react入门
Start learning the most employable library right now.
立即开始学习最受雇的图书馆。
React is one of the most popular JavaScript frameworks on the market, and shows no sign of slowing down any time soon. React is where the most developers, jobs, and opportunities exist. That’s not to say other frameworks & libraries are useless, but if you’re serious about your career, you should stick with React.
React是市场上最受欢迎JavaScript框架之一,并且没有任何迹象表明很快会放缓。 React是存在最多开发人员,工作和机会的地方。 这并不是说其他框架和库都没有用,但是如果您对自己的职业很认真,则应该坚持使用React。
React is a fairly complicated JavaScript framework, and I’d strongly recommend starting by learning HTML & JavaScript. That will make your life a lot easier.
React是一个相当复杂JavaScript框架,我强烈建议从学习HTML和JavaScript开始。 这将使您的生活更加轻松。
为什么React如此受欢迎? (Why is React so popular?)
React has the popularity it does because some of the brightest minds in programming built & maintain it (hint: Facebook engineers). Even if you don’t like Facebook for privacy reasons, they employ some of the greatest minds in the industry.
React之所以受欢迎,是因为在编程方面一些最聪明的人构建并维护了它(提示:Facebook工程师)。 即使您出于隐私原因不喜欢Facebook,他们也会雇用业内一些最有才华的人。
React is also dynamic & highly reusable. If you write one solid React component (we’ll cover what that is in a minute) you’re going to be able to reuse that code easily on your future projects.
React也是动态且高度可重用的。 如果您编写一个可靠的React组件(我们将在一分钟之内介绍完),您将能够在未来的项目中轻松地重用该代码。
准备一个React项目 (Getting a React Project Set Up)
Creating a functional React boilerplate template to start working with is pretty easy. Make sure you download Node.js. This will take a minute. So grab a cup of coffee. Then, just one simple command typed into the command line will get your boilerplate template ready.
创建功能性的样板模板开始使用非常简单。 确保下载Node.js。 这需要一分钟。 因此,抢一杯咖啡。 然后,只需在命令行中键入一个简单命令即可准备好样板模板。
npx create-react-app myreactname
Let’s break down what this means. ‘npx’ is a tool that allows you to use packages. The package in this case is ‘create-react-app’. And finally, ‘myreactname’ is the name of your project. You can change this to anything you want. The ‘create-react-app’ is super useful and comes with a lot of packages to save you time.
让我们解释一下这意味着什么。 'npx'是一个允许您使用软件包的工具。 在这种情况下,程序包是“ create-react-app”。 最后,“ myreactname”是您的项目的名称。 您可以将其更改为所需的任何内容。 “ create-react-app”非常有用,并且附带许多软件包,可以节省您的时间。
React模板的3个主要部分 (3 Main Parts of the React Template)
There are 3 major parts that come inside of ‘create-react-app’.
“ create-react-app”内部包含3个主要部分。
Each of these are very important, and we can break them down further. Let’s start with Babel.
这些都非常重要,我们可以进一步细分它们。 让我们从Babel开始。
Babel is a tool that makes different versions of JavaScript compatible with any browser. Babel allows old JavaScript code to be usable on modern browsers.
Babel是一种使不同版本JavaScript与任何浏览器兼容的工具。 Babel允许旧JavaScript代码在现代浏览器上可用。
Think of each different version of JavaScript as another language. With each new update, the JavaScript community goes from writing code in French to Chinese. And the next update turns JavaScript into English. Babel translates these different languages so the code can still be understood. This tool is as powerful as it sounds. (No wonder it gets downloaded 16+ million times a week since 2019).
将每个不同版本JavaScript视为另一种语言。 每次更新时,JavaScript社区都会从用法语编写代码变为用中文编写代码。 接下来的更新将JavaScript变成了英语。 Babel会翻译这些不同的语言,因此仍然可以理解代码。 这个工具听起来很强大。 (难怪自2019年以来,它每周被下载16+百万次)。
Next, there is Webpack. It bundles modules for JavaScript applications. Instead of having countless modules with their own dependencies, you can use just a few static assets.
接下来是Webpack 。 它捆绑了JavaScript应用程序的模块。 您可以只使用一些静态资产,而不必让无数个模块具有自己的依赖性。
And finally, there is Dev Server which is technically part of Webpack. This allows you to see & test your project locally in your browser.
最后, Dev Server从技术上讲是Webpack的一部分。 这使您可以在浏览器中本地查看和测试项目。
We can dissect many other packages & technologies within React, but those are the most fundamental to understand. You can get the big picture with just these.
我们可以在React中剖析许多其他包和技术,但是这些是最基本的了解。 有了这些,您就可以了解全局。
什么是组件? (What is a Component?)
A component is a function (or a class) that produces HTML content by using JSX. It also handles feedback from the user. Components play a big role in React applications.
组件是使用JSX生成HTML内容的函数(或类)。 它还处理来自用户的反馈。 组件在React应用程序中起着重要作用。
Components are great. They can be reused. Endlessly. If you write one solid component it can be used in every single React application you write for the rest of your career. They also manage their own state which can easily become part of a complex UI (user interface).
组件很棒。 它们可以重复使用。 不休。 如果您编写一个固体组件,那么在您余下的职业生涯中,它都可以在您编写的每个React应用程序中使用。 他们还管理自己的状态,这些状态很容易成为复杂UI(用户界面)的一部分。
Make sure to export your component so you can use it in your project! This is a common beginner mistake. One line at the bottom of your component file is all you need. It should look something like this.
确保导出组件,以便可以在项目中使用它! 这是一个常见的初学者错误。 您只需在组件文件底部的一行即可。 它看起来应该像这样。
export default App;
什么是App.js (What is App.js)
App.js is a file that will house your components. It is an example of a building block for you web application. You will import this (or any other component) into your main file which will be index.js.
App.js是一个文件,将容纳您的组件。 它是您的Web应用程序构建块的示例。 您将把这个(或任何其他组件)导入到您的主文件中,该文件将是index.js。
It is important to note that this component (App.js or any other component for that matter) is simply a JavaScript function or class. These components are the building blocks you will use to build your web application!
请务必注意,此组件(App.js或与此相关的任何其他组件)只是一个JavaScript函数或类。 这些组件是您用来构建Web应用程序的基础!
The React standard is to keep each individual component inside its own JavaScript file. And the App.js file will be the parent component of all your other components.
React标准是将每个单独的组件保留在其自己JavaScript文件中。 并且App.js文件将是您所有其他组件的父组件。
创建事物的4个步骤 (4 Steps to Create Something)
1.导入React (1. Import React)
This can be done by typing the following at the top of your JavaScript file. Note, if you’re using the boilerplate template this will be inside the index.js file. This will automatically come with your React boilerplate template when you start a project.
这可以通过在JavaScript文件顶部键入以下内容来完成。 请注意,如果您使用样板模板,则该模板将位于index.js文件中。 启动项目时,这将自动随您的React样板模板一起提供。
Import React from ‘react’
This will ensure that your file is making use of everything that comes with the ‘create-react-app’ boilerplate template.
这将确保您的文件充分利用“ create-react-app”样板模板随附的所有内容。
2.创建一个组件 (2. Create a component)
This can be done pretty easily with some simple code. Let’s write a small component here with some JSX (more on that soon). This code can go right into your index.js file. Feel free to copy & paste into your text editor if you’re following along.
使用一些简单的代码就可以很容易地做到这一点。 让我们在这里编写一些JSX的小组件(稍后再介绍)。 此代码可以直接进入您的index.js文件。 如果您要继续,请随时将其复制并粘贴到文本编辑器中。
const App = () => {return (<div><label>Enter your name: </label><input type=”text” /><button style={{backgroundColor: ‘red’, color: ‘black’}}> Submit </button></div>);};
Quick side note, this is simply a JavaScript function. You don’t have to write ‘function’ before the parentheses if you include the ‘=>’ before the curly braces.
快速的旁注,这只是一个JavaScript函数。 如果在花括号前加上“ =>”,则不必在括号前写“ function”。
3.显示您的React组件 (3. Display your React Component)
You can display your React component by passing it to ReactDOM.render(). Make sure your component is styled in JSX. It will resemble an HTML item but it will be your React component. It should look something like the following code.
您可以通过将其传递到ReactDOM.render()来显示React组件。 确保您的组件在JSX中设置了样式。 它类似于一个HTML项目,但它将是您的React组件。 它看起来应该类似于以下代码。
ReactDOM.render( <div> <MyApp /> <div>; )
4.运行开发服务器 (4. Run the Dev Server)
Open your command prompt and change your directory to your project file. Then type the following command into the command line. This can take a second but your React application should open up on your default browser.
打开命令提示符,然后将目录更改为项目文件。 然后在命令行中输入以下命令。 这可能需要一秒钟,但是您的React应用程序应该在默认浏览器上打开。
npm start
Now, your React application should be displaying in your browser locally. It should look something like this.
现在,您的React应用程序应该在本地浏览器中显示。 它看起来应该像这样。
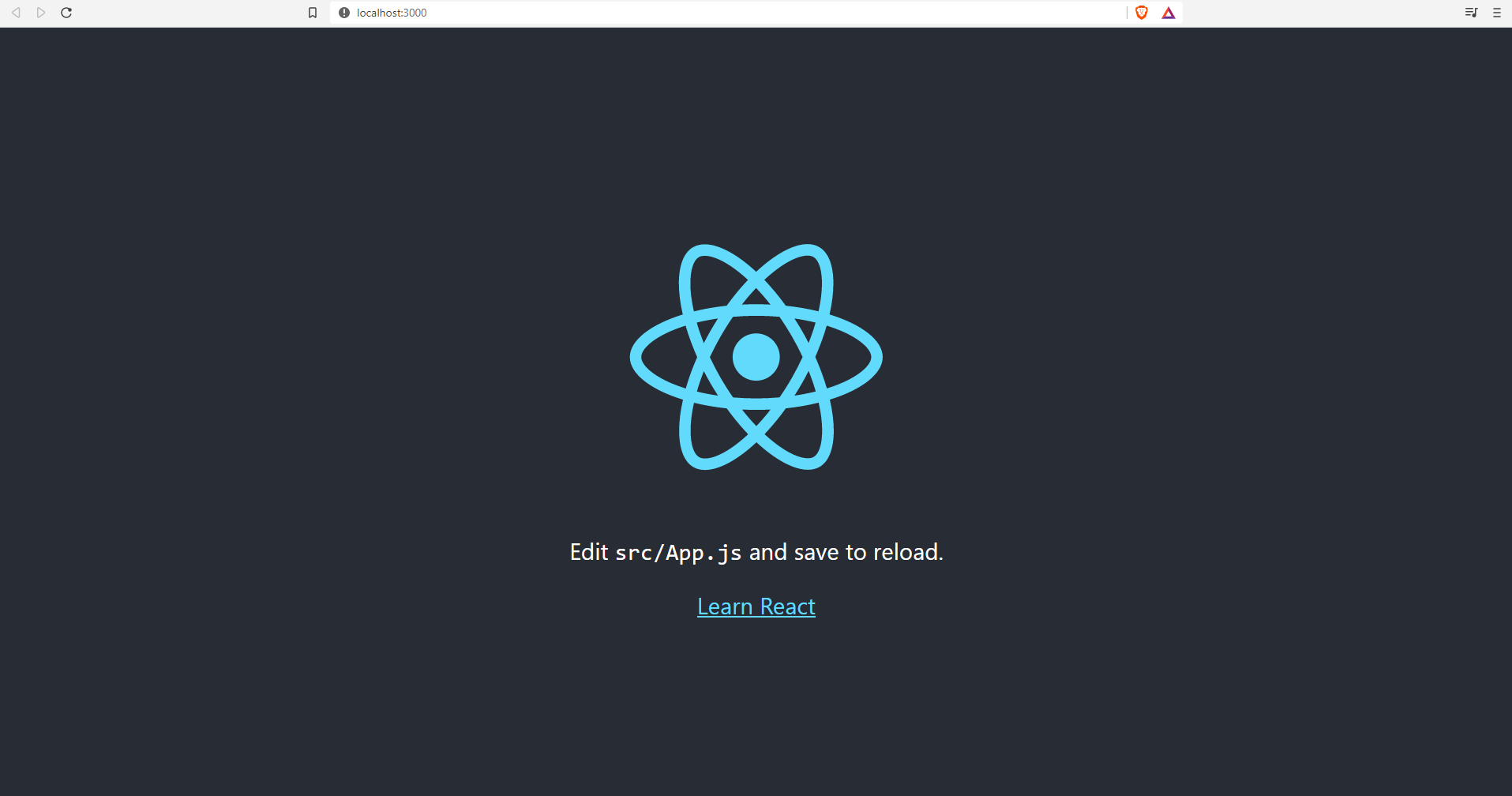
React vs ReactDOM (React vs ReactDOM)
Another important distinction to understand is React and ReactDOM. These are two parts of the puzzle that perform different operations to make sure things work.
要理解的另一个重要区别是React和ReactDOM。 这是拼图的两个部分,执行不同的操作以确保事情正常进行。
React is a reconciler that works with components.
React 是与组件一起使用的协调器。
ReactDOM is a renderer that turns JSX into HTML for the browser.
ReactDOM 是将JSX转换为浏览器HTML的渲染器。
模板文件 (Template Files)
Let’s discuss some of the default folders & files that come along with the React boilerplate template.
让我们讨论一下React样板模板随附的一些默认文件夹和文件。
src folder is for storing all the source code of the project (where your code will go)
src文件夹用于存储项目的所有源代码(您的代码将到达的位置)
public folder will hold all the static files associated with your project (.png, .jpeg, etc)
公用文件夹将与您的项目(png格式,JPEG格式等)相关联的所有静态文件
node_modules is a folder for all the project dependencies. You won’t have to mess around with this too much.
node_modules是所有项目依赖项的文件夹。 您不必太在意这个。
package.json records the project’s dependencies & configures the project itself.
package.json记录项目的依赖关系并配置项目本身。
package-lock.json records the exact version of the installed packages. This is important to stop packages from being updated & becoming incompatible.
package-lock.json记录已安装软件包的确切版本。 这对于阻止软件包更新和变得不兼容很重要。
Readme.md is for instructions on how to use the project. You should always write good documentation with your projects and this is where all that will go.
Readme.md是有关如何使用该项目的说明。 您应该始终为您的项目编写好的文档,这就是所有要做的事情。
JSX与HTML (JSX vs HTML)
JSX is JavaScript React code that looks a lot like HTML. Babel translates it into HTML to be displayed on the page. But it isn’t exactly like HTML. There are a few important differences to note. I’m going to assume you’re familiar with HTML & JavaScript, and if you aren’t, you should be starting there.
JSX是看起来很像HTMLJavaScript React代码。 Babel将其转换为HTML以显示在页面上。 但这并不完全像HTML。 有一些重要的区别要注意。 我假设您熟悉HTML和JavaScript,如果不熟悉,则应该从那里开始。
render() {
return (
<div className="styling"><h1>Welcome to my React web application! </h1>
<MyReactApp /></div>);}
There is different styling syntax for specific elements (class becomes className in JSX)
特定元素有不同的样式语法( 类在JSX中变为className )
- JSX can use JavaScript variables and HTML cannot JSX可以使用JavaScript变量,而HTML不能
Speaking of JSX, we should also discuss best practice. To indicate a JavaScript string, you should use normal quotation marks. You should use single quotes to refer to a non-JSX property. It will work either way, but it’s important to make your code as easily understood as possible. Let’s take a look at an example.
说到JSX,我们还应该讨论最佳实践。 要指示JavaScript字符串,应使用普通的引号。 您应该使用单引号来引用非JSX属性。 无论哪种方式都可以工作,但是使您的代码尽可能容易理解很重要。 让我们看一个例子。
const App = () => {return (<div><label className="label" for="name">Enter your name: </label><input type='text' /><button style={{backgroundColor: 'blue', color: 'white'}}> Submit </button></div>);};
Notice the className label is surrounded by double quotes. That’s because it is JSX code. Compare this to the button style. The colors blue and white are both using single quotes because they are not JSX, but CSS properties.
注意className 标签用双引号引起来。 那是因为它是JSX代码。 将此与按钮样式进行比较。 蓝色和白色都使用单引号,因为它们不是JSX,而是CSS属性。
I’m sure you noticed the CSS styling on the button style is surrounded by double curly braces. This is how you would change styling for a single element like this in React. It is oftentimes easier than creating an entire CSS document for a single element like this.
我确定您注意到按钮样式上CSS样式被双花括号包围。 这就是您如何在React中更改单个元素的样式的方法。 通常比这样为单个元素创建整个CSS文档容易。
I mentioned you can use JavaScript variables inside JSX. But there’s a very specific way to do so. You need to use curly braces{} inside the JSX code. Let’s look at an example.
我提到您可以在JSX中使用JavaScript变量。 但是有一种非常具体的方法。 您需要在JSX代码内使用花括号{}。 让我们来看一个例子。
const buttonText = "click here!"render() {
return <div> {buttonText} </div>;
}
The curly braces let React know you are trying to reference a JavaScript variable inside of the JSX code.
花括号让React知道您正在尝试引用JSX代码内部JavaScript变量。
结论 (Conclusion)
React js is a wonderful framework. It will take some work to get the hang of it, but this was hopefully a good place to start. Many developers encourage students to jump right into creating projects. But I think understanding some of the background & theory behind a framework makes learning much easier.
React js是一个很棒的框架。 要花很多时间才能完成工作,但是希望这是一个不错的起点。 许多开发人员鼓励学生立即着手创建项目。 但是我认为理解框架背后的一些背景和理论会使学习变得容易得多。
翻译自: https://medium.com/swlh/getting-started-with-react-2da1df9113a6
react入门