vue项目最佳实践
As you all know, Vue.js is a javascript based framework that has been growing rapidly in the last few years.
众所周知,Vue.js是一个基于JavaScript的框架,在最近几年中发展Swift。
There are many reasons behind this growth, including the simplicity of the framework, easy integrations, user-friendliness, fewer restrictions. These reasons have helped Vue.js to compete with Angular and React as well. In fact, it seems that Vue is in par with Angular and React in many aspects.
这种增长背后的原因很多,包括框架的简单性,易于集成,用户友好性,更少的限制。 这些原因已经帮助Vue.js与Angular和React竞争。 实际上,Vue在许多方面似乎都与Angular和React相提并论。
However, when I searched for the limitations of Vue, I have noticed that lack of support for large scale projects is popping up in every result. Knowing VueJS, I can confidently say that is not true.
但是,当我搜索Vue的局限性时,我注意到在每个结果中都出现了对大型项目缺乏支持的现象 。 了解VueJS后,我可以自信地说那不是事实。
So, In this article, I’m going to discuss 5 best practices that you can use to organize large scale Vue.js projects.
因此,在本文中,我将讨论5种最佳实践,可用于组织大型Vue.js项目。
1.使用Vue插槽使您的代码易于理解 (1. Use Vue Slots to make your code easily understandable)
Parent-Schild relationship is one of the most used methods when it comes to connecting your components with each other, but this may not be the best option in some situations. Imagine a situation where you have a large number of child components in a single parent component, you will have to use a large number of props and emitting events to handle those child components and it will become a mess in no time. This is the exact situation you will face on a large scale project and Vue.js has a spot-on solution for this problem as well.
父子关系是组件之间相互连接的最常用方法之一,但这在某些情况下可能不是最佳选择。 想象一下在单个父组件中有大量子组件的情况,您将不得不使用大量道具和发出事件来处理这些子组件,而这将很快变成一团糟。 这是您将在大型项目中面临的确切情况,Vue.js也提供了针对此问题的现场解决方案。
Slots are used in Vue.js to provide an alternative way to represent parent-child relationships. Slots provide you an outlet to place content in new places. A basic example of slots can be shown as follows:
Vue.js中使用了插槽,以提供表示父子关系的另一种方法。 插槽为您提供了将内容放置在新位置的渠道。 插槽的基本示例如下所示:
<div class="demo-content">
<slot></slot>
</div>
When above component it rendered <slot></slot> tag will be replaced by demo-content
当上面的组件呈现的<slot> </ slot>标签将被demo-content代替
<demo-content>
<h2>Hi!</h2>
<class-name name="Welcome to Vue!"></class-name>
</demo-content>
There are many different types of slots you can use in Vue projects. But the most important thing you need to remember is slots make a huge difference to your projects when it grows and it allows you to maintain good, understandable code throughout the project.
您可以在Vue项目中使用多种不同类型的插槽。 但是,您需要记住的最重要的事情是,插槽在增长时会对您的项目产生巨大的影响,它使您可以在整个项目中维护良好的,易于理解的代码。
2.建立并共享独立的组件 (2. Build & share independent components)
Follow the F.I.R.S.T principle to build your components to be: focused, independent, reusable, small & testable.
遵循FIRST原则 ,将您的组件构建为:专注,独立,可重用,小型且可测试。
“[..] the secret to efficiently building ‘large’ things is generally to avoid building them in the first place. Instead, compose your large thing out of smaller, more focused pieces. This makes it easier to see how the small thing fits within the broader scope of your large thing.”-Addy Osmani
“ [..]有效地构建“大型”事物的秘诀通常是首先避免构建它们。 取而代之的是,将大型事物由较小 ,更集中的片段组成。 这样一来,您就可以更轻松地看到小物件如何适合您的大物件。”- Addy Osmani
You can also use tools like Bit (Github) to source-control each of your project’s components independently and share it to Bit’s component hub.
您还可以使用Bit ( Github )之类的工具独立地 控制每个项目的组件,并将其共享给Bit的组件中心。
Shared components are displayed on Bit’s component hub with auto-generated docs and live examples.
共享的组件与自动生成的文档和实时示例一起显示在Bit的组件中心上。
They can be installed using NPM or “cloned” and modified, using Bit.
可以使用NPM进行安装,也可以使用Bit进行 “克隆”和修改。
This makes it easier to find, use, and maintain components (and, therefore, easier to maintain the project).
这使得查找,使用和维护组件变得更加容易(因此,更易于维护项目)。
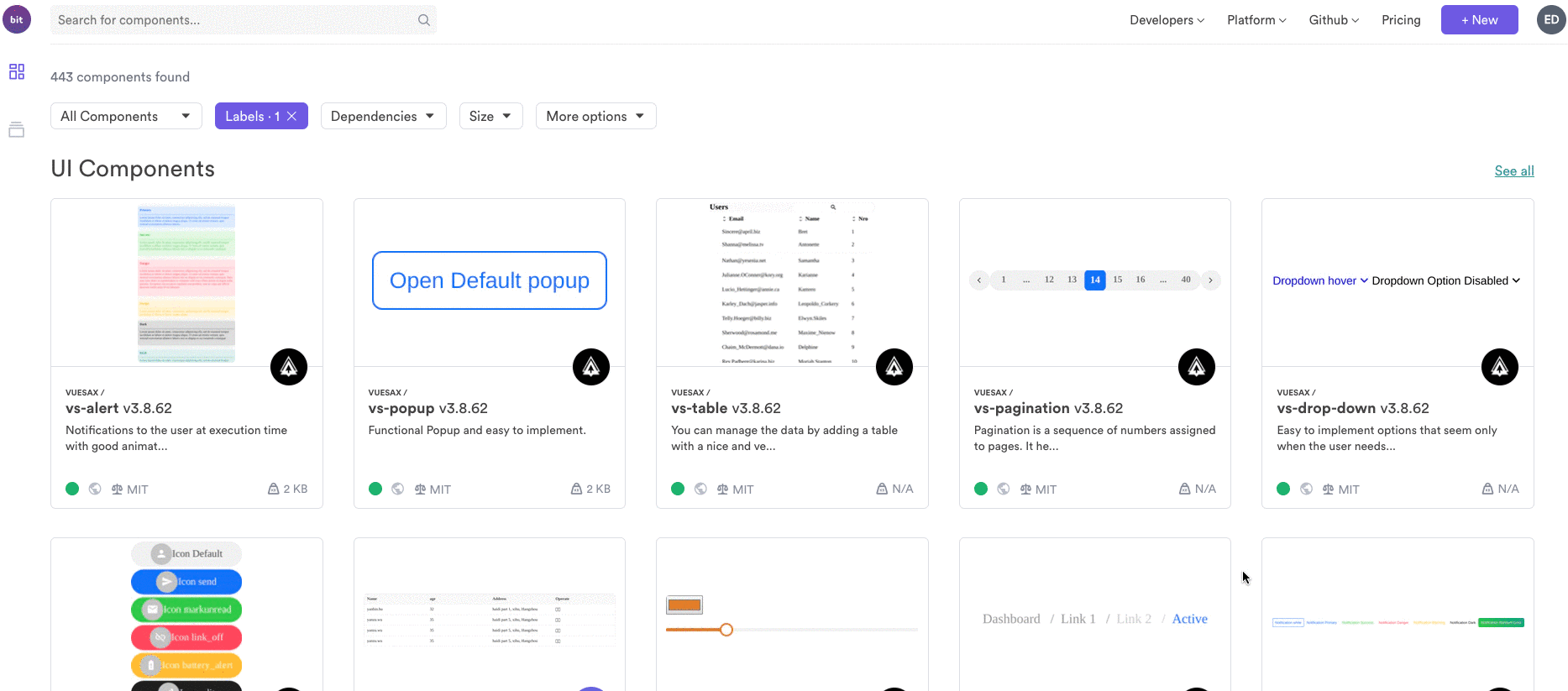
Read more about it here:
在这里阅读更多关于它的信息:
3.维护井井有条的VUEX商店 (3. Maintaining a well organized VUEX Store)
Vuex is a state management pattern in Vue.js and it acts as a centralized store for all components in your application. Over time, I have seen comments about the Vuex store saying that “Vuex restricts developers from structuring projects as they need”. But the truth is Vuex helps developers to structure their project in a more organized manner using a set of principles.
Vuex是Vue.js中的状态管理模式,它充当应用程序中所有组件的集中存储。 随着时间的流逝,我看到有关Vuex商店的评论说: “ Vuex限制了开发人员根据需要构造项目”。 但是事实是,Vuex使用一组原则帮助开发人员以更有条理的方式组织其项目。
Before getting into those principles, there are 4 main components in the Vuex store you should know. If you are familiar with these 4, you can easily structure your Vuex store in a more organized manner.
在了解这些原理之前,您应该了解Vuex存储中的4个主要组件。 如果您熟悉这4个,则可以轻松地以更有条理的方式构建Vuex商店。
- States: Used to keep data of your application 状态:用于保存您的应用程序的数据
- Getters: Used to access these state objects outside the store. Getter:用于访问商店外部的这些状态对象。
- Mutations: Used to modify state object. 变异:用于修改状态对象。
- Actions: Used to commit mutations. 行动:用于实施突变。
Assuming that you are familiar with those 4 components, let’s see what are the principles you need to follow.
假设您熟悉这四个组件,那么让我们看看您需要遵循的原则。
- You need to keep an application-level state centralized in the store. 您需要将应用程序级状态集中存储在商店中。
- States should always be mutated by committing mutations. 应当始终通过进行突变来改变国家。
- Asynchronous logic should be encapsulated and they can only be used with actions. 异步逻辑应该被封装,并且只能与动作一起使用。
If you can follow these 3 principles, your projects can be structured smoothly and if you feel store files are getting larger, you have full freedom to split them into separate files. An example project structure will look like below:
如果您可以遵循这3条原则,那么您的项目就可以顺利构建,并且如果您觉得存储文件越来越大,则可以将它们拆分成单独的文件。 一个示例项目结构如下所示:
├── index.html
├── main.js
├── api
├── components
└── store
├── index.js
├── actions.js
├── mutations.js
└── modules
模块化VUEX商店 (Modularizing the VUEX Store)
We are talking about large scale projects in this article and we can expect project files to be very large and complex in such projects. You need to manage your store in your own way and need to avoid the store overwhelming. So, it is advised to modularize your Vuex store in a way that you can understand easily. There is no defined way to break modules in a project and some developers modularize according to features while some others modularize according to data models. The final decision about modularizing is totally up to you and this will help both you and your team in the long term.
我们在本文中讨论的是大型项目,并且可以预期此类项目中的项目文件非常大而复杂。 您需要以自己的方式管理商店,并且需要避免商店拥挤。 因此,建议您以易于理解的方式对您的Vuex商店进行模块化。 没有打破项目中模块的明确方法,有些开发人员根据功能进行模块化,而另一些开发人员则根据数据模型进行模块化。 关于模块化的最终决定完全取决于您,这将长期对您和您的团队都有帮助。
store/
├── index.js
└── modules/
├── module1.store.js
├── module2.store.js
├── module3.store.js
├── module4.store.js
└── module5.store.js
使用助手来简化您的代码 (Use helpers to simplify your code)
In the first part of the article, I mentioned about 4 components used in Vuex store. Let’s consider a situation where you need to access those states, getters or you need to call actions, mutations in your component. I such situations you don’t need to create multiple computed properties or methods, you can easily use the helper methods (mapState
, mapGetters
, mapMutations
and mapActions
) to reduce the code. Let’s see those 4 helpers in action:
在本文的第一部分中,我提到了Vuex存储中使用的4个组件。 让我们考虑一种情况,您需要访问这些状态,获取器,或者需要调用操作,组件中的变异。 在这种情况下,您无需创建多个计算属性或方法,则可以轻松使用帮助器方法( mapState
, mapGetters
, mapMutations
和mapActions
)来减少代码。 让我们来看一下这四个助手:
mapState
mapState
If we need to call multiple store state properties or getters within a component, we can use mapState
helper to generate a getter function and this will reduce the number of code lines significantly.
如果需要在一个组件中调用多个存储状态属性或getter,则可以使用mapState
helper生成一个getter函数,这将大大减少代码行的数量。
import { mapState } from 'vuex'export default {
computed: mapState({
count: state => state.count,
countAlias: 'count',
countPlusLocalState (state) {
return state.count + this.localCount
}
})
}
mapGetters
mapGetters
mapGetters
helper can be used to map store getters to local computed properties.
mapGetters
帮助程序可用于将商店的吸气剂映射到本地计算的属性。
import { mapGetters } from 'vuex'export default {
computed: {
...mapGetters([
'count1',
'getter1',
])
}
}
mapMutations
mapMutations
mapMutations
helper can be used to commit mutations in component and it will map the component methods to store.commit
calls. Also, we can use mapMutations
to pass payloads as well.
mapMutations
帮助程序可用于提交组件中的突变,它将映射组件方法到store.commit
调用。 同样,我们也可以使用mapMutations
传递有效载荷。
import { mapMutations } from 'vuex'export default {
methods: {
...mapMutations({
cal: 'calculate' // map `this.cal()` to `this.$store.commit('calculate')`
})
}
}
mapActions
mapActions
This helper is used to dispatch actions in component and it maps component methods to store.dispatch
calls.
此帮助程序用于在组件中分派动作,并将组件方法映射到store.dispatch
调用。
import { mapActions } from 'vuex'export default {
methods: {
...mapActions({
cal: 'calculate' // map `this.cal()` to `this.$store.dispatch('calculate')`
})
}
}
4.不要忘记编写单元测试 (4. Don’t forget to write Unit Tests)
Testing is another important aspect of any project. As developers, we must test what we develop despite the importance or the size of the project. Especially when it comes to large scale projects, there are hundreds and thousands of small functions, and it is our duty to test each of these functions. Unit Tests come into action on such scenarios and it allows developers to test individual code units. Unit tests, not only avoid bugs, but it also improves the confidence of the development team about their work, whenever they do a change. When the projects grow over time, developers can add new features without any fear of breaking another by following a good unit testing mechanism from the beginning.
测试是任何项目的另一个重要方面。 作为开发人员,无论项目的重要性或规模如何,我们都必须测试开发的内容。 尤其是涉及大型项目时,有成千上万的小型功能,因此我们有责任测试每个功能。 单元测试在这种情况下生效,它使开发人员可以测试单个代码单元。 单元测试不仅可以避免错误,而且每当他们进行更改时,它也可以提高开发团队对其工作的信心。 当项目随着时间增长时,开发人员可以从一开始就遵循良好的单元测试机制来添加新功能,而不必担心会破坏其他功能。
If we consider unit testing in Vue.js, It is almost similar to all other frameworks’ unit testing approaches and you can use Jest, Karma, or Mocha with Vue.js easily. Despite the test framework, there are few general things that you need to keep in mind when unit tests are written.
如果我们考虑在Vue.js中进行单元测试,则它几乎与所有其他框架的单元测试方法相似,并且您可以在Vue.js中轻松使用Jest , Karma或Mocha 。 尽管有测试框架,但是在编写单元测试时,您需要记住的一些一般事项。
- The test must provide clear error messages id it fails. 测试必须提供明确的错误消息ID(失败)。
- Use a good assertion library. (Eg: there are build in assertion libraries for jest framework, Chai library is used with Mocha) 使用一个好的断言库。 (例如:在Jest框架中内置了断言库,Chai库与Mocha一起使用)
- Write unit tests to cover each Vue component. 编写单元测试以涵盖每个Vue组件。
By following these steps from be beginning of the project, you can drastically reduce the time spent on debugging and manual testing as the project structure grows.
通过从项目开始就遵循这些步骤,随着项目结构的发展,您可以大大减少调试和手动测试所花费的时间。
Apart from Unit Testing, Vue.js supports both E2E testing and Integration testing as similar to any other framework. So it will be a good practice to combine those to your project as well. Usually routing part is not tested using unit tests and it can be covered with E2E testing. Vue store is the most difficult part to test and the recommended method for that is integration testing because separate testing for states, actions, or getters is considered useless.
除了单元测试之外,Vue.js与其他任何框架一样都支持E2E测试和集成测试。 因此,将这些也结合到您的项目中将是一个好习惯。 通常,路由部分不会使用单元测试进行测试,并且可以通过端到端测试进行覆盖。 Vue存储是最难测试的部分,而推荐的方法是集成测试,因为对状态,动作或吸气剂的单独测试被认为是无用的。
结论 (Conclusion)
After going through the above technical capabilities of Vue.js, I feel that Vue.js is ready for large scale projects as any other framework and we can easily manage those projects without creating a mess. You guys may have a different idea or different view about this too. So, please share your thoughts with others in the response section. Cheers…!!!
在经历了Vue.js的上述技术能力之后,我觉得Vue.js与其他任何框架一样都可以用于大规模项目,并且我们可以轻松地管理这些项目而不会造成混乱。 你们可能对此也有不同的想法或不同的看法。 因此,请在回复部分与他人分享您的想法。 干杯…!!!
翻译自: https://blog.bitsrc.io/4-best-practices-for-large-scale-vue-js-projects-9a533450bdb2
vue项目最佳实践