react和react2
React.js is an open-source JavaScript library for building user interfaces. That word, *building*, is the crux of my whole comparison here. As difficult as React felt to initially pick up, you’ll quickly learn that much like legos, building something in React can be an incredibly fun experience. So, let’s take a journey…
React.js是用于构建用户界面的开源JavaScript库。 这个“建筑物”这个词是我整个比较中的关键。 尽管起初React很难,但您很快就会学到乐高积木,在React中构建东西会是一次非常有趣的体验。 因此,让我们一起旅行吧……
React has incredible documentation that opens up with three core principles. React is: declarative, component based, and is a tool that can be learned once and used anywhere. We’re going to focus on the first two. React being declarative essentially boils down to its ability to inject the proper JSX (an XML-like-syntax that renders web-views for you) at the appropriate time depending on your application. Being component based is where we start to see the lego comparison; these components allow you to break down your code into smaller, more digestible, and more legible plug-and-play type code in order to build very interactive webpages. For our example, we’re going to take a look at an application that was built to function much like a Pokédex (in fact, the only thing that was missing was the red, gameboy-like casing to really tie it all together).
React具有令人难以置信的文档 ,其中包含三个核心原则。 React是:声明式的,基于组件的,并且是一个可以一次学习并可以在任何地方使用的工具。 我们将专注于前两个。 从本质上讲,React是声明式的,可以归结为它根据应用程序在适当的时候注入适当的JSX(类似于XML的语法,可以为您提供Web视图)的能力。 基于组件是我们开始看到乐高比较的地方。 这些组件使您可以将代码分解为更小,更易消化和更清晰的即插即用类型代码,以构建具有高度交互性的网页。 对于我们的示例,我们将看一看构建为功能类似于Pokédex的应用程序(实际上,唯一缺少的是真正将它们绑在一起的红色,类似Gameboy的外壳)。
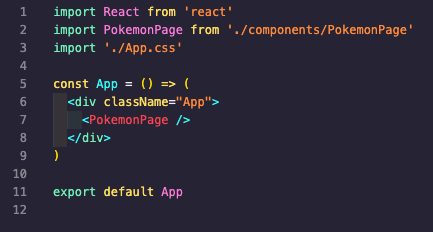
Want to read this story later? Save it in Journal.
想稍后再读这个故事吗? 将其保存在 日记本中 。
Above, you’ll notice our top-level App component that is going to house everything we build. We’re going to render this bad boy on our main JS file that will then render onto our webpage. What this ultimately allows us to do is utilize one element in our main HTML file (see below).
在上方,您会注意到我们的顶级应用程序组件将容纳我们构建的所有内容。 我们将在我们的主要JS文件上渲染这个坏男孩,然后将其渲染到我们的网页上。 这最终允许我们做的是利用主HTML文件中的一个元素(请参见下文)。


Once you’ve made it this far you can start getting really clever with the rest of your components. Legos, typically, build from the ground up (I don’t know you or how you play with legos, so I’ll just assume you conform to the status-quo). Unlike legos, React builds from the top down, in that starting with the main component (in this case, <App />), there are child components all the way down. In our example above, the child of App is <PokemonPage /> which will house everything we need to pass down state/props to its children.
一旦到此为止,您就可以开始对其余的组件变得非常聪明。 乐高玩具通常是从头开始的(我不认识您,也不知道您如何与乐高玩具一起玩,所以我只假设您符合现状)。 与乐高积木不同,React从上到下构建,因为从主要组件(在本例中为<App />)开始,一直有子组件。 在上面的示例中,App的子级是<PokemonPage />,它将容纳我们将状态/属性传递给其子级所需的所有内容。
Side note: state and props are the actual core of React but in the essence of time we’ll just go over the TL;DR — state is anything within a class component that you will need to adjust on the fly (i.e. any data you’re pulling from an API or the values you’ll be changing in a controlled form); props are the pieces of the application that are passed from a parent to a child. Another quick metaphor for clarity: you can consider your eye color, height, hair color, etc to be the props you inherit from your parents, whereas your overall fitness, your sense of style, etc can be considered your state.
旁注 :状态和道具是React的实际核心,但在时间的本质上,我们只讨论TL; DR -状态是您需要即时调整的类组件中的任何内容(即您所需要的任何数据)从API或您将以受控形式更改的值中提取); 道具是从父母传递给孩子的应用程序的一部分。 另一个清晰的比喻:您可以将眼睛的颜色,身高,头发的颜色等视为从父母那里继承来的道具,而整体的健康状况,风格感等则可以视为您的状态。
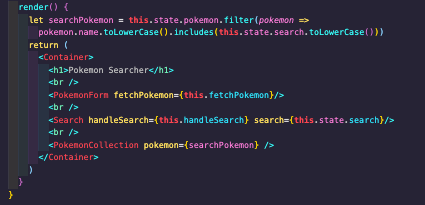
In our main container above, you can see a few more components start to manifest (<PokemonForm />, <Search />, <PokemonCollection />) that will continue to add more lego pieces to our application. As your application grows, you will have to make decisions regarding how the structure of the application should grow. Does this need to be a class component or functional component? Where should I hold state? Comparatively to VanillaJS, when you had to add event listeners to the document and target specific elements, React allows you to pass the functions that handle things like a click, submit, change, etc directly onto the component that needs it — which is a huge value add to both code structure and ease of use.
在上面的主容器中,您可以看到更多的组件开始显现(<PokemonForm />,<Search />,<PokemonCollection />),这些组件将继续为我们的应用程序添加更多的乐高积木。 随着应用程序的增长,您将不得不决定应用程序的结构应如何增长。 这需要是类组件还是功能组件? 我应该在哪里保持状态? 与VanillaJS相比,当您不得不向文档中添加事件侦听器并定位特定元素时,React允许您将处理诸如单击,提交,更改等操作的函数直接传递到需要它的组件上-这是一个巨大的任务增添代码结构和易用性。
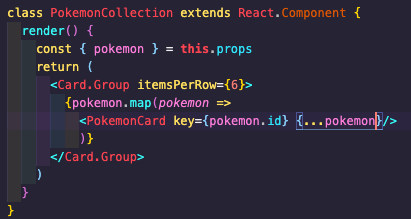
As we progress down the chain of ownership, we can render different JSX elements in order to accomplish the ultimate views we are looking for. In this case, we’re generating new “cards” to render each Pokémon in our database. Thinking ahead, if we came across a new Pokémon that didn’t exist in our app, we could build another component that allows us to submit a new entry. You’ll notice we built that functionality in this app (<PokemonForm /> in our main container), but rather than highlight more syntax, we’ll imagine that we can scale this application as we see fit…because legos.
当我们沿着所有权链前进时,我们可以呈现不同的JSX元素,以完成我们所寻找的最终视图。 在这种情况下,我们将生成新的“卡片”以渲染数据库中的每个神奇宝贝。 提前考虑,如果我们遇到了应用程序中不存在的新神奇宝贝,我们可以构建另一个组件来允许我们提交新条目。 您会注意到我们在此应用程序中构建了该功能(在主容器中为<PokemonForm />),但是我们没有想象要突出显示更多的语法,而是想象我们可以根据自己的喜好扩展此应用程序……因为legos。
When I think back to my childhood and the early days of playing with legos, I find the same level of excitement in building something from the ground up (or top down) when I’m playing around with a React application. Unless you’re like me and got the hand-me-downs from your older brother, most legos tend to come with a set of instructions. Typically, with React, before you start mashing the keyboard you’ll take the time to plan out a wireframe and lay out your webpage-to-be as a set of pre-development instructions. As your ideas grow and you start to think ahead for how the application might change, using React makes it very easy to continue to pile on the lego blocks (components) that will scale with your app. So, yeah, I don’t think we ever grow out of that phase of what makes playing with legos so fun, but our tastes and our tools definitely do change.
当我回想起童年和玩乐高玩具的早期时,当我在玩React应用程序时,从头开始(或从上到下)构建一些东西时,我会感到同样兴奋。 除非您像我一样,并且从您的哥哥那里得到了帮助,否则大多数乐高玩具都会附带一些说明。 通常,使用React,在开始融合键盘之前,您将花时间规划线框,并按照一组预开发说明来布置网页。 随着想法的发展,您开始就应用程序可能如何变化进行思考,使用React可以很容易地继续堆积将随您的应用程序扩展的lego块(组件)。 所以,是的,我不认为我们会脱离让乐高积木玩起来如此有趣的那个阶段,但是我们的品味和工具肯定会发生变化。
📝 Save this story in Journal.
story将这个故事保存在Journal中 。
👩💻 Wake up every Sunday morning to the week’s most noteworthy stories in Tech waiting in your inbox. Read the Noteworthy in Tech newsletter.
every💻每个星期天的早晨,您都可以在收件箱中等待本周最受关注的Tech故事。 阅读Tech Newsletter中的“值得注意” 。
翻译自: https://blog.usejournal.com/react-is-just-grown-up-legos-hear-me-out-22574a405b82
react和react2