react.js获取定位
Spreadsheets are popular since they act as a database that’s easy to modify for non-developers. Anyone with a laptop and internet connection can use spreadsheets and insert useful information.
电子表格很受欢迎,因为它们充当的数据库对于非开发人员来说很容易修改。 拥有笔记本电脑和互联网连接的任何人都可以使用电子表格并插入有用的信息。
As developers, we’re constantly searching for ways to improve our everyday workflow. Here’s a way to save time by fetching data straight from Google sheets with React and tabletop.js.
作为开发人员,我们一直在寻找改善日常工作流程的方法。 这是一种通过使用React和tabletop.js直接从Google表格中获取数据来节省时间的方法。
We’re going to use tabletop since it easily integrates with Google Spreadsheets. The library is used for getting JSON from a Google Spreadsheet without jumping through a thousand hoops.
我们将使用桌面,因为它可以轻松地与Google Spreadsheets集成。 该库用于从Google Spreadsheet获取JSON,而无需跳过上千圈。
入门 (Getting started)
Step One: make a Google Spreadsheet and click “Publish to Web.”
第一步:制作一个Google电子表格 ,然后点击“发布到网络”。
Step Two: Install the tabletop library.
第二步:安装桌面库。
yarn add tabletop
Step Three: Write the React component that fetches the data from Google sheets.
第三步 :编写React组件,以从Google表格中获取数据。
import React, { useEffect, useState, Fragment } from "react";
import Tabletop from "tabletop";
export default function App() {
const [data, setData] = useState([]);
useEffect(() => {
Tabletop.init({
key: "1TK1Qj6kfA90KbmFAdnIOtKUttpJUhZoZuOPy925c6nQ",
simpleSheet: true
})
.then((data) => setData(data))
.catch((err) => console.warn(err));
}, []);
return (
<>
<h1>data from google sheets</h1>
// render data here
</>
);
}
Note: we’re using React hooks — if you’re new to them, check out this article where I explained React hooks in depth.
注意:我们正在使用React钩子-如果您是不熟悉它们, 请查看本文 ,在其中我深入解释了React钩子。
Here’s what happens inside the React component above;
这是上面的React组件内部发生的情况;
We’re initializing the Tabletop library inside the
useEffect
hook. The init function takes an object as an argument. This is where we place the information about our Google sheets. Notice thekey
prop.我们正在
useEffect
挂钩中初始化Tabletop库。 init函数将对象作为参数。 我们在这里放置有关Google表格的信息。 注意key
道具。

key prop key prop
Once the tabletop library is done initializing, it’s going to fetch the data from google spreadsheets. If the query is successful, we store the retrieved data inside the react hook which is called
data
.桌面库完成初始化后,它将从Google电子表格中获取数据。 如果查询成功,我们将检索到的数据存储在称为
data
的react挂钩中。
渲染数据 (Rendering data)
Rendering the data on the screen is quite straight-forward. We’re going to map over the array of data.
在屏幕上渲染数据非常简单。 我们将映射数据数组。
return (
<>
<h1>data from google sheets</h1>
<ul>
{data.map((item, i) => (
<Fragment key={i}>
<li>URL -- {item.URL}</li>
<li>Email - {item.email}</li>
<li>Token - {item.token}</li>
<br />
</Fragment>
))}
</ul>
</>
);
这是结果 (Here’s the result)
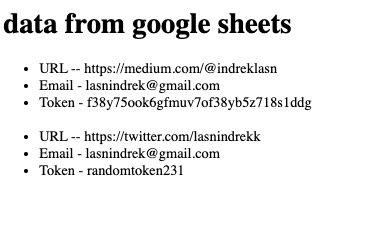
Well done! You did it.
做得好! 你做到了。
这是一些使用tabletop.js从Google表格中获取数据的陷阱 (Here are a few gotchas with fetching data from google sheets with tabletop.js)
- All columns must be named in the first row and may not contain any strange characters (%, $, &). 所有列都必须在第一行中命名,并且不能包含任何奇怪的字符(%,$和&)。
- Google assumes an empty row is the end of the data and doesn’t return any rows thereafter. Google假定数据的末尾为空行,此后不返回任何行。
NOTE: If your share URL has a
/d/e
in it, try refreshing the page to see if it goes away. If it doesn't, try this.注意:如果您的共享URL中包含
/d/e
,请尝试刷新页面以查看它是否消失。 如果不是,请尝试此 。If you want to deal with multiple sheets you can get rid of the
simpleSheet
property.如果要处理多个工作表,则可以摆脱
simpleSheet
属性。Check out the tabletop documentation for a deeper dive.
查看桌面文档 ,以进行更深入的了解。
这是完整的React组件 (Here’s the full React component)
import React, { useEffect, useState, Fragment } from "react";
import Tabletop from "tabletop";
import "./styles.css";
export default function App() {
const [data, setData] = useState([]);
useEffect(() => {
Tabletop.init({
key: "1TK1Qj6kfA90KbmFAdnIOtKUttpJUhZoZuOPy925c6nQ",
simpleSheet: true
})
.then((data) => setData(data))
.catch((err) => console.warn(err));
}, []);
return (
<>
<h1>data from google sheets</h1>
<ul>
{data.map((item, i) => (
<Fragment key={i}>
<li>URL -- {item.URL}</li>
<li>Email - {item.email}</li>
<li>Token - {item.token}</li>
<br />
</Fragment>
))}
</ul>
</>
);
}
In case you’re feeling curious, here’s the interactive demo. Try it out!
如果您感到好奇,这是交互式演示。 试试看!
Thanks for reading, happy coding!
感谢您的阅读,编码愉快!
react.js获取定位