javascript继承
Hi, and welcome to my first multi-part blog post! This week and next I’ll be talking about two types of inheritance in JavaScript: inheritance through prototypes and constructor functions, and inheritance through classes. These articles are for anyone that wants to gain a better understanding of these topics, as classes are a basic programming paradigm that’s important to have a strong grasp on, and prototypes are important to understanding JavaScript. These articles are also for myself; practicing explaining concepts in layman’s terms is imperative to solidifying one’s understanding of those concepts. I hope that by explaining these concepts to you I will better understand them myself. I won’t be covering everything about prototypes and inheritance in this article, but I’m confident readers will finish having learned a thing or two about JavaScript.
嗨,欢迎来到我的第一篇多篇博客文章! 本周和下周,我将讨论JavaScript中的两种继承:通过原型和构造函数的继承,以及通过类的继承。 这些文章适用于希望更好地理解这些主题的任何人,因为类是一种基本的编程范例,必须牢牢掌握,而原型对于理解JavaScript也很重要。 这些文章也适合我自己; 练习用外行的术语解释概念对于巩固人们对这些概念的理解是必不可少的。 我希望通过向您解释这些概念,我自己会更好地理解它们。 我不会在本文中介绍有关原型和继承的所有内容,但是我相信读者将学到一两个关于JavaScript的知识。
As always, I’d love to hear some feedback. Comment here, or reach out to me through email, Twitter, GitHub, or my LinkedIn. As of the writing of this article I am actively looking for work. Don’t hesitate to reach out if you’d like to get in touch concerning an employment opportunity.
和往常一样,我很想听听一些反馈。 在此处发表评论,或通过电子邮件 , Twitter , GitHub或我的LinkedIn向我联系。 在撰写本文时,我正在积极寻找工作。 如果您想就就业机会取得联系,请随时与我们联系。
JavaScript的继承 (Inheritance in JavaScript)
Inheritance in JavaScript refers to the ability of objects to inherit properties from other objects. Objects in JavaScript belong to what is called the prototype chain. Each object in JavaScript has a property that chains it to another object, its prototype. The prototype chain ends with null, which is what the Object class inherits from.
JavaScript中的继承是指对象从其他对象继承属性的能力。 JavaScript中的对象属于所谓的原型链。 JavaScript中的每个对象都具有一个将其链接到另一个对象(即原型)的属性。 原型链以null结束,这是Object类继承的对象。
When you make a new object in JavaScript, that object inherits certain properties from the Object class. We can see what new objects inherit from the Object class by typing the following in our console:
当您在JavaScript中创建新对象时,该对象将从Object类继承某些属性。 通过在控制台中键入以下内容,我们可以看到哪些新对象从Object类继承:
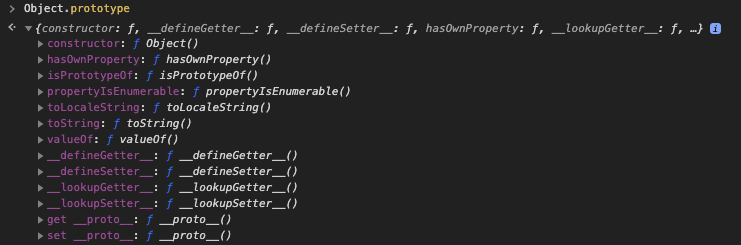
Therefore, we can see that all objects that inherit from the Object class will have the properties constructor(), hasOwnProperty(), etcetera. We can define our own constructor function that creates instances of the Object class, but with their own properties:
因此,我们可以看到从Object类继承的所有对象都将具有构造函数(),hasOwnProperty()等属性。 我们可以定义我们自己的构造函数,该函数创建Object类的实例,但是具有它们自己的属性:
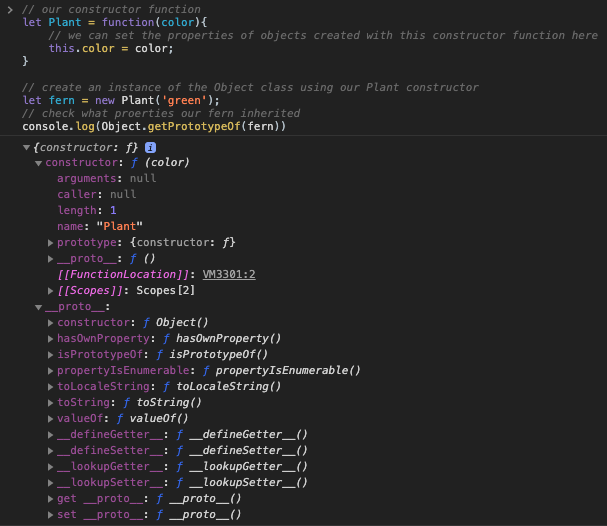
Here we defined the Plant constructor function. This constructor function takes one argument, color, of which each instance of Plant will have its own value (defined as this.color in the constructor function). Calling Object.getPrototypeOf(fern) (which is more or less the same as fern.__proto__) returns its constructor function (constructor) and the properties it has inherited (__proto__).
在这里,我们定义了Plant构造函数。 该构造函数使用一个参数color,每个Plant实例将具有自己的值(在构造函数中定义为this.color )。 调用Object.getPrototypeOf(fern) (与fern .__ proto__大致相同)将返回其构造函数( Constructor )及其继承的属性( __proto__ )。
This is where the fun begins: now we can add properties to the Plant constructor functions prototype property that will be inherited by all instances of the Plant constructor function, regardless of when they were made. Let’s add a function property to the Plant constructor function’s prototype property:
这就是乐趣的开始:现在,我们可以将属性添加到Plant构造函数的原型属性,该属性将被Plant构造函数的所有实例继承,无论何时创建它们。 让我们向Plant构造函数的原型属性添加一个函数属性:
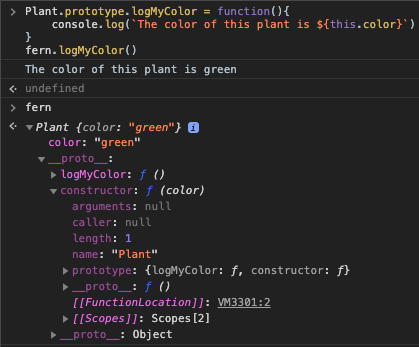
This is a great way to show the difference between .__proto__ and prototype. prototype is what we use to define properties that we want instances of a constructor function to inherit. In this case, we added the function logMyColor() to the prototype of our Plant constructor function. That caused all instances of that constructor function, currently only our instance called fern, to inherit that function in their .__proto__ property. It’s important to note that fern does not have the inherited function on itself, only on the .__proto__ property. This proves that our instance of the Plant constructor function, fern, in inheriting properties of the constructor function’s prototype property.
这是显示.__ proto__和prototype之间差异的好方法。 原型是用于定义属性的属性,我们希望构造函数的实例可以继承这些属性。 在这种情况下,我们将函数LogMyColor()添加到Plant构造函数的原型中。 这导致该构造函数的所有实例(当前仅称为蕨类的实例)在其.__ proto__属性中继承该函数。 重要的是要注意,蕨类自身不具有继承的功能,而仅在.__ proto__属性上具有。 这证明了我们的Plant构造函数的实例fern在继承构造函数的prototype属性的属性中。
This also shows how when we call a value of an object, JavaScript first looks for that value on that instance of the object. If it can’t find it, as is the case with our fern object here, it looks at what that object inherits from, in this case our Plant constructor function (or more specifically, its prototype property). If it can’t find it there JavaScript will continue looking up the prototype chain until it finds that value or ends at null. Let’s check to make sure this is true with the following:
这也说明了当我们调用对象的值时,JavaScript首先在对象的该实例上寻找该值。 如果找不到它,就像这里的蕨类对象一样,它将查看该对象继承自什么,在本例中为Plant构造函数(或更具体地说,其原型属性)。 如果找不到,JavaScript将继续查找原型链,直到找到该值或以null结尾。 让我们检查以下内容,以确保这是正确的:
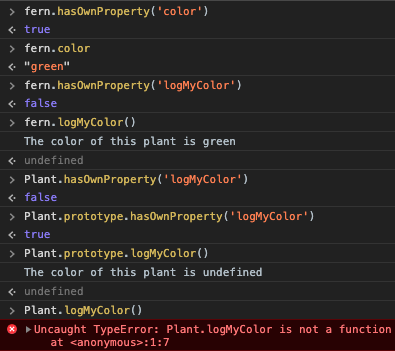
So we can see that fern has the color property, and we can call that property and see its value. We can check if fern has the property logMyColor and see that it does not have that property, but we can still call logMyColor() on our fern! Well, if it doesn’t have that property, but we can still call it, it must be.. I don’t know.. Inheriting (!) it from somewhere!
因此,我们可以看到蕨具有color属性,并且可以调用该属性并查看其值。 我们可以检查蕨类是否具有属性logMyColor,并查看它是否没有该属性,但是我们仍然可以在蕨类上调用logMyColor() ! 好吧,如果它没有该属性,但是我们仍然可以调用它,它一定是..我不知道..从某个地方继承 (!)!
If fern is an instance of the Plant constructor function, it must be inheriting logMyColor() from Plant, right? Well, kind of. It’s not inheriting it directly from Plant, but from Plant’s prototype property. So when we call logMyColor() on fern, JavaScript sees that fern does not have that property, then looks at its constructor function’s prototype property, Plant.prototype, for that property. In Plant’s prototype property JavaScript finds and executes logMyColor().
如果fern是Plant构造函数的实例,则它一定是从Plant继承logMyColor() ,对吗? 好吧,有点。 它不是直接从Plant继承它,而是从Plant的原型属性继承。 因此,当我们在蕨类上调用logMyColor()时,JavaScript会看到蕨类没有该属性,然后查看该属性的构造函数的原型属性Plant.prototype 。 在Plant的原型属性中,JavaScript查找并执行logMyColor() 。
关于.__ proto__与.prototype (On .__proto__ Versus .prototype)
.__proto__ is what we use to refer to the prototype properties instances of objects inherit, and is roughly equivalent to Object.getPrototypeOf(obj). .prototype is the property of our constructor function that holds values we want instances of that constructor function to inherit. However, values given to objects when they are created (like color) belong to that object, and values added to the prototype of the constructor function that created that object are inherited (like logMyColor).
.__ proto__是我们用来引用对象继承的原型属性实例的对象,它大致等效于Object.getPrototypeOf(obj) 。 。 prototype是构造函数的属性,该属性保存我们希望该构造函数的实例继承的值。 但是,创建对象时赋予它们的值(例如color )属于该对象,并且添加到创建该对象的构造函数原型中的值是继承的(例如logMyColor )。
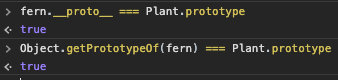
一切都很酷,但是为什么这很重要? (That’s All Very Cool, But Why Is This Important?)
That’s a great question. Understanding prototypes in JavaScript is important because it is one of the qualities unique to JavaScript, and it is in reality how classes in JavaScript work. I’ll talk more about this next week, but in the ECMAScript 2015 (also called ES6) release of JavaScript, the almighty JavaScript developers added the ability to create classes, just like all the other cool programming languages around had been doing for years. That’s right, it wasn’t until 2015 that JavaScript gained the ability to create classes. But JavaScript’s class syntax is really just syntactical sugar, because behind the scenes it’s just making constructor functions and instances of objects, literally exactly what I showed above.
这是一个很好的问题。 了解JavaScript中的原型很重要,因为它是JavaScript特有的特质之一,而实际上是JavaScript中的类如何工作。 下周我将详细讨论,但是在JavaScript ECMAScript 2015(也称为ES6)版本中,全能JavaScript开发人员增加了创建类的能力,就像周围所有其他出色的编程语言已经使用多年一样。 没错,直到2015年JavaScript才具备创建类的能力。 但是JavaScript的类语法实际上只是语法上的糖,因为在幕后它实际上是在构造函数和对象实例,实际上正是我上面显示的内容。
所以从这里到哪里 (So Where To From Here)
Man, there is so much more to learn about prototypes. If you want to know more, check out the links below to continue your reading. The biggest takeaway should be that prototypes exist, you may not see them much anymore, and if you want to create a class system, use JavaScript’s classes instead.
伙计,还有更多关于原型的知识。 如果您想了解更多信息,请查看下面的链接以继续阅读。 最大的收获应该是存在原型,您可能再也看不到它们了,如果要创建类系统,请改用JavaScript的类。
Next week I’ll be tackling the topic of classes in JavaScript. That article should be considerably easier to write, and in contrast to this article, probably make a lot more sense!
下周,我将讨论JavaScript中的类主题。 该文章应该更容易写,并且与本文相比,可能更有意义!
If you liked this article and want to discuss it further please feel free to reach out through one of the avenues below. The tech community has been nothing but warm and welcoming to me and I want to pay that forward.
如果您喜欢这篇文章并想进一步讨论,请随时通过以下一种方式与我们联系。 技术社区只不过是热情和欢迎我,我想为此付出代价。
进一步阅读 (Further Reading)
翻译自: https://medium.com/@tyler.greason/inheritance-in-javascript-bd9aa1146cb6
javascript继承