javascript 属性
HTML5 gave us the ability to add arbitrary pieces of information called data attributes to an element to use behind the scenes for any purpose you wish, and here I will demonstrate how to use them in JavaScript.
HTML5使我们能够向元素添加称为数据属性的任意信息,以在幕后用于您希望的任何目的,在这里,我将演示如何在JavaScript中使用它们。
I put data attributes to good use in my Interactive Periodic Table. There is plenty of information around on the topic but often with simplistic or contrived examples so here I will present a real-world usage with a simplified version of the Periodic Table.
我在交互式元素周期表中充分利用了数据属性。 关于该主题的信息很多,但通常带有简化或虚构的示例,因此在这里,我将通过简化版的周期表来介绍实际用法。
You can add a custom data attribute to an element by hard-coding it into the HTML, prefixing the name with data-
, for example:
您可以通过以下方式将自定义数据属性添加到元素,方法是将其硬编码到HTML中,并在名称前加上data-
,例如:
<div id=”div1" data-manufacturer=”nikon” data-model=”d6">Nikon D6</div>
<div id=”div1" data-manufacturer=”nikon” data-model=”d6">Nikon D6</div>
In JavaScript you can access an element’s data attributes for reading and writing using its dataset
property which is of DOMStringMap
type and is basically a collection of key/value pairs. The keys are the names used for the attributes but without the data-
prefix, so for the above example we would use manufacturer
and model
, as shown below.
在JavaScript中,您可以使用DOMStringMap
类型的元素数据dataset
属性访问元素的数据属性以进行读写,该属性基本上是键/值对的集合。 键是用于属性的名称,但没有data-
前缀,因此对于以上示例,我们将使用manufacturer
和model
,如下所示。
const element = document.getElementById(“div1”);console.log(element.dataset.manufacturer); // nikon
console.log(element.dataset.model); // d6element.dataset.manufacturer = “canon”;
element.dataset.model = “EOS 90D”;
It is also easy to find elements with certain data attribute values using querySelector
, for example:
使用querySelector
查找具有某些数据属性值的元素也很容易,例如:
const element = document.querySelector(“[data-manufacturer=’nikon’][data-model=’d6']”);
const element = document.querySelector(“[data-manufacturer='nikon'][data-model='d6']”);
Below is a screenshot of the simplified Periodic Table used for this example.
以下是此示例使用的简化周期表的屏幕截图。
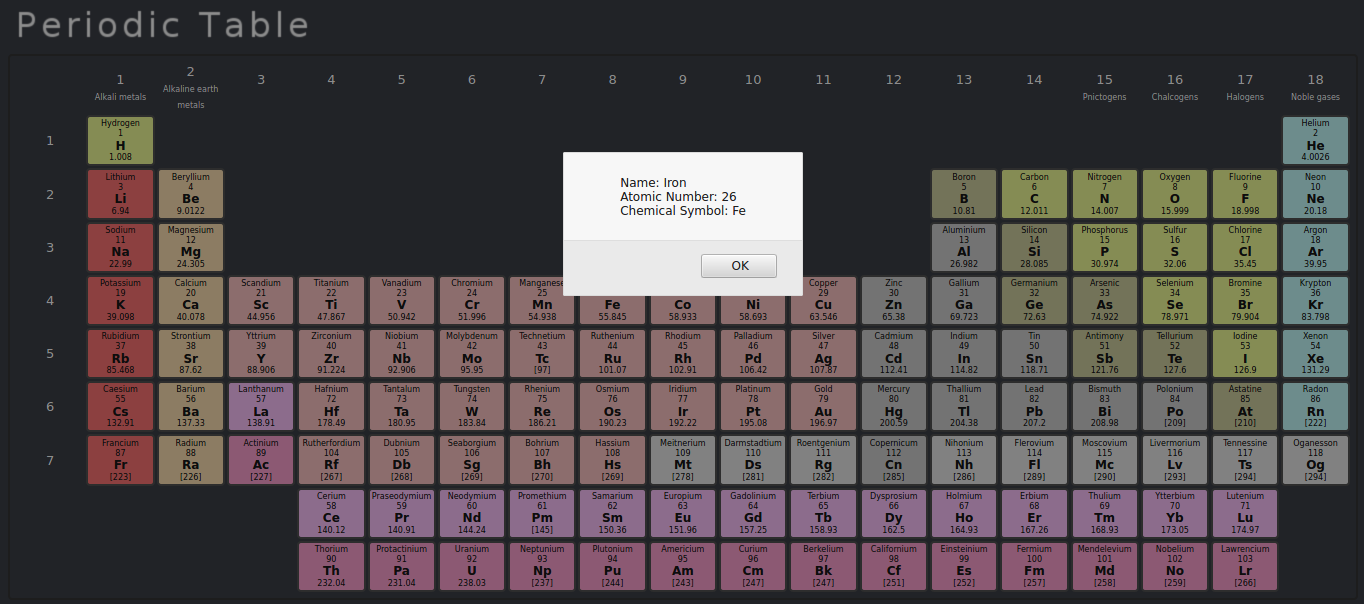
If you click on an element an alert is shown with the name, atomic number and chemical symbol of that element. You have probably guessed how we know which element* to show the details for: using its data attribute.
如果单击某个元素,则会显示一个警报,其中包含该元素的名称,原子序号和化学符号。 您可能已经猜到我们如何知道使用哪个元素*显示其数据的详细信息:使用其data属性。
I am going to have to use the word “element” quite a lot during this article. This is pretty annoying but I will try to point out whether I am referring to an HTML element or a type of atom unless it is obvious.
在本文中,我将不得不大量使用“元素”一词。 这很烦人,但除非显而易见,否则我将尝试指出是指HTML元素还是原子类型。
该项目 (The Project)
This project consists of an HTML file, a JavaScript file and a few subsiduary files, all of which are in the Github repository.
该项目包含一个HTML文件,一个JavaScript文件和一些辅助文件,所有这些文件都位于Github存储库中 。
I won’t show all the source code, just the bits relevant to data attributes. I have used them several times during this project but to avoid repetition I will just show where we carry out the following tasks:
我不会显示所有源代码,而只显示与数据属性相关的位。 在这个项目中,我已经多次使用它们,但是为了避免重复,我将仅说明我们执行以下任务的位置:
Setting the
data-atomicnumber
for a (chemical) element on its corresponding (HTML) element在相应的(HTML)元素上设置(化学)元素的
data-atomicnumber
- Finding an (HTML) element by its (chemical) element’s atomic number to set the background colour 通过(化学)元素的原子序数查找(HTML)元素以设置背景色
Retrieving the
data-atomicnumber
from a clicked (HTML) element to show the (chemical) element’s details从单击的(HTML)元素中检索
data-atomicnumber
以显示(化学)元素的详细信息
To populate the table we iterate a list of elements, adding each to the table with a corresponding data-atomicnumber
attribute.
为了填充表,我们迭代了一个元素列表,并使用相应的data-atomicnumber
属性将每个元素添加到表中。
currentcell.setAttribute(‘data-atomicnumber’, element.atomicnumber);
currentcell.setAttribute('data-atomicnumber', element.atomicnumber);
Here I have used setAttribute
instead of dataset.atomicnumber
just to show the alternative.
在这里,我只是使用setAttribute
而不是dataset.atomicnumber
来显示替代方案。
After the table is populated we need to set the colours to indicate the element’s categories. Again we iterate the data, finding the cell corresponding to each element like this:
填充表格后,我们需要设置颜色以指示元素的类别。 再次,我们迭代数据,找到与每个元素相对应的单元格,如下所示:
const currentcell = document.querySelector(`[data-atomicnumber=’${element.atomicnumber}’]`);
const currentcell = document.querySelector(`[data-atomicnumber='${element.atomicnumber}']`);
As seen in the Nikon D6 example above you can use more than one selector if necessary. In this project we know that the data is static so we will always find a cell but if this is not the case remember to check the return value of querySelector
for null. Also, you might need to use querySelectorAll
if there can be more than one matching element.
如上述尼康D6示例所示,如有必要,可以使用多个选择器。 在这个项目中,我们知道数据是静态的,因此我们将始终找到一个单元格,但是如果不是这种情况,请记住检查querySelector
的返回值是否为null。 另外,如果可以有多个匹配元素,则可能需要使用querySelectorAll
。
Finally we need to pick up the data-atomicnumber
attribute value when an element is clicked.
最后,我们需要在单击元素时获取data-atomicnumber
属性值。
const element = this._periodictable.GetElement(target.dataset.atomicnumber);
const element = this._periodictable.GetElement(target.dataset.atomicnumber);
What is actually happening here is that we are calling the GetElement
method of an instance of a class representing all chemical elements, picking up the dataset.atomicnumber
of the target (ie the HTML element that was clicked) to use as the method argument.
实际发生的是,我们正在调用代表所有化学元素的类的实例的GetElement
方法,获取目标(即单击HTML元素)的dataset.atomicnumber
用作方法参数。
So as you can see using data attributes greatly simplifies the process of both finding an HTML element when we need to do something to it (such as setting its colour) and identifying that element when a user interacts with it, for example by clicking on it.
如您所见,使用数据属性极大地简化了以下过程:既需要我们做某事(例如设置其颜色)时查找HTML元素,又可以在用户与其交互时(例如,通过单击它)识别该元素。
When I started working on the Periodic Table it soon became clear that data attributes were the way to go. There are no doubt other ways to accomplish the same thing but I am not sure what they are as I never gave the matter any thought!
当我开始处理元素周期表时,很快就清楚了数据属性是必经之路。 毫无疑问,其他方法可以完成同一件事,但是我不确定它们是什么,因为我从未想过此事!
javascript 属性