Why Do We Need Global State ?
为什么我们需要全球状态?
If we want to pass a data to a component from another one, we have few options. If they have a relation then it’s easy, we just pass the data as a prop. But as the complexity grows it is harder to track down the component tree and props. What if the components don’t have a relation? That’s where global state comes into play. Imagine Redux global store as a component at higher level than all of your componets and you can pass and fetch any data from there via actions and reducers at any level. In this article we will show you how to add and use Redux in your React Native App.
如果我们想将数据从另一个组件传递到组件,则没有什么选择。 如果他们有关系,那很容易,我们只是将数据作为道具传递。 但是随着复杂性的增加,更难追踪组件树和道具。 如果组件之间没有关系怎么办? 这就是全球国家发挥作用的地方。 想象一下Redux全局存储是比所有组件都更高级别的组件,并且您可以通过任何级别的动作和化简从那里传递和获取任何数据。 在本文中,我们将向您展示如何在React Native App中添加和使用Redux。
How To Install React-Redux
如何安装React-Redux
Open your project folder in your terminal and run commands below.
在终端中打开项目文件夹,然后运行以下命令。
npm install redux react-redux redux-persist @react-native-community/async-storage --save
What Are Reducers and Actions ?
什么是减速器和动作?
A reducer is a function that determines changes to an application’s state. It uses the action it receives to determine this change.
减速器是一种确定对应用程序状态的更改的功能。 它使用收到的操作来确定此更改。
As you go deep in Redux, you’ll find out that all you’re going to do will be in reducers and actions. If you can grasp these concepts well, you’ll master redux in no time. Let’s start.
当您深入研究Redux时,您会发现您要做的只是减少操作。 如果您能很好地掌握这些概念,那么您将很快掌握redux。 开始吧。
Write Your First Reducer
写你的第一个减速器
Let’s create a folder named redux in project level and inside that two more folders named actions and reducers. In reducers folder create a file. Let’s name it userReducer. We’ll use this reducer to pass down a string(name) and a boolean(loggedIn) value.
让我们在项目级别创建一个名为redux的文件夹,在其中再创建两个名为action和reducer的文件夹。 在reducers文件夹中创建一个文件。 我们将其命名为userReducer 。 我们将使用此reducer传递一个字符串(名称)和一个布尔值(loggedIn)。
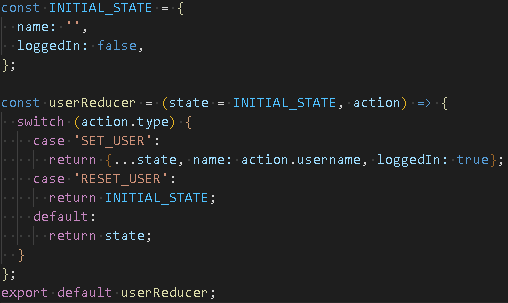
At the top we will define an Initial state for default values. After that we’ll define our reducer.
在顶部,我们将为默认值定义一个初始状态。 之后,我们将定义减速器。
Our reducer will have two parameters. First one is our state and the second one is the action that triggers our state changes. We change our state based on the type of action.
我们的减速器将有两个参数。 第一个是我们的状态,第二个是触发我们状态变化的动作。 我们根据操作类型来更改状态。
Write Your First Action
写你的第一个动作
In actions folder create a file named userActions. We have two actions, first one is for passing down the username to the reducer and second one is for resetting the reducer.
在动作文件夹中,创建一个名为userActions的文件。 我们有两个动作,第一个动作是将用户名传递给化简器,第二个是重置化简器。
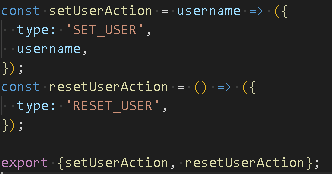
Root Reducer
根减少剂
If you have more than one reducer in your app then you need to create a root reducer to combine all your reducers. In reducers folder create another file named rootReducer.
如果您的应用程序中有多个Reducer,则需要创建一个根Reducer以结合所有Reducer。 在reducers文件夹中,创建另一个名为rootReducer的文件。
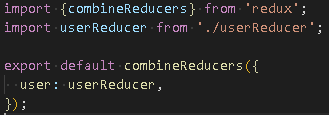
Note that when you export, left side of the object is how you will call the reducer state in components.
请注意,导出时,对象的左侧是如何在组件中调用reducer状态。
What is Redux-Persist ?
什么是Redux-Persist?
If you want to keep your data in redux even if you close your app you need redux-persist. In redux-persist you can decide which data you’ll keep and which data you’ll lose.
如果即使关闭应用程序也要保留数据在redux中,则需要redux-persist。 在redux-persist中,您可以决定保留哪些数据以及丢失哪些数据。
In redux folder create a file named store.
在redux文件夹中,创建一个名为store的文件。
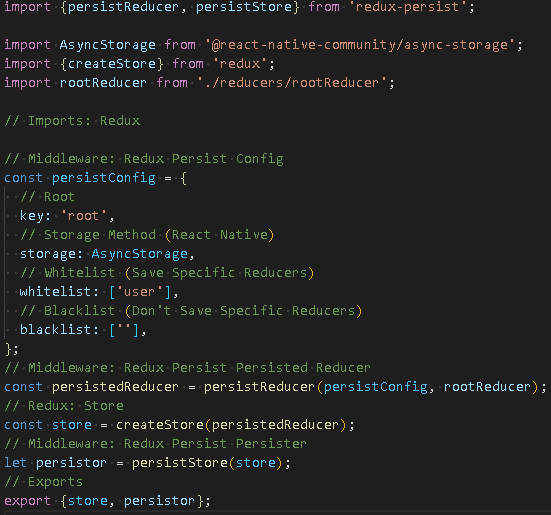
Now it’s time to wrap our app with the store we created.
现在是时候用我们创建的商店包装我们的应用程序了。
Let’s open our App.js file. To access redux data from all components we should wrap our app with Provider and give store as a prop.
让我们打开App.js文件。 要从所有组件访问redux数据,我们应该使用Provider包装我们的应用,并以store作为道具。
And for redux-persist, at a level below we wrap our app with PersistGate and give persistor as prop and set loading prop as null.
对于redux-persist,在以下级别,我们用PersistGate包装我们的应用程序,并将persister作为prop并将loading prop设置为null。
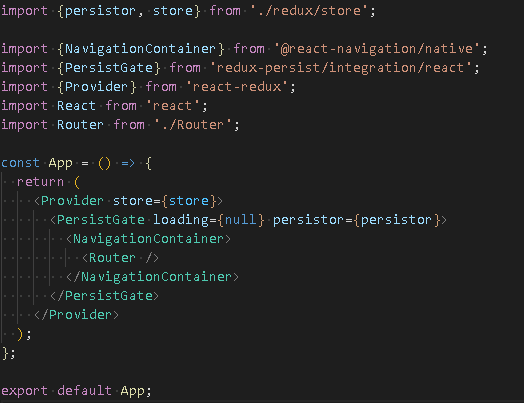
Now we’re ready to use our reducers and actions in our app.
现在,我们准备在我们的应用程序中使用我们的reducer和action。
So let’s use them.
因此,让我们使用它们。
What are MapStateToProps and MapDispachToProps ?
什么是MapStateToProps和MapDispachToProps?
How To Send Data To Redux Store?
如何将数据发送到Redux Store?
In this example we have a text input and a button for user to type their name and proceed with their name. We have a component called landingPage. In this file before we use actions we have to import them.
在此示例中,我们有一个文本输入和一个供用户键入其名称并继续其名称的按钮。 我们有一个名为landingPage的组件。 在此文件中,我们在使用操作之前必须先导入它们。

For triggering the action we need to use mapDispatchToProps function and to get the data from redux store we need to use mapStateToProps function.
为了触发动作,我们需要使用mapDispatchToProps函数,并且要从redux存储中获取数据,我们需要使用mapStateToProps函数。
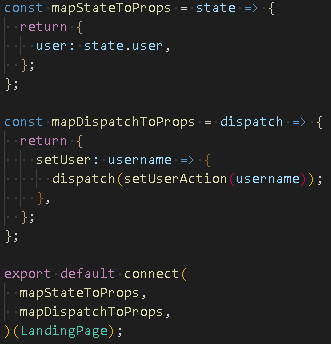
Dispatch is a function that will trigger our actions. We pass a parameter to the reducer.The reducer will use that parameter once the action is triggered.
调度功能将触发我们的行动。 我们将参数传递给化简器。一旦动作被触发,化简器将使用该参数。
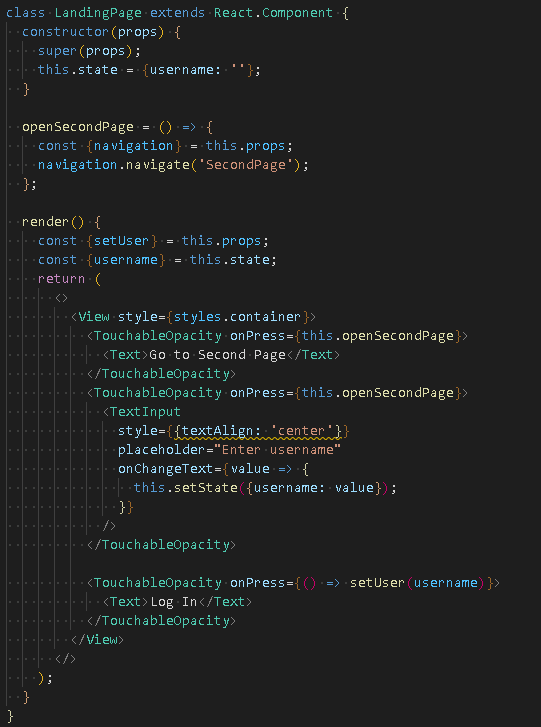
Using action is quite easy. We just need to define it from props and use it as a function. As you can see in the image above we pass the username state as parameter and call action. This will trigger our action and go to the reducer with the username data.
使用动作非常容易。 我们只需要从props中定义它,并将其用作函数即可。 如上图所示,我们将用户名状态作为参数传递并调用操作。 这将触发我们的操作,并使用用户名数据转到化简器。
How To Receive Data From Redux Store?
如何从Redux Store接收数据?
We have a second page to show user name in another component.
我们还有第二页显示另一个组件中的用户名。
For getting the data from redux store we need to use mapStateToProps function. We will get user from state of our store.
为了从redux存储中获取数据,我们需要使用mapStateToProps函数。 我们将从商店的状态获取用户。
And for logout we also define resetUserAction.
对于注销,我们还定义了resetUserAction。
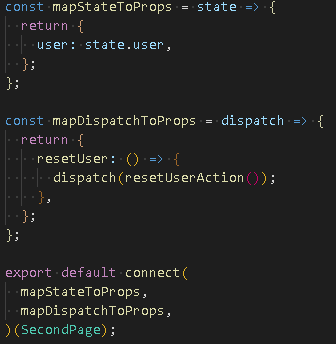
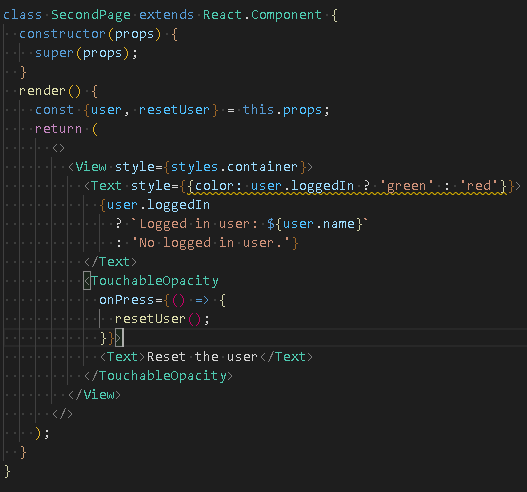
Using data from redux store is also quite easy. Like action; just define it from props and use it wherever you want.
使用redux存储中的数据也很容易。 喜欢动作; 只需从道具中定义它,然后在任何需要的地方使用它。
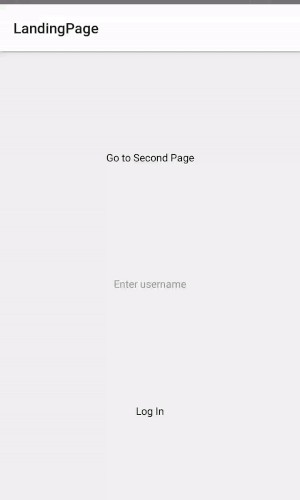
Conclusion:
结论:
We tried to show and explain how to use Redux in React-Native. Redux may take some time to learn but with practice and time you will master your skills. For more information use can check the Redux documents here.
我们试图展示和解释如何在React-Native中使用Redux。 Redux可能需要花费一些时间来学习,但是通过实践和时间,您将掌握自己的技能。 要获取更多信息,可以在此处查看Redux文档。
You can clone this project from here via github.
您可以通过github从此处克隆此项目。
MTA Team:
MTA团队:
Ali Gümüş Tosun - Semih Sevincli - Aslancan Yılmaz - Burak Arıcı
AliGümüşTosun - Semih Sevincli - AslancanYılmaz - BurakArıcı
LinkedIn Accounts:
领英帐户:
Ali Gümüş Tosun - Semih Sevinçli - Aslancan Yılmaz - Burak Arıcı
翻译自: https://medium.com/@MtaTeam/adding-redux-to-your-react-native-app-a1eeb6ee20b3