I know this article title will be surprising to you. That’s why you are here, right? 😀😀
我知道这篇文章标题会让您感到惊讶。 那就是为什么你在这里,对吗? 😀😀
Yes, manipulating Arrays length property value has effects on the elements in the Array. Let’s see how.
是的,操纵Arrays的length属性值会影响Array中的元素。 让我们看看如何。
EcmaSpec上的数组 (Array on EcmaSpec)
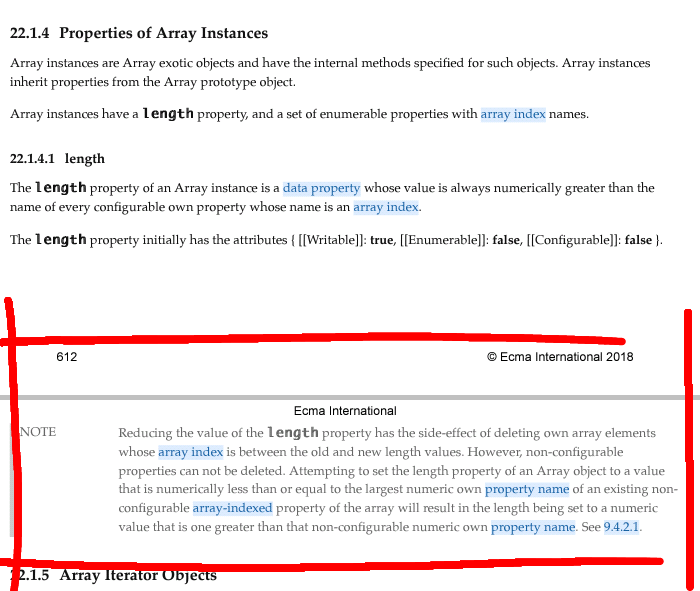
Looking at the Ecmaspec on Arrays, we can deduce that Array is a number-indexed Object in JS. It has a length property which indicates the number of the number-indexed properties on the Array.
查看数组上的Ecmaspec,我们可以推断出Array是JS中以数字索引的对象。 它具有length属性,该属性指示Array上数字索引属性的数量。
Number-indexed properties mean that the contents of an array are set by the index number as properties to the Array instance
数字索引属性意味着数组的内容由索引号设置为Array实例的属性
const names = ["Nnamdi", "Chidume"]
The names is an Array instance with elements “Chidume”, “Nnamdi”. These elements are set by their index number as property to the names instance. So we can refer to the elements by their index number:
名称是一个包含元素“ Chidume”,“ Nnamdi”的Array实例。 这些元素通过它们的索引号设置为names实例的属性。 因此,我们可以通过元素的索引号来引用它们:
names[0] // Nnamdi
names[1] // Chidume
So
所以
const names = ["Nnamdi", "Chidume"]
can be written like this:
可以这样写:
const names = {
0: "Nnamdi",
1: "Chidume"
}
just like an Object. Array is an object but their properties are numbers while Object's properties are set by names.
就像一个对象。 数组是一个对象,但是它们的属性是数字,而对象的属性是通过名称设置的。
Arrays have a length property that holds the number of the number-indexed properties on it. So, our names array will have a length property of 2 because names
have two elements.
数组具有一个length属性,该属性保存有数字索引属性。 因此,我们的名称数组的length属性为2,因为names
包含两个元素。
names.length // 2
the length of an array is by one-unit greater than the number-indexed properties in the array.
数组的长度比数组中的数字索引属性大一个单位。
Now, if we reduce the value of the length property, the elements of the array will be reduced.
现在,如果我们减少length属性的值,则数组的元素将减少。
For example:
例如:
The names array const names =["Nnamdi","Chidume"]
has a length of 2. If we set the length to be 1, the contents of the names array will be deleted to the value of the length property set.
名称数组const names =["Nnamdi","Chidume"]
的长度为2。如果将长度设置为1,则名称数组的内容将被删除为length属性集的值。
const names =["Nnamdi","Chidume"]l(names)names.length = 1l(names)
Output:
输出:
[ 'Nnamdi', 'Chidume' ]
[ 'Nnamdi' ]
See after setting the names
length property to 1, the "Chidume" element was removed from the names array. See, that JS pops off the elements from the right-hand side until the number of the number-indexed elements equals the value of the length set.
在将names
长度属性设置为1之后,请参见,从名称数组中删除了“ Chidume”元素。 可以看到,JS从右侧弹出元素,直到编号索引的元素的数量等于长度设置的值为止。
If we set the length to zero:
如果我们将长度设置为零:
const names = [ "Nnamdi", "Chidume" ]l(names)names.length = 0l(names)
Output:
输出:
[ 'Nnamdi', 'Chidume' ]
[]
We will have an empty array. All the elements in the names array were deleted.
我们将有一个空数组。 名称数组中的所有元素均已删除。
So we see that reducing the Array length makes the JS engine rush to delete the elements in the array so they tally up to the length value.
因此,我们看到减小Array的长度会使JS引擎急于删除数组中的元素,从而使它们符合长度值。
如何增加长度值? (How about increasing the length value?)
Good question, what happens when we increase the length value?
好问题,当我们增加长度值时会发生什么?
Increasing the length value will make the JS engine add empty items to the array.
增加长度值将使JS引擎将空项目添加到数组中。
const l=console.log
const names =["Nnamdi","Chidume"]l(names)names.length = 3l(names)
The names array has two elements, and the length is two. See set the length property to be 3 which is more than the length of the array.
名称数组包含两个元素,长度为两个。 请参见将length属性设置为3,该属性大于数组的长度。
Output:
输出:
[ 'Nnamdi', 'Chidume' ]
[ 'Nnamdi', 'Chidume', <1 empty item> ]
See, JS added an empty item to the names array so the length will add up to 3.
看,JS在名称数组中添加了一个空项目,因此长度总计为3。
If we set a length that is n-elements more than the original length, JS will add n-empty items more to the array.
如果我们设置的长度比原始长度大n个元素,那么JS会将更多的n个空项目添加到数组中。
const names =["Nnamdi","Chidume"]l(names)names.length = 5l(names)
See, we set length that is 3-elements more than the original length. JS will add 3 empty items to names array so it will compensate for the new length value.
瞧,我们将长度设置为比原始长度大3个元素。 JS将在名称数组中添加3个空项,因此它将补偿新的长度值。
Output:
输出:
[ 'Nnamdi', 'Chidume' ]
[ 'Nnamdi', 'Chidume', <3 empty items> ]
The empty items are undefined.
空项目是未定义的。
Lets’ try referencing one:
让我们尝试引用一个:
const names =["Nnamdi","Chidume"]l(names)names.length = 5l(names)l(names[2])
Output:
输出:
[ 'Nnamdi', 'Chidume' ]
[ 'Nnamdi', 'Chidume', <3 empty items> ]
undefined
We referenced index 2, we know it will contain an empty item. See, it logged undefined. Empty items are all undefined primitives.
我们引用了索引2,我们知道它将包含一个空项目。 看,它记录了未定义的。 空项目都是未定义的原语。
长度值操作会影响不可配置的属性吗? (Can non-configurable properties be affected by length value manipulation?)
Non-configurable properties are not affected.
不可配置的属性不受影响。
Let’s see an example:
让我们来看一个例子:
const names = ["Nnamdi", "Chidume"]
Object.defineProperty(names, 2, {
value: "David",
writable: false,
configurable: false
})
We added a third element using the Object.defineProperty(...)
to the names array because Arrays are indexed by numbers, we used the number 2
as property to add to the names Array. We set the value to "David", and make it non-configurable and non-writable.
我们使用Object.defineProperty(...)
向名称数组添加了第三个元素,因为数组是由数字索引的,我们使用数字2
作为属性来添加到名称数组。 我们将值设置为“ David”,并使它不可配置且不可写。
Non-configurable and non-writable means that the property cannot be overwritten.
不可配置和不可写意味着该属性不能被覆盖。
Now, if we reduce the length of the names array:
现在,如果我们减少名称数组的长度:
...l(names)names.length = 2l(names)
We reduced the length to 2. Normally, this should remove the “David” element to compensate for the new length, but because we have a non-configurable element “David”, it will not be removed.
我们将长度减小为2。通常,应删除“ David”元素以补偿新的长度,但是由于我们有不可配置的元素“ David”,因此不会将其删除。
Output:
输出:
[ 'Nnamdi', 'Chidume', 'David' ]
[ 'Nnamdi', 'Chidume', 'David' ]
The names array sill remains unchanged.
名称数组基石保持不变。
If we try increasing the length value
如果我们尝试增加长度值
...
names.length = 4
It will work normally. Empty items will be added to the names array:
它将正常工作。 空项目将添加到名称数组:
[ 'Nnamdi', 'Chidume', 'David', <1 empty item> ]
If we push new elements:
如果我们推新元素:
const names = ["Nnamdi", "Chidume"]
Object.defineProperty(names, 2, {
value: "David",
writable: false,
configurable: false
})names.push("Philip")
We pushed a new element “Philip”, it will have index 3
. names will have length 4.
我们推送了一个新元素“ Philip”,它将具有索引3
。 名称的长度为4。
If we try to reduce the names
length property to 2
如果我们尝试将names
length属性减少为2
...
l(names)names.length = 2l(names)
This should reduce the names array to:
这应该将名称数组减少为:
[ 'Nnamdi', 'Chidume', 'David', 'Philip' ] |
|
v
[ 'Nnamdi', 'Chidume' ]
But because the index 2 (“David”) is non-configurable, the names
reduction will stop at index 2, the "David" will not be removed, only "Philip" will be removed.
但是因为索引2(“ David”)是不可配置的,所以names
减少将在索引2处停止,“ David”将不会被删除,只有“ Philip”会被删除。
Output:
输出:
[ 'Nnamdi', 'Chidume', 'David', 'Philip' ]
[ 'Nnamdi', 'Chidume', 'David' ]
用例 (Use Cases)
One of the most useful uses cases I found out when using the length property, was when I wanted to copy an array to another array.
我在使用length属性时发现的最有用的用例之一是,我想将一个数组复制到另一个数组。
function copyTo(source, destination) {
for(let i =0: i< source.length;i++) {
destination[i] = source[i]
}
return destination
}
I found out that if the source array has elements more than the destination array I will have some uncopied elements left in the source,
我发现如果源数组中的元素多于目标数组,那么我的源中将剩下一些未复制的元素,
source = [1,2,3,4]
destination = [5,6]// after copyingdestination = [1,2,3]
and if the source array has elements less than the destination array, I will have unwanted elements still left in the destination array.
如果源数组中的元素少于目标数组,那么我将有多余的元素残留在目标数组中。
destination = [1,2,3,4]
source = [5,6]// after copying
destination = [5,6,3,4]
I used the length property manipulation to set it right.
我使用length属性操作对其进行了设置。
I just set the destination to the source array, so JS will either reduce or add more elements for me on the destination,
我只是将目标设置为源数组,因此JS会在目标上为我减少或添加更多元素,
function copyTo(source, destination) {
destination.length = source.length
for(let i =0: i< source.length;i++) {
destination[i] = source[i]
}
return destination
}
the destination gets trimmed down to the number of elements in the source array. So I set them from the source to the destination without errors. Perfect!!!
目标将被精简为源数组中的元素数。 因此,我将它们设置为从源到目标,没有错误。 完善!!!
结论 (Conclusion)
JS is awesome and tricky at the same time.
JS既出色又棘手。
Thanks for stopping by my little corner of the web. I think you’ll love my email newsletter about programming advice, tutoring, tech, programming and software development. Just sign up below:
感谢您停在我的网络小角落。 我想您会喜欢我的电子邮件通讯,其中涉及编程建议,辅导,技术,编程和软件开发。 只需在下面注册:
Follow me on Twitter.
在 Twitter上 关注我 。