react 组件引用组件
So, you want to take your React components to the next level? Implementing animations can greatly improve the customer experience and make your app feel more professional. Animations are one of many ways to accomplish this. In this article, I want to go through how you can easily animate your React components using framer-motion.
那么 ,您想将您的React组件提升到一个新的水平吗? 实施动画可以大大改善客户体验,并使您的应用程序更加专业。 动画是完成此操作的许多方法之一。 在本文中,我想介绍如何使用framer-motion轻松为React组件设置动画。
如何开始 (How to get started)
To set up your project to use framer-motion you can simply install it by using your JavaScript package managers like NPM or Yarn. Run any of the following commands inside of your React.js project and you will be ready to go.
要设置您的项目以使用framer-motion,您可以简单地通过使用JavaScript软件包管理器(例如NPM或Yarn)进行安装 。 在React.js项目中运行以下任何命令,您就可以开始使用。
$ npm install framer-motion$ yarn add framer-motion
不同类型的动画 (Different types of animations)
So, in framer-motion there a couple of different types of animations that you can use as tools to animate your components. Here are the ones we are going to cover in this article. You can also find much more information on the official framer-motion documentation site here.
因此,在成帧器运动中,可以使用几种不同类型的动画作为对组件进行动画处理的工具。 这是我们将在本文中介绍的内容。 您还可以找到的官方成帧器运动文档的网站更多的信息在这里 。
- Animating a single component 动画单个组件
- Animating component mounts and unmounts 动画化组件的安装和卸载
- Animating between component states 在组件状态之间进行动画处理
- Animating multiple components 动画化多个组件
动画单个组件 (Animating a single component)
So a good place to start trying out animation in React is to simply animate a single component. To do this, we will use the motion component provided by the framer-motion package. This lets us replace our default html divs with motion.div, and therefor get exposed the possibility to easily animate them. To animate a component in a x-axis, we can write the following.
因此,开始在React中尝试动画的一个好地方是简单地为单个组件设置动画。 为此,我们将使用framer-motion包提供的运动组件。 这使我们可以将默认的html div替换为motion.div,从而可以轻松实现动画设置。 要为x轴上的组件设置动画,我们可以编写以下内容。
import React from 'react'
import { motion }from 'framer-motion'const MyComponent = ({ children }) => (
<motion.div animate={{ x: 500 }}>
{children}
</motion.div>
)
And by using our component like this:
并通过使用这样的组件:
import MyComponent from "../components/component";export default function App() {
return (
<MyComponent>
<h3>This will animate</h3>
</MyComponent>
);
}
The output will look like this and it will animate directly when it mounts.
输出将看起来像这样,并且在安装时将直接进行动画处理。
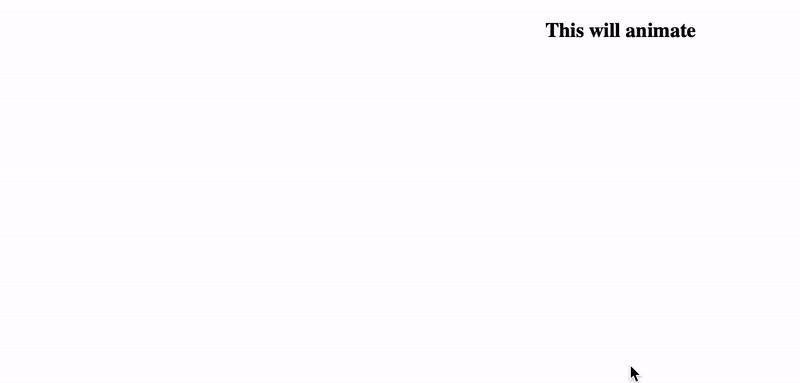
Although this might not seem like a very useful animation, this is just the basic concept of animating a React component. Now we will use our learnings to further develop our React components to use more complex animations to animate between states and mounts. Starting off, let us animate a component between being visible and not visible.
尽管这看起来可能不是非常有用的动画,但这只是使React组件动画化的基本概念。 现在,我们将使用我们的知识来进一步开发React组件,以使用更复杂的动画在状态和坐骑之间建立动画。 首先,让我们为可见和不可见之间的组件设置动画。
动画存在 (Animating presence)
Animating presence can be done in a couple of different ways. We will do this by just slightly updating our previous component, and in our parent component where we will add the AnimatePresence component provided by framer-motion to animate between mounting and unmounting from the dom. This most accurately represents how you most likely use your React components today. We will update our component to look like this:
可以通过几种不同的方式来对存在进行动画处理。 为此,我们将稍稍更新以前的组件,并在父组件中添加framer-motion提供的AnimatePresence组件,以在dom的安装和卸载之间进行动画处理。 这最准确地代表了您今天最有可能使用React组件的方式。 我们将更新组件,使其看起来像这样:
import { motion, AnimatePresence } from "framer-motion";export default function MyComponent({ children }) {
return (
<motion.div initial={{ opacity: 0 }}
animate={{ x: 250, opacity: 1 }}
exit={{ opacity: 0, x: 500 }}
>
{children}
</motion.div>
);
}
By adding the initial prop, updating the animate, and adding the exit prop we will ensure that the AnimatePresence component knows exactly how to animate this component when it mounts and unmounts from the dom. If we do not supply proper values the animation may not work. Now, to animate our component when it mounts and unmounts from the dom, we need to update our parent component a little bit.
通过添加初始道具,更新动画以及添加退出道具,我们将确保AnimatePresence组件确切知道如何在从dom安装和卸载该组件时为其设置动画。 如果我们没有提供适当的值,则动画可能无法正常工作。 现在,要在从dom安装和卸载组件时为其制作动画,我们需要对父组件进行一些更新。
import { useState } from "react";
import { AnimatePresence } from "framer-motion";
import MyComponent from "../components/component";export default function App() {
const [isOpen, setIsOpen] = useState(); return (
<div>
<button onClick={() => setIsOpen(!isOpen)}>
Toggle component
</button> <AnimatePresence>
{isOpen && (
<MyComponent>
<h3>This will animate</h3>
</MyComponent>
)}
</AnimatePresence>
</div>
);
}
Now, this is what our component should look like. When clicking the button the first time, the component is going to animate onto the page, and when clicking it again it is going to animate out from the page. Since we specified our initial, animate, and exit props on our component, framer-motion now knows how to animate it between its different states.
现在,这就是我们的组件的外观。 第一次单击该按钮时,组件将在页面上制作动画,再次单击该组件时,它将从页面上制作动画。 由于我们在组件上指定了初始,动画和退出道具,因此制框运动现在知道如何在其不同状态之间进行动画处理。
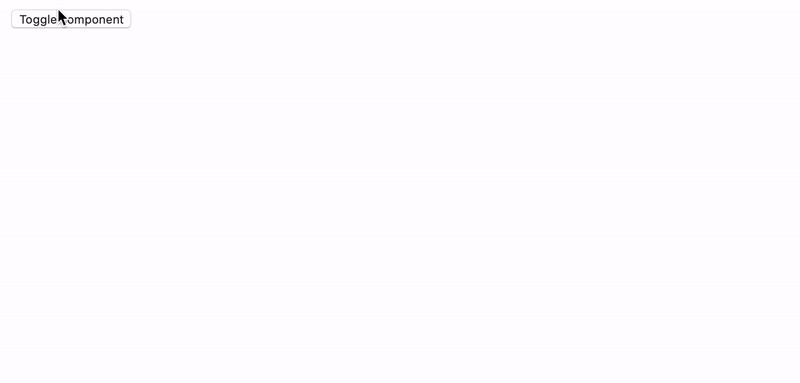
在组件状态之间进行动画处理 (Animating between component states)
Now, let us say your component itself has different local states — and when it changes you want your element to animate. We can accomplish this by using the motion element together with the layout property. We will try this out by creating a fairly usual but important component — a Switch.
现在,让我们说您的组件本身具有不同的局部状态-并且当它更改时,您希望元素进行动画处理。 我们可以通过将motion元素与layout属性一起使用来实现此目的。 我们将通过创建一个相当普通但重要的组件(一个Switch)来进行尝试。
import { motion } from "framer-motion";export default function Switch({ isOn, onToggle }) {
const styles = {
wrapper: {
display: "flex",
justifyContent: isOn ? "flex-end" : "flex-start",
borderRadius: 50,
width: 60,
padding: 4,
},
handle: {
height: 25,
width: 25,
background: "#fff",
borderRadius: "50%",
},
};return (
<motion.div
style={styles.wrapper}
onClick={() => onToggle(!isOn)}
animate={{ background: isOn ? "#006aff" : "#ddd" }}
>
<motion.div layout style={styles.handle} /> </motion.div>
);
}
And by using it like this in our parent component:
并在我们的父组件中像这样使用它:
import { useState } from "react";
import Switch from "../components/switch";export default function App() {
const [isOn, setIsOn] = useState(); return <Switch isOn={isOn} onToggle={() => setIsOn(!isOn)} />;
}
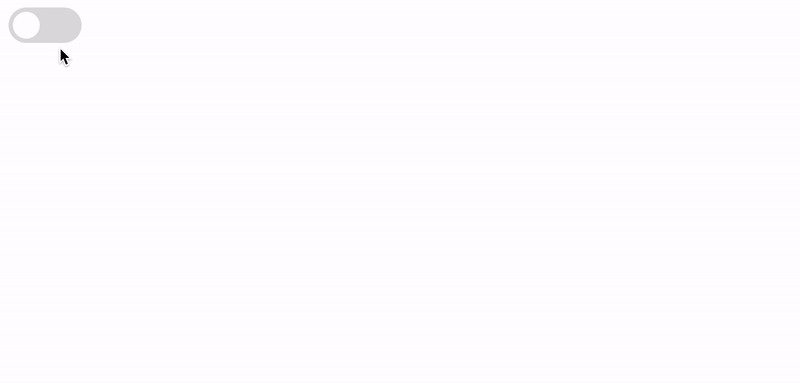
动画化多个组件 (Animating multiple components)
So, with our newly gathered knowledge on how to easily animate React components, we will now bring it all together to animate a more real world example with multiple components. Framer-motion certainly has a lot more features than we are going to cover in this article — but these examples will definitely help you kickstart your journey towards animating React components.
因此,利用我们新收集的有关如何轻松对React组件进行动画处理的知识,我们现在将所有这些知识整合在一起,以对具有多个组件的更真实的示例进行动画处理。 Framer-motion当然具有比我们在本文中介绍的功能更多的功能-但是这些示例无疑将帮助您启动动画React组件的旅程。
示例:动画通知 (Example: Animating notifications)
We will create a new component in our project called Notification, and from there we will start combining what we have learned to animate several notifications in a layout in a professional manner.
我们将在我们的项目中创建一个名为Notification的新组件,从那里我们将开始结合所学知识,以一种专业的方式在布局中为多个通知设置动画。
Here is the code for the new component. It is going to use the motion element with the initial, animate, and exit props to accomplish to the desired transition when mounting and unmounting from the dom.
这是新组件的代码。 在从dom安装和卸载时,将使用带有初始,动画和退出道具的运动元素来完成所需的过渡。
We are also going to apply the layout prop to make sure AnimatePresence knows that each component should be aware of its siblings to create smooth height adjustments to the wrapper.
我们还将应用布局道具,以确保AnimatePresence知道每个组件都应该知道其同级组件,以便为包装器创建平滑的高度调整。
import { motion } from "framer-motion";export default function Notification({ title, description, onClose, id }) {
const styles = {
wrapper: {
boxShadow: "0px 5px 30px 0px rgba(0,0,0,0.1)",
borderRadius: 5,
padding: 16,
margin: "1rem 0",
},
}; return (
<motion.div
layout
initial={{ opacity: 0, x: 200 }}
animate={{ opacity: 1, x: 0 }}
exit={{ opacity: 0, x: 200 }}
style={styles.wrapper}
>
<h2>{title}</h2>
<p>{description}</p>
<button onClick={() => onClose(id)}>Close</button>
</motion.div>
);
}
And to use the component we will add this to our parent component:
为了使用组件,我们将其添加到父组件中:
import { useState } from "react";
import { AnimatePresence } from "framer-motion";
import Notification from "../components/notification";export default function App() {
const [notifications, setNotifications] = useState([]); const pushNotification = (notification) => {
setNotifications([...notifications, notification]);
}; const styles = {
notifications: {
top: 0,
right: 0,
position: "fixed",
},
}; return (
<div>
<button
onClick={() =>
pushNotification({
title: "Test notification",
description:
"This is a test notification that is being animated using framer-motion",
id: Math.random(),
onClose: (id) => {
setNotifications((currentNotifications) =>
currentNotifications.filter(
(notification) => notification.id !== id
)
);
},
})
}
>
Push notification
</button> <div style={styles.notifications}>
<AnimatePresence>
{notifications.map((notification) => (
<Notification {...notification} key={notification.id} />
))}
</AnimatePresence>
</div>
</div>
);
}
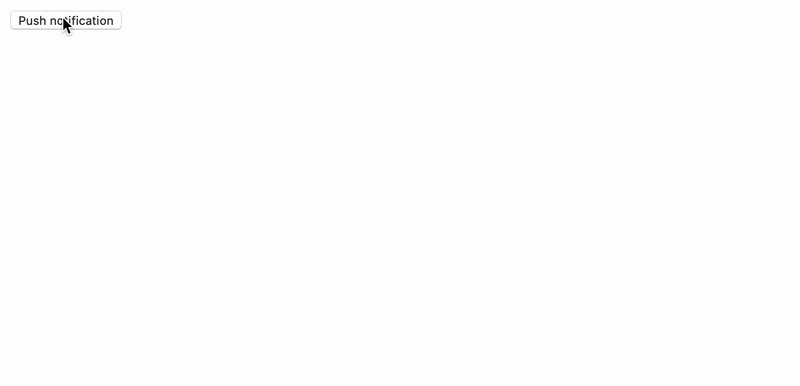
Voila! We have now built a way to animate notifications coming in on the page. This greatly enhances to experience for the user, compared to the notifications just appearing and disappearing from the page without any sort of transition. Just by supplying a few props and wrapping our components in a AnimatePresence we achieved this. This is really only scratching the surface of what animation libraries like framer-motion can do.
瞧! 现在,我们已经建立了一种使页面上出现的通知动起来的方法。 与仅在页面上显示和消失而没有任何过渡的通知相比,这极大地增强了用户的体验。 只需提供一些道具并将组件包装在AnimatePresence中,我们就可以实现这一目标。 这实际上只是在摸索像framer-motion这样的动画库可以做的事情。
接下来是什么? (What is next?)
Depending on your position in the industry, animations might not be the most important part of your product. For example, if you are bootstrapping a startup or building a very corporate software, animating every single React component might not be a very good return on investment (ROI). But if your work on a larger company where customer experience is greatly valued, or you are building your own startup and is really focused on design, animating your components can definitely enhance the customer experience and the general feel of your app.
根据您在行业中的位置,动画可能不是产品中最重要的部分。 例如,如果您要引导一家初创公司或构建一个非常公司化的软件,则为每个React组件设置动画可能不是一个很好的投资回报率(ROI)。 但是,如果您在一家重视客户体验的大型公司中工作,或者您正在建立自己的初创公司并且真正专注于设计,则对组件进行动画处理绝对可以增强客户体验和应用程序的整体感觉。
Animation really depends on the audience. If you are building an app for kids, then this is more or less a necessity. On the other hand — if you are building something for elderly people or for people with certain disabilities, you should consider reducing the amount of motion on your app. There are a lot of great resources on the web on how to manage this and make sure every user gets the most pleasant experience. Thank you for reading, and good luck with your journey of animating React components!
动画确实取决于观众。 如果您要为儿童构建应用程序,那么这或多或少是必要的。 另一方面,如果您要为老年人或某些残障人士建造东西,则应考虑减少应用程序的运动量。 Web上有很多有用的资源来帮助您管理并确保每个用户都获得最愉快的体验。 感谢您的阅读,并祝您在为React组件添加动画的过程中一切顺利!
翻译自: https://levelup.gitconnected.com/animating-your-react-components-8af7615ea61f
react 组件引用组件