If you’ve been programming for any amount of time, you would be familiar with arrays. They are among the first data structures taught in most programming lectures/courses. For good reason too, because they are pretty easy to work with. But in case you work in JavaScript, using arrays can be made a whole lot simpler with the help of some useful higher-order methods!
如果你已经对编程的任何时间,你会熟悉数组。 它们是大多数编程讲授/课程中最早讲授的数据结构之一。 同样有充分的理由,因为它们很容易使用。 但是,如果您使用JavaScript工作,则可以借助一些有用的高阶方法使使用数组变得更加简单!
The reason that these are called Higher Order Methods is that they can accept/return another function. If this seems a tad bit confusing, then it’s important that you understand why functions are first-class citizens in JavaScript. It is just a fancy way of saying that functions are just like any other type of data, which can be stored, accessed, passed as arguments, and even returned from another method!
之所以将它们称为“ 高阶方法”,是因为它们可以接受/返回另一个函数。 如果这看起来有些混乱,那么重要的是要了解为什么函数是 JavaScript中的一等公民 。 这只是一种奇特的说法,即函数就像任何其他类型的数据一样,可以存储,访问,作为参数传递,甚至可以从其他方法返回!
The following image does a pretty good job at describing what a higher-order function is
下图在描述什么是高阶函数方面做得很好
A quick heads up. These higher-order methods will require the usage of callbacks and they will be a lot easier to write if you are familiar with the arrow syntax of ES6. In case you’re not, you can go through the following section to see what it is. Here’s a pretty basic example:
快速抬起头。 这些高阶方法将需要使用回调,并且如果您熟悉ES6的箭头语法 ,它们将更容易编写。 如果您不是,则可以阅读以下部分以了解它是什么。 这是一个非常基本的示例:
// normal function definition
function add(a, b) {
return (a + b)
}// arrow syntax
const add = (a, b) => (a + b);
You can convert a normal function definition into its arrow syntax counterpart, using the following steps:
您可以使用以下步骤将普通函数定义转换为其箭头语法副本:
Remove the
function
keyword and replace it with eitherconst
orlet
orvar
. We can do this because functions are first-class objects in JavaScript. (Note: In case you want an anonymous function, just remove thefunction
keyword and move to step 2)删除
function
关键字,并将其替换为const
或let
或var
。 因为函数是JavaScript中的一流对象,所以我们可以这样做。 ( 注意:如果您要使用匿名函数 ,只需删除function
关键字,然后转到步骤2)Next, put an arrow symbol
=>
in front of the arguments’ list, to indicate that the code following it will be the body of the function.接下来,在参数列表的前面放置一个箭头符号
=>
,以指示其后的代码将成为函数的主体。After this, you can type curly braces and write the function body as usual. But, if your function body has just 1 line (the return statement), you can skip the curly braces, skip the
return
keyword, and just type out the expression that needs to be returned!之后,您可以键入大括号并照常编写函数主体。 但是,如果您的函数体只有1行(return语句),则可以跳过花括号,跳过
return
关键字,然后键入需要返回的表达式!For functions with no argument, just leave empty brackets before the
=>
symbol.对于不带参数的函数,只需在
=>
符号前留空括号。For functions with no argument, just leave empty brackets before the
=>
symbol.const alertMsg = () => alert("This is just an example!")
对于不带参数的函数,只需在
=>
符号前留空括号。const alertMsg = () => alert("This is just an example!")
Lastly, if you are handling just 1 argument in the function, you can skip the parenthesis around it.
最后,如果您只处理该函数中的1个参数,则可以跳过括号。
Lastly, if you are handling just 1 argument in the function, you can skip the parenthesis around it.
const squared = x => (x ** 2)
最后,如果您只处理该函数中的1个参数,则可以跳过括号。
const squared = x => (x ** 2)
Now that you have brushed up on the arrow syntax, let’s begin to understand some higher-order array methods!
现在,您已经精通了arrow语法,让我们开始了解一些高阶数组方法!
forEach(): Think of it as a less verbose implementation of a
for loop
. It invokes a function on each array element, and its syntax goes like this:forEach() :将其视为
for loop
的较不冗长的实现。 它在每个数组元素上调用一个函数,其语法如下所示:
array.forEach((element, index) => {
// some operations on the element
// maybe you want to use the index of the element
});
In case you want to see a pretty contrived example, take a look at the following example.
如果您想看到一个非常人为的示例,请看以下示例。
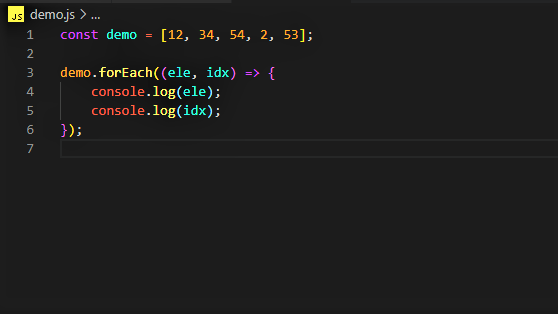
map(): If you’ve understood forEach(), then this is a piece of cake! It functions exactly like a forEach, but returns a new array, unlike the forEach() method. The syntax is as follows:
map() :如果您了解forEach(),那么这真是小菜一碟! 它的功能与forEach完全相同,但是与forEach()方法不同,它返回一个新数组 。 语法如下:
const returnedArr = array.map(currentEle => {
// some operation on currentEle
})
It’s slightly different to the forEach() method, but you should be able to use them interchangeably for most applications. In case you want to know about the differences, you can go through the following article.
它与forEach()方法稍有不同,但是您应该可以在大多数应用程序中互换使用它们。 如果您想了解不同之处,可以阅读以下文章。
reduce() is especially useful whenever you need to compute a single value based on the data stored in an array. As the name suggests, this reduces an array into a single value, but can be a little tricky to use! The callback function that this method accepts, works on each element of the array in a way that reduces the array to a single value. The syntax is as follows:
当您需要基于存储在数组中的数据计算单个值时, reduce()尤其有用。 顾名思义,这将数组简化为单个值,但使用起来可能有些棘手! 此方法接受的回调函数以将数组简化为单个值的方式作用于数组的每个元素。 语法如下:
const reducedVal = array.reduce(callback, initialVal);
Here, callback
needs to take 2 arguments. The first argument acts as an accumulator, whose value persists throughout the process. The second represents the current value of the array.
在这里, callback
函数需要2个参数 。 第一个参数充当累加器 ,其值在整个过程中都会保留。 第二个代表数组的当前值 。
A simple example can be to find the sum of the array elements.
一个简单的例子就是找到数组元素的总和。
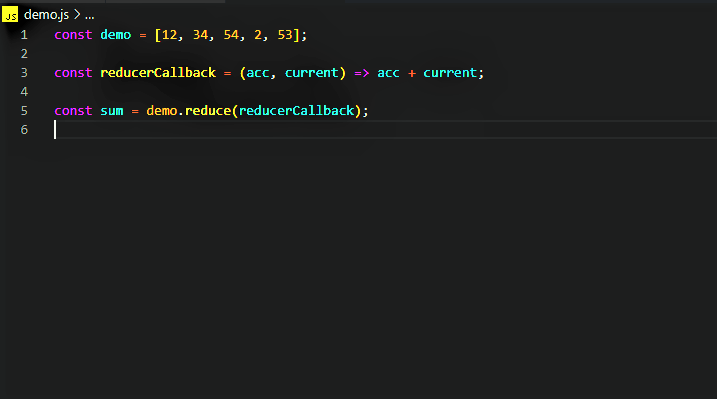
In the above example, the reduce()
method has a callback function called reducerCallback
(very creative, I know!). This callback needs to have 2 arguments, I called mine acc
and current
.
在上面的示例中, reduce()
方法具有一个称为reducerCallback
的回调函数(非常有创意,我知道!)。 这个回调需要有2个参数,我叫mine acc
和current
。
The basic idea is that the value in acc
is persisted each time the callback method is executed. This means that if the reducerCallback
is executed for the 2nd element of demo
, then the values of the arguments acc
and current
are 12 and 34 respectively. The callback then adds these two values and returns them. This returned value is now the new value stored in acc
其基本思想是,在值acc
每次执行回调方法时间持续 。 这意味着,如果该reducerCallback
针对的第二元件执行demo
,则参数的值acc
和current
分别为12和34。 然后,回调将这两个值相加并返回它们。 现在,此返回值是存储在acc
的新值
So, for the 3rd callback execution, the values of acc
and current
are 46 and 54. You can see how the array’s values are being used to get to a single value.
因此,对于第三次回调执行, acc
和current
的值为46和54 。 您可以看到如何使用数组的值获得单个值。
But I also mentioned a second argument called initialVal
in the syntax. This is set as the initial value of the acc
variable. In case you do not specify any initialVal
argument, acc
will take the array element at the 0th index as its default initial value.
但是我在语法中还提到了另一个名为initialVal
参数。 设置为acc
变量的初始值。 如果您未指定任何initialVal
参数,则acc
将第0个索引处的数组元素作为其默认初始值 。
Here is an article that gives you a verbose explanation about the workings of the reduce()
method
这是一篇为您提供关于reduce()
方法工作方式的详细说明的文章
The next method that is used often is filter(). It is very helpful if you want to extract a sub-array from a larger array, based on some common property. The syntax is as follows
经常使用的下一个方法是filter() 。 如果您要基于某个公共属性从更大的数组中提取子数组,这将非常有帮助。 语法如下
const filteredArr = array.filter(callback);
Here callback
accepts an argument current
that results is a boolean value being returned. Based on the return value, the current
value is pushed to filteredArr
.
这里的callback
接受一个current
的参数,结果是返回一个布尔值 。 基于返回值, current
值被推送到filteredArr
。
For example, suppose you want to separate the even numbers from a given array.
例如,假设您要从给定数组中分离偶数。
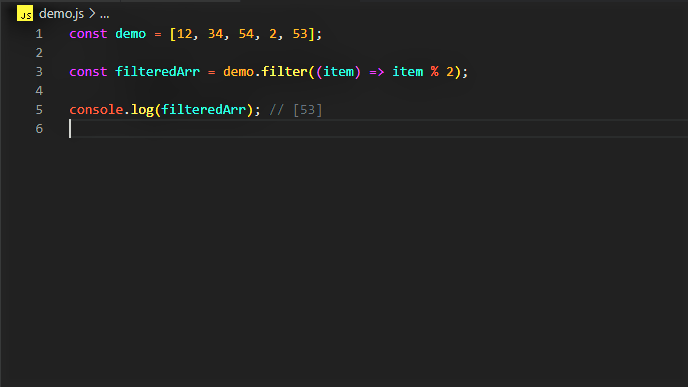
Here, the callback
function is anonymous and it accepts an argument that represents the current element of the demo
array. If the callback
returns true
then item
is pushed to the resultant array filteredArr
.
在这里, callback
函数是匿名的,它接受代表demo
数组当前元素的参数。 如果callback
返回true
则将item
推送到结果数组filteredArr
。
Here, for all the even numbers in demo
, our callback
returns a 0(zero), which is falsy in nature. Hence, all the even numbers are omitted from filteredArr
. On the other hand, all the odd numbers return 1(one) which is equivalent to true
. This way, our demo
array has been filtered!
在这里,对于demo
所有偶数,我们的callback
返回0(零),本质上是虚假的。 因此,从filteredArr
中省略了所有偶数。 另一方面,所有奇数均返回1(one) ,它等于true
。 这样,我们的demo
数组已被过滤!
You can go through the following article to see some more examples.
您可以阅读下面的文章,以查看更多示例。
Lastly, let’s understand a higher-order method used most often. sort() is a method that doesn’t quite work like we assume it would!
最后,让我们了解最常用的高阶方法。 sort()是一种无法像我们假设的那样有效的方法!
You would imagine that the following code works by sorting the array in ascending order by default, right?
您会想象下面的代码通过默认情况下按升序对数组进行排序而起作用,对吗?
const demo = [100, 20, 89, 3, 17];demo.sort();
But, it returns the array [100, 17, 20, 3, 89]
. “What? Why?”, I hear you say. Let’s understand why sort() has such a behavior.
但是,它返回数组[100, 17, 20, 3, 89]
。 “什么? 为什么?”,我听到你说。 让我们了解为什么sort()具有这种行为。
sort() assumes that all the array elements are String
by default, and sorts the elements based on the UTF-16 code values, in case it is not passed any callback!
sort()假定默认情况下所有数组元素均为String
,并在不传递任何回调的情况下,根据UTF-16代码值对元素进行排序!
This is why 100 will come before 20. In order to sort an array in a more traditional way (i.e, as numbers) we will need to use a callback function as follows array.sort(callback)
这就是为什么100在20之前的原因。为了以更传统的方式对数组进行排序(即,作为数字),我们将需要使用如下的回调函数array.sort(callback)
The callback
needs to accept 2 arguments and return a numeric value indicating how these 2 arguments need to be stored in the sorted array.
callback
需要接受2个参数,并返回一个数值,指示这两个参数需要如何存储在已排序的数组中。
Here is an example to sort an array of elements in ascending order:
这是一个以升序对元素数组进行排序的示例:
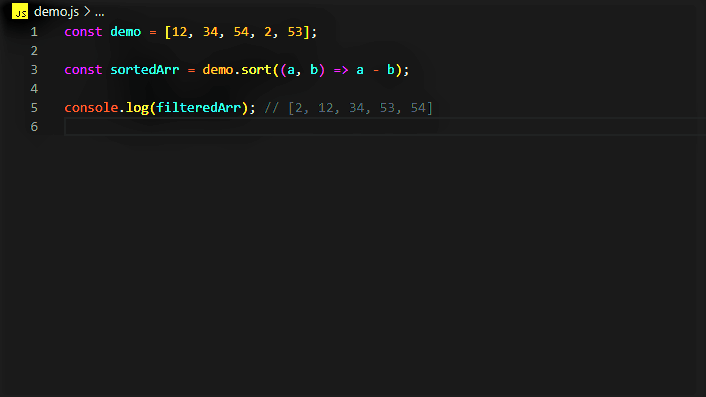
If
callback(a, b)
returns less than 0,a
comes beforeb
.如果
callback(a, b)
返回小于0,则a
在b
之前。If
callback(a, b)
returns 0,a
andb
are left at their current index.如果
callback(a, b)
返回0,则a
和b
保留在其当前索引处。If
callback(a, b)
returns greater than 0,b
comes beforea
如果
callback(a, b)
返回大于0,则b
在a
之前
Note that callback(a, b)
must always return the same value when given a specific pair of elements a
and b
as its two arguments.
注意,给定特定的一对元素a
和b
作为其两个参数时, callback(a, b)
必须始终返回相同的值。
Here a
and b
are the 2 consecutive elements of the demo
array, which are continuously compared in the callback
. Here, if you wanted to sort the array in descending order, all you need to do is change the callback
to the following.
这里a
和b
是demo
数组的2个连续元素,它们在callback
中连续进行比较。 在这里,如果要对数组进行降序排序,则只需将callback
更改为以下内容即可。
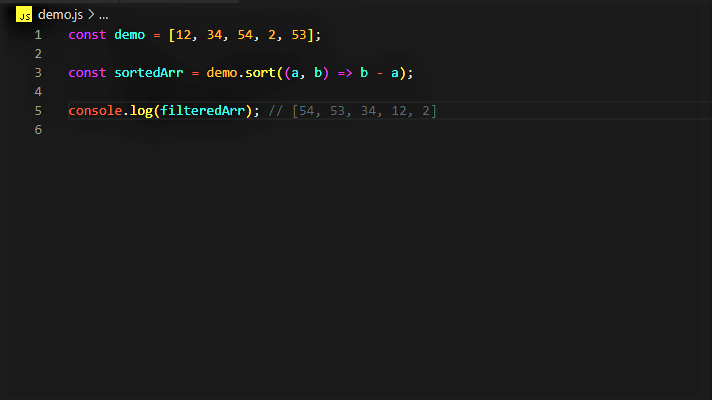
In case you want to look at more examples of how to use the sort() method, you can go through the following article.
如果您想查看有关如何使用sort()方法的更多示例,请阅读以下文章。
All said and done, these methods are a sliver of all the higher-order array methods offered by JavaScript. Although these are the methods that you’ll use on a more regular basis, it is not a futile attempt to go through the rest of the methods!
总而言之,这些方法只是JavaScript提供的所有高阶数组方法的一小部分。 尽管这些是您会更经常使用的方法,但尝试其余方法并不是徒劳的!
In case you wish to learn more about any of these array methods, or if you want to learn some more higher-order methods, I’d suggest that you go to the MDN Docs, as it provides a pretty thorough explanation of all the methods that JavaScript has to offer.
如果您想了解有关这些数组方法的更多信息,或者想了解更多高级方法,建议您使用MDN Docs ,因为它提供了所有方法的详尽解释。 JavaScript必须提供的。
翻译自: https://medium.com/weekly-webtips/what-are-higher-order-array-methods-in-javascript-85d318f30cbc