array.slice
Previously, we have implemented Array methods:
P以前,我们已经实现了Array方法:
- map and sort 映射和排序
- filter and concat 过滤器和连接器
- reduceRight reduceRight
Here, we continue with the implementation. We will take on splice
and slice
.
在这里,我们继续执行。 我们将进行splice
和slice
。
splice
和slice
(splice
and slice
)
Splice
拼接
Removes elements from an array and, if necessary inserts new elements in their place and returns the deleted elements.
从数组中删除元素,并在必要时在其位置插入新元素并返回删除的元素。
(method) Array.splice(start: number, deleteCount?:number, ...items: number[]): number[]
start the zero-based location in the array from which to start removing elements.
启动阵列从其开始删除元素在基于零位置。
deleteCount the number of elements to remove.
deleteCount要删除的元素数。
items elements to insert into the array in place of the deleted elements.
将要插入到数组中的items元素代替已删除的元素。
Let’s see examples.
让我们看一些例子。
We have an array:
我们有一个数组:
const arr = [56, 66, 99, 44]
If we want to splice the array from the second index.
如果要从第二个索引处拼接数组。
arr.splice(1)
splice will start from 66 and get the elements until it reaches element 44
the end of the array.
拼接将从66开始并获取元素,直到到达数组末尾的元素44
。
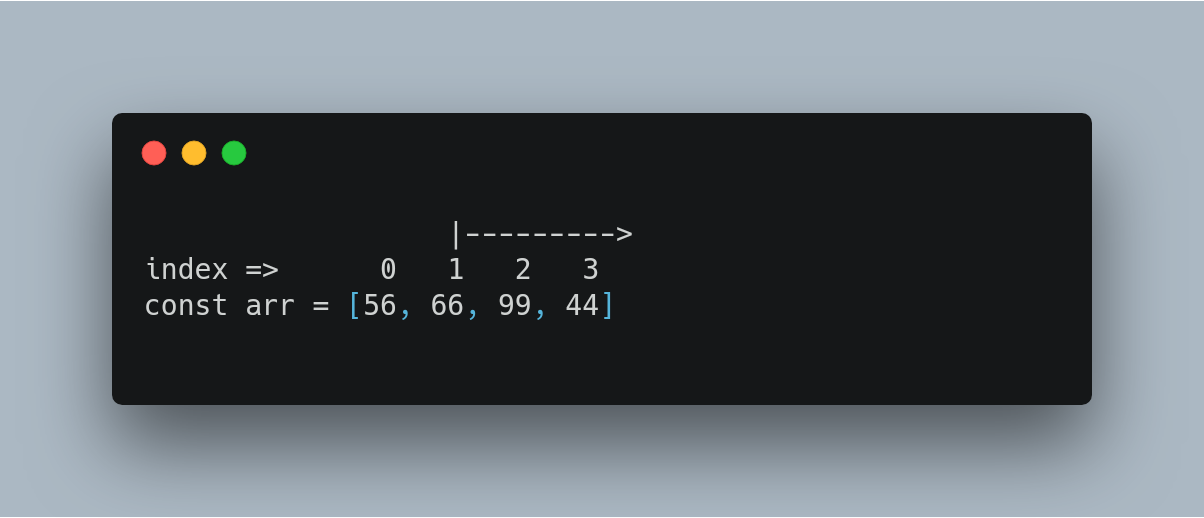
The elements will be returned:
元素将被返回:
[ 66, 99, 44 ]
Note : splice doesn’t get a section of the array. It cuts from the start index to the end of the array.
注意 :splice没有得到数组的一部分。 它从数组的起始索引切到结尾 。
Using the arr
, we can cut from the third index:
使用arr
,我们可以从第三个索引切入:
arr.splice(2)
Index 2 will start from element 99, so splice will start from element 99 to 44 and return them:
索引2从元素99开始,因此拼接将从元素99开始到44,并返回它们:
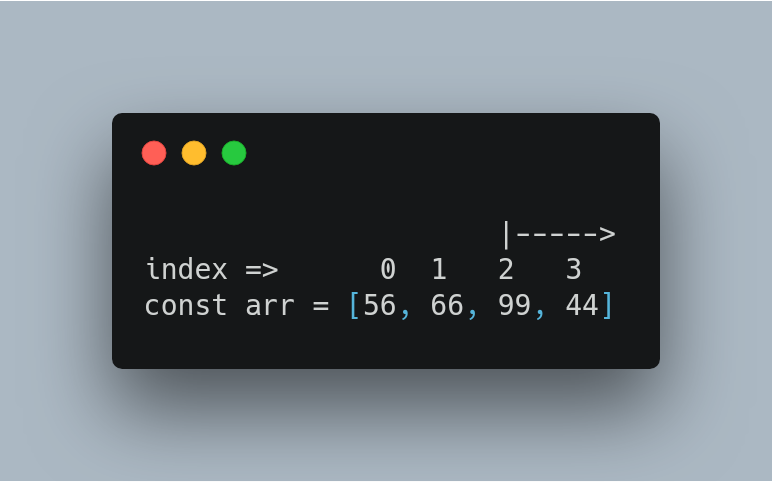
[ 99, 44 ]
Splicing from index 3 will start from 44 to the end of the array.
从索引3开始的拼接将从44开始到数组的末尾。
arr.splice(3)|----->
index => 0 1 2 3
const arr = [56, 66, 99, 44]
It will return:
它将返回:
[ 44 ]
What happens if we want to start from a negative index?
如果我们要从负索引开始怎么办?
Splice will reverse the order of index numbering.
接头将颠倒索引编号的顺序。
index starts from left to right, but passing a negative index will make splice start from the right to left.
索引从左到右开始,但是传递负索引将使接合从右到左开始。
Example:
例:
const arr = [56, 66, 99, 44]
l(arr.splice(-3))
We passed a negative index to splice -3
here.
我们在此处传递了一个负索引来拼接-3
。
splice will start from right down:
拼接将从右向下开始:
|----------->
index => -4 -3 -2 -1
const arr = [56, 66, 99, 44]
See the index doesn’t start from 0 but from -1
down.
看到索引不是从0开始,而是从-1
向下。
So, splice(-3) will start from element 66 to element 44:
因此,splice(-3)将从元素66开始到元素44:
[ 66, 99, 44 ]
Splice(-2) will be
接头(-2)将为
|----------->
index => -4 -3 -2 -1
const arr = [56, 66, 99, 44]
The result:
结果:
[ 99, 44 ]
Splice(-4) will be
接头(-4)将为
|--------------->
index => -4 -3 -2 -1
const arr = [56, 66, 99, 44]
It will start from element 56 to element 44:
它将从元素56开始到元素44:
[ 56, 66, 99, 44 ]
What happens if we pass start index that doesn’t exist?
如果我们通过不存在的起始索引会发生什么?
Example in our arr array:
我们的arr数组中的示例:
const arr = [56, 66, 99, 44]
And we want to slice from index 5. Now array index has no index 5. It only has index 0, 1, 2, 3.
我们要从索引5进行切片。现在数组索引没有索引5。它只有索引0、1、2、3。
Any reference after 3 will return undefined. When passed to splice, it will return the whole array.
3之后的任何引用将返回undefined。 当传递给splice时,它将返回整个数组。
arr.splice(5)
Result:
结果:
[ 56, 66, 99, 44 ]
Passing negatives indices will return the whole array too.
传递负数索引也将返回整个数组。
arr.splice(-5)
This is a negative index but not in range of arr negative indices it has.
这是一个负指标,但不在其负负指标范围内。
index => -4 -3 -2 -1
const arr = [56, 66, 99, 44]
The negative indices on arr start from -1,-2, -3, -4. There is no -5
arr的负索引从-1,-2,-3,-4开始。 没有-5
So, arr.splice(-5)
will return the whole array:
因此, arr.splice(-5)
将返回整个数组:
[ 56, 66, 99, 44 ]
Setting the number of elements to remove from the spliced array
设置要从拼接数组中删除的元素数
We will pass a second param to splice to set the number of elements to splice from an array.
我们将第二个参数传递给拼接,以设置要从数组中拼接的元素数量。
const arr = [56, 66, 99, 44]l(arr.splice(1, 2))
This will start from index 1 ie element 66, and cut two elements towards right:
这将从索引1开始,即元素66,并向右切两个元素:
|-->-|
index => 0 1 2 3
const arr = [56, 66, 99, 44]
The 2 we passed to splice made it splice two elements, if we had passed 3, it will cut three elements from index 1.
我们传递给splice的2使它拼接了两个元素,如果传递了3,它将从索引1切下三个元素。
The result:
结果:
[ 66, 99 ]
Can we insert elements inside the deleted elements?
我们可以在删除的元素内插入元素吗?
Yes, splice allows us to do just that by specifying a third optional argument. This third argument holds the elements to add in the place of the deleted elements.
是的,splice允许我们通过指定第三个可选参数来做到这一点。 第三个参数保存要添加的元素,以代替已删除的元素。
const arr = [56, 66, 99, 44]const spliceArr = arr.splice(1, 3, 0, 1)
This will cut the array from index 1 to index 3. The remaining argument in the splice call: 0, 1 will be added to the space deleted from the arr array.
这会将数组从索引1剪切到索引3。拼接调用中的其余参数:0,1将添加到从arr数组删除的空间中。
First, splice(1, 3) will make array arr
to be:
首先,splice(1,3)将使数组arr
为:
arr = [56]
Then 0 and 1 will be added to it.:
然后将0和1添加到其中:
arr = [56, 0, 1]
while the result spliceArr
will hold the result of splice(1,3)
而结果spliceArr
将保存splice(1,3)的结果
spliceArr = [66, 99, 44]
If we run it:
如果我们运行它:
const arr = [56, 66, 99, 44]const spliceArr = arr.splice(1, 3, 0, 1)
l("arr: ", arr)
l("spliceArr: ", spliceArr)
The result:
结果:
$ node array
arr: [ 56, 0, 1 ]
spliceArr: [ 66, 99, 44 ]
接头:可变性 (Splice: Mutability)
Is splice a mutating method or non-mutating? Let’s find out.
拼接是突变方法还是非突变方法? 让我们找出答案。
const arr = [56, 66, 99, 44]const spliceArr = arr.splice(2)
l("arr: " + arr, "spliceArr: " + spliceArr)
l(arr === spliceArr)
The result
结果
$ node array
arr: 56,66 spliceArr: 99,44
false
We see that arr has been mutated. It’s data structure/info/composition has been changed.
我们看到arr已被突变。 它的数据结构/信息/组成已更改。
splice affected the original array, arr. So we know that splice is a mutating method.
拼接影响了原始数组arr。 因此,我们知道剪接是一种变异方法。
重要的提示 (Important Note)
If we pass deleteCount to splice. It(splice) will cut off the specified deleteCount from the array and stuff back the remaining back to the original array.
如果我们将deleteCount传递给splice。 它(拼接)将从数组中删除指定的deleteCount,并将其余的填充回原始数组。
Example:
例:
const arr = [56, 66, 99, 44]const spliceArr = arr.splice(1,2)
This will cut the arr from element 66 to element 44, then will delete two elements from the extracted array.
这会将arr从元素66剪切到元素44,然后从提取的数组中删除两个元素。
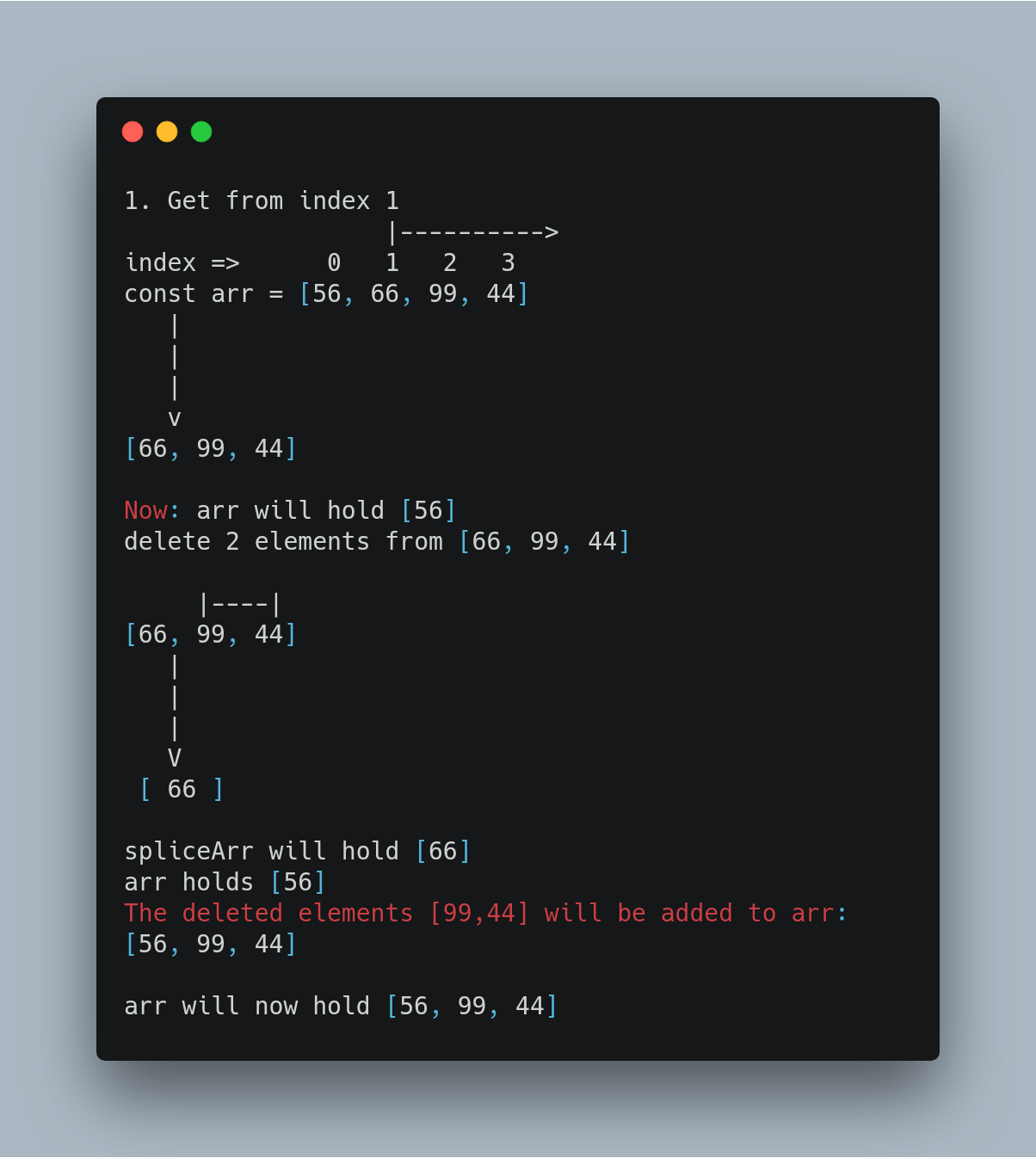
实施拼接 (Implementing splice)
Armed with what we have known of the splice method we implement our own:
有了已知的拼接方法,我们实现了自己的:
Let’s test our implementation:
让我们测试一下实现:
const arr = [56, 66, 99, 44]const spliceArr = arr.splice(1, 2, 0, 1)l(arr)
l(spliceArr)
Result:
结果:
$ node array
[ 56, 0, 1, 44 ]
[ 66, 99 ]
Same result with the original splice implementation. Also, it mutates the original array, here arr data structure was changed.
与原始接头实现相同的结果。 另外,它会更改原始数组,此处的arr数据结构已更改。
Another test:
另一个测试:
const arr = [56, 66, 99, 44]const spliceArr = arr.splice(1, 2)l(arr)
l(spliceArr)
Result:
结果:
$ node array
[ 56, 44 ]
[ 66, 99 ]
Slice returns a section of an array
Slice返回数组的一部分
(method) Array<number>.slice(start? : number, end?: number): number[]
start the beginning of the specified portion of the array.
从数组的指定部分开始。
end is the end of the specified portion of the array
end是数组指定部分的结尾
slice cuts from the index specified by start.
从start指定的索引处开始切片。
const brr = [56, 66, 99, 44]const marr = brr.slice(1)l(marr)
Result:
结果:
[ 66, 99, 44 ]
We sliced from index 1. So it will start from element 66, which is at index 1, to the end of the array at element 44.
我们从索引1进行切片。因此它将从索引1的元素66开始,到元素44的数组末尾。
|---------->
index => 0 1 2 3
const brr = [56, 66, 99, 44]
The elements [66, 99, 44] will be returned.
元素[66,99,44]将被返回。
If we specify an end index, slice will cut from the start index and stop at the index next to meet the end index.
如果我们指定了结束索引,则slice将从开始索引处剪切,并在下一个索引处停止,以符合结束索引。
const brr = [56, 66, 99, 44]const marr = brr.slice(1, 2)l(brr)
This will start from index 1, element 66 and stop at index 1. An index short of the end index, 2. The element at index 2 (99) won’t be included.
这将从索引1,元素66开始并在索引1处停止。索引比结束索引2短。索引2(99)处的元素将不包括在内。
So the result will be this:
因此结果将是这样的:
[ 66 ]
If we make the end index to be 3:
如果我们将结束索引设为3:
const brr = [56, 66, 99, 44]const marr = brr.slice(1, 3)
It will start at index 1 (66) and stop at index 2 (99).
它将从索引1(66)开始,然后从索引2(99)停止。
[ 66, 99 ]
Negative index
负指数
What happens when we pass a negative index?
当我们传递一个负索引时会发生什么?
slice will convert it to its positive index. How?
slice将其转换为其正索引。 怎么样?
slice counts postive index (from 0) from left to right. slice counts negative index from (starting from -1, -2) right to left:
slice从左到右计数阳性索引(从0开始)。 slice从(从-1,-2开始)从右到左计数负索引:
-ve index => -4 -3 -2 -1
+ve index => 0 1 2 3
const brr = [ 56, 66, 99, 44 ]
So if we do this:
因此,如果我们这样做:
const marr = brr.slice(-3)
slice will start from -ve index -3, which is 1 on the +ve index, and cut to the right.
slice将从-ve索引-3(在+ ve索引上为1)开始,并向右切割。
|----------->
-ve index => -4 -3 -2 -1
+ve index => 0 1 2 3
const brr = [ 56, 66, 99, 44 ][ 66, 99, 44 ]
Adding end index at the negative index:
在负索引处添加结束索引:
const brr = [56, 66, 99, 44]const marr = brr.slice(-3, 3)
slice will start from index 1 (converting negative index -3 to its +ve index relative) and stop at index 2:
slice将从索引1开始(将负索引-3转换为其相对的+ ve索引),并在索引2处停止:
|---|
-ve index => -4 -3 -2 -1
+ve index => 0 1 2 3
const brr = [ 56, 66, 99, 44 ][ 66, 99 ]
变异性 (Mutability)
Is slice a mutating or non-mutating method? Let’s find out:
切片是变异还是非变异方法? 让我们找出:
const brr = [56, 66, 99, 44]const marr = brr.slice(1, 3)const sliceArr = brr.slice(1, 3)l("brr:", brr)
l("sliceArr:", sliceArr)
l(brr === sliceArr)
Result:
结果:
brr: [ 56, 66, 99, 44 ]
sliceArr: [ 66, 99 ]
false
See the brr array from which we sliced wasn’t changed. It remained the same, unscathed ;)
看到我们切片的brr数组没有改变。 它保持不变,毫发无损;)
实作 (Implementation)
Test:
测试:
const brr = [56, 66, 99, 44]const sliceArr = brr.slice(-3)l("brr:", brr)
l("sliceArr:", sliceArr)
Result:
结果:
$ node array
brr: [ 56, 66, 99, 44 ]
sliceArr: [ 66, 99, 44 ]
Boom, the result is the same as the original slice method. Also, notice the original array, arr was not mutated.
动臂,结果与原始切片方法相同。 另外,请注意原始数组,arr没有被突变。
结论 (Conclusion)
I’ll say this is awesome.
我会说这很棒。
Remember, "What I do not understand, I cannot create."
记住,“我不了解,我无法创造。”
If you have comments, suggestions, corrections, or just wanna talk feel free to drop in the comments section or DM me or e-mail.
如果您有任何意见,建议,更正,或者只是想谈谈,请随时在“意见”部分或DM me或电子邮件中发表。
Thanks!!
谢谢!!
Thanks for stopping by my little corner of the web. I think you’ll love my email newsletter about programming advice, tutoring, tech, programming and software development. Just sign up below:
感谢您停在我的网络小角落。 我想您会喜欢我的电子邮件通讯,其中涉及编程建议,辅导,技术,编程和软件开发。 只需在下面注册:
Follow me on Twitter.
在 Twitter上 关注我 。
翻译自: https://medium.com/dev-proto/javascript-lets-implement-array-slice-and-array-splice-c97702806069
array.slice