rails 添加外键
Introduction
介绍
If you are brand new to ruby on rails like I am you may have been wondering about the implementation of a like ecosystem into your brand new application. If so, you may have noticed that you have to take in a myriad of things like account validations, how to present the button, and how your models are associated in your database. All of this stuff gets a little complicated, but the great part is that once you do it once you know how to implement it every single time! My goal in this tutorial is to walk you through that first time so that you can implement a like button on your next project easily and efficiently. Okay enough talk, let’s get started!
如果您是像我这样刚接触Ruby的人,那么您可能一直想知道如何在全新的应用程序中实现类似的生态系统。 如果是这样,您可能已经注意到,您必须进行许多事情,例如帐户验证,如何显示按钮以及如何在数据库中关联模型。 所有这些东西都变得有点复杂,但是最重要的是,一旦您做到了,便一次又一次知道如何实现它! 我在本教程中的目标是引导您第一次学习,这样您可以轻松高效地在下一个项目中实现一个赞按钮。 好的对话,让我们开始吧!
QUICK NOTE: This tutorial is meant for people who already have a good grasp of ruby on rails. If you struggle with associations, validations, or authentication I advise you to learn those aspects of rails first. I also strongly suggest using the devise gem for your authentication. Thanks!
快速说明:本教程适用于已经非常了解Ruby的人。 如果您在关联,验证或身份验证方面遇到困难,我建议您首先学习Rails的那些方面。 我也强烈建议您使用devise gem进行身份验证。 谢谢!
Step 1: Setting Up Your Associations
步骤1:建立关联

The first step in our like button journey is to map out our associations. For this example, we are going to keep it simple and say that we have a user, a post, and of course a like model. Our user model will have many likes, and so will our post model. This is because the user can like many posts (albeit they can only like each post once), and our post can be liked by users many times. This means we need to make our like model a join model in our database. To do this, first add your user and post models to the database with whatever fields you like, and then using your terminal add the like model like this:
我们的“喜欢”按钮之旅的第一步是规划我们的关联。 对于此示例,我们将使其保持简单,并说我们有一个用户,一个帖子,当然还有一个类似的模型。 我们的用户模型将有很多喜欢的地方,我们的后期模型也会有很多。 这是因为用户可以喜欢许多帖子(尽管他们只能喜欢每个帖子一次),并且我们的帖子可以被用户多次喜欢。 这意味着我们需要在数据库中使like模型成为联接模型。 为此,首先将您的用户添加到模型中,并使用所需的任何字段将模型发布到数据库中,然后使用终端添加如下所示的模型:
rails g model like user_id:belongs_to post_id:belongs_to
rails g model like user_id:belongs_to post_id:belongs_to
Step 2: Setting Up a Custom Route
步骤2:设定自订路线
The next step is to set up a custom route so that when our user clicks the like button we can go to a custom method and create our like. When we do that it should look something like this:
下一步是设置自定义路线,以便当我们的用户单击“赞”按钮时,我们可以转到自定义方法并创建我们的赞。 当我们这样做时,它应该看起来像这样:

put ‘/post/:id/like’ to: ‘posts#like’, as: ‘like’
put '/post/:id/like' to: 'posts#like', as: 'like'
Here we are sending a put request with the id of the post we want to like and we are calling our like method in our controller.
在这里,我们发送了一个请求请求,其请求的ID为我们想要的帖子的ID,并在控制器中调用了like方法。
Step 3: Creating Our Like Method
步骤3:建立喜欢的方法
Next we want to add the like method in our controller that the route will enact. Since we are liking posts we will want to put this method within our posts controller. The like method will look something like this:
接下来,我们想在控制器中添加类似方法,以执行路由。 由于我们喜欢帖子,因此我们希望将此方法放入我们的posts控制器中。 like方法将如下所示:

The only caveat here is that current_user will be replaced with whatever you use to represent the user that is logged in. If you are using the devise gem for authentication then this code will work for you since current_user is a method that comes with devise.
唯一需要注意的是,current_user将替换为您用来代表登录用户的任何内容。如果您使用devise gem进行身份验证,则此代码将为您工作,因为current_user是devise随附的方法。
Step 4: Making Sure They Can Only Like it Once
步骤4:确定他们只能喜欢一次
Now that we have our route and our controller set up, it is time to set up our model with some quick validation code. The first code we are going to write will be a way to validate using a method in our html. This code will be in our post model and look something like this:
现在我们已经设置了路线和控制器,是时候使用一些快速验证代码来设置模型了。 我们将要编写的第一个代码将是一种使用html中的方法进行验证的方法。 这段代码将在我们的帖子模型中,如下所示:

This checks to see if the user already like the post, or if they have yet to like it. If they have it will return true, and if not it will return false.
这将检查用户是否已喜欢该帖子,或者是否尚未喜欢它。 如果有,它将返回true,否则,将返回false。
Step 5: Adding a Second Validation to Our Like Model
步骤5:向我们的喜欢模型添加第二个验证
If we want to double check to make sure that our user can only like a post once, we can also add a uniqueness validation to our like model like so:
如果我们要仔细检查以确保我们的用户只喜欢一次帖子,我们还可以像这样向我们的喜欢模型添加唯一性验证:
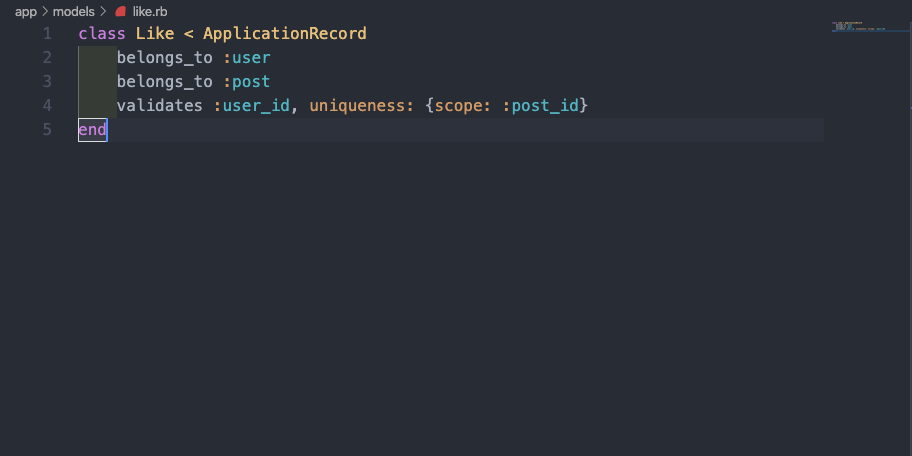
This uniqueness validation will make sure that a like’s user_id and post_id combination is unique. That way we have two methods making sure that our user can only like our post once.
这种唯一性验证将确保喜欢的user_id和post_id组合是唯一的。 这样,我们有两种方法可确保我们的用户只喜欢我们的帖子一次。
Final Step: Add the Button to Your ERB File
最后一步:将按钮添加到您的ERB文件中
Now all we have to do is add a button and a little logic to our post’s show.html.erb
file.
现在,我们要做的就是在我们帖子的show.html.erb
文件中添加一个按钮和一些逻辑。

Here we are using the user_signed_in?
method created by devise to check if our user is signed in (If you aren’t using devise you will have to make this method on your own). Then we are using our liked?
method to check if our user already liked that specific post, and if so we make our button’s disabled
attribute equal to true
. If they haven’t already liked it we have our button act like normal.
这里我们使用的是user_signed_in?
由devise创建的方法来检查我们的用户是否已登录(如果您不使用devise,则必须自行创建此方法)。 那么我们正在使用我们liked?
检查用户是否已经喜欢该特定帖子的方法,如果是,则使按钮的disabled
属性等于true
。 如果他们还不喜欢它,我们可以像平常一样操作按钮。
Conclusion
结论
That’s it you made your first like button congrats! It may seem daunting at first, but I hope that this tutorial helped steer you in the right direction to creating your first like button. If you have any questions be sure to leave a comment and I’ll try and get back to you as soon as possible. Happy coding!
就是这样,您第一次祝贺按钮就好了! 乍一看似乎令人望而生畏,但我希望本教程能够帮助您引导正确的方向来创建您的第一个“喜欢”按钮。 如果您有任何疑问,请务必发表评论,我会尽力尽快与您联系。 祝您编码愉快!
rails 添加外键