react和react2
Are you building an app that needs to be snappy for its users? Then you should probably consider doing optimistic updates. This will greatly improve the customer experience and the overall feel of your app. It will also better mimic the experience you have on a native desktop app.
您是否正在构建一个需要对其用户保持敏捷的应用程序? 然后,您可能应该考虑进行乐观更新。 这将极大地改善客户体验和您的应用程序的整体感觉。 它还将更好地模拟您在本机桌面应用程序上的体验。
什么是乐观更新? (What are optimistic updates?)
Optimistic updates are a way of updating data in your app. You generally want to have a local state inside of your app, and before making the HTTP request to update in the backend, you want to update the local state with the data you assume will be returned by the backend. This also prevents jumps or flashes between the old and new data.
乐观更新是一种更新应用程序中数据的方法。 通常,您希望在应用程序内部拥有一个本地状态,并且在后端进行HTTP请求更新之前,您希望使用假定后端将返回的数据来更新本地状态。 这也可以防止新旧数据之间的跳转或闪烁。
Patch local state with assumed data
使用假定的数据修补本地状态
Patch database in backend through HTTP
通过HTTP在后端修补数据库
Patch local state with returned values (optional)
使用返回值修补本地状态(可选)
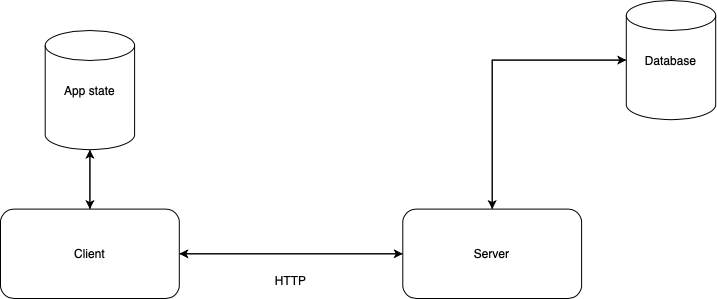
为什么要乐观地更新? (Why optimistic updates?)
As I briefly mentioned earlier, optimistic updates are good if you are building an app that is in dire need of performance and rapidness. For example, if you are building a Kanban board tool where your users can drag cards back and forth between columns waiting for the response from the server destroys the experience. Each time the user drags a card it’s going to jump back to its previous column until the server responds (which can take many seconds on a slow connection). You might think that this is only an issue with slow connections. But, if you try to implement something like a Kanban board this way, you will quickly realize, even if you’re on a really solid internet connection, that the card will quickly jump back to its previous position before taking its new one. Even if it’s just for a split second this can cause a user to have a pretty bad experience.
如前所述,如果您正在构建急需性能和快速性的应用程序,那么乐观更新是很好的选择。 例如,如果您正在构建看板板工具,则用户可以在列之间来回拖动卡,等待服务器的响应,这会破坏体验。 每次用户拖动卡时,它将跳回到上一列,直到服务器响应为止(在缓慢的连接上可能要花费几秒钟)。 您可能会认为这只是连接缓慢的问题。 但是,如果您尝试以这种方式实现类似看板的功能,即使您使用的是牢固的Internet连接,您也将很快意识到,该卡将在采用新卡之前Swift跳回其先前位置。 即使只是一瞬间,这也可能导致用户体验很差。
Improved UX
改进的用户体验
Better synced state
更好的同步状态
Offline functionality
离线功能
Native feel
本机的感觉
如何进行乐观更新? (How to do optimistic updates?)
Implementing optimistic updates can be done in so many different ways. Either you can just use a state hook and update it before requesting the server. I’ll cover how to do this in Redux since it’s pretty easy — and also gives offline functionality by default.
实施乐观更新的方法有很多种。 您可以只使用状态挂钩并在请求服务器之前对其进行更新。 我将在Redux中介绍如何执行此操作,因为它非常简单-默认情况下还提供了离线功能。
安装redux-offline和redux-thunk作为依赖项 (Install redux-offline and redux-thunk as dependencies)
npm install redux-offline redux-thunk
更新您的商店以使用中间件 (Update your store to use the middlewares)
import { applyMiddleware, compose, createStore } from "redux";
import { offline } from '@redux-offline/redux-offline';
import offlineConfig from '@redux-offline/redux-offline/lib/defaults';
import rootReducer from "./reducers";
import thunk from "redux-thunk";const store = createStore(
rootReducer,
compose(applyMiddleware(thunk), offline(offlineConfig))
);export default store;
更新您要乐观更新的操作 (Update the action you want to be optimistically updated)
dispatch({
type: MOVE_CARD,
payload: {
card: { ...card, columnId: toColumnId },
fromColumnId: card.columnId,
},
meta: {
offline: {
effect: {
url: `/columns/${fromColumnId}/cards/${id}`,
method: 'PATCH',
body: JSON.stringify({ order, toColumnId }),
},
commit: { type: 'MOVE_CARD_COMMIT' },
rollback: { type: 'MOVE_CARD_ROLLBACK', meta: { card, toColumnId } },
},
},
})
这里发生了什么事? (What’s happening here?)
- Dispatches the action MOVE_CARD with the assumed new card as payload. 使用假定的新卡作为有效负载调度动作MOVE_CARD。
- Makes the network request to update data on the server. 发出网络请求以更新服务器上的数据。
- If the network request succeeds we dispatch the MOVE_CARD_COMMIT action. Here, the payload will be the result of the actual HTTP request. Else, if it doesn’t succeed, we dispatch the MOVE_CARD_ROLLBACK and send the initial card (which will not be updated) as data to the reducer. 如果网络请求成功,我们将调度MOVE_CARD_COMMIT操作。 在这里,有效负载将是实际HTTP请求的结果。 否则,如果未成功,我们将调度MOVE_CARD_ROLLBACK并将初始卡(将不会更新)作为数据发送到减速器。
减速器 (The Reducer)
Now when we have our action ready to go, we need to set up a couple of switch-case statements in our reducer. This shouldn’t be very difficult, but it’s quite important to get right, since it won’t properly work otherwise and can cause some unwanted side effects, causing out-of-sync state.
现在,当我们准备好要采取的措施时,我们需要在化简器中设置几个switch-case语句。 这应该不是很困难,但是正确无误非常重要,因为否则它将无法正常工作,并且可能导致一些不良的副作用,从而导致状态不同步。
case MOVE_CARD:
return {
...state,
data: state.data.map((card) =>
card.id === action.payload.card.id &&
card.columnId === action.payload.fromColumnId
? action.payload.card
: card
),
}case MOVE_CARD_COMMIT:
return {
...state,
// Here you can for example update a loading state
}case MOVE_CARD_ROLLBACK:
return {
...state,
data: state.data.map((card) =>
card.id === action.meta.card.id &&
card.columnId === action.meta.toColumnId
? action.meta.card
: card
),
}
取得的成果 (The achieved result)
If you’ve done this properly, you should now be able to drag a card, or make an action of a similar interactive degree, and see it instantly being updated in your local Redux state. After a couple of seconds, your network request should be finished and you can use that data, however, you want. What you also can do is to construct an error in your network request. This should make the action update the local app state, but when the network request fails the state should get rolled back to how it previously was.
如果操作正确,您现在应该可以拖动卡片或进行类似互动程度的操作,并立即以本地Redux状态对其进行更新。 几秒钟后,您的网络请求应已完成,但是您可以使用该数据。 您还可以做的是在网络请求中构造一个错误。 这应该使操作更新本地应用程序状态,但是当网络请求失败时,该状态应回滚到以前的状态。
Another really neat thing with using redux-offline is that if you simulate your app in offline mode and start taking actions, the local state should still be updated, but no network requests should occur — until you switch back to online mode. Then, every network request for each action should’ve been saved to a buffer that is now going to be executed in consecutive order and get your app backend up to sync with the recently made actions on the client. This is great if a user for some reason has lost connection to your app, but you still want them to be able to make changes and see some sort result until they get their connection back again.
使用redux-offline的另一件真正的好事是,如果您在离线模式下模拟应用程序并开始执行操作,则本地状态仍应更新,但不会发生网络请求-直到切换回在线模式为止。 然后,对每个操作的每个网络请求都应该保存到缓冲区中,该缓冲区现在将以连续顺序执行,并使您的应用程序后端可以与客户端上最近执行的操作同步。 如果用户由于某种原因失去了与应用程序的连接,这很好,但是您仍然希望他们能够进行更改并查看排序结果,直到他们重新获得连接。
I hope some of this will help you to start making optimistic updates in React. As I mentioned in the beginning, there are many different ways of doing this — and this is just one way, that is especially good if you already use Redux for state management. I realize that this is not a full-fledged tutorial on how to set up Redux in your app, so if you have any questions feel free to leave them down below are send me a message. Thanks for reading!
我希望其中一些可以帮助您开始在React中进行乐观更新。 正如我在开始时提到的,有很多不同的方法可以做到这一点,而这只是一种方法,如果您已经使用Redux进行状态管理,那将特别好。 我意识到这不是有关如何在您的应用中设置Redux的完整教程,因此,如果您有任何疑问,请随时将其保留在下面,并给我发消息。 谢谢阅读!
翻译自: https://levelup.gitconnected.com/optimistic-updates-in-react-803003844cb0
react和react2