android 应用内更新
I’m sure we all must have come across the situations while working on the applications where we needed to send out an app update can say like a hotfix — maybe there’s a security issue or some bug which will break the experience for the users. In such scenarios earlier, we’ve needed to roll out a new update on the Google play store and wait for our users to receive the update. And if they don’t have automatic updates installed, we rely on the user visiting the play store to update our application or we send out a force update to the users.
我确信我们在处理需要发送应用程序更新的应用程序时一定会遇到各种情况,就像修复程序一样,也许是安全问题或某些错误会破坏用户的体验。 在这种情况下,我们需要在Google Play商店中推出新的更新,并等待我们的用户收到更新。 而且,如果他们没有安装自动更新,我们将依靠用户访问Play商店来更新我们的应用程序,或者我们向用户发送强制更新。
Keeping your app up-to-date on your users’ devices enables them to try new features, as well as benefit from performance improvements and bug fixes.
使应用程序在用户设备上保持最新状态,使他们能够尝试新功能,并从性能改进和错误修复中受益。
In-app updates is a Play Core library feature that introduces a new request flow to prompt active users to update your app.
应用内更新是Play Core库的一项功能,它引入了新的请求流,以提示活跃用户更新您的应用。
In simple words, it provides an option to the user which notifies the user that an app update is available and the user can select if they are interested in updating the app or not.
简而言之,它向用户提供了一个选项,通知用户可用的应用程序更新,并且用户可以选择是否对更新应用程序感兴趣。
Not only that, but it also provides an option to download the update of the app in the background, and once downloaded, install the update and restart the app and the user doesn’t have to do anything.
不仅如此,它还提供了一个在后台下载应用程序更新的选项,下载后,安装更新并重新启动应用程序,用户无需执行任何操作。
In-app updates work only with devices running Android 5.0 (API level 21) or higher and require you to use Play Core library 1.5.0 or higher. Additionally, in-app updates support apps running on only Android mobile devices and tablets, and Chrome OS devices.
应用内更新仅适用于运行Android 5.0(API级别21)或更高版本的设备,并且要求您使用Play Core库 1.5.0或更高版本。 此外,应用内更新支持仅在Android移动设备和平板电脑以及Chrome操作系统设备上运行的应用。
The Play Core library offers us two different ways in which we can prompt our users that an update is available — either using the Flexible or Immediate approach. Let’s take a look at each of them one by one-
Play Core库为我们提供了两种不同的方式,可以使用灵活或立即方式提示用户更新可用。 让我们一一看一下-
Flexible: A user experience that provides background download and installation with graceful state monitoring. This UX is appropriate when it’s acceptable for the user to use the app while downloading the update. For example, you want to urge users to try a new feature that’s not critical to the core functionality of your app.
灵活:一种用户体验,可通过后台状态监视提供后台下载和安装。 如果用户可接受在下载更新时使用该应用程序,则此UX适用。 例如,您想敦促用户尝试一项对您的应用程序的核心功能并不重要的新功能。
Immediate: A full-screen user experience that requires the user to update and restart the app to continue using the app. This UX is best for cases where an update is critical for continued use of the app. After a user accepts an immediate update, Google Play handles the update installation and app restart.
立即:全屏用户体验,要求用户更新并重新启动应用程序才能继续使用该应用程序。 此UX最适合更新对于继续使用该应用至关重要的情况。 用户接受即时更新后,Google Play会处理更新安装并重新启动应用程序。
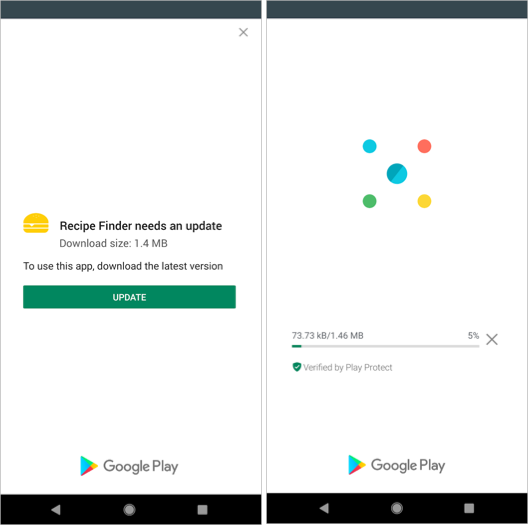
Now we all have an understanding of both the types of In-app updates available we will start implementing it.
现在我们都对两种可用的应用程序内更新类型有了了解,我们将开始实施它。
The very first step is to check for the update-
第一步是检查更新 -
Before requesting an update, you need to check if an update is available or not and also the type of update available. We do not want users to have a bad experience. To check for an update you can use the AppUpdateManager.
在请求更新之前,您需要检查更新是否可用以及更新的类型。 我们不希望用户体验不佳。 要检查更新,可以使用AppUpdateManager。
val appUpdateManager: AppUpdateManager = AppUpdateManagerFactory.create(baseContext)val appUpdateInfo = appUpdateManager.appUpdateInfo// Creates instance of the manager.
val appUpdateManager = AppUpdateManagerFactory.create(context)// Returns an intent object that you use to check for an update.val appUpdateInfoTask = appUpdateManager.appUpdateInfo// Checks that the platform will allow the specified type of update.
appUpdateInfo?.addOnSuccessListener { appUpdateInfo ->
if ((appUpdateInfo.updateAvailability() == UpdateAvailability.UPDATE_AVAILABLE ||
appUpdateInfo.updateAvailability() == UpdateAvailability.DEVELOPER_TRIGGERED_UPDATE_IN_PROGRESS) &&
appUpdateInfo.isUpdateTypeAllowed(AppUpdateType.FLEXIBLE)
) {
btn_update.visibility = View.VISIBLE
setUpdateAction(appUpdateManager, appUpdateInfo)
}}
Here we have created a button to show some UX experience. Whenever there will be a flexible update available we will show the update button to the users. As we have an update available of flexible types, we will start the update.
在这里,我们创建了一个按钮来显示一些UX体验。 只要有可用的灵活更新,我们就会向用户显示更新按钮。 由于我们有可用的灵活类型更新,因此将开始更新。
private fun setUpdateAction(manager: AppUpdateManager?, info: Task<AppUpdateInfo>) {
// Before starting an update, register a listener for updates.btn_update.setOnClickListener {
installStateUpdatedListener = InstallStateUpdatedListener {
btn_update.visibility = View.GONE
// tv_status is a textview to display the update status
tv_status.visibility = View.VISIBLE
when (it.installStatus()) {
InstallStatus.FAILED, InstallStatus.UNKNOWN -> {
tv_status.text = getString(R.string.info_failed)
btn_update.visibility = View.VISIBLE
}
InstallStatus.PENDING -> {
tv_status.text = getString(R.string.info_pending)
}
InstallStatus.CANCELED -> {
tv_status.text = getString(R.string.info_canceled)
}
InstallStatus.DOWNLOADING -> {
tv_status.text = getString(R.string.info_downloading)
}
InstallStatus.DOWNLOADED -> {
tv_status.text = getString(R.string.info_downloaded)
popupSnackbarForCompleteUpdate(manager)
}
InstallStatus.INSTALLING -> {
tv_status.text = getString(R.string.info_installing)
}
InstallStatus.INSTALLED -> {
tv_status.text = getString(R.string.info_installed)
manager?.unregisterListener(installStateUpdatedListener)
}
else -> {
tv_status.text = getString(R.string.info_restart)
}
}
}
manager?.registerListener(installStateUpdatedListener)
manager?.startUpdateFlowForResult( info.result, AppUpdateType.FLEXIBLE,
this, IN_APP_UPDATE_REQUEST_CODE
)
}}
While doing the update, you can handle the update related failure or cancellation by using the onActivityResult() as follows:
在执行更新时,可以通过使用onActivityResult()来处理与更新相关的失败或取消,如下所示:
/** This is needed to handle the result of the manager.startConfirmationDialogForResult request */override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
if (requestCode == IN_APP_UPDATE_REQUEST_CODE) {
if (resultCode != RESULT_OK) {
toastAndLog("Update flow failed! Result code: $resultCode")
// If the update is cancelled or fails,
// you can request to start the update again.
}
} else {
super.onActivityResult(requestCode, resultCode, data)
}
}
The following describes the different values you may receive from the onActivityResult()
callback:
以下内容描述了您可能从onActivityResult()
回调中收到的不同值:
RESULT_OK
: The user has accepted the update. For immediate updates, you might not receive this callback because the update should already be completed by Google Play by the time the control is given back to your app.RESULT_OK
:用户已接受更新。 对于即时更新,您可能不会收到此回调,因为在将控件交还给您的应用程序之前,Google Play应该已经完成了更新。RESULT_CANCELED
: The user has denied or canceled the update.RESULT_CANCELED
:用户已拒绝或取消了更新。ActivityResult.RESULT_IN_APP_UPDATE_FAILED
: Some other error prevented either the user from providing consent or the update to proceed.ActivityResult.RESULT_IN_APP_UPDATE_FAILED
:其他一些错误阻止用户提供同意或继续进行更新。
Install a Flexible Update
安装弹性更新
If you monitor the flexible update states and you detect the InstallStatus.DOWNLOADED
state, you need to restart the app to install the update.
如果您监视灵活更新状态并检测到InstallStatus.DOWNLOADED
状态,则需要重新启动应用程序以安装更新。
Unlike an immediate update, Google Play does not trigger an app restart for you. That’s because, during a flexible update, the user expects to keep using the app until they decide that they want to install the update.
与立即更新不同,Google Play不会为您触发应用重启。 这是因为在灵活更新期间,用户希望继续使用该应用程序,直到他们决定要安装更新为止。
So, it’s recommended that you provide the user some notification or UI indication to notify that an update is ready for installation and requests user confirmation to restart the app.
因此,建议您向用户提供一些通知或UI指示,以通知已准备好安装更新并请求用户确认以重新启动应用程序。
Here we will implement a snackbar to request the user confirmation to restart the app.
在这里,我们将实现一个快餐栏,以请求用户确认以重新启动应用程序。
/* Displays the snackbar notification and call to action. */private fun popupSnackbarForCompleteUpdate(appUpdateManager: AppUpdateManager?) {
Snackbar.make(
findViewById(R.id.mainLayoutParent),
"Ready to INSTALL.", Snackbar.LENGTH_INDEFINITE
).apply {
setAction("RESTART") { appUpdateManager?.completeUpdate() }
setActionTextColor(ContextCompat.getColor(applicationContext, R.color.colorAccent))
show()
}}
When you call appUpdateManager?.completeUpdate()
in the foreground, the platform displays a full-screen UI which restarts the app in the background. After the platform installs the update, the app restarts into its main activity.
当您在前台调用appUpdateManager?.completeUpdate()
时,平台将显示全屏UI,该UI在后台重新启动该应用。 平台安装更新后,该应用程序将重新启动进入其主要活动。
If you instead call appUpdateManager?.completeUpdate()
when the app is in the background, the update is installed silently without obscuring the device UI.
如果改为在后台运行应用程序时调用appUpdateManager?.completeUpdate()
,则更新将以静默方式安装,而不会模糊设备UI。
When the user brings your app to the foreground, it’s recommended that you check that your app doesn’t have an update waiting to be installed. If your app has an update in the DOWNLOADED
state, show the notification to request that the user install the update, as shown. Otherwise, the update data continues to occupy the user’s device storage.
当用户将您的应用程序置于前台时,建议您检查您的应用程序没有等待安装的更新。 如果您的应用程序的更新处于“已DOWNLOADED
状态,请显示通知以请求用户安装更新,如图所示。 否则,更新数据将继续占用用户的设备存储。
// Checks that the update is not stalled during 'onResume()'.
// However, you should execute this check at all app entry points.
override fun onResume() {
super.onResume()
appUpdateManager.appUpdateInfo
.addOnSuccessListener {
if (it.updateAvailability() == UpdateAvailability.UPDATE_AVAILABLE || UpdateAvailability.DEVELOPER_TRIGGERED_UPDATE_IN_PROGRESS) {
updateManager.startUpdateFlowForResult(
it,
IMMEDIATE,
this,
IN_APP_UPDATE_REQUEST_CODE)
}
}
}
}
处理立即更新 (Handle an immediate update)
If you are performing an immediate update, and the user consents to install the update, Google Play displays the update progress on top of your app’s UI across the entire duration of the update. During the update, if the user closes or terminates your app, the update should continue to download and install in the background without additional user confirmation.
如果您正在执行立即更新,并且用户同意安装此更新,则Google Play会在整个更新过程中在应用程序UI顶部显示更新进度。 在更新过程中,如果用户关闭或终止了您的应用,则更新应继续下载并在后台安装,而无需其他用户确认。
appUpdateInfo?.addOnSuccessListener { appUpdateInfo ->
if ((appUpdateInfo.updateAvailability() == UpdateAvailability.UPDATE_AVAILABLE ||
appUpdateInfo.updateAvailability() == UpdateAvailability.DEVELOPER_TRIGGERED_UPDATE_IN_PROGRESS) &&
appUpdateInfo.isUpdateTypeAllowed(AppUpdateType.IMMEDIATE)
) {
updateManager.startUpdateFlowForResult(it, IMMEDIATE, this, IN_APP_UPDATE_REQUEST_CODE)
}}
To check how to test In-app updates please refer: https://developer.android.com/guide/playcore/in-app-updates
要检查如何测试应用内更新,请参阅: https : //developer.android.com/guide/playcore/in-app-updates
结论 (Conclusion)
In this blog, we’ve learned about the new approach to in-app updates that are provided by the play core library. You can also check out how to set the appUpdatePriority and clientVersionStalenessDays.
在此博客中,我们了解了Play核心库提供的应用内更新的新方法。 您还可以查看如何设置appUpdatePriority和clientVersionStalenessDays。
Do checkout my GitHub repo for the implementation
检查我的GitHub存储库以实现
https://github.com/ashitaasati1608/InAppUpdate
https://github.com/ashitaasati1608/InAppUpdate
If you have any questions about the play core library or in-app updates then please do reach out.
如果您对Play核心库或应用内更新有任何疑问,请联系我们。
Happy Coding!!
快乐编码!
翻译自: https://medium.com/swlh/implementing-in-app-updates-in-android-26ea27609bd2
android 应用内更新