On Amazon Web Services the Serverless computational service par excellence remains Lambda, a must-have service when talking about this paradigm.
在Amazon Web Services上 ,无服务器计算服务仍是Lambda的佼佼者,Lambda是谈论此范例时必不可少的服务。
AWS Lambda allows you to use computational power without worrying about the management of the underlying servers, patches, software updates, or unexpected machine shutdowns. Using this paradigm, we can focus entirely on our application.
AWS Lambda允许您使用计算能力,而不必担心基础服务器的管理,补丁,软件更新或意外的计算机关闭。 使用这种范例,我们可以完全专注于我们的应用程序。
To date, AWS Lambda offers several runtime engines. Anyway, it is possible to create your own if you need to use a programming language not yet supported by AWS.
迄今为止,AWS Lambda提供了几种运行时引擎 。 无论如何, 如果您需要使用AWS尚不支持的编程语言 , 则可以创建自己的语言 。
The great aspect is: it’s up to us to choose the technology that best fits our needs when writing AWS Lambda Functions (FaaS): we can choose the programming language we are most familiar with, allowing us to reach our goals faster.
最重要的方面是:在编写AWS Lambda函数(FaaS)时,我们需要选择最适合我们需求的技术:我们可以选择我们最熟悉的编程语言,从而使我们更快地达到目标 。
In this article, we will cover how to develop a serverless backend quickly and effectively using TypeScript as a programming language.
在本文中,我们将介绍如何使用TypeScript作为编程语言来快速有效地开发无服务器后端 。
The application will leverage Express, a web application framework used for developing web services for Node.js. Node.js is the runtime environment — already supported by AWS — in which our TypeScript code, compiled in JavaScript, will run on, on Lambda.
该应用程序将利用Express,这是一个用于为Node.js开发Web服务的Web应用程序框架。 Node.js是AWS已支持的运行时环境,在该环境中,以JavaScript编译的TypeScript代码将在Lambda上运行。
After the Analysis, the proposed solution will be deployed on our AWS account. This project can be consulted and downloaded from GitHub!
分析之后,建议的解决方案将部署在我们的AWS账户上。 可以从GitHub咨询并下载该项目!
涉及的AWS服务 (AWS services involved)
Let’s start by proposing the architectural scheme of our Serverless application.
让我们从提出无服务器应用程序的体系结构方案开始。

The code will be released on an AWS Lambda function, whose invocation will only be possible through an API Gateway.
该代码将在AWS Lambda函数上发布,该函数只能通过API Gateway进行调用。
Obviously, a Data Source cannot be missing in a backend application. As we aim to take full advantage of the Serverless paradigm, Aurora Serverless will be involved as a relational database to save and retrieve our data.
显然,后端应用程序中不能缺少数据源。 当我们旨在充分利用无服务器范例时,Aurora Serverless将作为关系数据库参与其中,以保存和检索我们的数据。
In summary, we are going to use the following cloud services:
总而言之,我们将使用以下云服务:
- AWS Lambda AWS Lambda
- API Gateway API网关
- Aurora Serverless — Postgres Engine Aurora无服务器— Postgres引擎
- CloudFormation 云形成
深入基础架构管理:无服务器框架。 (Diving into infrastructure management: the Serverless framework.)
Nowadays, several tools are available for DevOps engineers to efficiently manage infrastructures, for example, the AWS CLI, the console, or one of the frameworks available online, such as Troposphere, Terraform, or AWS SAM.
如今,DevOps工程师可以使用多种工具来有效管理基础架构,例如,AWS CLI,控制台或在线可用的框架之一,例如Troposphere,Terraform或AWS SAM。
As explained above, our goal is to build a backend application quickly: that’s why we chose the Serverless framework.
如上所述,我们的目标是快速构建后端应用程序:这就是我们选择无服务器框架的原因。
Serverless is a framework written in Node.js allowing us to manage the lifecycle of our serverless applications. It supports several Cloud Providers and features.
Serverless是用Node.js编写的框架,可让我们管理无服务器应用程序的生命周期。 它支持多种云提供商和功能。
As for AWS, Serverless will allow us to create and manage the resources we need on our account using the Cloudformation stack.
对于AWS,Serverless将允许我们使用Cloudformation堆栈来创建和管理我们需要的帐户资源。
要求 (Requirements)
Before starting to work on the project, the following requirements need to be met:
在开始进行该项目之前,需要满足以下要求:
- AWS CLI correctly configured 正确配置了AWS CLI
- Have an Aurora Serverless (Postgres engine) on your AWS account 在您的AWS账户上安装Aurora Serverless(Postgres引擎)
Since we’re going to write an application in TypeScript, Node.js will be the runtime environment on which we’re going to run our code, compiled in JavaScript.
由于我们将使用TypeScript编写应用程序,因此Node.js将成为运行时环境,我们将在该运行时环境中运行以JavaScript编译的代码。
In order to deploy the Cloudformation stack on AWS, it is necessary to have the Serverless framework installed on the machine. To do so, run the npm install -g serverless command, and configure the AWS CLI credentials properly.
为了在AWS上部署Cloudformation堆栈,必须在计算机上安装无服务器框架。 为此,请运行npm install -g serverless命令,并正确配置AWS CLI凭证。
动手! (Hands-on!)
At this point we are ready to start: download the project from our GitHub repository and check its structure.
至此,我们准备开始:从GitHub存储库下载项目并检查其结构。
Let’s start by cloning the project:
让我们从克隆项目开始:
git clone https://github.com/besharpsrl/blog-serverless-backend-nodejs
git clone https://github.com/besharpsrl/blog-serverless-backend-nodejs
Enter the blog-serverless-backend-nodejs folder and run the npm install command to install all the necessary dependencies.
输入blog-serverless-backend-nodejs文件夹,然后运行npm install命令安装所有必需的依赖项。
Here is some clarification:
这里有一些澄清:
aws-sdk: Software Development Kit for using AWS services. In our case, I will use it to integrate with Aurora Serverless and to retrieve its login credentials from Secrets Manager.
aws-sdk :用于使用AWS服务的软件开发套件。 在我们的案例中,我将使用它与Aurora Serverless集成并从Secrets Manager中检索其登录凭据。
sequelize: widely used and supported ORM for Node.js.
sequelize :广泛用于Node.js的ORM。
serverless: Framework for the creation and management of the Serverless infrastructure.
serverless :用于创建和管理无服务器基础结构的框架。
express: Minimal and flexible web application framework for Node.js that offers a series of features for web application development.
express :用于Node.js的最小且灵活的Web应用程序框架,为Web应用程序开发提供了一系列功能。
tsoa: Framework used to write the controllers for the self-generation of Express routes. Thanks to it, it is also possible to generate OpenAPI specs valid for both version 2.0 and 3.0. It also offers automatic management for the validation of the input of our requests, without having to create and maintain boilerplate code.
tsoa :用于编写用于自动生成Express路由的控制器的框架。 多亏了它,还可以生成同时适用于2.0版和3.0版的OpenAPI规范。 它还提供了自动管理来验证我们的请求输入,而无需创建和维护样板代码。
You will find all these (and other) utility dependencies in the package.json file. In addition, it contains commands necessary for running and testing our APIs locally, as well as for releasing them on the AWS account.
您将在package.json文件中找到所有这些(以及其他)实用程序依赖项。 此外,它包含在本地运行和测试我们的API以及在AWS账户上发布它们所必需的命令。
By opening the project on your IDE, you will notice a scaffolding similar to the following:
通过在IDE上打开项目,您会发现类似于以下内容的脚手架:

Let’s dive deep into the application logic.
让我们深入研究应用程序逻辑。
We are going to describe a very simple use case: simple REST APIs offering CRUD functions to manage books in a library.
我们将描述一个非常简单的用例: 简单的REST API,提供了CRUD功能来管理图书馆中的书籍。
The logic resides under the book directory. Here is defined the tsoa Controller, the Service carrying both the business logic and the sequelize models.
逻辑位于book目录下。 此处定义了tsoa控制器,该服务同时包含业务逻辑和序列化模型。
In the app.ts file, instead, we will record the Express routes (autogenerated by tsoa starting from the Controllers), and then export a module that will be used as a handler for our Lambda function.
相反,在app.ts文件中,我们将记录Express路由(由tsoa从Controllers开始自动生成),然后导出一个模块,该模块将用作Lambda函数的处理程序 。
Everything is made possible through the statement:
通过以下语句,一切皆有可能:
module.exports.handler = sls(app)
module.exports.handler = sls(app)
Through this command, we are invoking a Serverless framework function able to create a wrapper around the Express routes. In this way, the handler of our AWS Lambda function will then know which Express route to route the REST API calls to.
通过此命令,我们正在调用一个无服务器框架功能,该功能可以围绕Express路由创建包装器。 这样,我们的AWS Lambda函数的处理程序将知道将REST API调用路由到哪个Express路由。
Finally, we just have to analyze the serverless.yml file:
最后,我们只需要分析serverless.yml文件 :
service: express-serverless
provider:
name: aws
runtime: nodejs12.x
region: eu-west-1
stage: ${env:NODE_ENV}
environment:
NODE_ENV: ${env:NODE_ENV}
iamRoleStatements:
- Effect: 'Allow'
Action:
- 'secretsmanager:GetSecretValue'
Resource:
- '*'package:
exclude:
- node_modules/**
- defer" defer" defer" defer" defer" defer" defer" defer" defer" src/**layers:
nodeModules:
path: layer
compatibleRuntimes:
- nodejs12.x…..functions:
app:
handler: dist/app.handler
layers:
- {Ref: NodeModulesLambdaLayer}
events:
- http:
path: /api
method: ANY
cors: true
- http:
path: /api/{proxy+}
method: ANY
cors: true
vpc:
securityGroupIds:
- {Ref: LambdaSecurityGroup}
subnetIds:
- "subnet-1"
- "subnet-2"
- "subnet-3"plugins:
- serverless-offline
Note that in this file all the essential parts of the infrastructure are defined, such as the Cloud Provider on which we are going to deploy our Lambda function, its handler (the module we exported to the app.ts file) and the Lambda Layers to attach the function to.
请注意,在此文件中,定义了基础架构的所有必要部分,例如我们要在其上部署Lambda函数的Cloud Provider,其处理程序(我们导出到app.ts文件的模块)以及将Lambda层附加功能。
With this configuration, in fact, we will create a Lambda Layer containing all the node modules. By doing so, we considerably reduce the size of our function, obtaining performance advantages during its execution.
实际上,使用此配置,我们将创建一个包含所有节点模块的Lambda层。 通过这样做,我们大大减小了函数的大小,在执行过程中获得了性能优势。
Let’s go ahead: how is the Lambda function invoked? Through the http events defined in the Serverless file! This allows the creation of an API Gateway with which it will be possible to call our function.
让我们继续:Lambda函数如何调用? 通过Serverless文件中定义的http事件! 这允许创建一个API网关,通过它可以调用我们的函数。
The resource with path /api/{proxy+} will be the one that will proxy the backend routes. We have thus created a single resource on the API Gateway side that allows us to invoke all the REST APIs.
路径为/ api / {proxy +}的资源将是代理后端路由的资源。 因此,我们在API网关端创建了一个资源,该资源使我们可以调用所有REST API。
本地测试 (Local testing)
Not everyone knows that it is possible to test APIs without necessarily having to release them on AWS Lambda every time you make changes in the source code. That’s the great advantage of the Node.js package called serverless-offline!
并非所有人都知道,无需在每次更改源代码时都必须在AWS Lambda上发布API,就可以测试API。 那就是称为serverless-offline的Node.js包的巨大优势!
It is a Serverless plugin that emulates AWS Lambda and API Gateway on your machine allowing you to speed up development activities.
它是一个无服务器插件,可在您的计算机上模拟AWS Lambda和API网关,从而可以加快开发活动。
Before you can use it, however, you will need to locally create a Postgres database and run the Sequelize migrations:
但是,在使用它之前,您将需要在本地创建一个Postgres数据库并运行Sequelize迁移:
From the root of the project, we execute the command
从项目的根目录开始,执行命令
docker-compose up -d
And then:
然后:
npm run migrate-db-local
At this point, we can test APIs. Run the npm run start command to compile our TypeScript code in JavaScript and emulate the Lambda via the serverless-offline plugin.
至此,我们可以测试API。 运行npm run start命令,以JavaScript编译我们的TypeScript代码,并通过无服务器离线插件模拟Lambda。
As soon as the command is executed, you should see the following output on the terminal:
执行命令后,您应该在终端上看到以下输出:

At this point, we are ready to test the API with the tool we are most familiar with (Postman, Curl). For example, using the curl command we can execute the command from the terminal:
至此,我们已经准备好使用我们最熟悉的工具(Postman,Curl)来测试API。 例如,使用curl命令,我们可以从终端执行命令:
curl http://localhost:3000/dev/api/book/
卷曲 http:// localhost:3000 / dev / api / book /
部署 (Deploy)
Go ahead and take the last step: the application deploy.
继续进行最后一步:部署应用程序。
Running the npm run deploy-dev command will start the release process. The first time the following output will appear:
运行npm run deploy-dev命令将启动发布过程。 第一次出现以下输出:
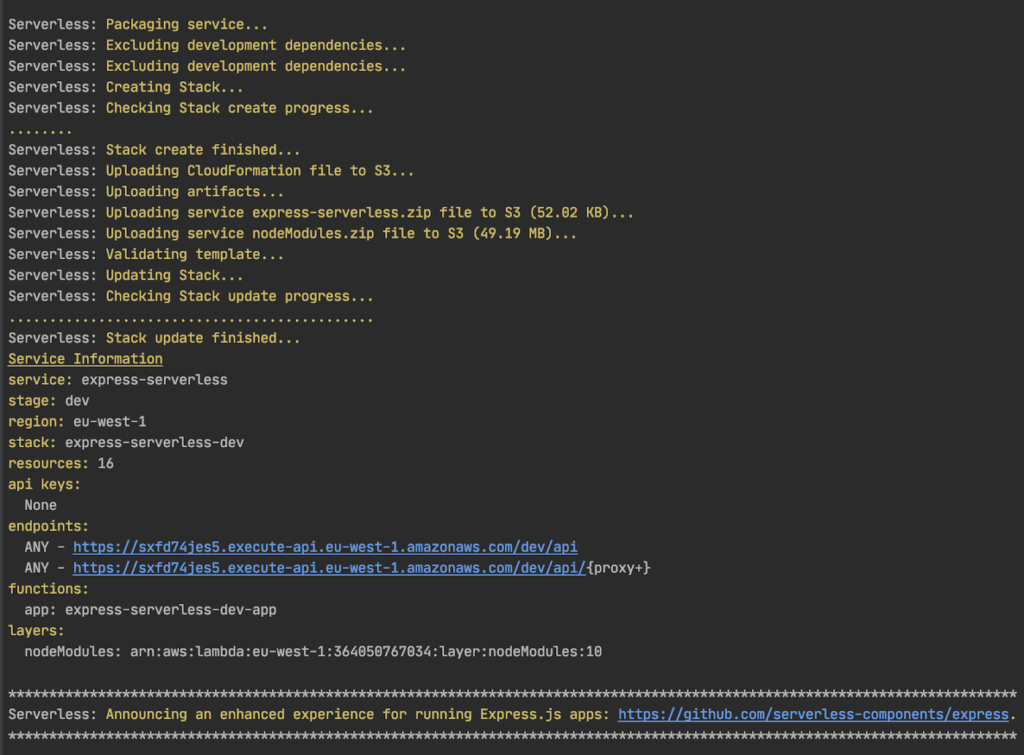
On the AWS account, a Cloudformation stack containing the resources we need has been generated.
在AWS账户上,已生成包含我们所需资源的Cloudformation堆栈。
By accessing the console, we will find the just-created Lambda function among the list of Lambda functions available
通过访问控制台,我们将在可用的Lambda函数列表中找到刚创建的Lambda函数。

Before testing the APIs it is necessary to run the Sequelize migrations to create tables on the Aurora Serverless database. Be sure you are able to connect to the database on AWS. To do this, it will be necessary to create a bastion machine on our AWS account and divert traffic from ours to the bastion. Use the sshuttle command:
在测试API之前,必须运行Sequelize迁移以在Aurora Serverless数据库上创建表。 确保您能够连接到AWS上的数据库。 为此,有必要在我们的AWS账户上创建一个堡垒机,并将流量从我们的流量转移到堡垒。 使用sshuttle命令:
sshuttle --dns -r ubuntu@EC2_BASTION_IP YOUR_VPC_CIDR --ssh-cmd 'ssh -i YOUR_PEM_KEY'
At this point, launch the migration by running the following command:
此时,通过运行以下命令启动迁移:
npm run migrate-dev
Once the migrations on Aurora Serverless are completed, testing the APIs through API Gateway is the only thing left to do.
完成Aurora Serverless上的迁移后,剩下要做的就是通过API网关测试API。
The API Gateway endpoint has already been printed in the output of the npm run deploy-dev command. Let’s try it:
API Gateway端点已经打印在npm run deploy-dev命令的输出中。 让我们尝试一下:
curl https://sxfd74jes5.execute-api.eu-west-1.amazonaws.com/dev/api/book/
卷曲 https://sxfd74jes5.execute-api.eu-west-1.amazonaws.com/dev/api/book/
The release process of our Serverless infrastructure on the AWS account is completed!
我们在AWS账户上的无服务器基础架构的发布过程已完成!
结论 (Conclusion)
To conclude, in this article we discussed how to create a Serverless application on AWS Lambda using Node.js as the runtime engine.
总而言之,在本文中,我们讨论了如何使用Node.js作为运行时引擎在AWS Lambda上创建无服务器应用程序。
We decided to write the proposed project with TypeScript to have the advantage of using a transpiled, widely supported, and known language.
我们决定使用TypeScript编写拟议的项目,以具有使用经过翻译,广泛支持且已知的语言的优势。
The choice of using Node.js as a runtime engine allows for a very limited Cold Start time as JavaScript is directly interpreted by the underline engine. Moreover, thanks to the configuration used, the source code is separated from the dependencies — saved on Lambda Layer, instead — drastically reducing the size of our source and improving startup performance.
使用Node.js作为运行时引擎的选择允许非常有限的冷启动时间,因为下划线引擎直接解释了JavaScript。 此外,由于使用了配置,因此源代码与依赖项分开了,而是保存在Lambda层上,从而极大地减少了源代码的大小并提高了启动性能。
Happy with this solution? :)
对这个解决方案满意吗? :)
See you in 15 days on #Proud2beCloud for a new article!
15天后在# Proud2beCloud上与您见面 !