javascript 堆
A stack is a basic linear data structure, in which the insertion and deletion of items happens at one end called top of the stack. It follows the order of LIFO (last in first out) or FILO (first in last out), the last element added to the stack will be the first element removed from the stack. The classic real-life example for stack is the stack of plates in a buffet, the plate at the top is always the first one to be removed. The stack data structure is commonly used in managing function invocations, undo/redo, and routing.
一叠 是一种基本的线性数据结构,其中项目的插入和删除发生在称为堆栈顶部的一端。 它遵循LIFO(先进先出)或FILO(先进先出)的顺序,添加到堆栈中的最后一个元素将是从堆栈中删除的第一个元素。 现实生活中经典的堆叠示例是自助餐中的盘子堆叠,顶部的盘子始终是第一个要卸下的盘子。 堆栈数据结构通常用于管理功能调用,撤消/重做和路由。
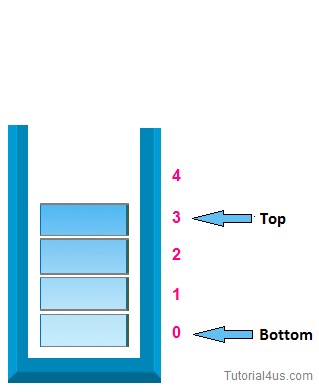
There is no built-in stack data structure in JavaScript, but it is relatively simple to implement.
JavaScript中没有内置的堆栈数据结构,但是实现起来相对简单。
阵列实施 (Array Implementation)
Even though there is no built-in stack data structure in JavaScript, we use array data structure with the help of the push()
, pop()
, unshift()
, and shift()
methods to create stacks. Amongst these methods, unshift()
and shift()
need to reindex the elements in the array, thus it increases the time complexity. So push()
and pop()
are our first choices. To understand the array implementation of stacks better, let’s apply it to the leetcode problem below.
即使JavaScript中没有内置的堆栈数据结构,我们也可以在push()
, pop()
, unshift()
和shift()
方法的帮助下使用数组数据结构来创建堆栈。 在这些方法中, unshift()
和shift()
需要重新索引数组中的元素,因此会增加时间复杂度。 因此push()
和pop()
是我们的首选。 为了更好地理解堆栈的数组实现,我们将其应用于下面的leetcode问题 。
Given a string containing just the characters '('
, ')'
, '{'
, '}'
, '['
and ']'
, determine if the input string is valid.
给定仅包含字符'('
, ')'
, '{'
, '}'
, '['
和']'
的字符串,请确定输入字符串是否有效。
An input string is valid if:1. Open brackets must be closed by the same type of brackets.2. Open brackets must be closed in the correct order.
如果满足以下条件,则输入字符串有效:1。 开括号必须用相同类型的括号封闭2。 开括号必须以正确的顺序关闭。
Note that an empty string is also considered valid.
请注意,空字符串也被视为有效。
Example 1:Input: "()"
Output: true
示例1:输入: "()"
输出: true
Example 2:Input: "()[]{}"
Output: true
示例2:输入: "()[]{}"
输出: true
Example 3:Input: "(]"
Output: false
示例3:输入: "(]"
输出: false
Example 4:Input: "([)]"
Output: false
示例4:输入: "([)]"
输出: false
Example 5:Input: "{[]}"
Output: true
示例5:输入: "{[]}"
输出: true
Usually, when we are dealing with valid parentheses problems, we use stack data structure as part of the solution.
通常,当我们处理有效的括号问题时,我们将堆栈数据结构用作解决方案的一部分。
链表实现 (Linked List Implementation)
To implement stacks with a linked list, we create Stack
class and Node
class.
为了实现具有链表的Stack
,我们创建了Stack
类和Node
类。
How can we push a new node to the stack? First, we need a value for creating a new node. If there are no nodes in the stack, set the first and last property to be the newly created node. If there is at least one node, create a variable that stores the current first property on the stack and reset the first property to be the newly created node. Set the next property on the new first property to be the previously created variable. Don’t forget to increment the size of the stack by one.
我们如何将新节点推入堆栈? 首先,我们需要一个用于创建新节点的值。 如果堆栈中没有节点,则将first和last属性设置为新创建的节点。 如果有至少一个节点,请创建一个变量,该变量将当前的第一个属性存储在堆栈上,并将第一个属性重置为新创建的节点。 将新的第一个属性上的下一个属性设置为先前创建的变量。 不要忘记将堆栈的大小增加一。
How do we pop the node? If there are no nodes in the stack, it returns null. If there are nodes in the stack, create a temporary variable to store the first property on the stack. If there is only one node, set the first and last property to be null. If there is more than one node, set the first property to be the next property on the current first. Decrement the size by one and return the value of the node that was removed.
我们如何弹出节点? 如果堆栈中没有节点,则返回null。 如果堆栈中有节点,请创建一个临时变量以将第一个属性存储在堆栈中。 如果只有一个节点,则将first和last属性设置为null。 如果有多个节点,则将第一个属性设置为当前第一个属性的下一个属性。 将大小减一,然后返回已删除节点的值。
堆栈大O (The Big O of Stacks)

翻译自: https://medium.com/@g.aierken/stacks-in-javascript-d2f0e4404eac
javascript 堆