date-fns日期格式化
总览 (Overview)
Ever written a method to get the time of day in GMT? or have wondered if I need to convert the time to UTC? Most applications have some common date-time manipulations which are often repeated and after some time become very difficult to maintain. To add upon this when internationalizing an application Date and Time manipulations are one of the key challenges you will face. So much so that you will lose track of time amending it all 😉.
有没有写过一种获取格林尼治标准时间的方法? 还是想知道我是否需要将时间转换为UTC? 大多数应用程序具有一些常见的日期时间操作,这些操作通常会重复执行,并且在一段时间后变得很难维护。 在国际化应用程序时添加此功能日期和时间操作是您将面临的主要挑战之一。 如此之多,以至于您将失去修改所有内容的时间😉。
Tick Tock, Tick Tock! Fear not for we have you covered. date-fns
is one such package that provides simple helper functions for date-time manipulation. With internationalization options available you can always leverage it and go international quickly.
滴答滴答,滴答滴答! 不要害怕,因为我们已经覆盖了您。 date-fns
是一个这样的程序包,它提供了用于日期时间操作的简单帮助程序功能。 有了国际化选项,您始终可以利用它并Swift走向国际。
强调 (Highlights)
Getting started with date-fns is pretty easy. It can be installed by running the command npm install date-fns
. All helper methods can either be imported through the main package or you can install only the required submodule. One such is fp
which has two copies of methods with and without format options.
date-fns入门非常容易。 可以通过运行命令npm install date-fns
。 所有辅助方法都可以通过主软件包导入,也可以仅安装所需的子模块。 其中之一就是fp
,它具有方法的两个副本,带有和不带有格式选项。
/* Babel or ES6 */
import {methodName} from "date-fns";/* Node or requireJs */
const methodName = require("date-fns").methodName;
It deserves extra brownie points as it provides typescript support without any additional packages and helps developers in not having to maintain another dev dependency. Its package structure promotes tree-shaking
which helps in overall application size and hence we suggest installing the main module as only the required methods would get bundled in. The developer has to handle one of three types namely:
它提供了打字稿支持而无需任何其他程序包,因此值得额外的布朗尼点,并帮助开发人员不必维护另一个dev依赖项。 它的包结构促进了tree-shaking
这有助于整个应用程序的大小,因此,我们建议安装主模块,因为仅捆绑了所需的方法。开发人员必须处理以下三种类型之一:
Interval: It is a simple representation of the time interval between two date instances. It is purely used in methods that operate on time spans.
时间间隔:它是两个日期实例之间时间间隔的简单表示。 它仅用于按时间跨度运行的方法。
Locale: Represents data of the desired country which is used in formatting date.
区域设置:表示在格式化日期中使用的所需国家/地区的数据。
Duration: The main difference between a standard
Date
object and the Duration object is that the latter is devoid of any Locale information. A Duration can consist partially any of the following:持续时间:标准
Date
对象和Duration对象之间的主要区别是后者没有任何语言环境信息。 持续时间可以部分由以下任意一项组成:
{
years, months, weeks, days, hours, minutes, seconds
}
Most helpers provided can be categorized into the following four categories based on the dimension of date-time they help simplify.
根据提供的大多数帮助程序,它们可以简化的日期时间范围可分为以下四类。
时间帮手 (Time helpers)
These helpers mainly focus on manipulating and validating predicates on aspects of time such as seconds, minutes, hours. It provides useful utilities on time spans through the Interval
type. The following table gives a nice overview of a few methods:
这些帮助程序主要专注于处理和验证有关时间方面的谓词,例如秒,分钟,小时。 它通过Interval
类型在时间跨度上提供有用的实用程序。 下表很好地概述了几种方法:
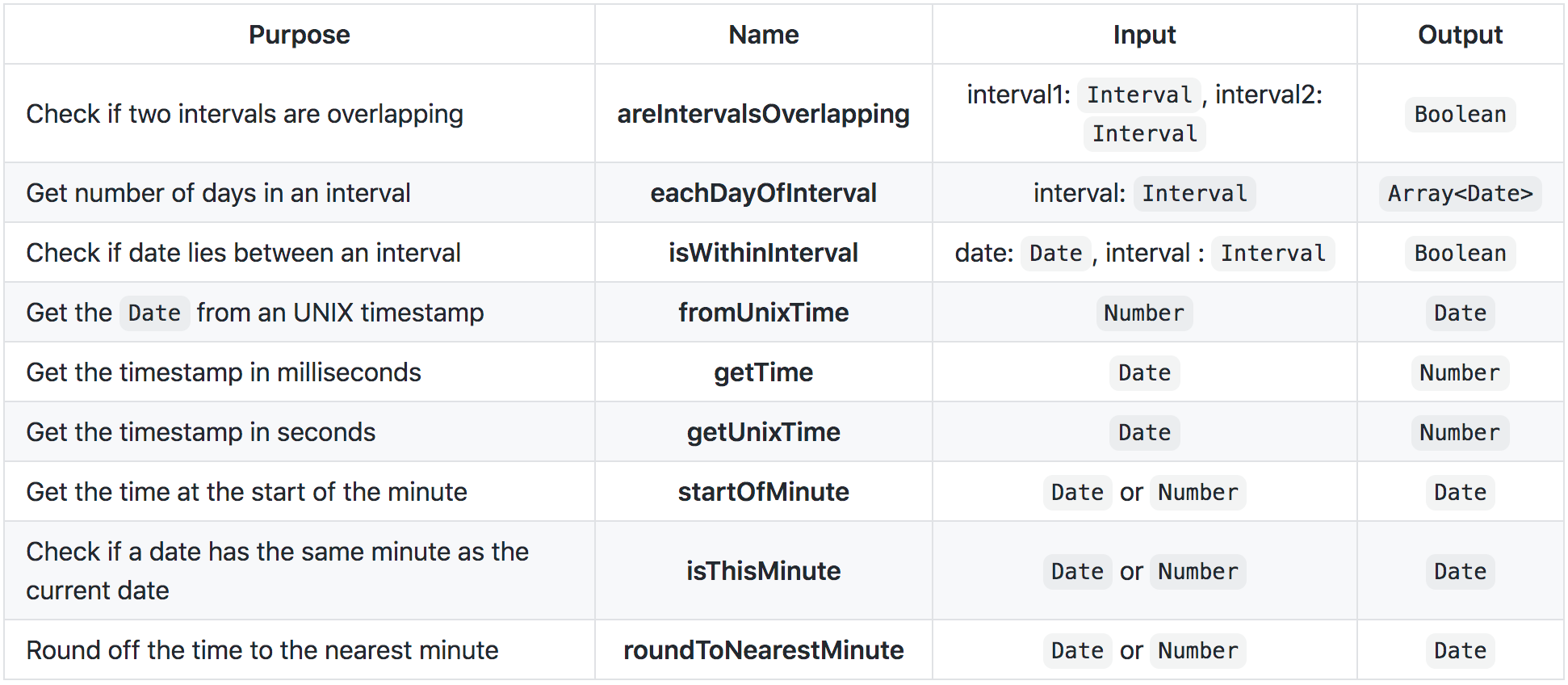
Some examples to help familiarise you with the above functions are:
以下示例可帮助您熟悉上述功能:
const interval1 = {
start: new Date(2014, 0, 10), // 10th Jan 2014
end: new Date(2014, 11, 21) // 21st Dec 2014
};const interval2 = {
start: new Date(2014, 6, 10), // 10th July 2014
end: new Date(2015, 0, 10) // 10th Jan 2015
};const interval3 = {
start: new Date(2015, 6, 10), // 10th July 2015
end: new Date(2020, 11, 10) // 10th Dec 2020
};const interval4 = {
start: new Date(2015, 11, 10), // 10th Dec 2015
end: new Date(2015, 11, 10) // 10th Dec 2015
};
areIntervalsOverlapping:
areIntervalsOverlapping:
console.log(areIntervalsOverlapping(interval1, interval2));
// => true
console.log(areIntervalsOverlapping(interval1, interval3));
// => false
eachDayOfInterval:
eachDayOfInterval:
console.log(eachDayOfInterval(interval4));
// => [Sun Jan 10 2016 00:00:00 GMT+0530 (India Standard Time)]
isWithinInterval:
isWithinInterval:
console.log(isWithinInterval(Date.now(), interval1)); // => false
console.log(isWithinInterval(Date.now(), interval3)); // => true
fromUnixTime:
来自UnixTime:
console.log(fromUnixTime(1595157314));
// => Sun Jul 19 2020 16:45:14 GMT+0530 (India Standard Time)
getTime:
getTime:
console.log(getTime(Date.now())); // => 1595157440507
getUnixTime:
getUnixTime:
console.log(getUnixTime(Date.now())); // => 1595157492
startOfMinute:
startOfMinute:
console.log(startOfMinute(Date.now())); // => Sun Jul 19 2020 17:02:00 GMT+0530 (India Standard Time)
isThisMinute:
isThisMinute:
console.log(isThisMinute(Date.now())); // => true
console.log(isThisMinute(new Date(2020, 6, 10, 17, 4))); // => false
roundToNearestMinute: rounds off the time to the start of the minute that the given date is closest to. We can change this by providing the
nearestTo
option which can be between 1 - 30. If it is equally distant to both the previous and next interval, then it rounds up.roundToNearestMinute :将时间四舍五入到给定日期最接近的分钟的开始。 我们可以通过提供
nearestTo
选项(可以在1到30之间)来更改此值。如果它与上一个和下一个间隔都相等,则将其四舍五入。
console.log(roundToNearestMinutes(Date.now()));
// => Sun Jul 19 2020 17:12:00 GMT+0530 (India Standard Time)console.log(roundToNearestMinutes(Date.now(), {nearestTo: 15}));
// => // => Sun Jul 19 2020 17:15:00 GMT+0530 (India Standard Time)console.log(roundToNearestMinutes(new Date(2020, 6, 10, 17, 13), {nearestTo: 8}));
// => Fri Jul 10 2020 17:16:00 GMT+0530 (India Standard Time)console.log(roundToNearestMinutes(new Date(2020, 6, 10, 17, 11), {nearestTo: 8}));
// => Fri Jul 10 2020 17:08:00 GMT+0530 (India Standard Time)/* here 17:12 is equidistant from 17:8 and 17:16, the method rounds it off to 16 */console.log(roundToNearestMinutes(new Date(2020, 6, 10, 17, 12), {nearestTo: 8}));
// => Sun Jul 19 2020 17:16:00
Let’s see how we can apply our time helpers to some real-life use cases:
让我们看看如何将我们的时间助手应用于一些实际的用例:
- Calculate if a booking has come within the time of registration: 计算预订是否在注册时间内到达:
const checkIfBookingIsValid = timeOfBooking => isWithinInterval(timeOfBooking, {
start: startTimeOfRegistration,
end: endTimeOfRegistration
});
- Specify when next the scheduled job is to run: 指定下一个计划作业何时运行:
const getNextStartTimeOfJob = (lastExecutionTime, {days, hours, minutes, seconds}) => add(lastExecutionTime, {days, hours, minutes, seconds})
约会助手 (Date helpers)
While the time helpers are useful in handling the micro-aspects, the Day helpers are used to manipulate the macro-aspects such as Days, Weeks, Quarters, Years, Decades. These helpers simplify our applications where we might need to calculate if the user is above an age threshold, group data, and perform a bulk operation. More such highlighted functions are as shown below:
尽管时间助手在处理微观方面很有用,但是日助手用于操纵宏观方面,例如天,周,季度,年,十年。 这些帮助程序简化了我们的应用程序,在这些应用程序中,我们可能需要计算用户是否超过年龄阈值,对数据进行分组并执行批量操作。 更多此类突出显示的功能如下所示:
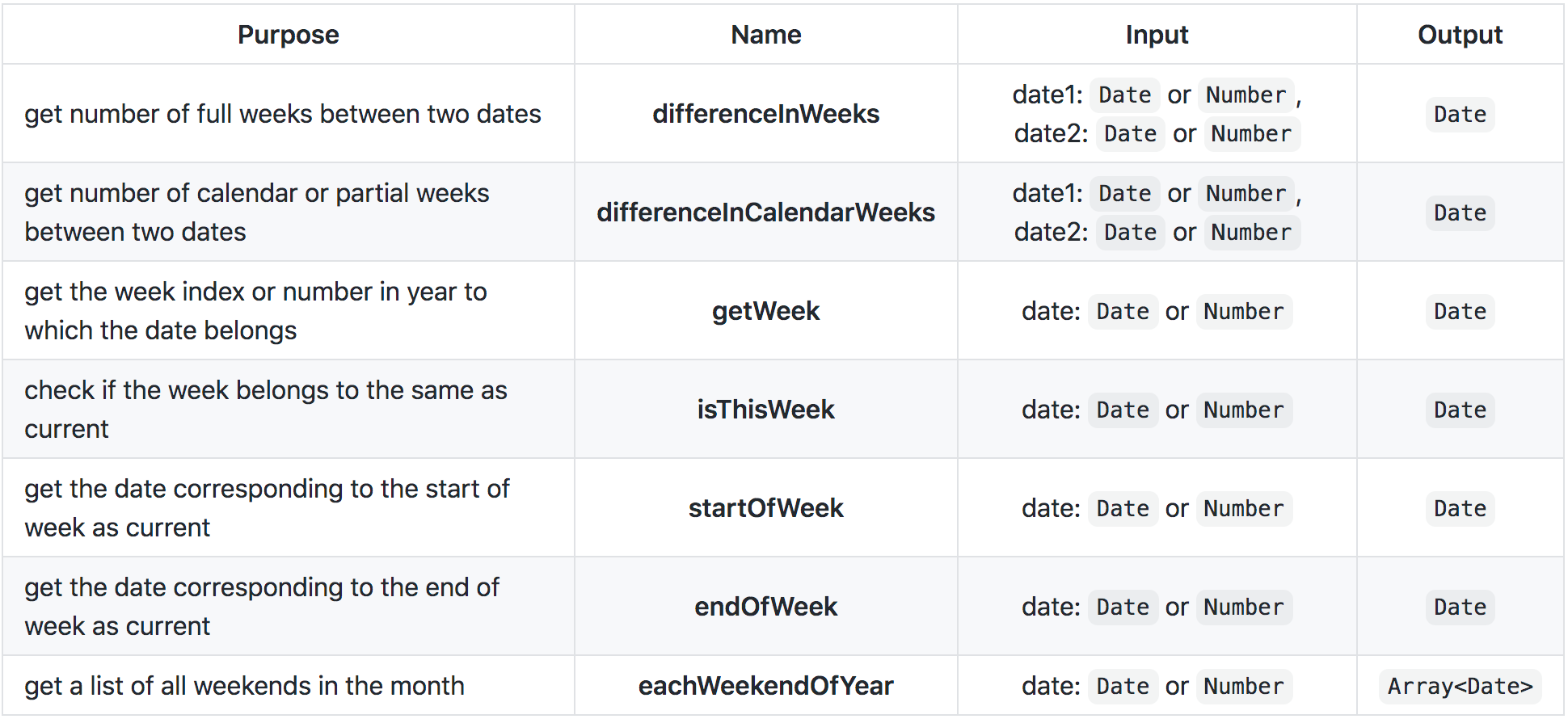
Most methods explained for weeks above are extended to months, quarters, and years. such as differenceInMonths
, differenceInCalendarMonths
, isSameMonth
, isSameYear
, setMonth
, setYear
, eachWeekendOfMonth
,etc.
上面几周解释的大多数方法都扩展到了几个月,一季度和几年。 例如differenceInMonths
, differenceInCalendarMonths
, isSameMonth
, isSameYear
, setMonth
, setYear
, eachWeekendOfMonth
等。
Some examples to help familiarise you with the above functions are:
以下是一些有助于您熟悉上述功能的示例:
differenceInWeeks: The left date must be the latest, else the method returns a -1.
DifferenceInWeeks :剩余日期必须是最晚的日期,否则该方法将返回-1。
console.log(differenceInWeeks(new Date(2015, 11, 22), new Date(2015, 11, 10))); // => 1
differenceInCalendarWeeks:
differentInCalendarWeeks :
console.log(differenceInCalendarWeeks(new Date(2015, 11, 25), new Date(2015, 11, 10))); // => 2
getWeek: We can also specify what day the week starts on through the
weekStartsOn
flag. It ranges from 0-6 with 0 as Sunday. The week number starts from 1.getWeek :我们还可以通过
weekStartsOn
标志指定星期weekStartsOn
。 它的范围是0-6,星期日是0。 周数从1开始。
console.log(getWeek(Date.now())); // => 30
console.log(getWeek(Date.now(), {weekStartsOn: 1})); // => 29
isThisWeek:
isThisWeek:
console.log(isThisWeek(Date.now())); // => true
eachWeekendOfYear
每年的每个周末
console.log(eachWeekendOfYear(Date.now()));
// => [Sat Jan 04 2020 00:00:00 GMT+0530 (India Standard Time)...]
常用助手和用例 (Common Helpers and use cases)
操作简单 (Simple operations)
Apart from the above we can add, subtract and get the difference on two Date
objects and their naming is extremely intuitive as illustrated below:
除上述内容外,我们还可以添加,减去和获取两个Date
对象的差值,其命名极为直观,如下所示:
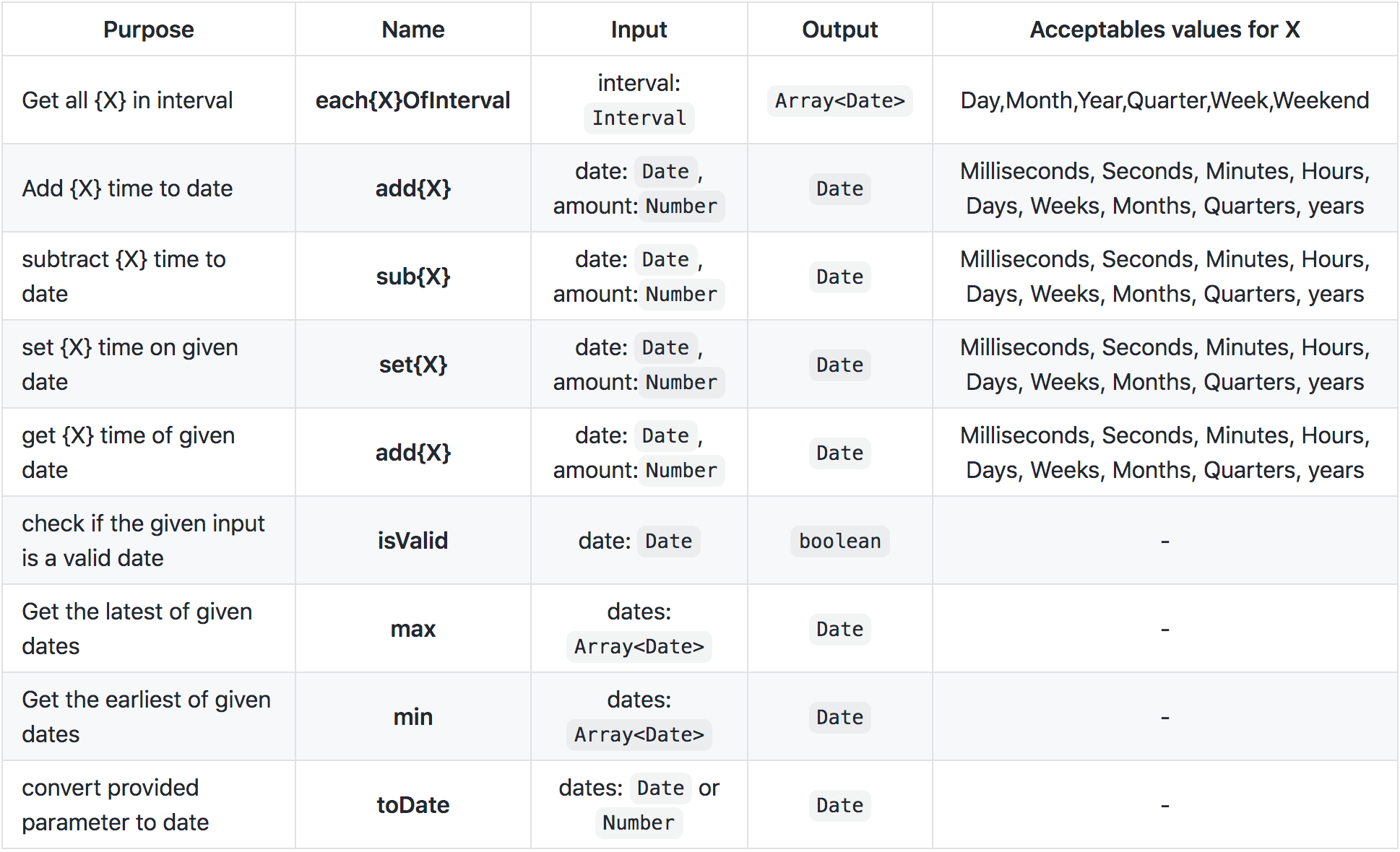
Some examples detailing the above methods are:
详细说明上述方法的一些示例是:
addQuarters(new Date("2020-01-01"), 3);
// => Thu Oct 01 2020 05:30:00 GMT+0530 (India Standard Time)max([new Date(2020, 2, 22), new Date(2020, 2, 2)]);
// => Sun Mar 22 2020 00:00:00 GMT+0530 (India Standard Time)
格式化日期时间 (Formatting date time)
One of the most common ask is to format date and time in a particular format that suits the application requirement. This also means that it is the most self-managed method which may go out of hand if the format were to change. The date-fns format
util has a wide variety of formatting options and the signature of the method is so easy that we can change the format with the update of a string.
最常见的要求之一是将日期和时间格式化为适合应用程序要求的特定格式。 这也意味着如果更改格式,这是最容易管理的方法,可能会失去控制。 date-fns format
实用程序具有多种格式设置选项,该方法的签名非常简单,我们可以通过更新字符串来更改格式。
format(date, formatOptions)
While the formatOptions
list is extensive some examples to cover a few of the most commonly used are:
尽管formatOptions
列表很广泛,但一些示例涵盖了一些最常用的示例:
const date = new Date("2020-07-19 18:32:00");format(date, "yy-MM-dd") => 20-07-19
format(date, "dd/MM/yyyy") => 19/07/2020
format(date, "do LLL yyyy") => 19th Jul 2020
format(date, "HH:mm:ss") => 18:32:00
format(date, "dd/MM/yyy hh:mm:ss x") => 19/07/2020 12:32:00 +0530
format(date, "dd/MM/yyy hh:mm:ss O) => 19/07/2020 06:32:00 GMT+5:30
format(new Date("2020-07-19 00:32:00"), "hh:mm:ss a") => 12:32:00 PM
format(new Date("2020-07-19 12:00:00"), "hh:mm:ss b") => 12:00:00 noon
format(new Date("2020-07-19 00:32:00"), "kk:mm:ss") => 24:32:00
format(new Date("2020-07-19 00:32:00"), "KK:mm:ss") => 00:32:00//second timestamp
format(date, "t") => 1595163720
//millisecond timestamp
format(date, "T") => 1595163720000/* some really interesting built-ins *///Localized date
format(date, "P") => 07/19/2020
format(date, "PPPP") => Sunday, July 19th, 2020
format(date, "PPPP G") => Sunday, July 19th, 2020 AD//Localized time
format(date, "p") => 6:32 PM
format(date, "pppp") => 6:32:00 PM GMT+05:30// Localized date and time
format(date, "PPPPpppp") => Sunday, July 19th, 2020 at 6:32:00 PM GMT+05:30
If you do not require such minute locale options while formatting then you can use lightFormat
. Its called the same way of format and does not consider certain options which relate to Era
, Quarter
, Extended year
.
如果在格式化时不需要这些分钟的区域设置选项,则可以使用lightFormat
。 它称为相同的格式,并且不考虑与Era
, Quarter
, Extended year
有关的某些选项。
lightFormat(new Date("2020-07-19 18:32:00"), "dd/MM/yy") => 19/07/20
lightFormat(new Date("2020-07-19 18:32:00"), "p") => throws RangeError
date-fns allows us to format intervals and durations through its formatDistance
and formatDuration
methods. The main difference between them is that the former formats the date in reference to a given date and the latter simply represents the duration in words. formatDistanceToNow
is the same as formatDistance
with the reference set to now()
date-fns允许我们通过其formatDistance
和formatDuration
方法来格式化间隔和持续时间。 它们之间的主要区别在于,前者参考给定的日期设置日期格式,而后者仅表示单词的持续时间。 formatDistanceToNow
与formatDistance
相同,引用设置为now()
formatDistance(Date.now(), sub(Date.now(), {hours: 4}));
// => About 4 hoursformatDistance(Date.now(), sub(Date.now(), {hours: 4}), {addSuffix: true});
// => in about 4 hoursformatDistance(Date.now(), sub(Date.now(), {seconds: 4}), { addSuffix: true, includeSeconds: true});
// => in less than 5 secondsformatDistanceStrict(Date.now(), sub(Date.now(), {seconds: 4}), { addSuffix: true, includeSeconds: true });
// => in 4 secondsformatDuration({days: 4, hours: 3}); // => 4 days 3 hoursformatDuration({days: 4, hours: 3}, {delimiter: "|"});
// => 4 days|3 hoursformatDuration({days: 4, hours: 3, months: 44}, {delimiter: "|", format: [ "months", "hours"]})
// => 44 months|3 hours
ISO格式 (ISO format)
The ISO date format is a standard way of representing date defined as YYYY-MM-DDTHH:mm:ss.sssZ
whereas UTC is a primary time standard. The ISO format always has the time standard as UTC and is signified by the Z
at the end. date-fns has methods to achieve all the above functionalities in the ISO format. Some are illustrated below:
ISO日期格式是表示日期的标准方式,定义为YYYY-MM-DDTHH:mm:ss.sssZ
而UTC是主要时间标准。 ISO格式的时间标准始终为UTC,并在末尾用Z
表示。 date-fns具有以ISO格式实现上述所有功能的方法。 如下图所示:
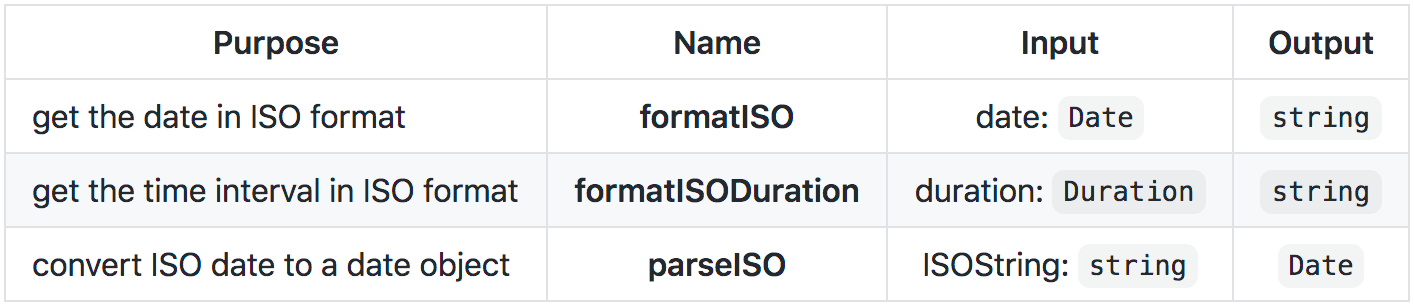
国际化 (Internationalization)
Most developers and apps face the issue of internationalization as it is not at the top of the TODO
list. date-fns has an accompanying module called date-fns-tz
for handling timezone formatting and conversions. This helper module can be installed by running npm install date-fns-tz
and also provides typescript support.
大多数开发人员和应用程序都面临国际化的问题,因为它不在TODO
列表的顶部。 date-fns具有一个称为date-fns-tz
的随附模块,用于处理时区格式和转换。 可以通过运行npm install date-fns-tz
来安装此帮助程序模块,并且还提供打字稿支持。
将分区时间转换为UTC和ISO (Converting zoned time to UTC and ISO)
If your system has the date-time stored in a particular timezone and have to convert it to UTC then the zonedTimeToUTC
method is the way to go. It takes a date and the timezone in the IANA
format of the input date time.
如果您的系统将日期时间存储在特定时区中,并且必须将其转换为UTC,则可以使用zonedTimeToUTC
方法。 它采用输入日期时间的IANA
格式的日期和时区。
zonedTimeToUTC(date, originTimeZone)examples:zonedTimeToUtc(new Date("Sun Jul 19 2020 14:41:43"), "America/Los_Angeles");
// => Mon Jul 20 2020 03:11:43 GMT+0530 (India Standard Time)zonedTimeToUtc(new Date("Sun Jul 19 2020 14:41:43"), "Asia/Bangkok");
// => Sun Jul 19 2020 13:11:43 GMT+0530 (India Standard Time)
将UTC转换为分区时间 (Converting UTC to zoned time)
Consider the use case where you are to send notifications or emails to your users, It is a hassle for them if you send the time and date details in UTC and they have to convert it to their local time. Hence another common use case is to convert the UTC time stored in the database to any timezone. This is achieved by utcToZonedTime
. This too takes a date and timezone in IANA
format, but the timezone represents the zone to which you want to convert it.
考虑一下您要向用户发送通知或电子邮件的用例,如果您使用UTC发送时间和日期详细信息并且他们必须将其转换为本地时间,这对他们来说很麻烦。 因此,另一个常见用例是将存储在数据库中的UTC时间转换为任何时区。 这是通过utcToZonedTime
实现的。 这也需要采用IANA
格式的日期和时区,但是时区表示要将其转换为的时区。
utcToZonedTime(date, destinationTimeZone)examples:console.log(utcToZonedTime(Date.now(), "Asia/Kolkata"));
// => Mon Jul 20 2020 02:06:59 GMT+0530 (India Standard Time)console.log(utcToZonedTime(Date.now(), "America/Los_Angeles"));
// Sun Jul 19 2020 13:36:59 GMT+0530 (India Standard Time)
评估指标 (Evaluation Metrics)
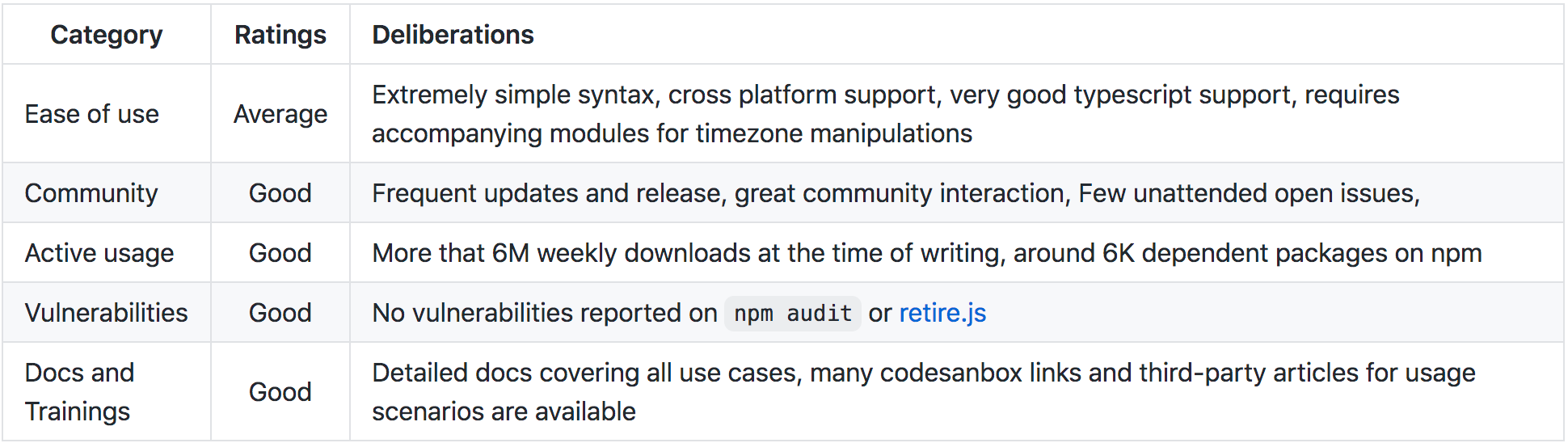
检查包装和一些阅读材料 (Check out the package and some reading materials)
Try these methods out at https://codesandbox.io/s/lyq47o17z9
https://en.wikipedia.org/wiki/List_of_tz_database_time_zones
https://zh.wikipedia.org/wiki/List_of_tz_database_time_zones
套餐的视频审查 (Video review of the package)
Video review of the package with interesting use cases and in-depth exploration of the features coming soon! For more related content, check out Unpackaged Reviews
对该视频进行了视频回顾,并附带了有趣的用例,并对即将推出的功能进行了深入探索! 有关更多相关内容,请查看未包装的评论
披露事项 (Disclosures)
The content and evaluation scores mentioned in this article/review is subjective and is the personal opinion of authors at Unpackaged Reviews based on everyday usage and research on popular developer forums. They do not represent any company’s views and are not impacted by any sponsorships/collaboration.
本文/评论中提到的内容和评估得分是主观的,是无包装评论中作者的个人观点,基于日常使用和对流行的开发者论坛的研究。 它们不代表任何公司的观点,也不受任何赞助/合作的影响。
date-fns日期格式化