react 跨站脚本
什么是React? (What is React?)
React is a JavaScript library for building user interfaces. React was developed by Facebook and Instagram. React deals with View in MVC (Model — View — Controller). React is declarative, rather than imperative. Declarative code (such as HTML) is a programming paradigm that expresses what to do without describing the how to.
React是一个用于构建用户界面JavaScript库。 React是由Facebook和Instagram开发的。 React处理MVC中的View (模型-视图-控制器)。 React是声明性的,而不是命令性的。 声明代码(如HTML)是一种编程范式,表达什么而不用描述如何 去做 。
为什么要React? (Why React?)
React give you tools for interacting with the DOM that are much cleaner and effective than with raw JavaScript or jQuery. The HTML, CSS, JavaScript can be held in one component, they are like “super powered” HTML tags. It is fast and supports code modularity and reuse.
React为您提供了与DOM进行交互的工具,比与原始JavaScript或jQuery进行交互的工具更为有效。 HTML,CSS,JavaScript可以包含在一个组件中,就像“超级强大”HTML标签一样。 它速度很快,并支持代码模块化和重用。
React概念 (React Concepts)
There are many react concepts that all serve importance. To keep this post, concise and sweet we will discuss the basic foundational ones listed.
有许多React概念都很重要。 为了使这篇文章简洁明了,我们将讨论列出的基本基础知识。
- Components 组件
- JSX JSX
- Rendering Elements 渲染元素
- Function vs Class Components and Props 功能与类组件和道具
- State and Handling Events 状态和处理事件
There are many more main concepts with additional depth. This post attempts to summarize some main concepts at a high level.
还有更多的其他主要概念。 这篇文章试图概括一些主要概念。
React是基于组件的 (React is Component-Based)
Components are the building blocks to React written application. Imagine your UI (a web page application), this can be split into independent, reusable pieces. Consider each piece in isolation. Each piece is a component as shown below.
组件是React书面应用程序的构建块。 想象一下您的UI(一个网页应用程序),它可以分为独立的,可重复使用的部分。 独立考虑每个部分。 每一块都是一个组成部分 如下所示。
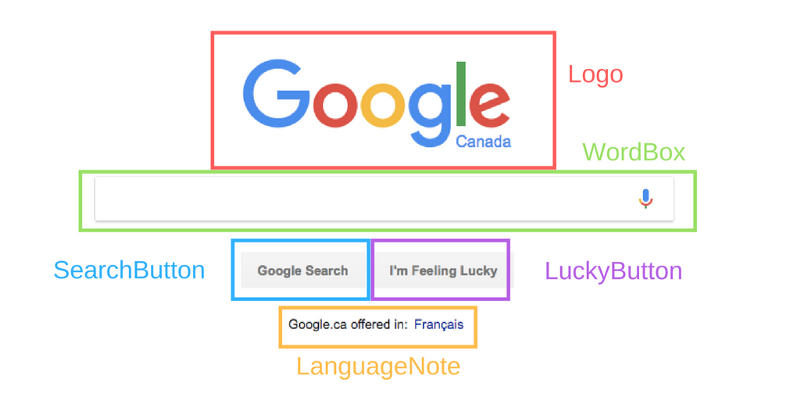
Each component is a JavaScript function that returns (renders) React elements to the screen (DOM).
每个组件都是一个JavaScript函数,可将React元素返回(渲染)到屏幕(DOM)。
React需要JSX吗? (Does React need JSX ?)
JSX is a syntax extension to JavaScript. React uses this language to describe what the UI should look like. It looks like HTML but with the full power of JavaScript. See the example syntax.
JSX是JavaScript的语法扩展。 React使用这种语言来描述UI的外观。 它看起来像HTML,但具有JavaScript的全部功能。 请参见示例语法。
const element = <h1>Hello, world!</h1>;
JSX is not required for using React. However, JSX makes it very convenient. See the difference.
使用React不需要JSX。 但是,JSX使其非常方便。 看到不同。
Code that does NOT use JSX:
不使用JSX的代码:
class Hello extends React.Component {
render() {
return React.createElement('div', null, `Hello ${this.props.toWhat}`);
}
}
ReactDOM.render(
React.createElement(Hello, {toWhat: 'World'}, null),
document.getElementById('root')
);
Code written with JSX:
用JSX编写的代码:
class Hello extends React.Component {
render() {
return <div>Hello {this.props.toWhat}</div>;
}
}
ReactDOM.render(
<Hello toWhat="World" />,
document.getElementById('root')
);
Try more snippets in Babel’s online tool to appreciate JSX ES6 syntax.
在Babel的在线工具中尝试更多片段,以欣赏JSX ES6语法。
Any valid JavaScript expression are placed inside curly braces in JSX. By default, React DOM escapes any values embedded in JSX before rendering. This prevents XSS attacks.
任何有效JavaScript表达式都放在JSX中的花括号内。 默认情况下,React DOM在呈现之前转义JSX中嵌入的任何值。 这样可以防止XSS攻击。
渲染元素 (Rendering Elements)
An element describes what is seen on the screen. React elements are plain objects where the React DOM uses to update the actual DOM. Elements are what components are made of.
元素描述屏幕上看到的内容。 React元素是React DOM用于更新实际DOM的普通对象。 元素是组成组件的元素。
In the HTML file, identify where you want to place the react element or component. For example, in the HTML file there is:
在HTML文件中,标识要放置react元素或组件的位置。 例如,在HTML文件中有:
<div id="app"></div>
Everything inside this <div>
will be managed by the React DOM. An application built with just React have a single root DOM node. If React is integrated into an existing app, there may be varying root DOM nodes.
<div>
所有内容都将由React DOM管理。 仅使用React构建的应用程序具有单个根DOM节点。 如果将React集成到现有应用程序中,则可能会有不同的根DOM节点。
To render a React element or component, use ReactDOM.render():
要渲染React元素或组件,请使用ReactDOM.render() :
ReactDOM.render(element, placeWeWantToPutElementOrComponent)// example
const element = <h1>Hello, world</h1>;
ReactDOM.render(element, document.getElementById('app'));
React only updates what is necessary. The React DOM compares the element and its children to the previous, and only applies the necessary DOM updates.
React只更新必要的内容。 React DOM将元素及其子元素与之前的元素进行比较,并且仅应用必要的DOM更新。
功能与类组件和道具 (Functional vs Class Components and Props)
We will discuss state in the following section. The main difference between functional and class components are:
我们将在下一节中讨论状态。 功能和类组件之间的主要区别是:
- Functional Components are Stateless (simply accepts data and display them to UI) 功能组件是无状态的(仅接受数据并将其显示到UI)
- Class Components are Stateful (more complex by implementing logic and state) 类组件是有状态的(通过实现逻辑和状态来变得更复杂)
Let’s write our first component as functional. Below is a simple functional component in ES6 syntax showing how we can put React components within another.
让我们将第一个组件编写为功能组件。 以下是ES6语法中的一个简单功能组件,展示了如何将React组件放在另一个组件中。
Note: Always start component names with a capital letter. React treats components with lowercase letters as DOM tags <div />
and <Container />
represent a component.
注意:组件名称始终以大写字母开头。 React将具有小写字母的组件视为DOM标签<div />
和<Container />
表示组件。
Components can accept arbitrary inputs (called “props”, which stands for properties) and returns a React element. This is a way to pass data into components, making them more dynamic and reusable. Props are immutable and cannot be changed once passed in from a parent.
组件可以接受任意输入(称为“ props”,代表属性)并返回React元素。 这是一种将数据传递到组件中的方法,使它们更具动态性和可重用性。 道具是不可变的,一旦从父母那里传递过来就无法更改。
const Component = () => (
<h1>I am a child component!</h1>
);const Container = (props) => (
<div>
<h1>I am parent {props.name} component!</h1>
<Component />
</div>
);ReactDOM.render(<Container name="Container" />, document.getElementById('app'));
We can re-write the Container component into a class component with Java Script's ES6 classes to make things more dynamic.
我们可以使用Java Script的ES6类将Container组件重新编写为类组件,以使事情更加动态。
class Container extends React.Component {
render() {
return (
<div>
<h1>
I am the parent
</h1>
<Component />
</div>
);
}
}const Component = () => (
<h1>I am a child component!</h1>
);ReactDOM.render(<Container name="Container" />, document.getElementById('app'));
The example above:
上面的例子:

ReactDOM.render()
renders the<Container name="Container" />
to the DOM. The container component has{name="Container"}
as it’s props.ReactDOM.render()
将<Container name="Container" />
呈现给DOM。 容器组件具有{name="Container"}
作为道具。The
<Container />
component returns a<div >
with the<h1 >
and the inner<Component />
<Container />
组件返回带有<h1 >
和内部<Component />
的<div >
<Component />
- React DOM updates the DOM to match this React DOM更新DOM以使其与此匹配
Class components must have a function called render()
. Both <Container />
components (functional and class) above are equivalent from React’s point of view. You should use functional components over class components unless you need component “state” discussed in a later section. State lets React components change their output in response to user actions, network responses, and anything else.
类组件必须具有一个称为render()
的函数。 从React的观点来看,以上两个<Container />
组件(功能和类)都是等效的。 除非需要后面一节中讨论的组件“ 状态 ”,否则应在类组件上使用功能组件。 状态可让React组件根据用户操作,网络响应以及其他任何情况更改其输出。
州 (State)
To make applications interactive, our components need to do more than receive props. Class components can have information about their current situation which is called state stored in a Java Script object. State can be used to update and re-render data changes. State is only available on class components. The key word this
inside the component refers to the component it’s within.
为了使应用程序具有交互性,我们的组件需要做的不仅仅是接收道具。 类组件可以具有有关其当前状态的信息,该信息称为状态,存储在Java Script对象中。 状态可用于更新和重新呈现数据更改。 状态仅在类组件上可用。 关键的词this
在组件内部是指它的内部组件。
The simple example below has a ToDoList
component with it’s current state as NOT being done (set to false).
下面的简单示例包含一个ToDoList
组件,其当前状态为未完成(设置为false)。
class ToDoList extends React.Component {
constructor(props) {
super(props);
this.state = {
done: false,
};
} render() {
const status = this.state.done ? 'Good Work' : 'YOU HAVE WORK';
return (
<div>
<h1>
{status}
</h1>
</div>
);
}
}ReactDOM.render(<Container name="Container" />, document.getElementById('app'));
Once state is initialized, the way to update state is with setSate()
. Do not modify state directly. The only place to assign this.state
is the constructor.
一旦状态被初始化,更新状态的方法就是使用setSate()
。 不要直接修改状态。 分配this.state
的唯一位置是构造函数。
Let’s interact with our component to let it know we have completed our ToDoList
and display the correct status. Let’s say there is a button we can click to inform this change.
让我们与组件进行交互,以使其知道我们已经完成了ToDoList
并显示正确的状态。 假设有一个按钮可以单击以通知此更改。
事件处理 (Event Handling)
Handing events with React elements is similar to handling events on DOM elements. There are some differences.
汉鼎事件有React的元素相似,在处理DOM元素的事件。 有一些区别。
- React events use camelCase, not lowercase React事件使用camelCase,而不是小写
- Pass a function within curly braces in JSX as the event handler, not a string 在JSX中的花括号内传递一个函数作为事件处理程序,而不是一个字符串
Cannot return false to prevent default behavior, must
preventDefault()
explicitly无法返回false以防止出现默认行为,必须明确地阻止Default
preventDefault()
Reference SyntheticEvents reference defined by React
参考React定义的SyntheticEvent参考
Be careful with
this
in JSX callback. Class methods are not bound by default. Remember to bindthis.eventHandleMethod.bind(this)
or with ES6 arrow function before passing intoonClick
.在JSX回调中
this
要小心。 默认情况下,类方法未绑定。 在传递给onClick
之前,请记住绑定this.eventHandleMethod.bind(this)
或使用ES6箭头函数。
Let’s add a way the user can interact with the component by clicking a button. When they click the button, let’s update the state done to be true with setSate()
, which therefore updates the status.
让我们添加一种用户可以通过单击按钮与组件进行交互的方式。 当他们单击按钮时,让我们使用setSate()
将状态更新为true,从而更新状态。
class ToDoList extends React.Component {
constructor(props) {
super(props);
this.state = {
done: false,
};
} handleClick(event) {
this.setState({ done: true });
} else {
this.setState({ done: false });
}
}; render() {
const status = this.state.done ? 'Good Work' : 'YOU HAVE WORK';
return (
<div>
<h1>
{status}
</h1>
<button onClick={
Done
</button>
</div>
);
}
}ReactDOM.render(<Container name="Container" />, document.getElementById('app'));
Now when the user clicks this Done button, the ToDoList
component updates the new state, which therefore renders a new status to display.
现在,当用户单击此“完成”按钮时, ToDoList
组件将更新新状态,因此将呈现新状态以供显示。
There is so much more we can do with nested components talking between each other using their props and their events. Props don’t have to be just information, they can be functions as well. We can also pass arguments to event handlers. There are other resources out there that will show these simple examples.
我们可以做很多事情,嵌套组件使用它们的道具和事件彼此对话。 道具并不仅仅是信息,它们也可以是功能。 我们还可以将参数传递给事件处理程序。 还有其他资源将显示这些简单的示例 。
I hope this provides a simple overview of some basic concepts in React. These concepts have more depth and there are other powerful concepts I have not discussed. React’s website was my resource and has amazing tutorial’s and guides to further discover.
我希望这提供了React中一些基本概念的简单概述。 这些概念更加深入,还有其他一些我尚未讨论的强大概念。 React的网站是我的资源,并且有很棒的教程和指南供您进一步发现。
I have completed my 6th week of Hack Reactor and we built a YouTube video player with React. Using interactions with YouTube’s data API. We are onto Redux/React next. Hope this guide is useful for someone out there!
我已经完成了第6周的Hack Reactor,我们用React构建了一个YouTube视频播放器。 与YouTube的数据API进行交互。 接下来是Redux / React。 希望本指南对那里的人有用!
翻译自: https://medium.com/dev-genius/java-script-lets-talk-react-basics-322629bb6e62
react 跨站脚本