react生命周期
If you have exposure to react you have probably heard of life cycles.
如果您有React的余地,您可能已经听说过生命周期。
To understand react lifecycles, let’s understand how a web application generally behaves.
要了解React生命周期,让我们了解Web应用程序的一般行为。
If you want to briefly summarize how a web page runs from beginning to end, here is how it’s like:
如果您想简要概述网页从头到尾的运行方式,则如下所示:
- before rendering 渲染之前
- rendering 渲染
- listening for changes to state or props 聆听状态或道具的变化
- finish rendering 完成渲染
Ultimately, when you go to facebook and see a bunch of posts, the web application actually makes an api to retrieve those posts before rendering.
最终,当您访问Facebook并看到一堆帖子时,Web应用程序实际上会制作一个api,以便在渲染之前检索这些帖子。
Afterward, the application renders this piece of information(via render function). It listens to any changes or events that affect the state or props. When the state or the props of any components changes, the application gets re-rendered.
之后,应用程序渲染此信息(通过render函数)。 它侦听影响状态或道具的任何更改或事件。 当任何组件的状态或属性发生变化时,应用程序将重新呈现。
In react terms, they are represented in this diagram:
用React术语,它们在此图中表示:
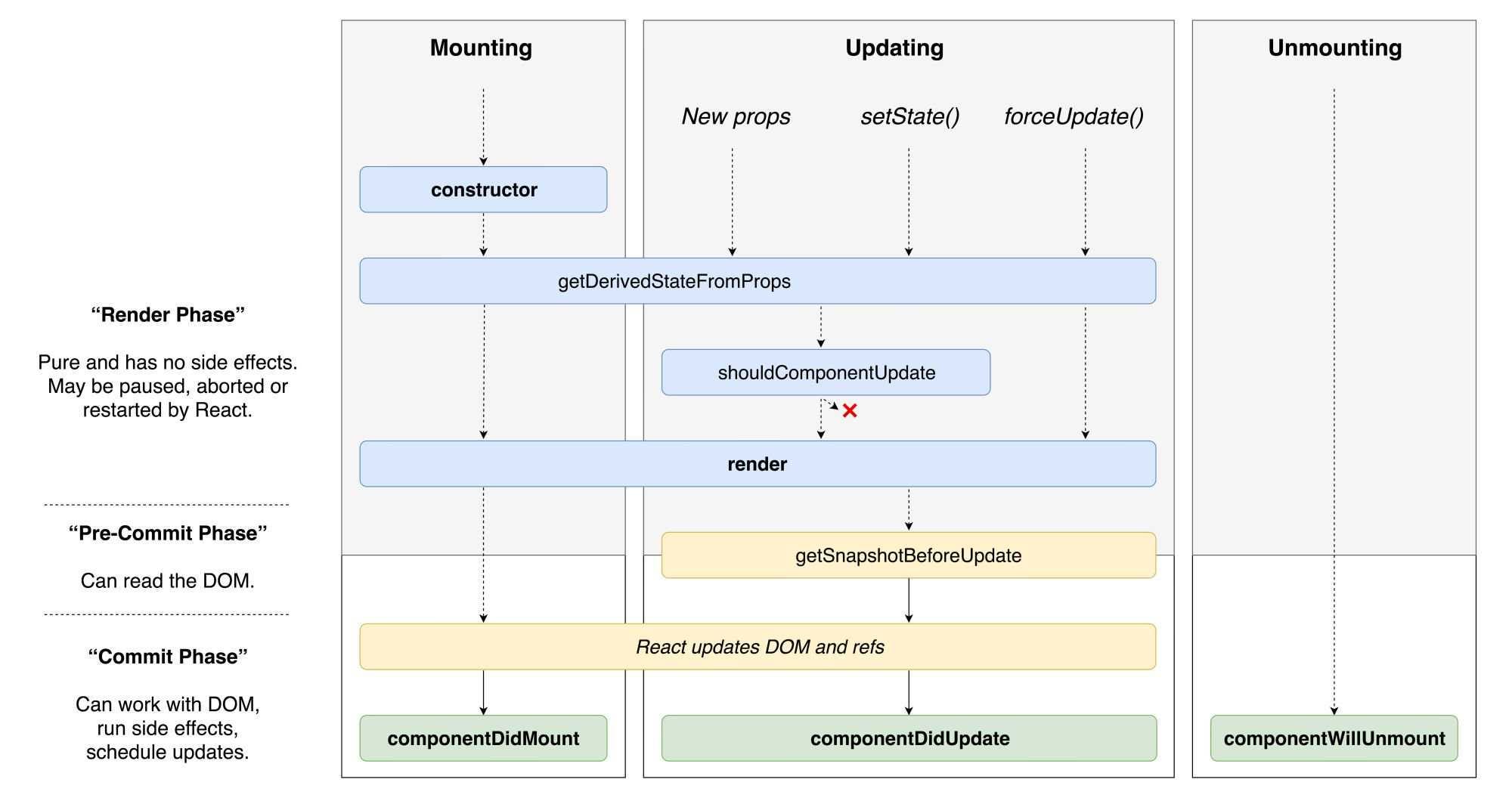
There are several lifecycles that affect how components are being rendered:
有几个生命周期会影响组件的呈现方式:
- constructor 构造函数
- componentDidUpdate componentDidUpdate
- componentDidMount componentDidMount
- render 渲染
- getDeriveStateFromProps getDeriveStateFromProps
Let’s talk about constructor. Constructor is automatically called every time an object is created from a class. If you declare a constructor, super(props) needs to be called in order to receive props properly. Otherwise, this.props will be undefined and it can lead to all kinds of unwanted bugs.
让我们谈谈构造函数。 每次从类创建对象时,都会自动调用构造函数。 如果声明构造函数,则需要调用super(props)才能正确接收props。 否则,this.props将是未定义的,并且可能导致各种有害的错误。
Constructor also bind event handlers and functions during this stage.
构造函数还在此阶段绑定事件处理程序和函数。
Here is an example of how a constructor would look like:
这是一个构造函数的示例:
class constructorExample extends React.Component {
constructor(props){
super(props);
this.state = {
fieldOne: ''
};
this.printFunc = this.printFunc.bind(this);
}
printFunc(){
console.log('printing!');
}
render(){
//returns some jsx
return (
<div>Hi My name is {this.state.fieldOne}</div>
);
}
}
How about componentDidUpdate? ComponentDidupdate gets called after componentDidMount and will only get called if the internal state of the component did changes, or if the component receive prop changes.
怎么样componentDidUpdate? ComponentDidupdate在componentDidMount之后被调用,并且仅在组件的内部状态发生更改或组件接收到属性更改时才被调用。
You can view the following for additional reference:
您可以查看以下内容以获取其他参考:
class ComponentUpdate extends React.Component {
constructor(props){
super(props);
this.state = {
fieldOne: ''
};
this.printFunc = this.printFunc.bind(this);
}
componentDidUpdate(prevProps, prevState){
if(this.props.eleOne && prevProps.eleOne !== this.props.eleOne){
//do something
}
}
render(){
//returns some jsx
return (
<div>Hi My name is {this.state.fieldOne}</div>
);
}
}
What about ComponentDidMount?
ComponentDidMount呢?
ComponentDidMount is called once, right before render is called. This is the lifecycle where you make api calls to load data remotely:
ComponentDidMount在调用render之前被调用一次。 这是您进行api调用以远程加载数据的生命周期:
class ComponentMount extends React.Component {
constructor(props){
super(props);
this.state = {
data: []
}
}
componentDidMount(){
fetch(/*some api url*/).then(res => res.json()).then(data => this.setState({ data });
}
render(){
return(
<React.Fragment>
{data.map(eachData => <div>{eachData}</div>}
</React.Fragment>
);
}
}
Render, as said before, is the part that throws the jsx to the browser, as seen above.
如前所述,渲染是将jsx投向浏览器的部分,如上所示。
GetDeriveStateFromProps is a lifecycle where you update your internal state after changes to props. Here is an example:
GetDeriveStateFromProps是生命周期,您可以在其中更改道具后更新内部状态。 这是一个例子:
class deriveComponent extends Component {
state = {
name: this.props.name,
id: this.props.userID
};
static getDerivedStateFromProps(props, state) {
// Any time the current user changes,
// Reset any parts of state that are tied to that user.
if (props.userID !== state.prevPropsUserID) {
return {
id: props.userID,
name: props.name
};
}
return null;
}
// ...
}
That’s it to the lifecycles. Why not create an application from scratch and play around with these lifecycles?
这就是生命周期。 为什么不从头开始创建应用程序并尝试这些生命周期呢?
Nothing is better than learning these concepts by applying it.
没有什么比通过应用来学习这些概念更好的了。
Happy coding!
祝您编码愉快!
翻译自: https://medium.com/swlh/understand-life-cycles-in-react-77d467f0b26c
react生命周期