{
public byte[] Get (string key);
public void Refresh (string key);
public void Remove (string key);
public void Set (string key, byte[] value, Microsoft.Extensions.Caching.Distributed.DistributedCacheEntryOptions options);
}
在Windows上本地安装Redis服务器和客户端 (Installing Redis server and client locally on Windows)
Option — Redis has been coded and tested on Linux. Fortunately, Microsoft is maintaining the Windows port of Redis and can be downloaded from here .
选项-Redis已在Linux上进行了编码和测试。 幸运的是,Microsoft正在维护Redis的Windows端口,可以从此处下载。
Option — To run the most recent version of Redis, you could run Redis via Windows Subsystem for Linux.
选项-要运行Redis的最新版本,您可以通过Windows Subsystem for Linux运行Redis 。
If you are following the first option, which is good enough for simple key-value caching, then further steps are below:
如果您遵循第一个选项(对于简单的键值缓存就足够了),那么下面是进一步的步骤:
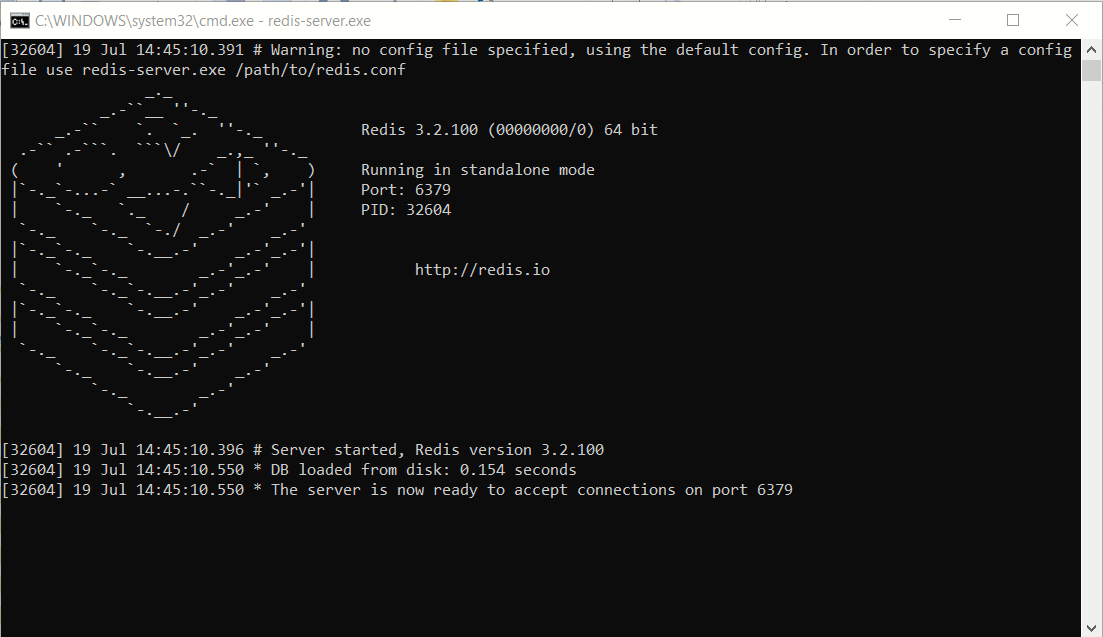
Download and extract the contents to a local folder. E.g. C:\RedisServer
下载内容并将其解压缩到本地文件夹。 例如C:\ RedisServer
Navigate to c:\RedisServer\ and type ‘redis-server.exe’ and then hit ENTER. If everything went well then you can expect this on the CMD prompt
导航到c:\ RedisServer \并键入' redis-server.exe ',然后按Enter。 如果一切顺利,那么您可以在CMD提示符下期待它
- You should see the PORT number (6379) where Redis is listening for active connections 您应该看到Redis正在侦听活动连接的端口号(6379)
- If the port used by Redis is already locked down by another process then you could use a command line option to specify the port. 如果Redis使用的端口已被另一个进程锁定,则可以使用命令行选项指定端口。
Redis命令行客户端 (Redis command line client)
When you download the Windows 10 port of Redis , the package also includes a client executable redis-cli.exe. The CLI can help you test the server and carry out basic data manipulations as shown in the examples below.
当您下载Windows 10 Redis端口时,该软件包还包含一个客户端可执行文件redis-cli.exe 。 CLI可以帮助您测试服务器并执行基本数据操作,如下面的示例所示。
如何启动客户端并连接到Redis服务器? (How to launch the client and connect to the Redis server?)
When installed locally:
在本地安装时:
redis-cli.exe -h localhost -p 6379
From a virtual machine on Azure. Remember to enable non-SSL port because the Redis client may not be compatible with SSL
从Azure上的虚拟机。 记住要启用非SSL端口,因为Redis客户端可能与SSL不兼容
redis-cli.exe -h <host name from Azure> -p 6379 -a <password from Azure>
命令示例—列出所有键 (Example command — List all keys)
redis localhost:6379> keys * 39) "foo:rand:000000000016"
40) "foo:rand:000000000017"
41) "foo:rand:000000000018"
42) "foo:rand:000000000019"
43) "mykey-f9d21bca-48f6-4cf8-b68d-1652b9533633"
44) "mykey-43d5b08a-5b60-4c72-8820-dcb6f6372b4b"
命令示例—删除特定密钥 (Example command — Delete a specific key)
redis localhost:6379> del mykey-0001
命令示例—删除所有键 (Example command — Delete all keys)
redis localhost:6379> flushall
示例命令—设置键值缓存的项目 (Example command — Set a key-value cached item)
localhost:6379> set key001 value001
Ok
示例命令—获取一个缓存项并提供密钥 (Example command — Get a cached item give the key)
localhost:6379> get key001
"value001"
C#客户端应用程序—使用StackExchange通用客户端进行编程 (C# client application — Programming with StackExchange general purpose client)
We will continue to confine our discussions to using Redis as a key-value pair cache. Redis has a .NET package which wraps up the underlying TCP/IP communications and gives us a nice IDistributedCache to work with.
我们将继续讨论将Redis用作键值对缓存。 Redis有一个.NET包,它包装了基础的TCP / IP通信,并为我们提供了很好的IDistributedCache 。
哪个NUGET包? (Which NUGET package?)
StackExchange.Redis is a managed wrapper which abstracts the low level client server interactions with the Redis server. This package is completely dependency injection aware.
StackExchange.Redis是一个托管包装,它抽象了低级客户端服务器与Redis服务器的交互。 这个包是完全依赖注入的。
如何在不依赖注入的情况下创建IDistributedCache实例 (How to create an instance of IDistributedCache without Dependency Injection)
This snippet demonstrates creating an instance of IDistributedCache via explicit construction, given the host and port number. This is a very simple example and I would recommend following the DI route
此代码段演示了在给定主机和端口号的情况下,通过显式构造创建IDistributedCache实例的过程 。 这是一个非常简单的示例,我建议您遵循DI路线
[TestMethod]
public void BasicCreation()
{
try
{
string server = "localhost";
string port = "6379";
string cnstring = $"{server}:{port}";
var redisOptions = new RedisCacheOptions
{
ConfigurationOptions = new ConfigurationOptions()
};
redisOptions.ConfigurationOptions.EndPoints.Add(cnstring);
var opts = Options.Create<RedisCacheOptions>(redisOptions);
IDistributedCache cache = new Microsoft.Extensions.Caching.StackExchangeRedis.RedisCache(opts);
string expectedStringData = "Hello world";
cache.Set("key003", System.Text.Encoding.UTF8.GetBytes(expectedStringData));
var dataFromCache = cache.Get("key003");
var actualCachedStringData = System.Text.Encoding.UTF8.GetString(dataFromCache);
Assert.AreEqual(expectedStringData, actualCachedStringData);
}
catch (Exception ex)
{
Trace.WriteLine(ex.ToString());
throw;
}
}
如何通过依赖注入创建IDistributedCache的实例? (How to create an instance of IDistributedCache via dependency injection?)
This snippet demonstrates creating an instance of IDistributedCache via the ServiceCollection DI container, given the host and port number.
此代码段演示了在给定主机和端口号的情况下, 如何通过ServiceCollection DI容器创建IDistributedCache的实例。
[ClassInitialize]
public static void Init(TestContext context)
{
var builder = new ConfigurationBuilder();
builder.AddJsonFile("settings.json", optional: false);
Config = builder.Build();
ServiceCollection coll = new ServiceCollection();
coll.AddStackExchangeRedisCache(options =>
{
string server = Config["redis-server"];
string port = Config["redis-port"];
string cnstring = $"{server}:{port}";
options.Configuration = cnstring;
});
var sprovider = coll.BuildServiceProvider();
//Use sprovder to create an instance of IDistributedCache
var cache = sprovider.GetService<IDistributedCache>();
}
如何为执行管理操作的IServer接口创建实例? (How to create an instance the IServer interface for performing management operations?)
The IServer interface is useful for managing an instance of Redis cache.
IServer接口对于管理Redis缓存实例非常有用。
//The overridable Configure method of a Azure function Startup class
public override void Configure(IFunctionsHostBuilder builder)
{
var configurationBuilder = new ConfigurationBuilder();
configurationBuilder.AddEnvironmentVariables();
IConfiguration config = configurationBuilder.Build();
builder.Services.AddSingleton<IConfiguration>(config);
builder.Services.AddSingleton<RedisConfiguration>(provider => new RedisConfiguration
{
ConnectionStringTxn = provider.GetRequiredService<IConfiguration>()["REDISDEMO_CNSTRING"]
});
builder.Services.AddSingleton<REDIS.IServer>(this.CreateRedisServerCallBack);
}
private REDIS.IServer CreateRedisServerCallBack(IServiceProvider provider)
{
var redisConfig = provider.GetService<RedisConfiguration>();
var cnstringAdmin = redisConfig.ConnectionStringAdmin;
//You need allowAdmin=true to call methods .FlushDatabase and .Keys()
//https://stackexchange.github.io/StackExchange.Redis/Basics.html
var redis = REDIS.ConnectionMultiplexer.Connect(cnstringAdmin);
var firstEndPoint = redis.GetEndPoints().FirstOrDefault();
if (firstEndPoint == null)
{
throw new ArgumentException("The endpoints collection was empty. Could not get an end point from Redis connection multiplexer.");
}
return redis.GetServer(firstEndPoint);
}
public class RedisConfiguration
{
public string ConnectionStringAdmin => $"{this.ConnectionStringTxn},allowAdmin=true";
public string ConnectionStringTxn { get; internal set; }
public override string ToString()
{
return $"{ConnectionStringTxn}";
}
}
IServer或IDistributedCache-我们需要哪一个? (IServer or IDistributedCache — which one do we need ?)
The interface IDistributedCache will address the requirements if you key-value pair caching is all you need. However, if you want to manage the Redis cache server itself (e.g. flush the cache, enumerate keys, etc.) then the interface IServer is more powerful
如果只需要键值对缓存,则IDistributedCache接口将满足要求。 但是,如果要管理Redis缓存服务器本身(例如,刷新缓存,枚举键等),则接口IServer会更强大
如何使用IDistributedCache而不需要在本地安装Redis服务器? (How to work with IDistributedCache without having to intall Redis server locally?)
The class MemoryDistributedCache provides an inproc implementation of IDistributedCache and very useful during local development and debugging.
该类MemoryDistributedCache提供IDistributedCache的进程内实现和地方发展和调试过程中非常有用的。
[TestMethod]
public void ExampleTestMethod()
{
var expectedData = new byte[] { 100, 200 };
var opts = Options.Create<MemoryDistributedCacheOptions>(new MemoryDistributedCacheOptions());
IDistributedCache cache = new MemoryDistributedCache(opts);
cache.Set("key1", expectedData);
var cachedData = cache.Get("key1");
Assert.AreEqual(expectedData, cachedData);
//Use the variable cache as an input to any class which expects IDistributedCache
}
如何在本地开发过程中有条件地注入MemoryDistributedCache的IDistributedCache实现? (How to conditionally inject an IDistributedCache implementation of MemoryDistributedCache during local development?)
You are using the key-value caching functionality of Redis. You are coding an Azure function (could be an ASP.NET Core Web app) and you want to develop and debug the code. Do you need to install Redis locally? Not neccessary. Through a couple of lines of clever DI, you can “fool” your classes to use the MemoryDistributedCache implementation of the interface IDistributedCache.
您正在使用Redis的键值缓存功能。 您正在编码一个Azure函数(可以是ASP.NET Core Web应用程序),并且您想要开发和调试代码。 您需要在本地安装Redis吗? 没必要。 通过几个聪明的DI线,你可以“傻瓜”你的类使用MemoryDistributedCache实现接口IDistributedCache的。
In this snippet we are checking for the the value of an environment variable and conditionally injecting the desired implementation of IDistributedCache
在此代码段中,我们正在检查环境变量的值,并有条件地注入IDistributedCache的所需实现。
[TestMethod]
public void Condition_Injection_Of_IDistributedCache()
{
var builder = new ConfigurationBuilder();
builder.AddJsonFile("settings.json", optional: false);
Config = builder.Build();
ServiceCollection coll = new ServiceCollection();
if (System.Environment.GetEnvironmentVariable("localdebug") == "1")
{
coll.AddDistributedMemoryCache();
}
else
{
coll.AddStackExchangeRedisCache(options =>
{
string server = Config["redis-server"];
string port = Config["redis-port"];
string cnstring = $"{server}:{port}";
options.Configuration = cnstring;
});
}
var provider = coll.BuildServiceProvider();
var cache = provider.GetService<IDistributedCache>();
}
Redis Azure入门 (Getting started with Redis on Azure)
使用门户创建Redis缓存 (Creating a Redis cache using the Portal)
Creating an instance of Redis cache using the Azure portal is fairly easy. Step by step guidance from Microsoft can be found here. You should remember to select the correct region and the pricing tier. The region is important because you want the cache to be in close proximity to your application/web servers for lowest latency. You should select the pricing tier as per your requirements. As of July 2020, I am not aware of any consumption plan for Redis cache, i.e. you pay a flat monthly fee. I have presented a snapshot of Redis pricing from the Azure pricing calculator page.
使用Azure门户创建Redis缓存实例非常容易。 在此处可以找到Microsoft的分步指导。 您应该记住选择正确的区域和定价层。 该区域很重要,因为您希望缓存与应用程序/ Web服务器非常接近,以使延迟最小。 您应该根据需要选择定价层。 截至2020年7月,我还没有任何关于Redis缓存的使用计划,即您每月支付固定费用。 我已经从Azure定价计算器页面提供了Redis定价的快照。
I would not mind chosing the C0 instance for a medium sized Development environment. However, for production, I would go for C2 or higher.
我不介意为中等规模的开发环境选择C0实例。 但是,对于生产而言,我会选择C2或更高级别。
如何使用PowerShell和ARM模板创建新的Azure Redis缓存? (How to create a new Azure Redis cache using PowerShell and ARM templates?)
The cmdlet New-AzResourceGroupDeployment is your friend. The ARM template for Redis cache can be found in the central ARM template’s repository here.
该cmdlet New-AzResourceGroupDeployment是您的朋友。 对Redis的缓存的ARM模板可以在中央ARM模板的存储库中找到这里 。
$envname="dev"
$resourcegroupname="rg-$envname-redis-demo"
$location="uksouth"
$rediscache="saudemo001-$envname"
$storageaccountname="stgsaudemo001"
$subscription="Pay-As-You-Go-demo" #Change this as per your subscriptions
Set-AzContext -Subscription $subscription
#
#Create resource group
#
New-AzResourceGroup -Name $resourcegroupname -Location $location -Force -Verbose
"Created resource group:{0}" -f $resourcegroupname
#
#Create storage account
#
"Creating storage account"
New-AzResourceGroupDeployment -ResourceGroupName $resourcegroupname -TemplateFile $scriptfolder\arm-storageaccount\template.json -TemplateParameterFile $scriptfolder\arm-storageaccount\parameters.json -location $location -storageAccountName $storageaccountname -Verbose
$oStorageAccount=Get-AzResource -ResourceGroupName $resourcegroupname -Name $storageaccountname
"Storage account created:{0}" -f $oStorageAccount.Name
#
#Create Redis cache
#
"Creating Redis Cache"
$urlRedisAzureArm="https://raw.githubusercontent.com/Azure/azure-quickstart-templates/master/101-redis-cache/azuredeploy.json"
New-AzResourceGroupDeployment -ResourceGroupName $resourcegroupname -TemplateUri $urlRedisAzureArm -redisCacheName $rediscache -redisCacheSKU "Basic" -existingDiagnosticsStorageAccountId $oStorageAccount.ResourceId -Verbose
The deployment can be easily automated via CI/CD on Azure Devops following the steps shown in the script above.
按照上面脚本中显示的步骤,可以通过Azure Devops上的CI / CD轻松地自动进行部署。
如何获得连接字符串? (How to get the connection string?)
You have deployed an instance of Redis cache via CI/CD. Good. But, how does your client application become aware about the existence of this cache instance. Your next step would be to update the connection string in the Configuration settings of your Azure function/Webapp
您已通过CI / CD部署了Redis缓存实例。 好。 但是,您的客户端应用程序如何知道此缓存实例的存在。 下一步将是更新Azure函数/ Webapp的配置设置中的连接字符串。
#
#Get connection parameters for the newly created Redis instance
#The variable $rediscache is defined in the snippet where we created the Redis cache
#
$redisConfig=Get-AzRedisCache -name $rediscache
$redisKeys=Get-AzRedisCacheKey -Name $rediscache
"Host name {0}" -f $redisConfig.hostname
"Port number {0}" -f $redisConfig.port
"SSL Port number {0}" -f $redisConfig.SslPort
"Key {0}" -f $redisKeys.PrimaryKey
我们如何衡量Redis缓存的性能? (How do we benchmark the performance of Redis cache?)
为什么我们需要对Redis缓存的性能进行基准测试? (Why do we need to benchmark the performance of Redis cache?)
The primary motivation for using a distributed cache is to make the application perform better. In most scenarios the central data storage becomes the bottleneck. Benchmarking the distributed cache gives us an idea of how much latency and throughput to expect from the cache for various document sizes.
使用分布式缓存的主要动机是使应用程序性能更好。 在大多数情况下,中央数据存储成为瓶颈。 对分布式缓存进行基准测试可以使我们了解到,对于各种文档大小,缓存需要多少等待时间和吞吐量。
使用开箱即用的Redis缓存redis-benchmark.exe工具 (Using the out of box Redis cache redis-benchmark.exe tool)
In the following example I am stressing the cache on my local computer with a document of size 1000 bytes for a total of 5000 requests with the default number of concurrent threads (50). To reduce the overheads of network latencies, this test was carried out from a virtual machine which is located in the same Azure location as the Redis cache. In the example below we can see that 94.56% of the requests took less than or equal to 10 milliseconds at an overall throughput of 6544.50 requests per second.
在以下示例中,我在本地计算机上使用大小为1000字节的文档来强调高速缓存,该文档用于总共5000个请求,并发线程数为默认值(50)。 为了减少网络延迟的开销,此测试是从与Redis缓存位于同一Azure位置的虚拟机进行的。 在下面的示例中,我们可以看到94.56%的请求花费了小于或等于10毫秒的时间,每秒的总吞吐量为6544.50请求。
redis-benchmark.exe -h ****.redis.cache.windows.net -p 6379 -a ******* -n 500 -d 1000 -t set,get====== GET ======
5000 requests completed in 0.76 seconds
50 parallel clients
1000 bytes payload
keep alive: 10.68% <= 1 milliseconds
10.26% <= 2 milliseconds
30.00% <= 3 milliseconds
33.58% <= 5 milliseconds
33.62% <= 6 milliseconds
37.16% <= 7 milliseconds
48.62% <= 8 milliseconds
78.00% <= 9 milliseconds
94.56% <= 10 milliseconds
97.72% <= 11 milliseconds
97.96% <= 12 milliseconds
98.32% <= 13 milliseconds
98.34% <= 19 milliseconds
98.74% <= 20 milliseconds
98.96% <= 41 milliseconds
99.02% <= 60 milliseconds
99.28% <= 61 milliseconds
99.86% <= 62 milliseconds
100.00% <= 62 milliseconds
6544.50 requests per second
结果 (Results)
有附带的源代码吗? (Is there any accompanying source code?)
The accompanying sample code can be found in this Github repo. This implements a basic Azure HTTP trigger and the Azure ARM templates and PowerShell script for deployment
随附的示例代码可在此Github存储库中找到。 这实现了基本的Azure HTTP触发器以及Azure ARM模板和PowerShell脚本以进行部署
翻译自: https://medium.com/@saurabh.dasgupta1/about-7fb96fb1f80d