javascript面试
JavaScript has evolved so much over the years that it’s difficult to categorize. Today, I’m going to dive into whether JavaScript is Synchronous or Asynchronous and what workflow looks like under the hood. And of course, also give you the answer most asking questions in interviews. Let’s jump into the article.
多年来,JavaScript已发展如此之多,以至于很难进行分类。 今天,我将深入探讨JavaScript是同步的还是异步的,以及工作流程的外观。 当然,也可以在面试中给您答案最多的问题。 让我们跳到这篇文章。
JavaScript如何工作? (How Does JavaScript Work?)
Imagine getting this question during an interview or how about this explain the difference between Asynchronous and synchronous in JavaScript? Or maybe they ask you to explain the statement “JavaScript is a single-threaded language that can be non-blocking!’’ Don’t worry I’m going to help you with those questions in this article.
想象一下在面试中遇到这个问题,或者这如何解释JavaScript中异步和同步之间的区别? 或者,也许他们要求您解释“ JavaScript是一种可以无阻塞的单线程语言!”的说法。不要担心,我将在本文中为您解决这些问题。
We don’t need to know How JavaScript works internally to write a program. But NO! It is important to learn. I see a lot of people who have been developers for years and not know this, it’s like being a pilot and not knowing How an Airplane can fly! okay, that’s a little bit dramatic there but you get my point so let’s start first.
我们不需要知道JavaScript在内部如何工作来编写程序。 但是不! 学习很重要。 我看到很多人已经从事开发工作多年,却一无所知,这就像是飞行员而不是飞机的飞行方式! 好的,那有点戏剧性,但是您明白我的意思,所以让我们先开始。
什么是程序? (What is a Program?)
Well, a program has to do some simple things:
好的,程序必须做一些简单的事情:
Allocate Memory — it has to allocate memory otherwise, we wouldn’t be able to have variables or even have a file on our computer.
分配内存 - 否则必须分配内存,否则我们将无法在计算机上拥有变量甚至文件。
Parse and Execute — it also has to parse and execute scripts which means read and run commands.
解析和执行 -它也必须解析和执行脚本,这意味着读取和运行命令。
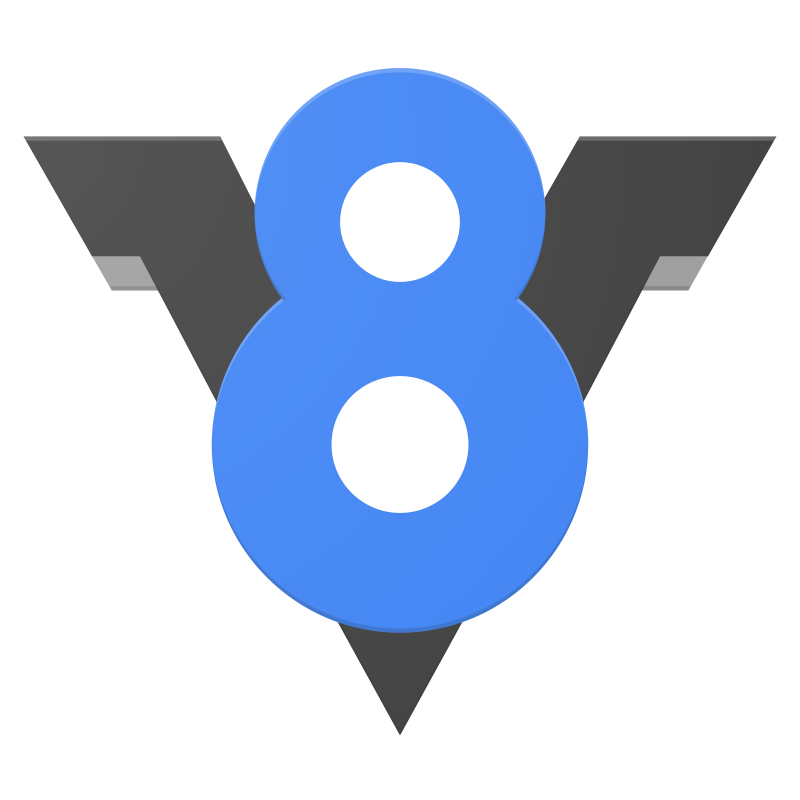
Now, We also know that there’s the JavaScript Engine each browser implements in chrome is the V8 and V8 Engine reads the JavaScript that we write in changes into machine-executable instructions for the browser now the engine consists of two parts a Memory Heap and a Call Stack.
现在,我们也知道 每个浏览器在chrome中实现的JavaScript引擎是V8,而V8引擎将我们在更改中编写JavaScript读取为浏览器的机器可执行指令,现在该引擎由内存堆和调用栈两部分组成。
Now the Memory Heap, This is where the memory allocation happens and then the Call Stack is where the code is read and executed it tells you where you are in the program so let’s simplify this and show you what I mean.
现在是Memory Heap ,这是发生内存分配的地方,然后Call Stack是读取和执行代码的地方,它告诉您程序中的位置,因此让我们简化一下并告诉我我的意思。
When declaring a variable, JavaScript will automatically allocate the memory for the variables.
声明变量时,JavaScript将自动为变量分配内存。
const a = 100; //allocate memory
const b = 10;
const c = 1; //allocate memory
We’ve just Allocated memory now when we assign this the javascript engine is going to remember that oh yeah a has a value of one hundred. We’ve just used up the Memory Heap and I can keep going, I can keep copying and pasting and changing these variables to B and C and I can change the values too. now that’s an issue that we see with this there’s something called a Memory Leak that you’re gonna hear as you become a developer and what we’ve done here is we’ve created all these variables Global Variables that are in the Memory Heap.
现在,当我们分配此代码时,javascript引擎将要记住,哦,a的值为100。 我们刚刚用完了内存堆 我可以继续,我可以继续复制和粘贴并将这些变量更改为B和C,也可以更改这些值。 现在这是我们看到的一个问题,当您成为开发人员时会听到一种称为“ 内存泄漏”的信息 ,而我们在这里所做的就是创建了所有这些变量 内存堆中的 全局变量 。
Imagine if I had just this page full of variables and instead of just numbers they’re like very big arrays well, memory leaks happen. When you have unused memory such as let’s say we’re not using the variable A but it’s Global well by having unused memory just laying around, it fills up this Memory Heap and that’s why you might hear why Global Variables are Bad.
想象一下,如果我只有这个充满变量的页面,而不仅仅是数字,它们就像很大的数组,那么就会发生内存泄漏。 当您有未使用的内存(例如,假设我们不使用变量A),而只是通过放置未使用的内存来实现全局变量时 ,它会填充此内存堆,这就是为什么您可能会听到为什么全局变量无效的原因。
Global variables are bad because if we don’t forget to clean up after ourselves we fill up this memory heap and eventually, the browser will not be able to work all right. So that’s Memory Allocation.
全局变量是不好的,因为如果我们不忘记自己清理后就填满了这个内存堆,最终浏览器将无法正常工作。 这就是内存分配。
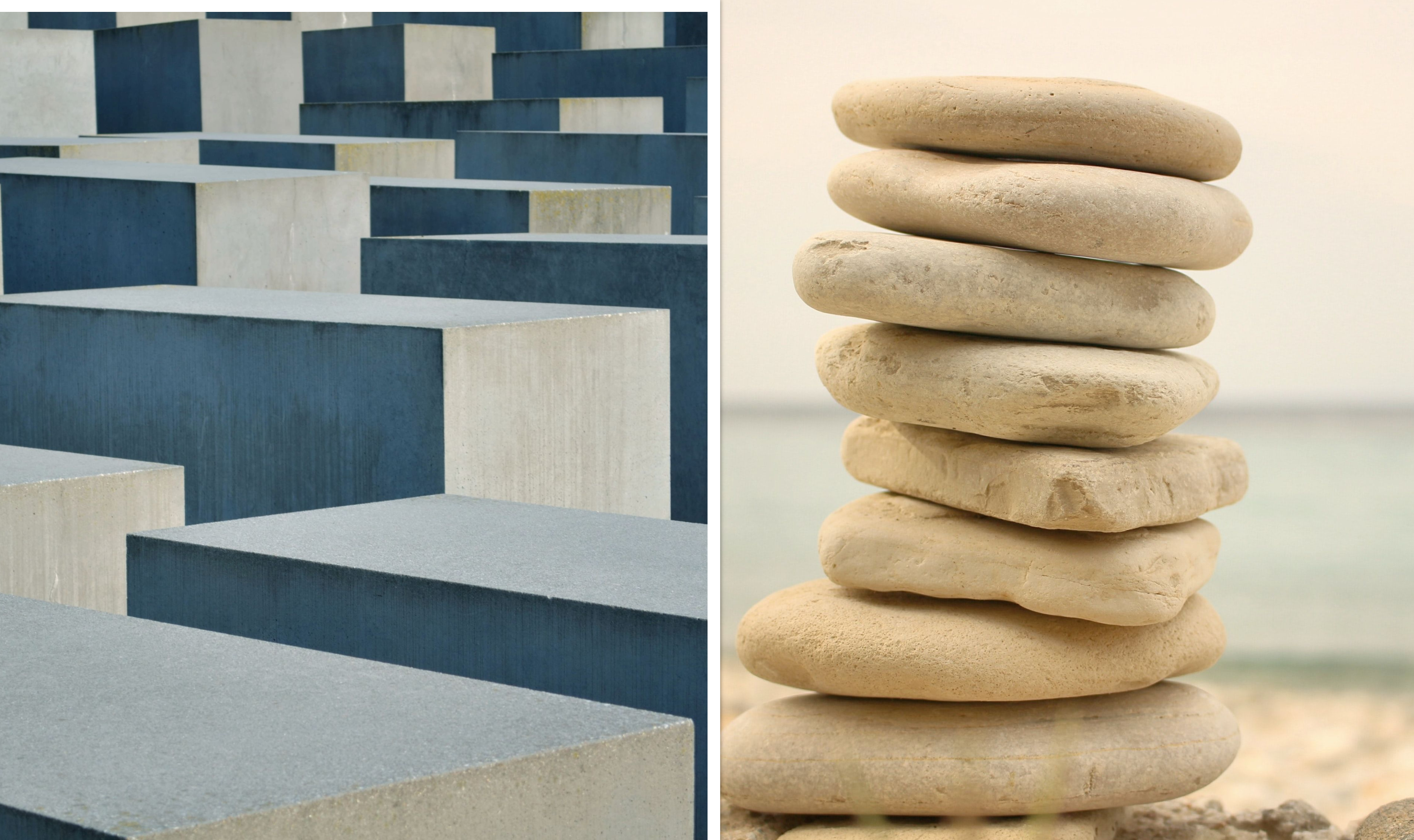
let’s talk about the Call Stack what is that well again with a call stack we can have something like this:
让我们谈谈呼叫堆栈 调用堆栈又有什么好处,我们可以得到以下内容:
//CALL STACK
console.log(“1”);
console.log(“2”);
console.log(“3”);
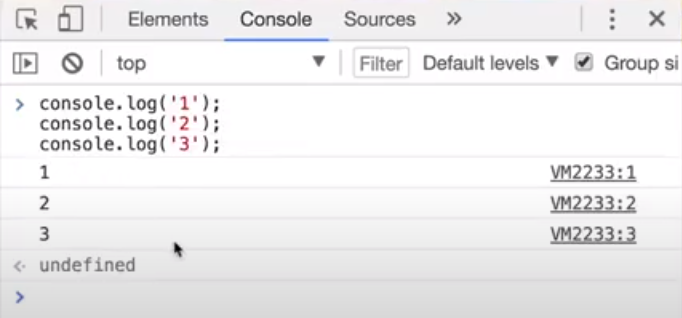
Console.log will go one, Console.log two, and then finally Console.log three. If I run this code in my browser terminal well I get one two three. If you remember that’s what the Call stack does? It reads and executes our Scripts.
Console.log将进入第一, Console.log将进入第二,然后Console.log将进入第三。 如果我在浏览器终端中很好地运行此代码,我将得到一二三。 如果您还记得那是调用堆栈的作用? 它读取并执行我们的脚本。
Let’s Run step by step:
让我们一步一步地运行:
- It reads the first line console.log it gets put in this call stack, and then it runs it and creates 1. 它读取第一行console.log,并将其放入此调用堆栈中,然后运行它并创建1。
- Then it says okay I’m removing the first console.log because I just finished running it. I’m gonna place the second console.log into my call stack. Adds it on here and says yes execute and then it removes that it and pops it. 然后它说好的,我要删除第一个console.log,因为我刚完成它的运行。 我将第二个console.log放入我的调用堆栈中。 在这里添加它,并说是执行,然后将其删除并弹出。
- Then gets console.log 3 and logs console log three and then finally removes. 然后获取console.log 3,并记录控制台日志3,最后将其删除。
同步JavaScript (Synchronous JavaScript)
JavaScript is a Single-Threaded Language that can be non-blocking.
JavaScript是一种单线程语言,可以是非阻塞的。
JavaScript is a Single-Threaded language that can be non-blocking. Single-threaded means that it has only one Call Stack and one call stack only do one thing at a time mean Synchronous and as you saw a call stack is First in Last Out so, whatever’s at the top of the call stack gets run first then below that, and below that, until the call stack is empty now other languages can have Multiple Call Stacks and those are called Multi-Thread.
JavaScript是一种单线程语言,可以是非阻塞的。 单线程意味着它只有一个调用栈,并且一个调用栈一次只能做一件事,这意味着是同步的,并且正如您所看到的那样,调用栈是“ 后进先出”,因此,调用栈顶部的所有内容都会先运行在此之下以及以下,直到调用栈为空,现在其他语言可以具有多个调用栈 ,这些被称为多线程。
You can also see how that might be beneficial to have multiple call stacks so that we don’t keep waiting around for stuff. Then Why was JavaScript designed to be Single-Threaded?
您还可以看到拥有多个调用堆栈可能有什么好处,这样我们就不必再等待其他东西了。 那为什么将JavaScript设计为单线程的呢?
Well, running code on a Single-Thread can be quite easy since you don’t have to deal with complicated scenarios that arise in a Multi-Threaded Environment.
嗯,在单线程上运行代码非常容易,因为您不必处理多线程环境中出现的复杂情况。
Now, you may have heard of the site Stack Overflow. If you’re a developer you use it on a daily basis and have you ever wondered what stack overflow means? Well, stack overflow is this:
现在,您可能已经听说过网站堆栈溢出。 如果您是开发人员,那么您每天都会使用它,您是否想过堆栈溢出意味着什么? 好吧,堆栈溢出是这样的:
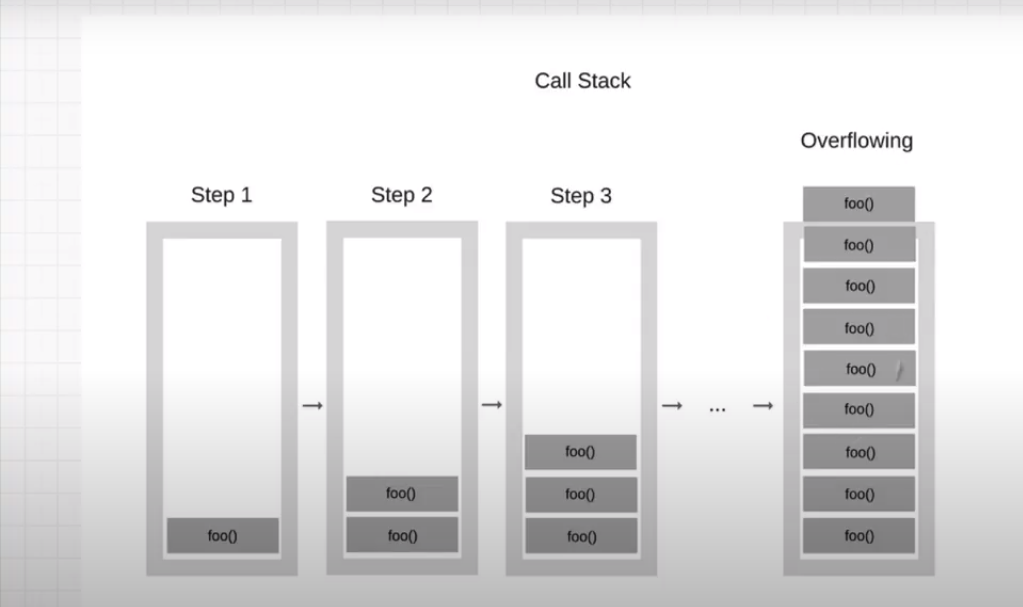
When a step is overflowing, kind of like we talked about Memory Leaks and How the Memory heap of a JavaScript engine can overflow! well with stack overflow this happens when the call stacks just get bigger and bigger and bigger until it just doesn’t have enough space anymore.
当一个步骤溢出时,就像我们在谈论内存泄漏以及JavaScript引擎的内存堆如何溢出一样! 随着堆栈溢出,当调用堆栈变得越来越大,直到不再有足够的空间时,会发生这种情况。
How can we do that? Can we recreate a stack overflow? yeah, I can show you quickly that to create a function foo as we have in here and this function will just have foo and we’re just gonna run our foo function alright that looks confusing but what is happening here? This is something called Recursion.
我们该怎么做? 我们可以重新创建堆栈溢出吗? 是的,我可以很快向您展示创建一个函数foo的方法,就像我们在此处创建的那样,这个函数将只有foo,而我们只是要运行我们的foo函数,看起来看起来很混乱,但是这里发生了什么? 这就是所谓的 递归。
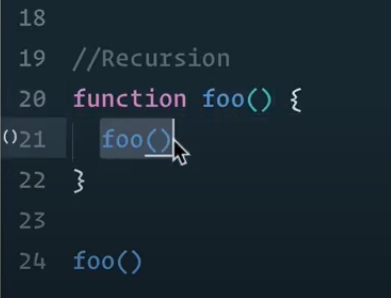
Recursion means a function that calls itself so if you look at what this function do we run foo and foo gets executed. What foo had does is? We run through again so it just keeps looping over and over again having a recursion but there’s no end in sight right? We keep adding foo to the call stack, we keep adding it over and over and so on…
递归表示一个调用自身的函数,因此,如果您查看此函数的功能,我们将运行foo并执行foo。 foo做的是什么? 我们再次进行遍历,因此它只是不断地循环遍历,但是递归没有,对吧? 我们不断将foo添加到调用堆栈中,不断地将其添加等等。
We have a stack overflow so if you want to have fun go into your browser go into your console and run something like this and see what happens you’re gonna get a stack overflow.
我们有一个堆栈溢出的问题,因此,如果您想玩得开心,请进入浏览器,进入控制台并运行类似的内容,然后看看会发生什么情况,从而导致堆栈溢出。
Alright so hopefully, this now makes sense the JavaScript Engine V8 and Chrome has a Memory Heap and a Call Stack.
希望如此,现在JavaScript引擎V8和Chrome具有内存堆和调用栈了 。
Now JavaScript is single-threaded only one statement is executed at a time but there is a problem, now isn’t it what if line 2 of console.log was a big task we needed to do maybe loop through an array that had millions of items. What would happen there?
现在JavaScript是单线程的,一次只能执行一个语句,但是有一个问题,如果console.log的第2行是一项我们需要做的大任务,也许不行,那可能遍历具有数百万个数组的数组项目。 那里会发生什么?
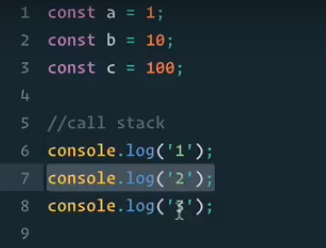
Well, with Synchronous Tasks if we have one function that takes up a lot of time it’s going to hold up the line.
好吧,对于“ 同步任务”,如果我们有一个函数需要占用大量时间,那么它将使这条生产线停滞不前。
例: (Example:)
Imagine a buffet restaurant, If all the people want to eat but Bobby says, hold on guys I have to keep eating and putting bacon on my plate well everybody has to wait in line so, sounds like we need something non-blocking. Remember our first statement that we made in this article? JavaScript is a Single-Threaded Language that can be non-blocking. Ideally, we don’t wait around for things that take time so how do we do this?
想象一下一个自助餐厅,如果所有人都想吃,但是鲍比说,坚持伙计们,我必须继续吃饭,把培根放在我的盘子上,每个人都必须排队等候,所以听起来我们需要无阻塞的东西。 还记得我们在本文中所做的第一句话吗? JavaScript是一种单线程语言,可以是非阻塞的。 理想情况下,我们不要等待耗时的事情,那么我们该如何做呢?
Well, Asynchronous to the rescue. We need something more than just Synchronous Tasks. You’re thinking to yourself, How do we do Asynchronous programming tasks?
好吧,与救援异步 。 我们需要的不仅仅是同步任务 。 您在想自己,我们如何执行异步编程任务?
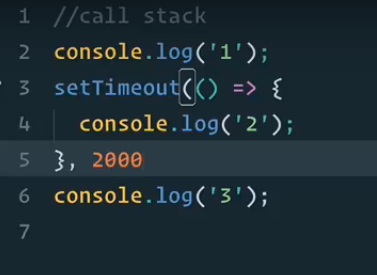
Well, we can do Asynchronous Programming by doing something like this, setTimeout which we’re going to talk about is a function that comes within our browsers and it allows us to create a Timeout and we can just give it the first parameter is the function that we want to run and then the second parameter is how many seconds we want to wait so, let’s say I want to wait two seconds so 200 minutes 2,000 milliseconds. If I do this now let’s run into the console and see what happens. Try yourself too to see what happens.
好吧,我们可以通过执行以下操作来进行异步编程 ,我们将要讨论的setTimeout是我们浏览器中附带的一个函数,它允许我们创建一个Timeout ,我们可以给它第一个参数是该函数我们要运行,然后第二个参数是我们要等待多少秒,比方说,我要等待2秒,所以需要200分钟2,000毫秒。 如果现在执行此操作,让我们进入控制台,看看会发生什么。 也尝试一下自己,看看会发生什么。
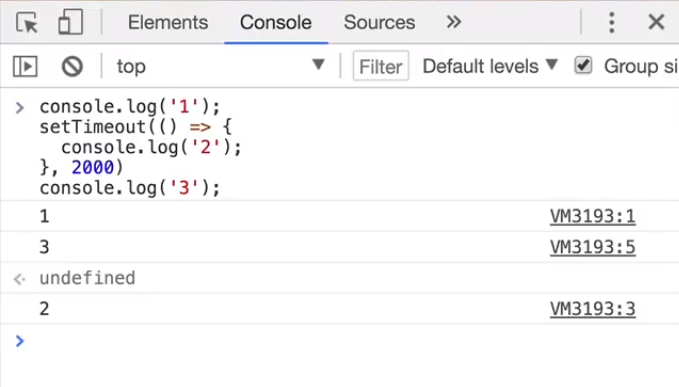
Well, what just happened? we have console.log one then console.log 3 and then console.log 2. After 2 seconds later it looks like we just skipped this whole step and then put this at the very end well you’ve just witnessed Asynchronous Programming.
好吧,刚才发生了什么? 我们有console.log一个,然后是console.log 3,然后是console.log2。两秒钟后,看来我们只是跳过了整个步骤,然后把它放到了最后,您已经亲眼目睹了异步编程。
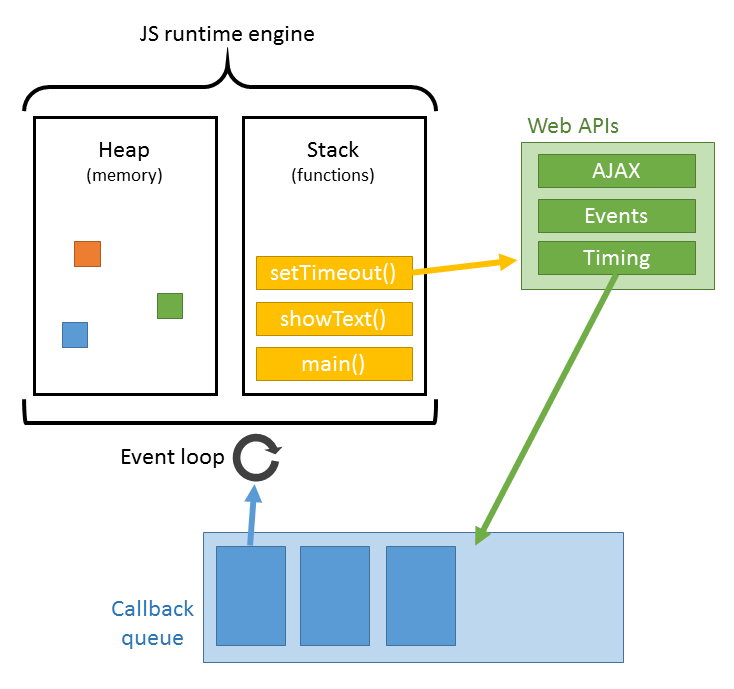
In order to understand this and what just happened, I need to take you to the next part and that is in order for JavaScript as we know it to run for the JavaScript engine with Memory heap and Call stack to run. We need more than just The JavaScript engine, we need what we call a JavaScript Runtime Environment.
为了理解这一点以及刚刚发生的事情,我需要带您进入下一部分,这是为了让JavaScript运行,因为我们知道它可以在具有内存堆和调用堆栈JavaScript引擎上运行。 我们不仅需要JavaScript引擎,还需要所谓的JavaScript运行时环境 。
The JavaScript Runtime Environment is part of the browser, it’s included in the browser’s. They have extra things, On top of the Engine, they have something called Web APIs, Callback Queue, and an Event Loop.
JavaScript运行时环境是浏览器的一部分,包含在浏览器中。 他们有一些额外的东西,除了引擎之外,还有一些叫做Web API,回调队列和事件循环的东西。
As you can see here, Set Timeout is part of the Web API it’s not technically part of JavaScript, is it? It’s what the browser’s give us to use so, we can do the Asynchronous programs.
如您在此处看到的, Set Timeout是Web API的一部分,从技术上讲,它不是JavaScript的一部分,对吗? 这是浏览器给我们使用的,因此,我们可以执行异步程序 。
Okay so looking at this diagram let’s see if we can figure out what our code was doing.
好了,所以看这张图,让我们看看是否可以弄清楚我们的代码在做什么。
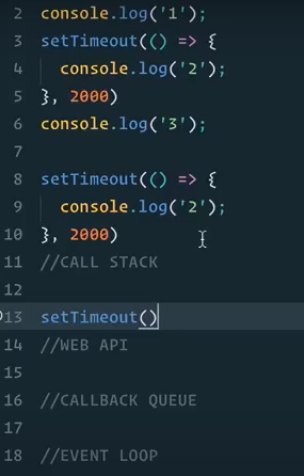
We can create here our own call stack, We’ll have Web API, then we’ll have a Callback queue and an Event loop. Just like we have in our JavaScript runtime environment so what’s happening here:
我们可以在这里创建自己的调用堆栈,使用Web API,然后使用回调队列和事件循环。 就像我们在JavaScript运行时环境中一样 ,这里发生了什么:
- Well first we have console.log that goes into the call stack and that gets run so we log console.log to the browser. 首先,我们有console.log进入调用堆栈并开始运行,因此我们将console.log登录到浏览器。
We then get Set Timeout into our call stack because we finish this first task we’re going to the second one, And what set timeout what’s gonna happen is? Well if the call stack is gonna say okay a half set timeout and because the set timeout is not part of JavaScript but part of the Web API it has this special characteristic what’s gonna happen is it triggers the Web API and says that Set Timeout has just been caught and because we notified a Web API, we can pop it out of the call stack.
然后,将Set Timeout放入调用堆栈,因为完成了第一个任务,我们将转到第二个任务,那么将会发生什么set timeout? 好吧,如果调用堆栈要说可以设置一半的超时时间,并且因为设置的超时时间不是JavaScript的一部分,而是Web API的一部分,则它具有此特殊特征,它将触发Web API并说Set Timeout仅被捕获,并且因为我们通知了Web API,我们可以将其弹出调用堆栈。
- Now the web API starts a timer here a timer of two seconds but it’s gonna know that in two seconds you have to do something and because the call stack is empty the JavaScript engine now goes to console.log 3. And executes this so that makes sense right now we’ve done 1 and 3 but we still have set timeout to seconds in the web API. 现在,Web API在此处启动了一个计时器,该计时器为2秒,但它会知道您必须在2秒钟内执行某些操作,并且由于调用堆栈为空,因此JavaScript引擎现在进入console.log 3。现在我们已经完成了1和3,但是我们仍然在Web API中将超时设置为秒。
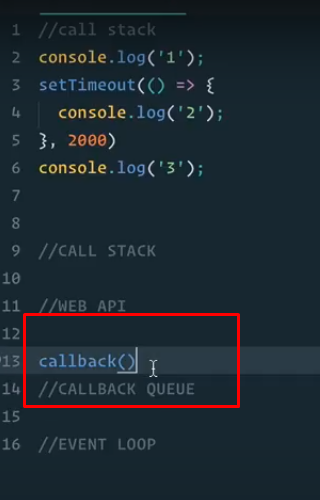
- Now after 2 seconds when our time limit is up, The Web API is gonna say ok SetTimeout should be run let’s see what’s inside of it well we have console.log, so what’s going to happen is?It’s going to say hey set timeout is done we have a callback and this callback of setTimeout we added to the callback queue saying that hey we have to run something we’re ready to Run It. 现在两秒钟之后,当我们的时间限制到了,Web API会说好的SetTimeout应该运行,让我们看看其中有什么内容,我们有了console.log,所以将要发生的事情是什么?完成一个回调,并将此setTimeout回调添加到回调队列中,说嘿,我们必须运行一些我们准备运行的东西。
The last part the Event Loop over here checks and says hey is the call stack empty and it keeps checking all the time. If the stack is empty and there’s nothing running right now on the JavaScript engine it’s going to say, Hey do we have any callbacks? it’s gonna check the callback queue and say, Hmm is anything in there because the call stack is empty we can throw something in there and make it do some work in our case we say, oh yeah I do let me put this into the call stack so now we move the callback into the call stack and then the callback we run it and by running we see that we have console.log.
事件循环的最后一部分检查并说嘿,调用堆栈为空,并且一直保持检查状态。 如果堆栈为空,并且JavaScript引擎上现在没有任何运行,这将说明,嘿,我们有任何回调吗? 它会检查回调队列并说:Hmm在里面,因为调用堆栈为空,我们可以在其中放一些东西,在我们说的情况下,它可以做一些工作,哦,是的,我让我将其放入调用堆栈因此,现在我们将回调移动到调用堆栈中,然后运行我们的回调,并通过运行我们看到有console.log。
So it’s going to say console.log, it’s going to run this function and once it’s done it’s going to pop it out of the call stack and again we’re done with the callback so we remove it and there you go, We’re done everything is empty and we’ve just run this Example, it’s gonna go through the entire Web API Callback Queue, Event loop.
所以要说console.log,它将运行此函数,完成后将其从调用堆栈中弹出,我们再次完成回调,因此我们将其删除,然后就可以了,一切都空了,我们只运行了这个示例,它将遍历整个Web API Callback Queue, Event loop 。
Wooh! There’s was a lot of information but hopefully, that makes sense to you of Why we noticed this behavior and I want to challenge your understanding here knowing what you know and what I just taught you what happens if I change this setTimeout to zero? that means zero seconds I’m going to give you a second to think about what will happen.? And Answer yourself.
喔! 有很多信息,但希望对您有意义,因为我们为什么注意到此行为,而我想挑战您的了解,因为如果将setTimeout更改为零,您将了解什么以及我刚刚教给您的信息。 这意味着零秒,我将给您一点时间来考虑会发生什么。 并回答你自己。
Now, In the end, If you’re able to understand this Article. You’ll actually have a lot of people that, Hire for JavaScript roles asking questions like this in an interview and you have to explain why that is? so, I hope that makes sense to you and you can use that to your advantage in the next interview.
现在,最后,如果您能够理解本文。 实际上,您会吸引很多人,在面试中聘请JavaScript角色询问类似问题,您必须解释为什么会这样吗? 因此,我希望这对您有意义,您可以在下一次面试中利用它来发挥自己的优势。
I’ll give you an example to understand it more better. Imagine calling your teacher with a question:
我会给你一个例子来更好地理解它。 想象给您的老师打个电话:
The Synchronous way is you call the teacher you wait on the phone until the Teacher answers the phone and ask him the question and hopefully get an answer so, You let the phone ring until he picks up but you’re not doing anything else in the meantime.
同步方式是您打电话给老师,您在电话上等待,直到老师接听电话并问他问题并希望得到答案,这样,您可以让电话响起直到他接听,但您在电话中没有做任何其他事情。与此同时。
The Asynchronous way means you send a text to a teacher with a question and then when the teacher he or she has the time will respond to you and call you with the answer so, You can do other stuff in between.
异步方式意味着您将有问题的文本发送给老师,然后在老师有空的时候给您答复,并给您答案,这样您就可以在中间进行其他操作。
So JavaScript is Asynchronous when you can leave it a message and a call back tells you, Hey Mr. the teacher has a message for you when you’re not too busy. And that’s why we call it a callback function in a callback queue we’re calling back to let them know that hey there’s some stuff waiting for you now we see over here.
因此,当您可以给JavaScript留下消息并回叫告诉您时, JavaScript是异步的 ,嘿,老师,当您不太忙时,会为您发送消息。 这就是为什么我们在回调队列中将其称为回调函数,以便让他们知道,现在我们在这里看到了一些东西在等着您。
让我们回顾一下: (Let’s recap:)
What we just learned? JavaScript is a single-threaded language that can be non-blocking. It has one Call Stack and it does one thing at a time.
我们刚刚学到了什么? JavaScript是一种单线程语言 ,可以是非阻塞的 。 它只有一个调用堆栈,并且一次只做一件事。
In order to not block the Single-thread, it can be Asynchronous with Callback Functions and these callback functions get to run in the background through the Callback queue and then the Event Loop to bring it back into the Call Stack. So, the next time you get asked about these topics, you should have a little bit more confidence to answer that question.
为了不阻塞单线程 ,它可以与回调函数 异步 ,并且这些回调函数可以通过回调队列在后台运行,然后通过事件循环在后台将其带回到调用堆栈中。 因此,下次您被问到这些主题时,您应该有更多的信心来回答这个问题。
I hope that this was helpful!
我希望这会有所帮助!
翻译自: https://medium.com/javascript-in-plain-english/hectic-javascript-interviews-questions-8fdf7ddd0e53
javascript面试