java 读取 文本块
Java基础 (Java Basics)
Java, compared to other programming languages, is a bit verbose. A lot of people and companies like using Java, for their production environment, because of available support and skill. Still, it is one of the older programming languages, Java increased its pace in the last few years. Beyond than improving performance, it also added features that you generally don’t relate to Java, like lambdas. With such new features, it is trying to make a programmer’s job easier as well as to have less verbose code. One such attempt is to introduce “text blocks”, launched as a preview feature in JDK 13 and 14. Planned to come out of preview in JDK 15, that will be released in Sep’20, with OpenJDK release in Mar’21. Nonetheless, if you are using JDK 13 or 14, you can still use this slick feature.
与其他编程语言相比,Java有点冗长。 由于可用的支持和技能,很多人和公司喜欢在其生产环境中使用Java。 尽管如此,它仍然是较旧的编程语言之一,但Java在最近几年中加快了步伐。 除了提高性能外,它还添加了通常与Java不相关的功能,例如lambda。 有了这样的新功能,它试图使程序员的工作更轻松,同时减少冗长的代码。 一种这样的尝试是引入“文本块”,该文本块在JDK 13和14中作为预览功能启动。计划在JDK 15中退出预览,该预览将在9月20日发布,OpenJDK在3月21日发布。 但是,如果您使用的是JDK 13或14,则仍可以使用此功能。
多行字符串 (Multi-line strings)
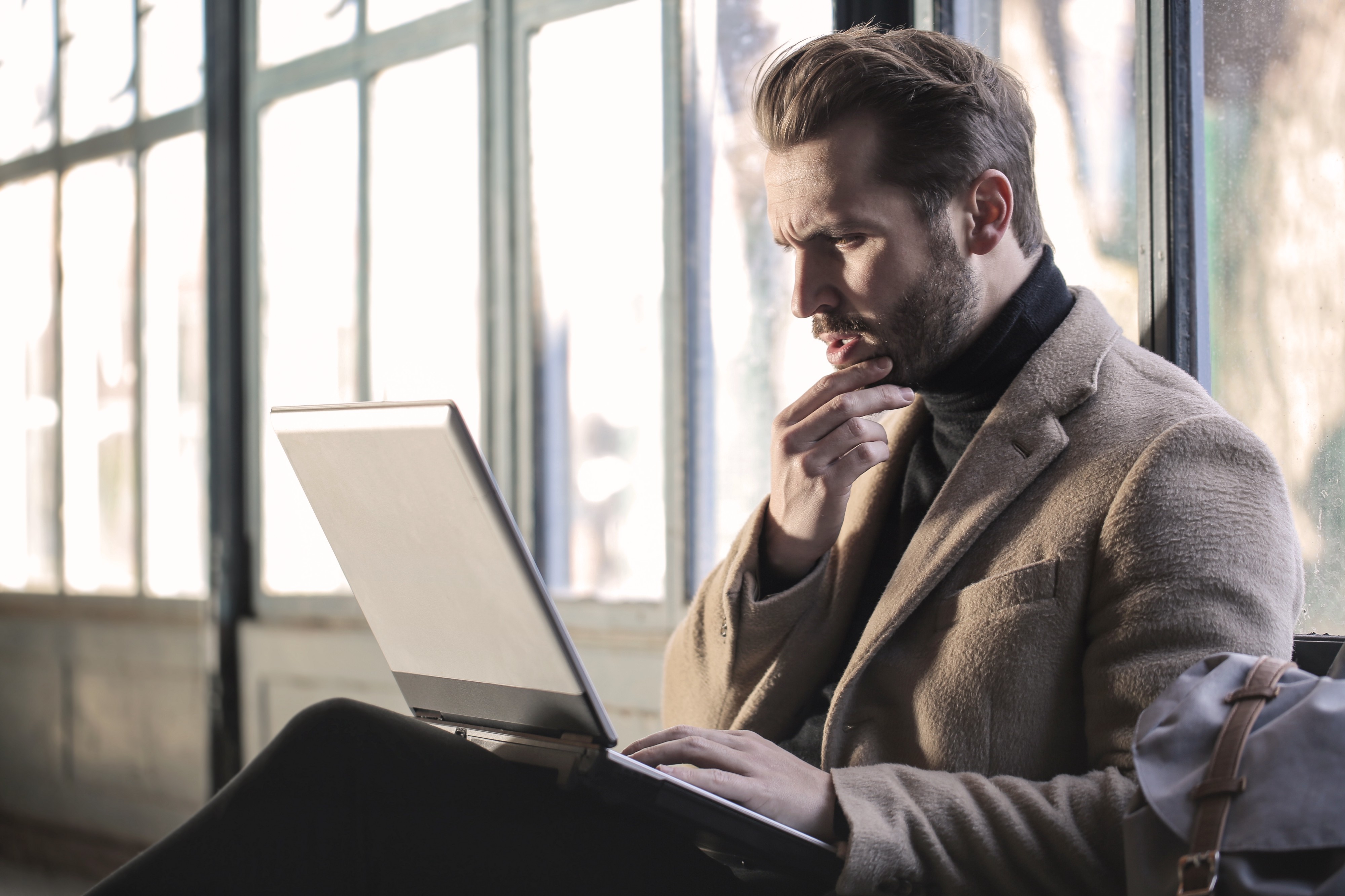
A multi-line string is a block of text, that spans across multiple lines. To create this, you need to add line separators to the text, so that the compiler understands how to render that string.
多行字符串是一个文本块,跨多行。 为此,您需要在文本中添加行分隔符,以便编译器了解如何呈现该字符串。
String myMultiLineText = "This is first line of my multi-line string.\nI'm on second line now";
This will produce the following output:
这将产生以下输出:
This is first line of my multi-line string.
I'm on second line now!
Here, by adding “\n”, I’m adding a new line to the string. But, actually, the line separator is dependent on the operating system. So, if you are using Windows/Mac OS 9 or before, you might have to use “\r” or “\r\n” as the escape sequence.
在这里,通过添加“ \ n”,我在字符串中添加了新行。 但是,实际上,行分隔符取决于操作系统。 因此,如果您使用的是Windows / Mac OS 9或更低版本,则可能必须使用“ \ r”或“ \ r \ n”作为转义序列。
Windows: '\r\n'
Mac (OS 9-): '\r'
Mac (OS 10+): '\n'
Unix/Linux: '\n'
Now, to make my code more generic, I have to account for such cases. Java has a way to get the line separator from system properties. Here is the updated code-
现在,为了使我的代码更通用,我不得不考虑这种情况。 Java有一种从系统属性获取行分隔符的方法。 这是更新的代码-
String newLineChar = System.getProperty("line.separator");
String myMultiLineText = "This is first line of my multi-line string."+newLineChar+"I'm on second line now";
Ok, very quickly, my simple code became more verbose and looks cluttered. Imagine the multi-line string with more (>5) lines! Not so easy on the eyes, right. We can use String methods like concat, join or use libraries like StringBuilder, Guava Joiner to make it a bit cleaner.
好的,很快,我的简单代码变得更加冗长,显得混乱。 想象一下多行字符串中有更多(> 5)行! 对眼睛不太容易,对。 我们可以使用诸如concat的String方法,join或使用诸如StringBuilder,Guava Joiner之类的库来使它更简洁。
使用字符串concat / join (Using String concat/join)
String myMultiLineTextUsingConcat = "first line"
.concat(newLineChar)
.concat("second line");String myMultiLineTextUsingJoin = String.join(newLineChar, "first line", "second line");
使用StringBuilder (Using StringBuilder)
StringBuilder myMultiLineStringBuilder = new StringBuilder()
.append("first line")
.append(newLineChar)
.append("second line")
.toString();
使用番石榴连接器 (Using Guava Joiner)
String myMultiLineTextUsingGuavaJoiner = Joiner.on(newLineChar)
.join(ImmutableList.of("first line", "second line"));
So, there are many ways to create such multi-line strings, where depending on the use case, one might be better than other. But, you can see that none of these, looks clean or easy to read, could lead to more error prone code.
因此,有很多方法可以创建这种多行字符串,根据使用情况,其中一种可能会比其他更好。 但是,您可以看到这些看上去干净或易于阅读的内容均不会导致易于出错的代码。
文字块 (Text Blocks)
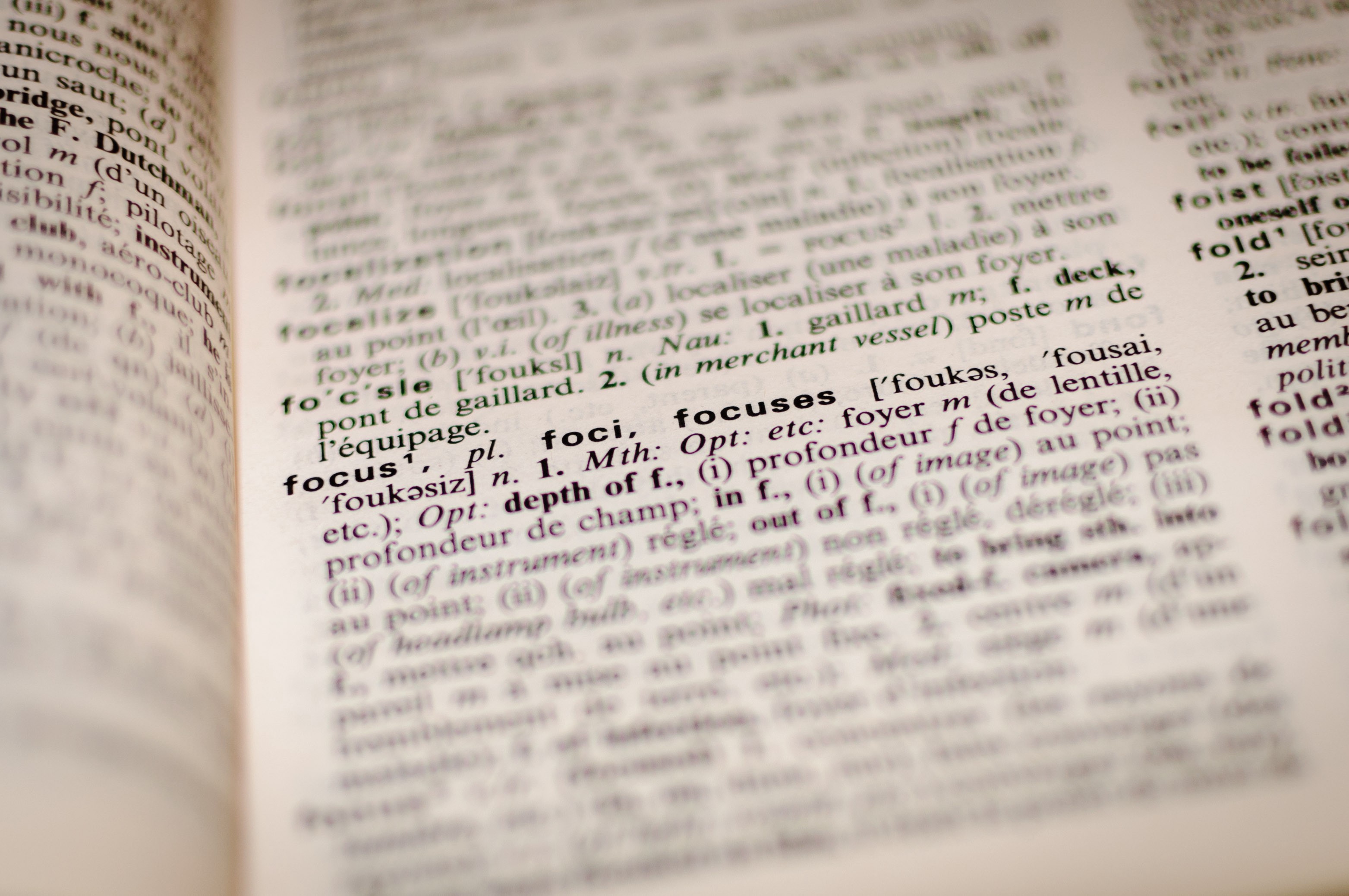
The text blocks feature allows you to create multi-line strings, in an intuitive way, the way it is supposed to be.
文本块功能使您可以以直观的方式创建多行字符串。
A text block is a multi-line string literal that avoids the need for most escape sequences, automatically formats the string in a predictable way, and gives the developer control over format when desired.
文本块是一种多行字符串文字,它避免了大多数转义序列的需要,以一种可预测的方式自动设置字符串的格式,并在需要时使开发人员可以控制格式。
Our multi-line string now becomes-
我们的多行字符串现在变为-
String myMultiLineText = """
first line
second line
""";
This makes it much simpler and is very easy to read and understand. You just need to enclose your string within delimiters, that is a sequence of three double quote characters ("""
).
这使其更简单,并且非常易于阅读和理解。 您只需要将字符串括在定界符 s中,即三个双引号字符( """
)的序列。
The content may include double quote characters directly, unlike the characters in a string literal. The use of \"
in a text block is permitted, but not necessary or recommended. Fat delimiters ("""
) were chosen so that "
characters could appear unescaped, and also to visually distinguish a text block from a string literal.
与字符串文字中的字符不同,内容可以直接包含双引号字符。 使用\"
在文本块是允许的,但不是必需的或推荐的。脂肪分隔符( """
)被选择为使得"
字符可能出现未逸出,并且还视觉上区分从一个字符串文字文本块。
At run time, a text block is evaluated to an instance of String
, just like a string literal. Instances of String
that are derived from text blocks are indistinguishable from instances derived from string literals.
在运行时,像字符串文字一样,将文本块求值为String
的实例。 从文本块派生的String
实例与从字符串文字派生的实例没有区别。
缩进 (Indentation)
Do note on the indentation, that I indented my string, so that I get desired spacing in my output. This produces the following output-
请注意缩进,即我使字符串缩进,以便在输出中获得所需的间距。 这将产生以下输出-
first line
second line
However, if I want to add a space to my second line. I can do that by-
但是,如果我想在第二行中添加一个空格。 我可以这样做-
String myMultiLineText = """
first line
second line
""";
Based on where the beginning delimited is started, compiler tries to understand the intent in the spacing, so that you can properly indent your code, as well as add spaces to your text block. Imagine you are creating HTML code in the string-
根据起始定界符的开始位置,编译器将尝试了解空格的含义,以便您可以适当地缩进代码,并在文本块中添加空格。 假设您要在字符串中创建HTML代码-
String html = """
<html>
<body>
<p>Hello, world</p>
</body>
</html>
""";
The output contains no extra spaces in the beginning and also your code is rightly indented.
输出的开头不包含多余的空格,并且您的代码也正确缩进。
<html>
<body>
<p>Hello, world</p>
</body>
</html>
Here is the HTML example using dots to visualize the spaces that are added for indentation:
这是使用点HTML示例,以可视化为缩进添加的空间:
String html = """
..............<html>
.............. <body>
.............. <p>Hello, world</p>
.............. </body>
..............</html>
..............""";
All these dots are removed during compile time processing, that the compiler assumes you added it for indenting your code.
在编译时处理过程中,所有这些点都将被删除,编译器假定您添加了这些点是为了缩进代码。
格式化 (Formatting)
Often, you would want to insert run time values into your string, like-
通常,您可能希望在字符串中插入运行时值,例如-
String welcomeText = String.format("Welcome, %s !", name);
You can still use String format method to format the text block-
您仍然可以使用String格式化方法来格式化文本块-
String welcomeText = String.format("""
Welcome, %s !
""", name);
To avoid such verbose constructs, there is a new method formatted that can help with this.
为了避免这种冗长的构造,有一种格式化的新方法可以帮助解决这个问题。
String welcomeText = """
Welcome, %s !
""".formatted(name);
Much better, right!
好多了,对!
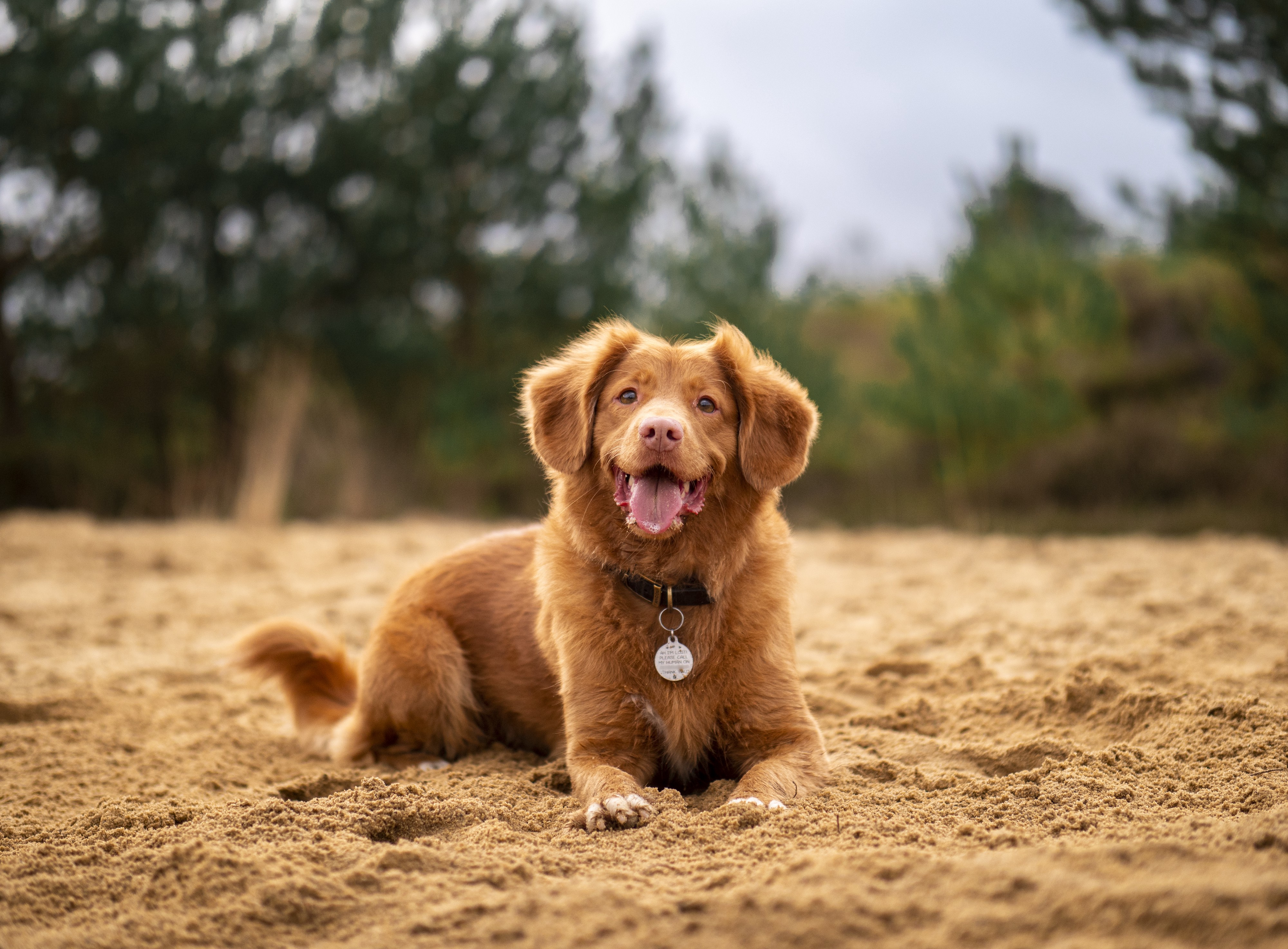
结论 (Conclusion)
Overall, text blocks is a nifty feature in Java, that provides lot of functionalities to create multi-line strings. Adding this in your code, definitely makes your code more readable and clean. Start experimenting and have fun!
总体而言,文本块是Java中的一个漂亮功能,它提供了创建多行字符串的许多功能。 将其添加到您的代码中,绝对可以使您的代码更具可读性和简洁性。 开始尝试并享受乐趣!
翻译自: https://levelup.gitconnected.com/new-kid-in-the-block-text-blocks-in-java-e8a273b34b4e
java 读取 文本块