lerna
I showed you the best practices to create a JavaScript SDK in last article. One of the best practices that I described was the importance of controlling the package’s version and share the codebase between them.
在上一篇文章中,我向您展示了创建JavaScript SDK的最佳实践。 我描述的最佳实践之一是控制程序包版本并在它们之间共享代码库的重要性。
In this article, I’ll show how monorepo can help you to achieve those principles.
在本文中,我将展示monorepo如何帮助您实现这些原则。
什么是Monorepo? (What is Monorepo?)
In a nutshell, monorepo is a strategy where you will have all the application’s code in the same repository.
简而言之,monorepo是一种策略,您可以在同一存储库中拥有所有应用程序的代码。
There are some cases that a monorepo fits well. For example, when you have an application with a mobile app, backend, frontend, and so on. With monorepo, we will have a folder for each of these parts and share code between them.
在某些情况下,monorepo非常合适。 例如,当您的应用程序带有移动应用程序时,后端,前端等等。 使用monorepo,我们将为每个部件提供一个文件夹,并在它们之间共享代码。
Another suitable case for this strategy is when you are creating a lib. The Angular framework is a famous example of that and uses this project structure.
此策略的另一种合适情况是在创建库时。 Angular框架就是一个著名的例子,并使用此项目结构。
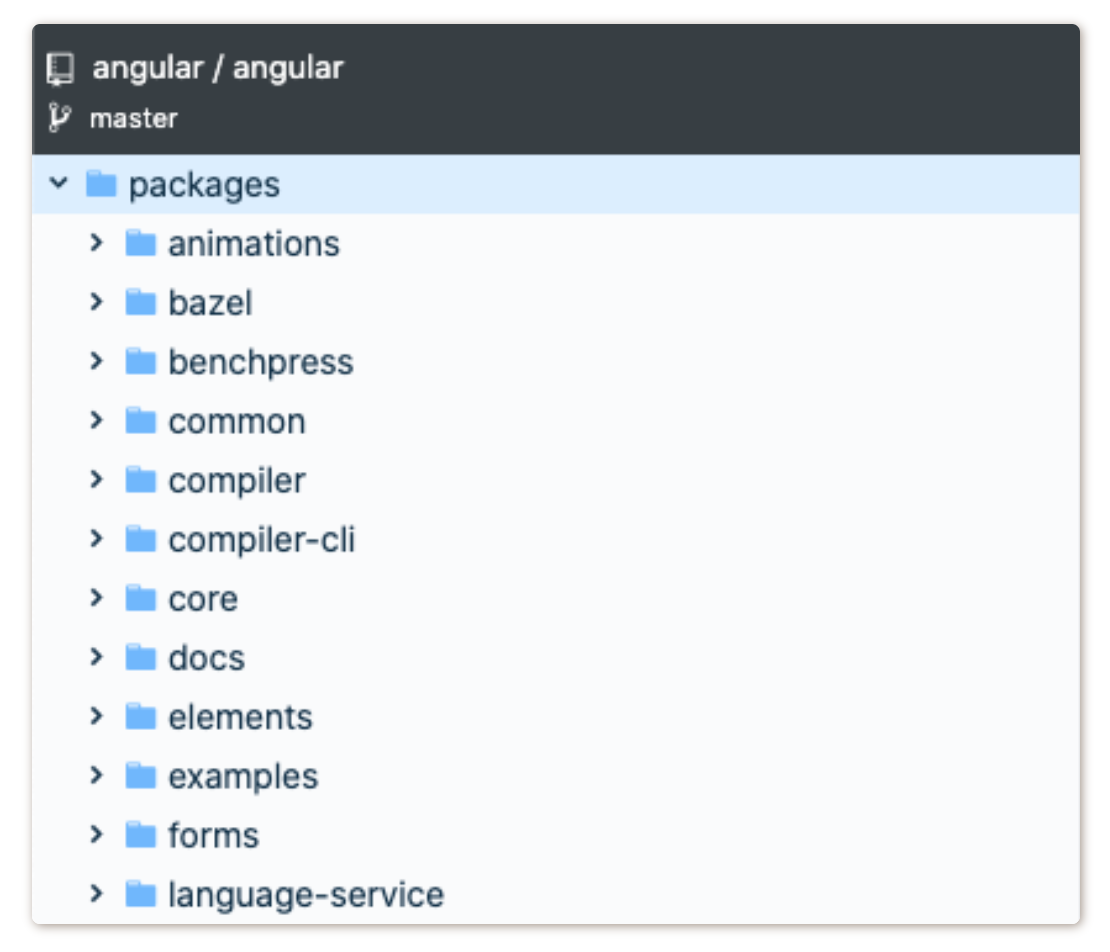
The project has a well-done decoupling with a lean core separated from the other modules as animations, forms, router, etc. In this Angular project in specific, all the modules have the same version number.
该项目具有良好的去耦,其精益核心与其他模块(如动画,表单,路由器等)分开,在此Angular项目中,所有模块的版本号均相同。
But with monorepo, you can have unique versions for each module as well.
但是,使用monorepo,每个模块也可以具有唯一的版本。
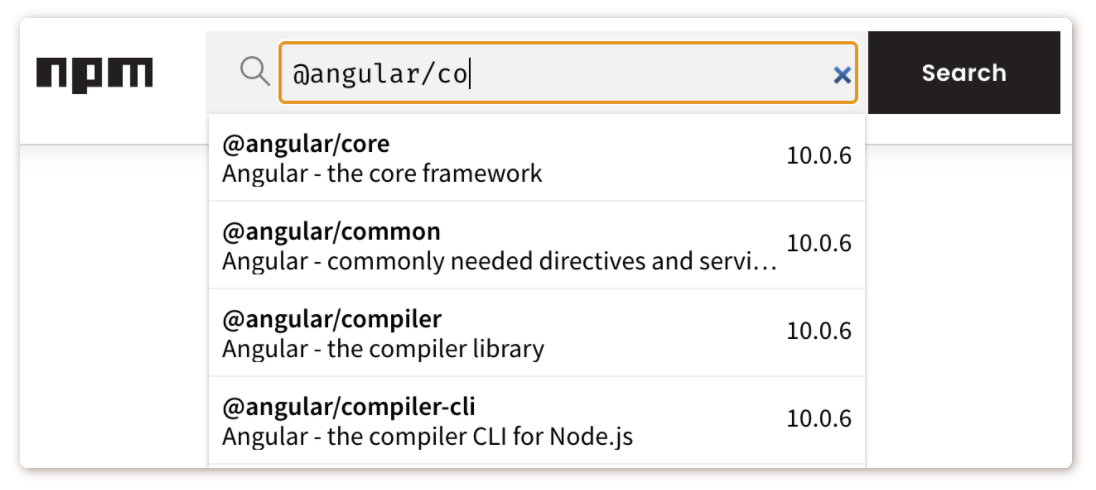
After publishing this monorepo to NPM, we will have separate packages for each model.
在将此Monorepo发布到NPM之后,我们将为每个模型提供单独的软件包。
三大好处 (Three Main Benefits)
♻️ Ease of code reuse — When we have a project with many modules, but with the same codebase, it is good to have all these modules altogether in a monorepo. For example, an SDK that has a package for different frameworks’ components.
轻松实现代码重用 —当我们的项目中有许多模块,但是具有相同的代码库时,最好将所有这些模块都放在一个monorepo中。 例如,SDK具有用于不同框架组件的软件包。
📦 Simplified dependency management — In a multiple repository environment where many projects depend on a third-party dependency, that dependency might be downloaded or built many times. In a monorepo, the build can be easily optimized, as referenced dependencies all exist in the same codebase. You can achieve it using Yarn Workspaces.
📦简化的依赖关系管理 -在许多项目依赖第三方依赖项的多存储库环境中,该依赖项可能会被多次下载或构建。 在monorepo中,可以轻松优化构建,因为引用的依赖项都存在于同一代码库中。 您可以使用Yarn Workspaces实现它。
🚀 Unified versioning — We have one source of truth for all packages. It’s a good practice when you have an API with sub-modules, it will allow you to identify whether the module you are using came from the same codebase. For example, when a new version of Angular (core package) is published, all “sub-packages” will have the same version.
🚀统一版本控制 -我们为所有软件包提供了一个真实的来源。 当您拥有带有子模块的API时,这是一个好习惯,它将使您能够确定所使用的模块是否来自同一代码库。 例如,当发布新版本的Angular(核心软件包)时,所有“子软件包”将具有相同的版本。
让我们创建我们的Monorepo (Let’s Create Our Monorepo)
For our example, I will create an SDK that has support to some frameworks components (React, Vue, and Angular).
对于我们的示例,我将创建一个支持某些框架组件(React,Vue和Angular)的SDK。
入门 (Getting Started)
First, we will execute the following command lines.
首先,我们将执行以下命令行。
$ mkdir my-sdk && cd $_
$ npx lerna init
npx lerna init
will create the Lerna configuration file as well as the package.json
file and the packages
folder:
npx lerna init
将创建Lerna配置文件以及package.json
文件和packages
文件夹:
.
├── lerna.json
├── package.json
└── packages
We’ll modify the lerna.json
and package.json
files to support Yarn Workspaces (available since yarn@0.27+).
我们将修改lerna.json
和package.json
文件以支持Yarn工作区 (自yarn@0.27+起可用)。
For the lerna.json
file will add the following properties:
对于lerna.json
文件,将添加以下属性:
"npmClient: "yarn"
: to run all commands with yarn"npmClient: "yarn"
:使用yarn运行所有命令"useWorkspaces": true
: to enable integration with workspaces"useWorkspaces": true
:启用与工作空间的集成
For the package.json
file will add the following property:
对于package.json
文件,将添加以下属性:
"workspaces": ["packages/*"]
: where the modules/packages are located"workspaces": ["packages/*"]
:模块/软件包所在的位置
创建包 (Creating the Packages)
Now it’s time to create our packages that will be orchestrated by Lerna and Yarn. In this example, we will create four packages: core
, react
, vue
, and angular
.
现在是时候创建由Lerna和Yarn精心策划的程序包了。 在此示例中,我们将创建四个包: core
, react
, vue
和angular
。
$ mkdir -p packages/{core,react,vue,angular}
Now we have this structure:
现在我们有了这个结构:
.
├── lerna.json
├── package.json
└── packages
├── angular
├── core
├── react
└── vue
The core
folder will contain our shared code between our packages. We can have our server integrations, interface definitions, base classes, and utilities for building the SDK components.
core
文件夹将包含我们在软件包之间的共享代码。 我们可以使用服务器集成,接口定义,基类和实用程序来构建SDK组件。
The other folders will have our components’ particular behavior. Each package will have the package.json
file with its dependencies.
其他文件夹将具有我们组件的特定行为。 每个软件包都将具有package.json
文件及其相关性。
For instance, you can follow these articles to create the component for each framework in this example.
例如,在本示例中,您可以按照这些文章为每个框架创建组件。
Pro tip: remove all
node_modules
folders inside the packages and then runyarn install
in the root folder. It will install the dependencies for all packages. We will only have anode_modules
folder inside a package, whether we have a version conflict between the packages dependencies.提示 :删除软件包中的所有
node_modules
文件夹,然后在根文件夹中运行yarn install
。 它将安装所有软件包的依赖项。 无论包依赖关系之间是否存在版本冲突,我们在包内都只会有一个node_modules
文件夹。
测试并构建所有程序包 (Testing and Building All Packages)
Now that we have all packages created, we can build our whole repo. We will use Lerna to execute the scripts inside our packages.
现在我们已经创建了所有软件包,我们可以构建我们的整个仓库。 我们将使用Lerna在程序包中执行脚本。
First, we need to create a build script inside each `package.json`. For example, if you followed the instructions to create a React lib above the build and test script inside the packages/react/package.json
must be:
首先,我们需要在每个package.json内部创建一个构建脚本。 例如,如果您按照说明在packages/react/package.json
内的构建和测试脚本之上创建React库,则必须为:
{ "name": "my-sdk-component",
"version": "1.0.0",
...
"scripts": {
"build": "microbundle-crl --no-compress --format modern,cjs",
"test": "run-s test:unit test:lint test:build",
...
},
...
}
To run all the packages tests and build them, we will define theses scripts in our root package.json
.
要运行所有程序包测试并构建它们,我们将在根package.json
定义这些脚本。
Now to test and build your entire repo just run:
现在要测试并构建整个仓库,只需运行:
$ yarn test
$ yarn build
结论 (Conclusion)
Now we know how to create, test, and build our monorepo. In the next article, I’ll show you how to publish those packages using the GitHub Actions CI/CD.
现在我们知道了如何创建,测试和构建我们的monorepo。 在下一篇文章中,我将向您展示如何使用GitHub Actions CI / CD发布这些软件包。
翻译自: https://medium.com/swlh/creating-testing-and-building-a-monorepo-with-lerna-and-yarn-1ced540e04c7
lerna