Set Methods
设定方法
(1) Three Features of the Sets
(1)集合的三个特征
There are 3 important features of sets. Sets are collections that are:
集合有3个重要特征。 集合是以下集合:
unique elements
独特元素
mutable, but the elements in a set must be immutable (hashable)
可变的,但集合中的元素必须是不可变的(可哈希)
unordered
无序的
By the previous code, we can also list all the methods of the set.
通过前面的代码,我们还可以列出集合的所有方法。
[method for method in dir(set) if not method.startswith("__")]
then,
然后,
['add',
'clear',
'copy',
'difference',
'difference_update',
'discard',
'intersection',
'intersection_update',
'isdisjoint',
'issubset',
'issuperset',
'pop',
'remove',
'symmetric_difference',
'symmetric_difference_update',
'union',
'update']
(2) Set Methods
(2)设定方法
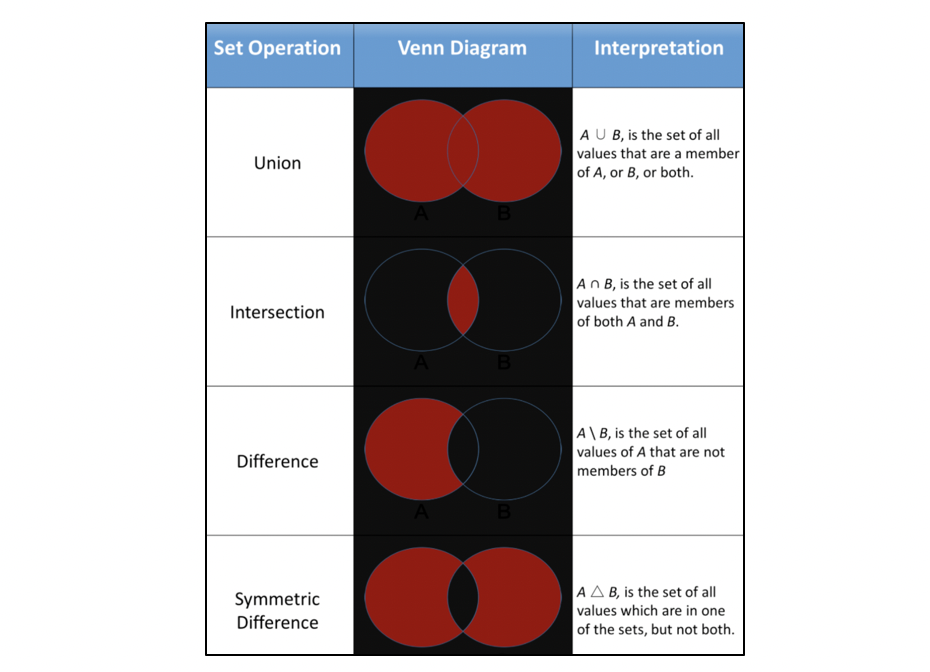
Suppose we have two sets, old and new, then,
假设我们有两套旧的和新的,
- union 联盟
new.union(old)
- intersection 路口
new.intersection(old) # or new & old
- difference 区别
new.difference(old) # or new - old
- symmetric difference 对称差异
new.symmetric_difference(old) # new ^ old
- add an element to the set (in-place function) (no change if already exists) 将元素添加到集合中(就地功能)(如果已经存在,则保持不变)
new.add('a')
- copy a set (because set is mutable) 复制一个集合(因为集合是可变的)
New = new.copy()
- update a set with its union with another set (in-place) (which is also be considered as union_update but they just define this name like that) 用一个联合与另一个联合(就地)来更新一个联合(也被视为union_update,但他们只是这样定义此名称)
new.update(old) # the same of new = new.union(old)
- update a set with its intersection with another set (in-place) 更新一个集与另一个集的交集(就地)
new.intersection_update(old)
- update a set with its difference with another set (in-place) 用一个差异与另一个差异更新一个差异(就地)
new.difference_update(old)
- update a set with its symmetric difference with another set (in-place) 用另一个集合(就地)用其对称差异更新一个集合
new.symmetric_difference_update(old)
- discard an element in this set (in-place) (when this element does not exist, it will in-place the original set) 丢弃此集合中的某个元素(就地)(当此元素不存在时,它将代替原始集合)
new.discard('a')
- randomly pop an element form the set (in-place, return the poped element) 从集合中随机弹出一个元素(就位,返回弹出的元素)
new.pop()
- check whether two set not have a same element 检查两个集合是否没有相同的元素
new.isdisjoint(old)
- check whether a set is the subset of another set 检查一个集合是否是另一个集合的子集
new.issubset(old)
- check whether a set is the superset of another set 检查一个集合是否是另一个集合的超集
new.issuperset(old)
- clear a set (in-place) 清除一组(就地)
new.clear()
Example #1. What is the output of the following program?
范例#1。 以下程序的输出是什么?
a = [1,2,3,4]
b = [5,6,7,8]
P = set(a)
P = P.update(set(b))
print(P)
Output:
输出:
None
This is because update is an in-place method.
这是因为update是就地方法。
Example #2. What is the output of the following program?
范例#2。 以下程序的输出是什么?
aSet = {1, 'PYnative', ('abc', 'xyz'), True}
print(aSet)
Output:
输出:
{1, 'PYnative', ('abc', 'xyz')}
This is because the hash for True and the hash value for 1 are the same.
这是因为True的哈希值和1的哈希值相同。
Example #3. What is the output of the following program?
范例#3。 以下程序的输出是什么?
aSet = {1, 'PYnative', ['abc', 'xyz'], True}
print(aSet)
Output:
输出:
Error
This is because the list is not hashable.
这是因为该列表不可散列。
Example #4. What is the output of the following program?
范例#4。 以下程序的输出是什么?
sampleSet = {"Yellow", "Orange", "Black"}
sampleSet.update(["Blue", "Green", "Red"])
print(sampleSet)
Output:
输出:
{'Green', 'Orange', 'Black', 'Blue', 'Red', 'Yellow'}
Note that the argument for method union
, update
, differnce
, differnce_update
, intersection
, intersection_update
, symmetric_difference
, symmetric_difference_update
, isdisjoint
, issubset
, and issuperset
can be set, list, tuple, or any kinds of iterable data type.
注意,对于方法的参数union
, update
, differnce
, differnce_update
, intersection
, intersection_update
, symmetric_difference
, symmetric_difference_update
, isdisjoint
, issubset
和issuperset
可设定,列表,元组,或任何种类的可迭代数据类型。
(3) Set application
(3)安排申请
- Eliminate Duplicated Elements消除重复元素
By changing a list to a set, we can eliminate all the duplicated elements in that list.
通过将列表更改为集合,我们可以消除该列表中所有重复的元素。
set(myList)
- Membership Testing 会员资格测试
It will be faster if we use set for membership test compare with a list.
如果将set用于成员资格测试与列表进行比较,它将更快。
anitem in set(myList)
2. Dictionary Methods
2.词典方法
(1) Dictionary Methods In A Nutshell
(1)简而言之字典方法
The feature of the dictionary is that the dictory itself is mutable but the keys in the dictionary must be immutable.
字典的特点是字典本身是可变的,但字典中的键必须是不变的。
[method for method in dir(dict) if not method.startswith('__')]
then,
然后,
['clear',
'copy',
'fromkeys',
'get',
'items',
'keys',
'pop',
'popitem',
'setdefault',
'update',
'values']
(2) Dictionary Methods (Suppose we have dictionary cart)
(2)词典方法(假设我们有词典购物车)
return the keywords of a dictionary (the return type is a
dict_keys
type and this can be iterated)返回字典的关键字(返回类型是
dict_keys
类型,可以迭代)
cart.keys()
return the values of a dictionary (the return type is a
dict_values
type and this can be iterated)返回字典的值(返回类型是
dict_values
类型,可以迭代)
cart.values()
return list of tuples of keywords and keywords of a dictionary (the return type is a
dict_items
type and this can be iterated)返回关键字的元组列表和字典的关键字(返回类型是
dict_items
类型,可以迭代)
cart.items()
- Never use keyword index: keyword index is definitely not a good idea because if you haven’t got a key inside a dictionary then it will raise an error. We can use this to assign the value of a keyword and this is a good to do. 永远不要使用关键字索引:关键字索引绝对不是一个好主意,因为如果您在字典中没有关键字,那么它将引发错误。 我们可以使用它来分配关键字的值,这是一件好事。
cart['apple'] # avoid using things like this to get a value
- get the value by keyword index with one argument keyword. This is a much better way because it will return None if there this keyword doesn’t exist. 使用一个参数关键字按关键字索引获取值。 这是一种更好的方法,因为如果此关键字不存在,它将返回None。
cart.get('Apples')
- get the value by keyword index, if this keyword doesn’t exist, add this keyword to the dictionary and assign the value of this keyword as the second argument. This is always being used to initialize a dictionary. 通过关键字索引获取值,如果该关键字不存在,则将该关键字添加到字典中,并将该关键字的值分配为第二个参数。 总是使用它来初始化字典。
cart['Apples'] = cart.get('Apples', 0)
Or we can use this to get any value by index.
或者我们可以使用它通过索引获取任何值。
cart.get('Limes', "Not on in the shopping cart")
- pop an item by keyword, this will return the poped value (in-place). We can not pop a keyword that doesn’t exist in this dictionary. 按关键字弹出项目,这将返回弹出值(就地)。 我们无法弹出该词典中不存在的关键字。
cart.pop()
- randomly pop an item, this will return the poped item in a tuple (in-place) 随机弹出一个项目,这将在一个元组中返回弹出项目(就地)
cart.popitem('Apples')
- add a new item to the given dictionary (in-place) 将新项添加到给定词典中(就地)
cart.update({'Banana': 5})
or
要么
cart.update(Banana=5)
or
要么
cart.update([(Banana,5)])
- change the value of an existing keyword in the dictionary 更改字典中现有关键字的值
cart.update({'Apples': 5})
- set a default None value to a not existing keyword, and return None if the keyword doesn’t exist, while return the value of this keyword if the keyword exists (if the keyword exists, it won’t change anything) (in-place) 将默认的None值设置为不存在的关键字,如果该关键字不存在,则返回None;如果该关键字存在,则返回此关键字的值(如果该关键字存在,则不会更改任何内容)(就地)
cart.setdefault('Apples')
- setup a dictionary from an iteration of keys, the values will be initialized to None 从键的迭代中设置字典,值将被初始化为None
dict.fromkeys(myList)
We can also assign the same value for all the keywords.
我们还可以为所有关键字分配相同的值。
dict.fromkeys(myList, myValues)
- copy and assign a dictionary 复制并分配字典
new_cart = cart.copy()
- clear a dictionary 清除字典
cart.clear()
- remove an item from the dictionary (not using method, if we want to use the method, use pop, and we haven’t got remove here) 从字典中删除一个项目(不使用方法,如果要使用该方法,请使用pop,但此处没有将其删除)
del cart['Apples']
Example #1. What is the output of the following program?
范例#1。 以下程序的输出是什么?
keys = {'a', 'e', 'i', 'o', 'u' }
value = [1]
vowels = dict.fromkeys(keys, value)
value.append(2)
print(vowels)
Output:
输出:
{'a': [1, 2], 'u': [1, 2], 'o': [1, 2], 'e': [1, 2], 'i': [1, 2]}
This is because list is mutable.
这是因为列表是可变的。
Example #2. What is the output of the following program?
范例#2。 以下程序的输出是什么?
cart_1 = {}
cart_1.update({'Apples': 3})
cart_1.update({'Oranges': 3})
print(cart_1)
cart_2 = {}
cart_2.update({'Oranges': 3})
cart_2.update({'Apples': 3})
print(cart_2)
print(cart_1 == cart_2)
Output:
输出:
{'Apples': 3, 'Oranges': 3}
{'Oranges': 3, 'Apples': 3}
True
This is because Python 3.6 keeps insertion order in dictionaries. But it doesn’t contain a real order, so the dictionaries with different insertion order can still be equal.
这是因为Python 3.6将插入顺序保留在字典中。 但是它不包含真实顺序,因此具有不同插入顺序的字典仍然可以相等。
Example #3. What is the output of the following program?
范例#3。 以下程序的输出是什么?
dict1 = {"name": "Mike", "salary": 8000}
temp = dict1.pop("age")
print(temp)
Output:
输出:
Error
Example #4. What is the output of the following program?
范例#4。 以下程序的输出是什么?
dict1 = {"name": "Mike", "salary": 8000}
temp = dict1.get("age")
print(temp)
Output:
输出:
None
Note that we have get in this case.
注意,在这种情况下,我们得到了。
(3) Tricky Staff with List Comprehension
(3)具有列表理解能力的花招
- Sort the insertion order of a dictionary by value按值对字典的插入顺序进行排序
dict(sorted(d.items(), key=lambda x: x[1]))
- Find keys in a dictionary that match a value 在字典中查找与值匹配的键
[k for k, v in myDictionary.items() if v == target_value]
- randomly pick an item 随机选择一个项目
import random
random.choice(list(d.items()))
3. Counter
3.柜台
Counter is a special type of dictionary that count the number of each unique element in a list. This is not a built-in function so we have to import it at the front.
计数器是一种特殊类型的字典,用于计算列表中每个唯一元素的数量。 这不是内置函数,因此我们必须在前面导入它。
from collections import Counter
For example, if we run
例如,如果我们运行
Counter("abracadabra")
then,
然后,
Counter({'a': 5, 'b': 2, 'r': 2, 'c': 1, 'd': 1})
This is commonly used in the settings of an NLP problem.
这通常在NLP问题的设置中使用。
4. General Questions
4.一般问题
Example #1. What is the output of the following program?
范例#1。 以下程序的输出是什么?
A = dict([(len, 'yes'),
(1+3j, 'yes'),
((2,3), 'yes'),
(15, 'yes'),
('foo', 'yes'),
(max, len),
(max, [1,2,3]),
(max, 12),
(max, dict([(1,2)]))])
print(A[max])
Output:
输出:
{1: 2}
functions are allowed for the keyword of the dictionary, things are not immutable(if/not/else/for/dict/list/etc…) are not allowed. For several keywords with the same context, we can only get the last one by indexing.
字典的关键字允许使用函数,不允许事物是不可变的(如果/不/其他/ for / dict /列表/等)。 对于具有相同上下文的几个关键字,我们只能通过建立索引来获取最后一个。
Example #2. What is the output of the following program?
范例#2。 以下程序的输出是什么?
d1 = {1, 2, 3}
d3 = {}
d3.update(d1)
print(d3)
Output:
输出:
Error
Can not update an empty set.
无法更新一个空集。
Example #3. What is the output of the following program?
范例#3。 以下程序的输出是什么?
d2 = {1: 1, 2: 2, 3: 3}
d4 = {}
d4.update(d2)
print(d4)
Output:
输出:
{1: 1, 2: 2, 3: 3}
翻译自: https://medium.com/adamedelwiess/advanced-python-5-set-dictionary-and-counter-1b81a9c3b89e