The popularity of MobX is getting higher and higher. So does the need to decrease the size of the bundle. And not only that, but we also seek to write cleaner and maintainable code in our projects. So how can we achieve this?
MobX的受欢迎程度越来越高。 因此,也需要减小束的大小。 不仅如此,我们还试图在我们的项目中编写更简洁,可维护的代码。 那么我们怎样才能做到这一点呢?
Since the React team released hooks from v16.8.0 — we were introduced with a new nice way to write the components in a functional way. In my personal opinion — the use of the hooks over the HOC’s looks way cleaner. Accessing the data from the store with hooks also seems cleaner. We tend to write less code with functional components comparing to big chunks of code that we used to write some times with Class-like components. That is one of the ways to increase the maintainability of the code.
自从React团队发布了v16.8.0版本的钩子以来,我们就被引入了一种新的很好的方式来以功能性方式编写组件。 我个人认为,HOC上的钩子看起来更干净。 使用钩子从存储访问数据似乎也更干净。 与以前使用类类组件编写代码的大块代码相比,我们倾向于使用功能组件编写的代码更少。 这是增加代码可维护性的方法之一。
捆的大小呢? (What about bundle size?)
Using lightweight packages alternatives is a great way to reduce the final bundle size. And we can do that with many packages. Remember using Moment.js? I don’t recall using it for a while because it has a way bigger bundle size that the alternative Date-fns package. Even tho the Moment still has twice more downloads and stars.
使用轻型包装替代品是减小最终捆束尺寸的好方法。 我们可以使用许多软件包来做到这一点。 还记得使用Moment.js吗? 我不记得有一段时间使用它了,因为它比替代的Date-fns包具有更大的捆绑包大小。 即使在片刻中,下载量和星级也增加了两倍。

Mobx-react-lite is a lightweight binding to glue Mobx stores and functional React components. And the size of it is smaller, but maybe not as much as you would expect. Nevertheless — this is all we need.
Mobx-react-lite是一种轻量级绑定,用于粘合Mobx存储和功能性的React组件。 而且它的大小较小,但可能没有您期望的那么大。 但是,这就是我们所需要的。

让我们开始吧 (Let’s get started)
We will need a React project setup and two dependencies to get started:
我们将需要一个React项目设置和两个依赖来开始:
yarn add mobx mobx-react-liteORnpm install mobx mobx-react-lite
For a simple example, we can create a Counter Store with some actions and values. I’m using Typescript with the feature enabled to support decorators syntax, but it does not matter if you are using decorators or functions for our case.
举一个简单的例子,我们可以创建一个带有一些动作和值的柜台商店。 我正在使用Typescript并启用了支持装饰器语法的功能,但是对于我们的案例而言,使用装饰器或函数并不重要。
In order to access the store from the component we will need to create the instance of the store and, of course, share it in some way with components. React Context is a quite good fit for this task and we can leverage it for our needs. Let’s create a stores.ts
file with the store instances and Context wrapper.
为了从组件访问商店,我们将需要创建商店的实例,并且当然要以某种方式与组件共享。 React Context非常适合此任务,我们可以利用它满足我们的需求。 让我们用商店实例和上下文包装器创建一个stores.ts
文件。
Good. Now we have a stores
variable to which we can save instances of the Mobx stores. We freeze the object in order to avoid any unexpected changes in it. But, of course, this is an optional step.
好。 现在我们有了一个stores
变量,可以在其中保存Mobx商店的实例。 我们冻结对象以避免任何意外更改。 但是,当然,这是一个可选步骤。
We created a React Context based on the store’s variables, and also created a Stores Provider component, we will use it shortly.
我们基于商店的变量创建了一个React Context,还创建了一个Stores Provider组件,我们将很快使用它。
Now, let’s update the root index.tsx
file with a Context Provider wrapper. Follow this code snippet.
现在,让我们使用上下文提供程序包装器更新根index.tsx
文件。 请遵循此代码段。
Very well. At this point, we have a place, where we keep our stores. We also have a way to share the stores with the components, but not completely. To access that context we have to create two custom and quite handy hooks. One will return all stores, another — specific store of preference.
很好。 在这一点上,我们有一个地方,我们保留我们的商店。 我们也有一种与组件共享商店的方法,但不是完全共享的。 要访问该上下文,我们必须创建两个自定义且非常方便的钩子。 一个将退回所有商店,另一个将退回特定的偏好商店。
Alright, now we have it. See that weird type definition for the useStore
hook? It would give us proper types for the passed store key.
好吧,现在我们有了它。 看到useStore
挂钩的奇怪类型定义了吗? 它将为传递的商店密钥提供适当的类型。
<T extends keyof typeof stores>
(store: T): typeof stores[T]
We will only accept store
variable type if it’s one of the keys of the stores
object. So, in our case, it will only accept a counterStore
string and will return the corresponding type of the given store. Nice, isn’t it?
如果它是stores
对象的键之一,我们将只接受store
变量类型。 因此,在我们的例子中,它将仅接受counterStore
字符串,并将返回给定商店的相应类型。 很好,不是吗?
Ok, let’s finally access the store, its methods, and properties from the actual component. To do that we have to modify App.tsx
file with the custom hooks and Mobx Observer wrapper.
好的,让我们最后从实际组件中访问商店,商店的方法和属性。 为此,我们必须使用自定义钩子和Mobx Observer包装器修改App.tsx
文件。
We access the counter store with it’s key and get the store in return. Also, we have to wrap the component into the observer
function to allow the component to listen for the store changes. But still, we do not destruct stores.
我们使用它的密钥访问柜台商店,并获得商店的回报。 另外,我们必须将组件包装到observer
函数中,以允许组件侦听商店更改。 但是,我们仍然不会破坏商店。
const counterStore = useStore("counterStore");
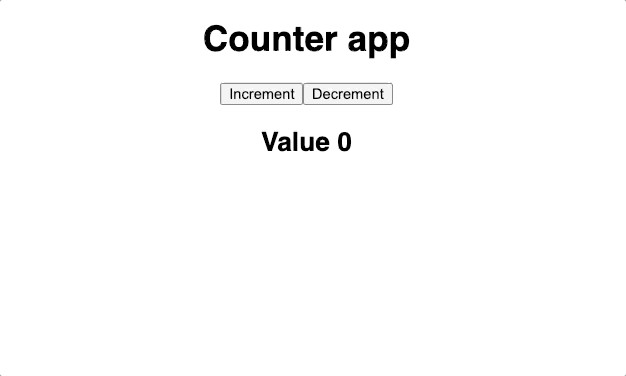
The app is working as expected, cool! Let’s see the TypeScript hints that we got from this setup and the ways it will cover our back from doing wrong things.
该应用程序按预期运行,很酷! 让我们看看从该设置中获得的TypeScript提示,以及它做错事情后将如何掩盖我们的后盾。
The first thing to mention is the code completion feature. When we will add the useStore
hook and start passing a string there as an argument — a key hint will be shown. We also will not be able to pass non-existing store keys. Nice!
首先要提到的是代码完成功能。 当我们添加useStore
钩子并开始在其中传递字符串作为参数时,将显示一个键提示。 我们也将无法传递不存在的商店密钥。 真好!


This also works for the case when we have multiple stores as well. We can access them with a separate useStore
hook or with a useStores
hook as well. Check this out.
当我们也有多个商店时,这也适用。 我们可以用一个单独的访问它们useStore
钩或用useStores
钩为好。 看一下这个。

测验(Testing)
Sure thing we need to test this. In this article, we won’t make tests for the store itself, since this is a topic of a different nature.
当然,我们需要对此进行测试。 在本文中,我们不会对商店本身进行测试,因为这是一个不同性质的主题。
I find it easy enough to use Jest alongside with React Testing Library. So, let’s add the necessary dependencies.
我发现将Jest与React Testing Library一起使用很容易。 因此,让我们添加必要的依赖项。
yarn add @testing-library/react-hooks react-test-renderer -DORnpm install @testing-library/react-hooks react-test-renderer --save-dev
Cool! Now we can start testing our hooks file. Nothing too fancy here, but still necessary.
凉! 现在我们可以开始测试我们的钩子文件了。 这里没什么好看的,但仍然有必要。
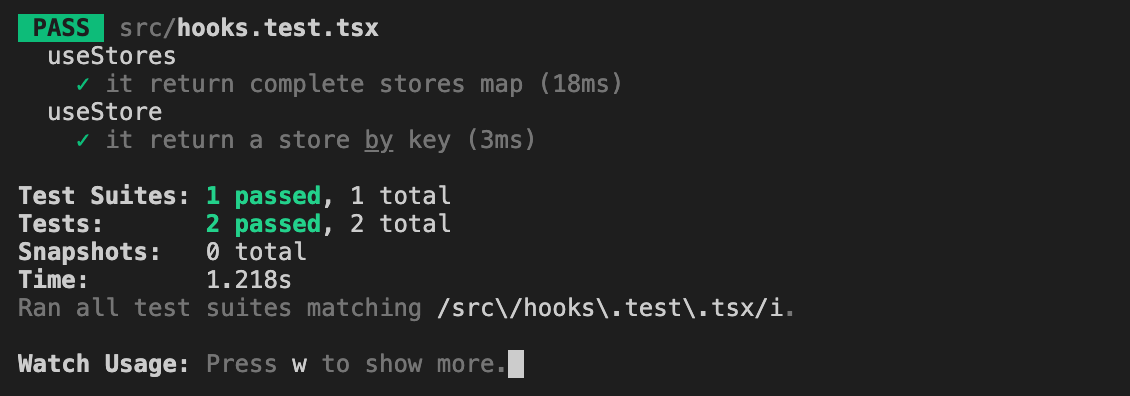
组件测试如何?(How about component tests?)
To do that we will need a few more dependencies. To test the components and to perform user events.
为此,我们将需要更多的依赖关系。 测试组件并执行用户事件。
yarn add @testing-library/react @testing-library/user-event -DORnpm install @testing-library/react @testing-library/user-event --save-dev
Good! Let’s test the components now. We can mock the useStore
hook to always return a specific store using Jest. And, we can be type-safe with a few extra lines of code. For every test we want the hook to return a new store. But, for one of the tests, we will change that slightly and replace the initial value of the counter. Check this code snippet.
好! 让我们现在测试组件。 我们可以模拟useStore
挂钩,以始终使用Jest返回特定的商店。 而且,我们可以使用几行额外的代码来保证类型安全。 对于每个测试,我们都希望钩子返回新的存储。 但是,对于其中一项测试,我们将对其进行一些更改并替换计数器的初始值。 检查此代码段。
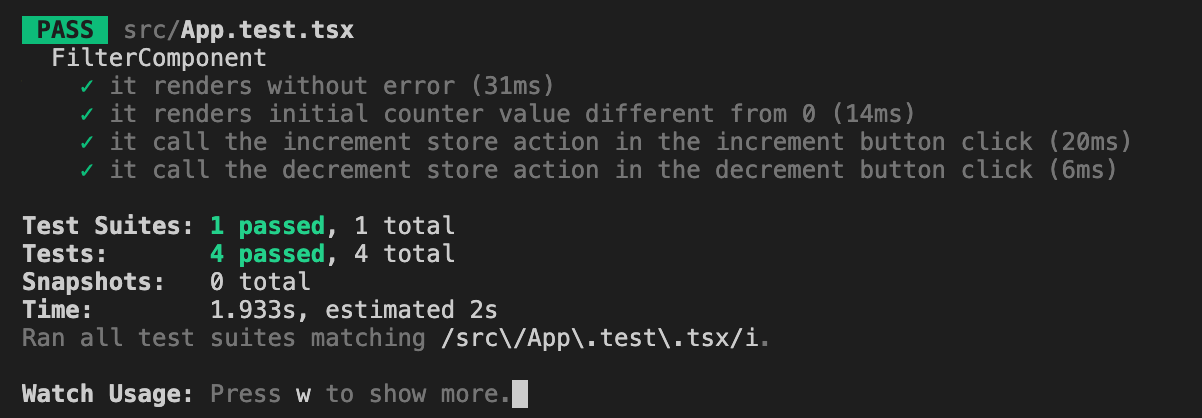
Quite simple, isn’t it? Yep! And we got a green light as well!
很简单,不是吗? 是的我们也获得了绿灯!
概要 (Summary)
Mobx-react-lite is actually all I need in my personal projects. Would this be your choice of favor as well? Let me know in the comments below 😉.
Mobx-react-lite实际上是我个人项目中所需要的。 您也会选择这吗? 在下面的评论中让我知道。
The entire project is available on the GitHub link.
感谢您阅读本主题! (Thanks for reading this topic!)
翻译自: https://medium.com/javascript-in-plain-english/all-you-need-is-mobx-react-lite-47ba0e95e9c8