firebase 云推送
历史 (History)
If you are working with Android long enough you could have known about Google Cloud Messaging (GCM), a mobile notification service. It was developed by Google that enables third-party application developers to send notification data or information from servers to applications and web free for charge
如果您使用Android的时间足够长,那么您可能已经了解移动通知服务Google Cloud Messaging(GCM)。 它由Google开发,可让第三方应用程序开发人员免费将通知数据或信息从服务器发送到应用程序和网络,
The GCM service was first announced in June 2012 as a successor to Google’s now-defunct Android Cloud to Device Messaging (C2DM) service, citing improvements to authentication and delivery, new API endpoints, and messaging parameters, and the removal of limitations on API send-rates and message sizes.
GCM服务于2012年6月首次发布,它是Google现已淘汰的Android Cloud to Device Messaging(C2DM)服务的继任者,理由是对身份验证和传递,新API端点和消息传递参数的改进以及对API发送限制的取消-费率和邮件大小。
It has been superseded by Google’s Firebase Cloud Messaging (FCM). On April 10, 2018, Google deprecated GCM. The GCM server and client APIs were removed on May 29, 2019.
它已被Google的Firebase Cloud Messaging (FCM)取代。 Google在2018年4月10日弃用了GCM。 GCM服务器和客户端API已于2019年5月29日删除。
In this article, we’re going to learn what’s FCM, how we can integrate it into the app’s, and sending sample messages through FCM.
在本文中,我们将学习什么是FCM,如何将其集成到应用程序中以及如何通过FCM发送示例消息。
什么是Firebase Cloud Messaging? (What is Firebase Cloud Messaging?)
Firebase Cloud Messaging (FCM) is a cross-platform cloud messaging solution that lets you reliably send messages at no cost. In simple words, we can say that FCM is a highly sophisticated and advanced version of GCM.
Firebase Cloud Messaging(FCM)是一种跨平台的云消息传递解决方案,可让您可靠地免费发送消息。 简而言之,我们可以说FCM是GCM的高度复杂和高级的版本。
FCM provides a reliable and battery-efficient connection between your server and devices that allows you to deliver and receive messages via notifications on iOS, Android, and the web at no cost. It has many interesting features like
FCM在服务器和设备之间提供了可靠且省电的连接,使您可以通过iOS,Android和Web上的通知免费发送和接收消息。 它具有许多有趣的功能,例如
- Advanced message targeting 进阶讯息定位
- Customized notification content 定制的通知内容
- A/B test notifications, etc A / B测试通知等
FCM is a powerful and scalable service delivering hundreds of billions of messages per day with 95% of messages being delivered in 250 milliseconds. FCM with zero-cost and unlimited features developers can play around to experiment and analyze things. It’s very easy to integrate. The overall picture of the FCM is as below
FCM是一项功能强大且可扩展的服务,每天可传递数千亿条消息,其中95%的消息在250毫秒内被传递。 具有零成本和无限制功能的FCM开发人员可以进行试验和分析。 集成非常容易。 FCM的总体情况如下
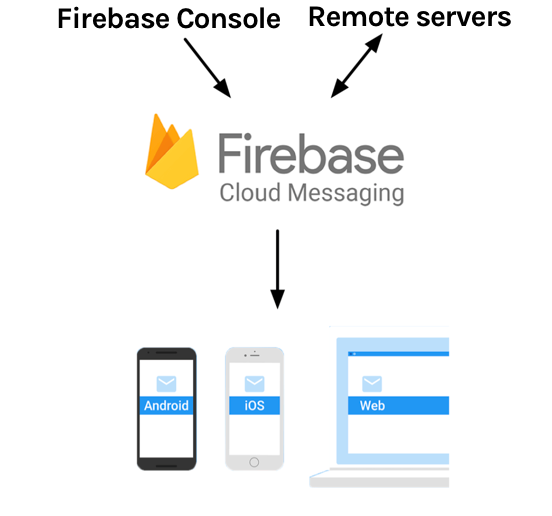
实作 (Implementation)
Let’s break this into a step-by-step procedure for better understanding.
让我们将其分解为逐步的过程,以便更好地理解。
- Register app at Firebase console 在Firebase控制台上注册应用
- Setup the Firebase stuff at the app level 在应用程序级别设置Firebase内容
- Set up the FCM SDK 设置FCM SDK
- Configure the app manifest file 配置应用清单文件
- Access the device registration token 访问设备注册令牌
- Custom notification handling 自定义通知处理
- Sending messages to clients from Firebase console. 从Firebase控制台向客户端发送消息。
Let’s analyze each step mentioned above in detail.
让我们详细分析上面提到的每个步骤。
在Firebase控制台上注册应用 (Register app at Firebase console)
From the Firebase console, open or add your project. Creating a project is simple just click on add project and follow two simple steps like giving a name to the project, enabling disabling analytics, and account selection. Now that we have successfully