monoid
For those interested in functional programming, I’ll talk about monoids and why they’re very important to understand ahead of time.
对于那些对函数式编程感兴趣的人,我将讨论monoid及其为何对提前了解非常重要。
Don’t get confused: This isn’t monad — it’s monoid. I’m pretty sure you already know of monoids and you use them almost every day — you just didn’t know the term for them.
不要感到困惑:这不是monad ,而是monoid。 我敢肯定,您已经知道monoid,并且几乎每天都在使用它们-您只是不知道它们的用语。
阅读之前 (Prior to Reading)
This is a series on functional programming, so you might not understand what this article is going to talk about if you haven’t read the previous posts.
这是一个有关函数式编程的系列文章,因此,如果您没有阅读以前的文章,那么您可能不明白本文将要讨论的内容。
您可以查看与此主题相关的其他帖子 (You can check out other posts related to this topic)
身份功能 (Identity Function)
Let’s assume there’s a function named identity
that takes A
and returns A
.
假设有一个名为identity
的函数,该函数采用A
并返回A
const identity: <A>(a: A): A => a;interface Student {
name: string;
age: number;
}identity<number>(3) // 3
identity<string>('hello') // hello
identity<Student>({
name: 'Bincent',
age: 5
}); // { name: 'Bincent', age: 5 }
In functional programming, this useless function (seems useless) is an important factor for many other concepts (such as monoids) that we’re about to talk about.
在函数式编程中,这个无用的函数(似乎无用)是我们要讨论的许多其他概念(例如monoid)的重要因素。
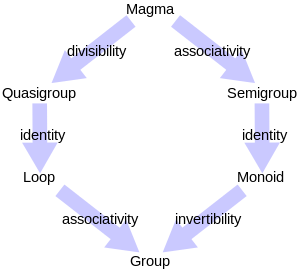
Basically, a monoid is a set of elements that holds the rules of the semigroup and the identity-element rule.
基本上,monoid是一组元素,其中包含半组规则和恒等元素规则。
If S
is a set of elements, a
is a member of S
, and ·
is a proper binary operation, a·e = e·a ∈ S
must be satisfied to be a monoid.
如果S
是一组元素, a
是S
的成员,并且·
是适当的二元运算, a·e = e·a ∈ S
必须满足a·e = e·a ∈ S
才能成为单半曲面。
Identity: a ∈ S, a·e = e·a = a ∈ S
Some documentation calls this using the number 1 and the any alphabet in subscript — for example, 1x referring to the identity on the variable x. Or some documentation uses just a single alphabet letter, such as i or e.
一些文档使用数字1和下标中的任何字母来称呼它,例如1x表示变量x的标识。 或某些文档仅使用单个字母,例如i或e。
That’s all there is to know about monoids, let’s practice with some simple examples.
这就是有关monoid的全部知识,让我们通过一些简单的示例进行练习。
Monoid示例 (Monoid Examples)
Here’s the class Monoid
that’d help you understand its concept.
这是类Monoid
,可以帮助您了解其概念。
This class has three methods:
此类具有三种方法:
concat
: Takes two arguments and returns the sum of the argumentsconcat
:接受两个参数并返回参数的总和identity
: Takes one argument and returns the argument without doing anythingidentity
:接受一个参数,不做任何事情就返回该参数pick
: Takes one argument that refers to the count value for the number of elements to pick up from the set — this is for just picking up the random elementspick
:采用一个参数,该参数引用要从集合中拾取的元素数量的计数值-这是为了拾取随机元素
And two assertion functions:
还有两个断言函数:
assertNumber
: Checks if the argument is a number type. If not, it throws an error.assertNumber
:检查参数是否为数字类型。 如果不是,则抛出错误。assertTrue
: Checks if the argument is aboolean
type andtrue
. If not, it throws an error.assertTrue
:检查参数是否为boolean
型且为true
。 如果不是,则抛出错误。
const set = new Monoid([1, 2, 3, 4, 5]);
I defined a set that consists of numbers only, so the set set
is a set of numbers (even though I put only five numbers, just assume it has numerous numbers).
我定义了一个仅由数字组成的集合,因此该集合set
是一组数字(即使我只输入了五个数字,也假设它有许多数字)。
问题1:是否满足加法操作中岩浆的规则? (Question 1: Does this satisfy the rule for magma on the adding operation?)
const [a, b] = set.pick(2);
assertNumber(a);
assertNumber(b);
assertNumber(a + b);
Yes. With two random numbers from the set, each element is a number type, and adding them is also a number type.
是。 集合中有两个随机数,每个元素都是数字类型,将它们相加也是数字类型。
问题2:这是否满足加法运算中半分组的规则? (Question 2: Does this satisfy the rule for a semigroup on the adding operation?)
const [x, y, z] = set.pick(3);
assertNumber(set.concat(set.concat(x, y), z));
assertNumber(set.concat(x, set.concat(y, z)));
assertTrue(
set.concat(set.concat(x, y), z) ===
set.concat(x, set.concat(y, z))
);
Also, yes. With three random numbers from the set, (x+y)+z
and x+(y+z)
are a number type, and they’re giving you the same result — the order of operations doesn’t matter (associativity).
还可以 在集合中有三个随机数时, (x+y)+z
和x+(y+z)
是数字类型,它们给出的结果相同-操作顺序无关紧要(关联性)。
问题3:这是否满足加法运算中monoid的规则? (Question 3: Does this satisfy the rule for monoids on the adding operation?)
const [r] = set.pick(1);
assertNumber(r);
assertNumber(set.identity(r));
assertTrue(set.identity(r) === r);
Yes! Whatever the r
from picking a random number was, identity
returns the argument doing nothing. Well, it adds zero to the argument, but it doesn’t change the argument at all.
是! 无论从随机数中选择r
是什么, identity
返回不执行任何操作的参数。 好吧,它为参数添加了零,但根本没有改变参数。
结果是…… (The result is that …)
The set set
is a monoid.
集合set
是一个monoid。
下一步是什么? (What’s Next?)
Understanding what magmas, semigroups, and monoids are isn’t the top most important thing to study or learn in functional programming, but if you know these concepts ahead of time, it’ll help you understand the other theories as well, such as category theory.
理解什么是岩浆,半群和半群半人并不是在函数式编程中学习或学习的最重要的事情,但是如果您提前了解这些概念,也可以帮助您理解其他理论,例如类别理论。
The next topic of this series might be the category theory and functors.
本系列的下一个主题可能是类别理论和函子。
翻译自: https://medium.com/better-programming/functional-programming-series-2-what-is-a-monoid-75fb23af4319
monoid