构建react项目
JavaScript is one of the most popular programming language for front end developer, and it is part of the fundamentals to learning a tech stack. But just learning JavaScript is not enough, most of the time when programmers work on a project, we need to use some kind of frameworks to back up the vanilla JavaScript. React is one of the most popular JavaScript frameworks along with Angular and Vue. That being said, each framework has its own strengths and weaknesses. React being a smaller library and easier to start with as a beginner, while Angular is a bigger framework with lots of codes written in TypeScript. Let us dive deeper into React and what tips that I have learned while building projects with react.
JavaScript是前端开发人员最流行的编程语言之一,并且是学习技术堆栈的基础知识的一部分。 但是仅仅学习JavaScript是不够的,大多数时候程序员在进行项目工作时,我们需要使用某种框架来备份原始JavaScript。 React是Angular和Vue最受欢迎JavaScript框架之一。 话虽如此,每个框架都有自己的优点和缺点。 React是一个较小的库,较容易入门,而Angular是一个较大的框架,其中包含许多用TypeScript编写的代码。 让我们更深入地了解React以及我在使用React构建项目时学到的技巧。
First thing first, to start building a new react project, the most convenient way to do so is with terminal command npx create-react-app
followed by the project name. React is developed by Facebook, and it is very simple to use and comes with very detailed instructions and documentations, with create-react-app, react gives us a lot of structured files and setups that we can mitigate and start building our project asap. As we can see from the snippet below, react has built out the DOM render for our project along with some starter logo image and test files. Notice on the top where it says node_modules folder and it is grayed out, node modules is the folder with all the libraries for React and that folder will not be uploaded to GitHub since it is a very large folder with hundreds of files, which will consume a lot of time when it comes to uploading and saving the files to GitHub. (Check out my other blog about Git & GitHub tutorial). On that note, create-react-app gave us a file called .gitignore, as the name applies, it ignores the files when using git commands. (node_modules is one of the folders/files that will be ignored when it is time to upload the files to GitHub).
首先,要开始构建一个新的react项目,最方便的方法是使用终端命令npx create-react-app
后接项目名称。 React是由Facebook开发的,使用起来非常简单,并带有非常详细的说明和文档以及create-react-app,react为我们提供了许多结构化文件和设置,我们可以缓解这些问题和设置并尽快开始构建我们的项目。 从下面的代码片段中可以看到,react为我们的项目构建了DOM渲染以及一些入门徽标图像和测试文件。 注意顶部显示的是node_modules文件夹,它显示为灰色,node modules是包含所有React库的文件夹,该文件夹不会上传到GitHub,因为它是一个非常大的文件夹,包含数百个文件,将占用大量文件在将文件上传和保存到GitHub上花费了大量时间。 (查看有关Git和GitHub教程的其他博客)。 关于这一点,create-react-app给了我们一个名为.gitignore的文件,顾名思义,当使用git命令时,它将忽略这些文件。 (node_modules是将文件上载到GitHub时将被忽略的文件夹/文件之一)。
After creating our new project, the next thing that we need to do is to run it, npm start
command will do the trick. As we probably will notice, a webpage with localhost:3000
will open and the react logo image will be spinning around to show that our project has been successfully started. Yes, it looks super cool, but it is not what we are building. Therefore, we need to tweak and modify some files that create-react-app
gave us.
创建新项目后,下一步需要运行它, npm start
命令可以解决问题。 我们可能会注意到,将打开一个包含localhost:3000
的网页,并且React徽标图像将旋转,以表明我们的项目已成功启动。 是的,它看起来很棒,但这不是我们要构建的。 因此,我们需要调整和修改create-react-app
提供给我们的一些文件。
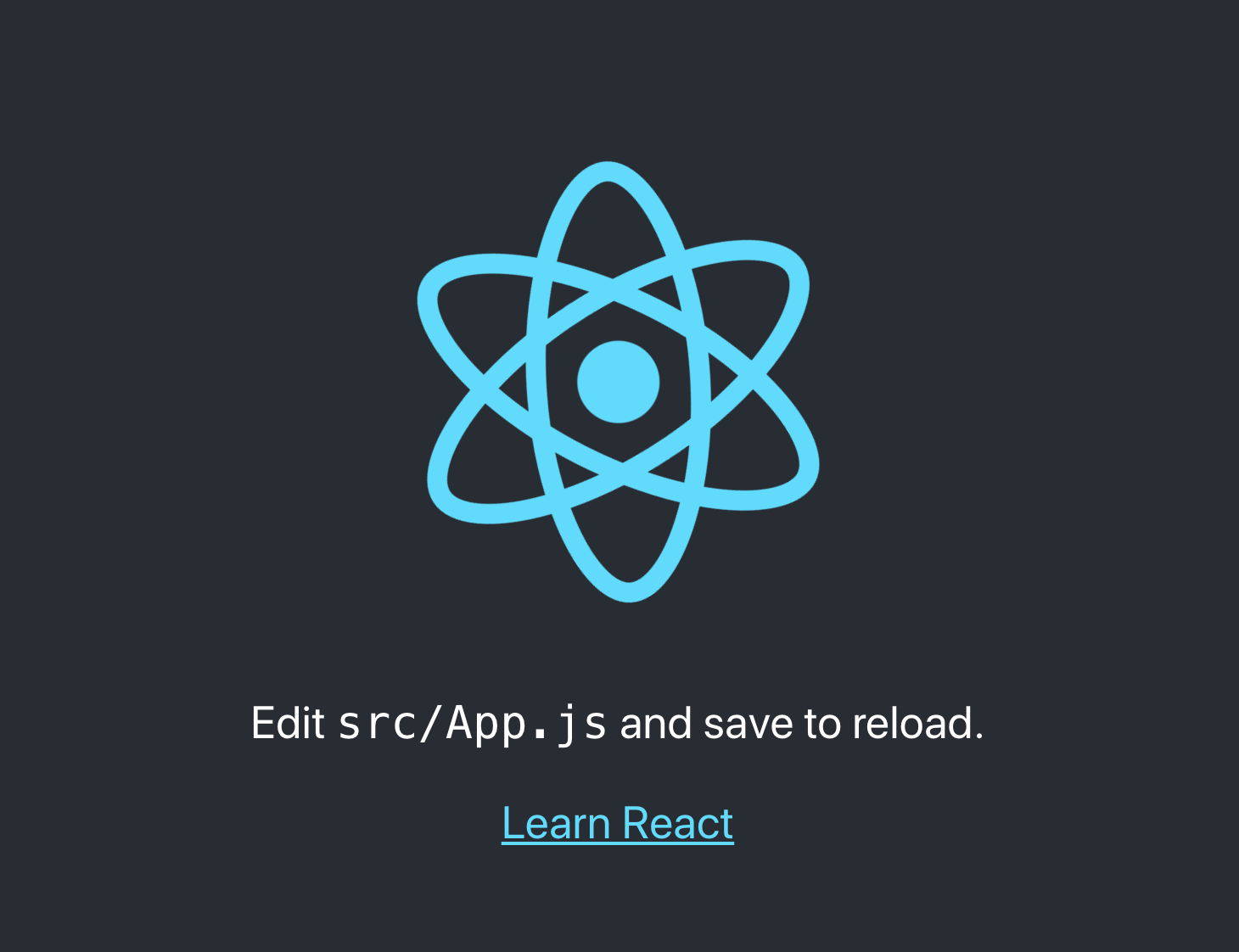
First of all, for a starter project, we simply don’t need app.test.js and setupTests.js which is a unit testing files to help us test our codes. Though the react logo looks cool but we don’t necessary need it to build our project, therefore deleting the logos and editing the app.js where the logo image is being called. Cleaning up the codes in app.css is also advised since React has given us some default CSS styling which we might not want to use.
首先,对于一个入门项目,我们根本不需要app.test.js和setupTests.js(这是一个单元测试文件)来帮助我们测试代码。 尽管react徽标看起来很酷,但是我们不需要构建我们的项目,因此删除徽标并编辑调用徽标图像的app.js。 还建议清理app.css中的代码,因为React给了我们一些我们可能不想使用的默认CSS样式。
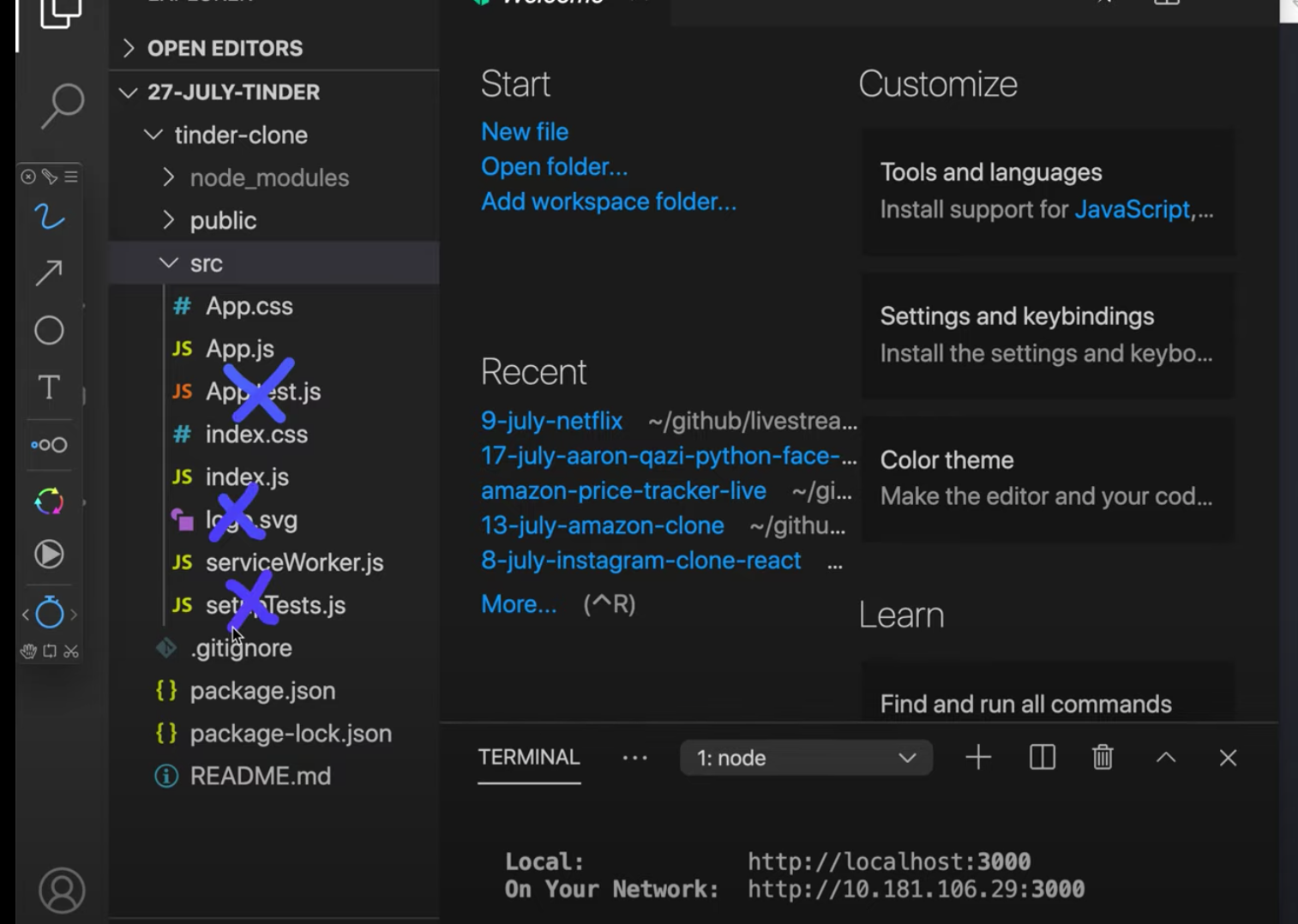
After cleaning up the starter codes, we are ready to begin building our project! In my recent learning and following the tutorial guide of Clever Programmer, I have learned so much about React and what are some of the best practices to use React. For example, file structure can be an issue when it comes to building a project. At the start, we might simply put all the files in the src folder, which can be managed at first, but as the project grows bigger, it is not efficient to file management if we jam all the files in src folder. A better management of files system can be using Component and Container based folders to separate and track all the files. Another popular way to manage files is based on components, whichever related components and its CSS files will go under a folder, which can be tracked easily throughout the project development phase.
清理了启动程序代码后,我们准备开始构建我们的项目! 在最近的学习中,并遵循Clever Programmer的教程指南,我学到了很多关于React以及使用React的最佳实践的知识。 例如,在构建项目时,文件结构可能是一个问题。 首先,我们可能只是将所有文件放在src文件夹中,这可以首先进行管理,但是随着项目的扩大,如果我们将所有文件都塞在src文件夹中,则文件管理效率不高。 更好的文件系统管理可以使用基于组件和容器的文件夹来分隔和跟踪所有文件。 另一个流行的文件管理方式是基于组件,任何相关的组件及其CSS文件都将放在一个文件夹下,该文件夹可在整个项目开发阶段轻松跟踪。
On the note of CSS file, we usually like to have each individual CSS file for every component, unless there are two features that can share and use the same styling, otherwise we usually want to separate its styling to its own CSS file, this way it can be easily tracked and modified when we need to. If we have all the styling in app.css file, which can work, however it will cause some confusion and make the file so large when there are different stylings of different features, and when we want to modify some styling of one feature, we have to go through all the mess in app.css to find out which styling we want to modify.
关于CSS文件,通常我们希望每个组件都有一个单独CSS文件,除非有两个可以共享和使用相同样式的功能,否则我们通常希望通过这种方式将其样式分离到自己CSS文件中我们可以根据需要轻松地对其进行跟踪和修改。 如果我们在app.css文件中拥有所有样式,并且可以使用,但是,如果存在不同样式的不同样式,并且当我们想要修改一个样式的样式时,它将引起一些混乱并使文件变得很大。必须遍历app.css中的所有混乱,以找出我们想要修改的样式。
In my recent learning to build a tinder-clone application, I have realized there are different ways of setting up routers in react. Router basically allows us to have routes to different pages based on what buttons we click. To start with Routers we need to install react-router with the command npm install react-router-dom
once the package is installed, we can start our routing by setting it up in index.js file, as shown in the snippet below.
在我最近学习的构建tinder-clone应用程序的过程中,我意识到有多种方法可以在react中设置路由器。 路由器基本上使我们能够根据单击的按钮来路由到不同的页面。 要开始使用路由器,我们需要在安装软件包后使用npm install react-router-dom
命令npm install react-router-dom
,我们可以通过在index.js文件中进行设置来启动路由,如下面的代码片段所示。
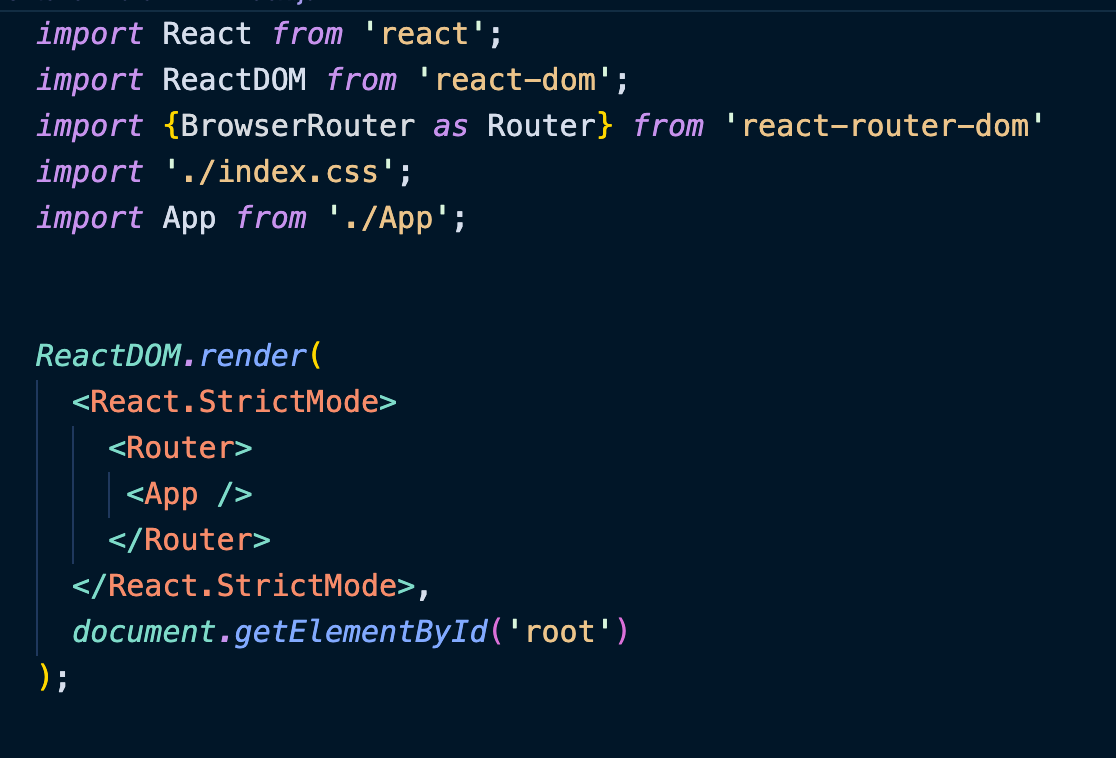
What I have learned recently is that instead of putting the router in index.js file, we can also set up the router within app.js file, which is more direct and easier to understand. In the snippet shown below, we have an example of importing BrowserRouter and setting up the router on app.js, switching the path of the route from ‘/’ which is the main page, to the route ‘/chat’ which is a chatting page for our app. One neat trick I like to use is on the line <Route exact path='/'>
I used the word exact path which only allows the app to go to main page when it is not specified with any routes. In this case, if we type ‘/chat’, it will bring us to the chat page, and only if we don’t specify what route it is, then it will be directed to the main page.
我最近了解到的是,除了将路由器放在index.js文件中之外,我们还可以在app.js文件中设置路由器,这更加直接和易于理解。 在下面显示的代码段中,我们有一个导入BrowserRouter并在app.js上设置路由器的示例,将路由的路径从主页的“ /”切换为聊天的路由“ / chat”我们的应用程序页面。 我想使用的一个巧妙技巧是在<Route exact path='/'>
我使用了精确路径一词,该词仅在未使用任何路由指定时允许应用程序转到主页。 在这种情况下,如果我们键入“ / chat”,它将带我们进入聊天页面,并且只有当我们不指定它的路由时,它才会被定向到主页。
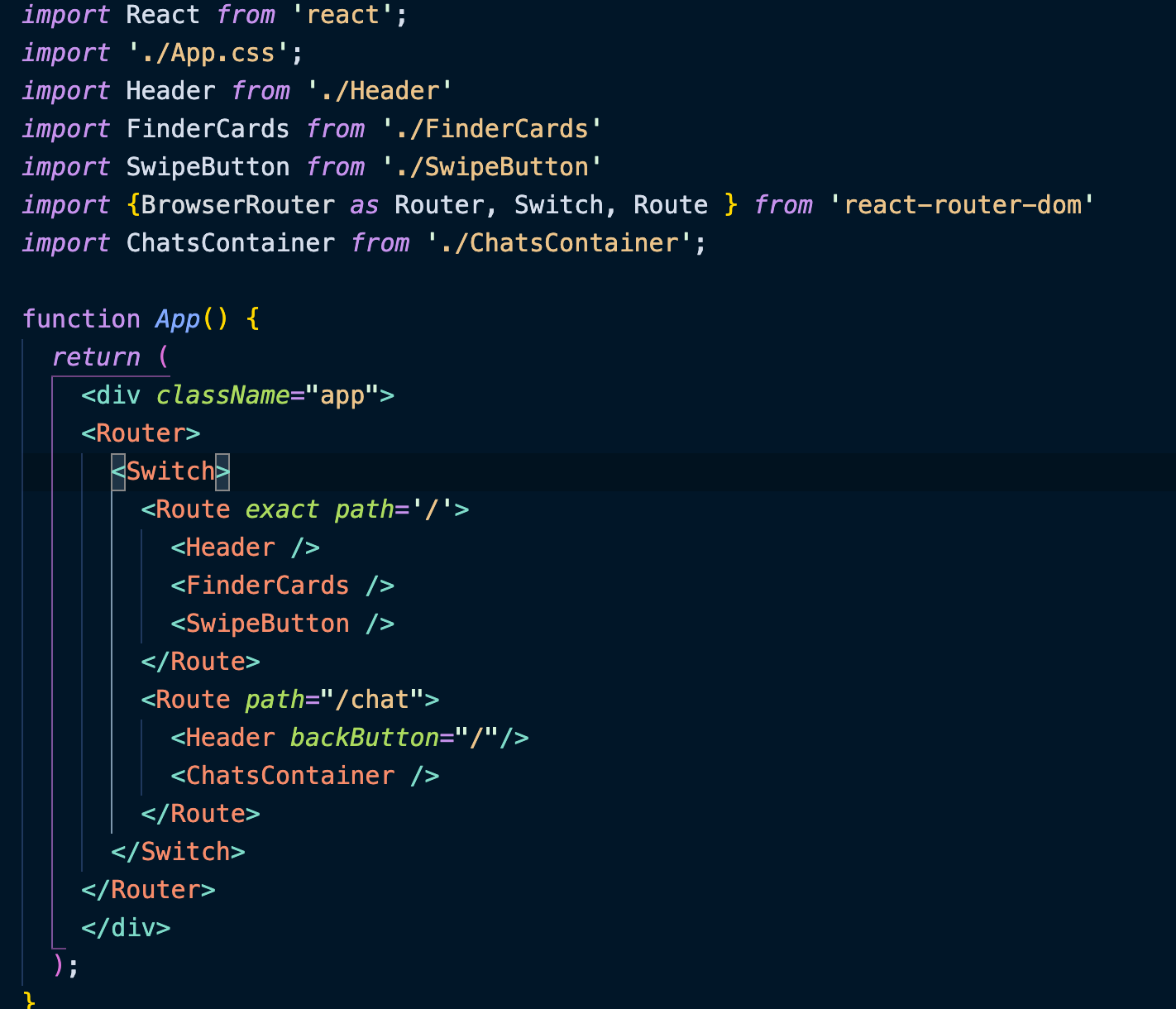
Another cool feature with react-router-dom is that we can use link with it. Let’s say we have a logo image for our app, and we want to make sure that when we click on the logo, it will bring us to the main page of our app. We can gladly do so with react-router-dom, shown in the code snippet below. import link from react-router-dom, followed by using <Link to our main page> attaching it with the logo image for our app.
react-router-dom的另一个很酷的功能是我们可以使用链接。 假设我们有一个应用程序的徽标图像,并且我们希望确保在单击徽标时将其带到应用程序的主页。 我们可以很高兴地用react-router-dom做到这一点,如下面的代码片段所示。 从react-router-dom导入链接,然后使用<链接到我们的主页>将其附加到我们应用的徽标图像中。
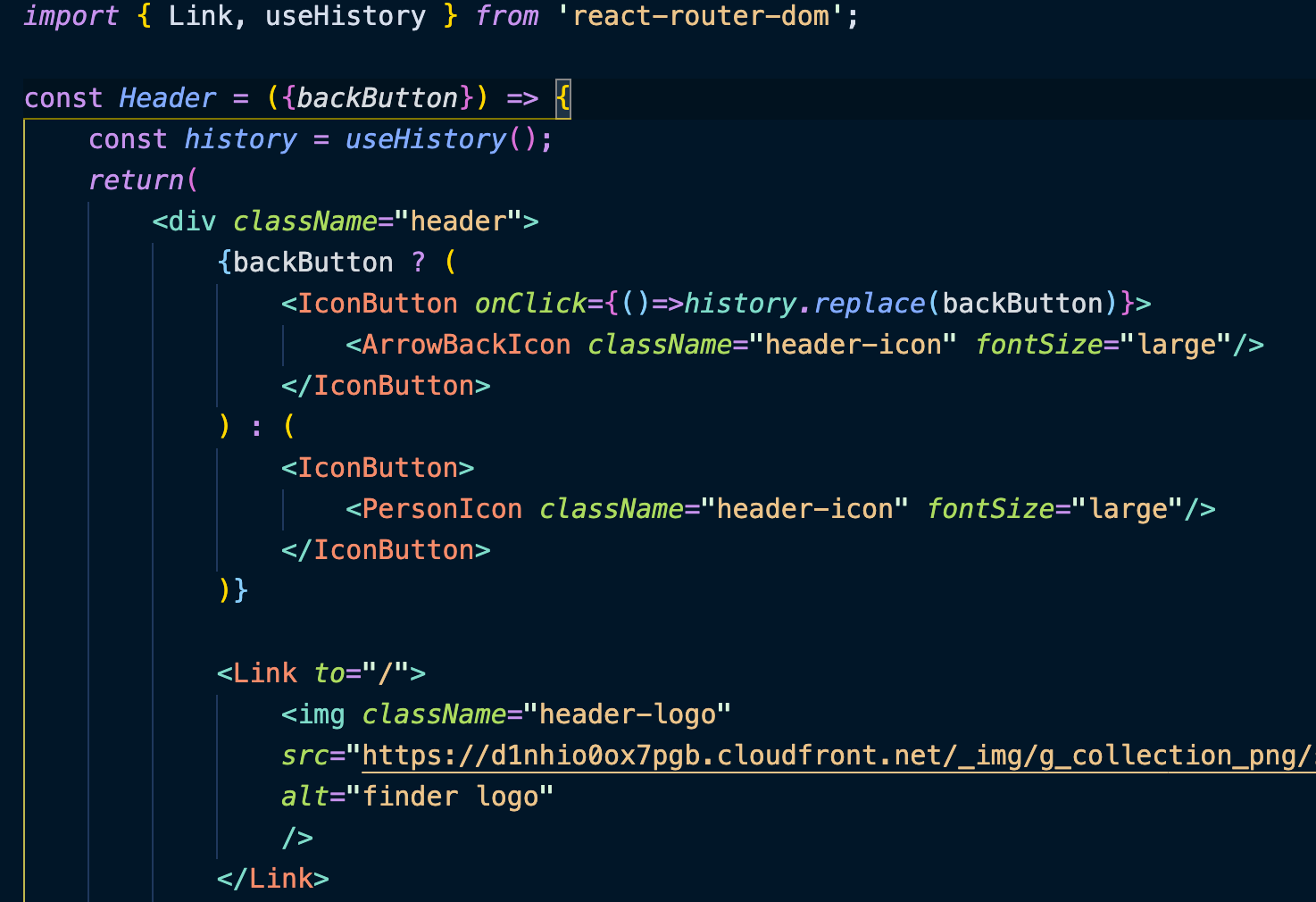
The following video is an example of what I built with a link to go back and forth between main page and chat page of the app.
以下视频是我使用链接构建的示例,该链接可在应用程序的主页和聊天页面之间来回切换。
All in all, there are a lot of things that we can learn about React.js, and I certainly haven’t covered everything. There are so many more things to learn and I am excited to dive into them day by day, and share with the world what I have learned! Thanks for reading my article, for more information about the topics, checkout the following links:
总而言之,我们可以从React.js中学到很多东西,我当然还没有涵盖所有内容。 还有很多东西要学习,我很高兴能每天深入学习它们,并与世界分享我所学到的东西! 感谢您阅读我的文章,有关主题的更多信息,请查看以下链接:
构建react项目