react高级
角度的 (Angular)
Have you ever wondered why Angular is so powerful with forms? In this article, I will walk you through this topic to understand why and how Angular is better with forms compared to React or Vuejs.
您是否曾经想过,为什么Angular的表单功能如此强大? 在本文中,我将引导您完成本主题,以了解为什么和与React或Vuejs相比,Angular在表单上更好。
Contents:
内容:
- What Reactive Form is 什么是React形式
- Three Basic Blocks 三个基本块
- FormBuilder 表格生成器
- Validators 验证者
- The Magic 魔术
什么是React形式 (What Reactive Form is)
Angular provides 2 ways to work with forms which are template-driven form and reactive form.
Angular提供了两种使用表单的方式,即模板驱动形式和React形式 。
While template-driven form is dealing with form by using directives, Reactive form allows Angular developers to handle forms at the Angular template component.
虽然模板驱动的表单通过使用指令处理表单,但是Reactive表单允许Angular开发人员在Angular模板组件处处理表单。
Reactive Form will bring more benefits compared to template-driven from such as:
与模板驱动相比,“响应式表单”将带来更多好处,例如:
- The code will be testable. 该代码将是可测试的。
- Keep all form logic in the same place. 将所有表单逻辑放在同一位置。
Easy to handle multi-level form (I will highlight this at The Magic section).
易于处理的多级表单(我将在“魔术”部分中突出显示此表单)。
- This approach is friendly with reactive programming. 这种方法与React式编程很友好。
三个基本块 (Three Basic Blocks)
There are 3 fundamental building blocks of Angular Reactive Form:
AngularReact形式有3个基本构建块:
- FormControl 表格控件
- FormGroup 表格组
- FormArray 表格数组
When we build reactive forms in Angular, they all come from these three basic blocks.
当我们在Angular中构建React形式时,它们都来自这三个基本模块。
In order to use Reactive Form, import ReactiveFormsModule
from the @angular/forms
package and add it to your NgModule's imports
array.
为了使用Reactive Form,请 从 @angular/forms
包中 导入 ReactiveFormsModule
并将其添加到NgModule的 imports
数组中。
1. FormControl (1. FormControl)
Initializing Form Controls
初始化表单控件
Here is how we create form control in Angular with 4 different syntaxes.
这是我们如何使用4种不同的语法在Angular中创建表单控件。
First, we can init form control with value only.
首先,我们只能使用值初始化表单控件。
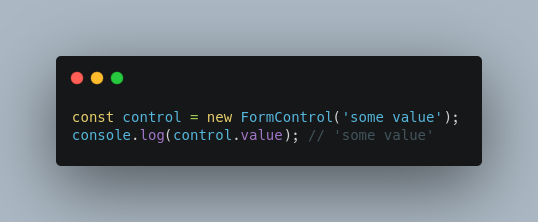
Second, we can create form control with the disabled key.
其次,我们可以使用禁用键创建表单控件。
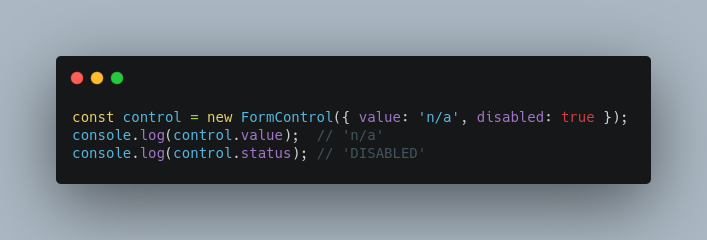
Third, we can add validation rule as the second parameter by using validators
第三,我们可以使用验证器将验证规则添加为第二个参数
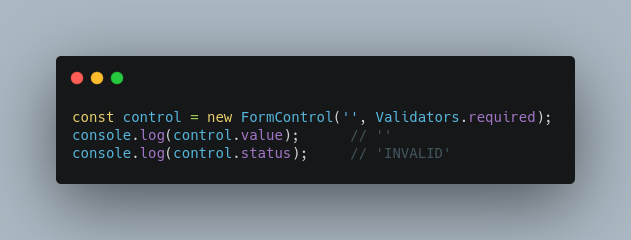
Finally, we sometimes need to call the backend to do validation. In that case, we will use asyncValidators for asynchronous validation.
最后,有时我们需要调用后端进行验证。 在这种情况下,我们将使用asyncValidators进行异步验证。
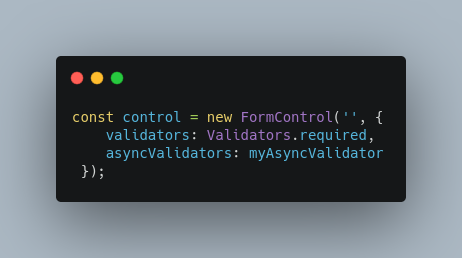
Using updateOn
使用updateOn
To increase the performance, we can use updateOn for some special input that does not need to update every time that user keydown.
为了提高性能,我们可以对某些特殊输入使用updateOn,而这些输入不需要每次用户按下键盘时都进行更新。
We can use a specific event to update such as blur or submit. The default value is change.
我们可以使用特定事件进行更新,例如模糊或提交 。 默认值为change 。
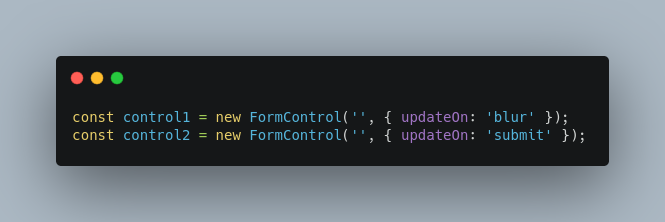
Reset the control
重置控件
We can use the reset
function to change the value of form control.
我们可以使用reset
函数来更改表单控件的值。
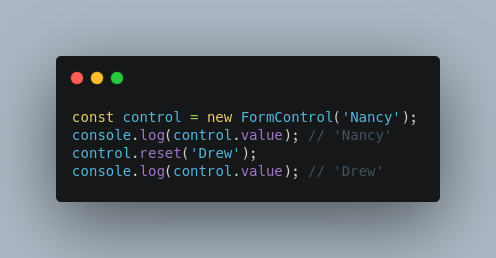
FormControl provides a lot of useful APIs. You can read here.
FormControl提供了许多有用的API。 您可以在这里阅读。
2. FormGroup (2. FormGroup)
A FormGroup aggregates the values of each child FormControl into one object, with each control name as the key.
一个FormGroup将每个子FormControl的值聚合到一个对象中,并以每个控件名称作为键。
It calculates its status by reducing the status values of its children.
它通过减少其子代的状态值来计算其状态。
Create a FromGroup
创建一个FromGroup
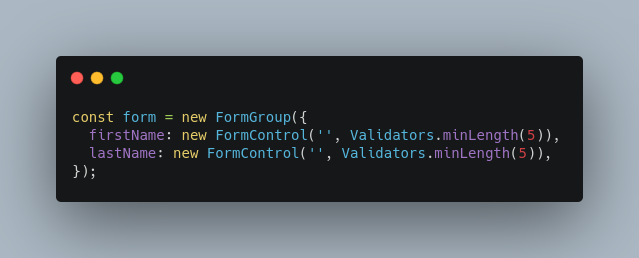
Create a form group with a group-level validator
使用组级验证器创建表单组
We can include group-level validators as the second argument. This will help us to handle validation more easily when we have more than one child control.
我们可以将组级验证器作为第二个参数。 当我们有多个子控件时,这将帮助我们更轻松地进行验证。
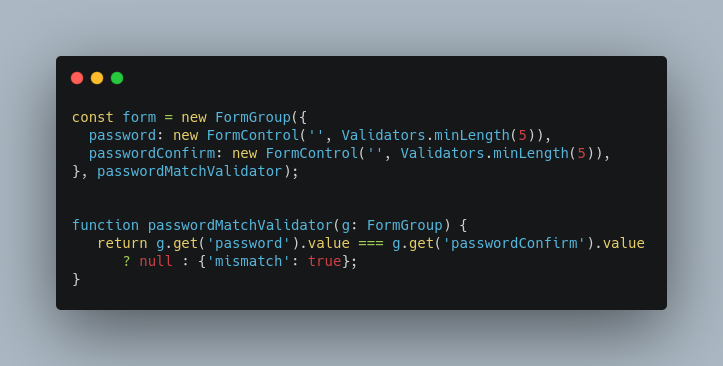
We can use async validators together with validators as part of an options object.
我们可以将异步验证器与验证器一起用作选项对象的一部分。
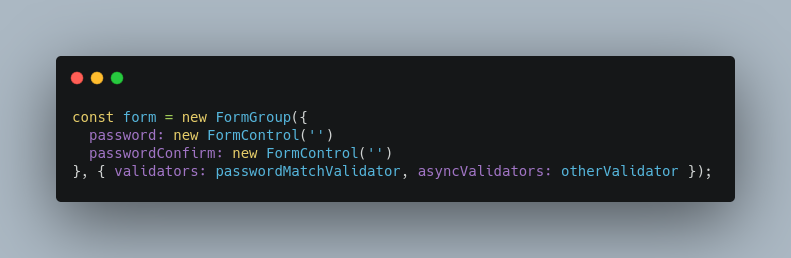
Using updateOn
使用updateOn
This option is similar like updateOn at FormControl. But, when we set it at group level, all child controls will inherit the option value.
此选项类似于FormControl的updateOn 。 但是,当我们在组级别设置它时,所有子控件都将继承该选项值。
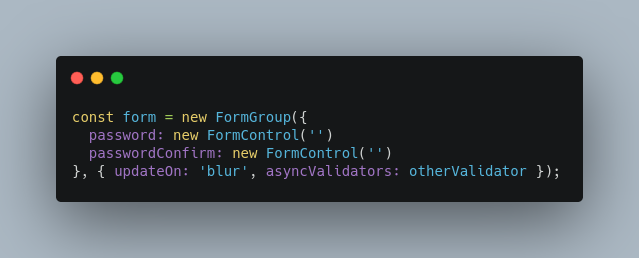
In case that the child has explicitly specified a different updateOn value, it will not use updateOn value at the group level.
如果子级已显式指定其他updateOn值,则它将不在组级别使用updateOn值。
FormGroup provides a lot of useful APIs. You can read here.
FormGroup提供了许多有用的API。 您可以在这里阅读。
3. FormArray (3. FormArray)
A FormArray aggregates the values of each child FormControl into an array.
FormArray将每个子FormControl的值聚合到一个数组中。
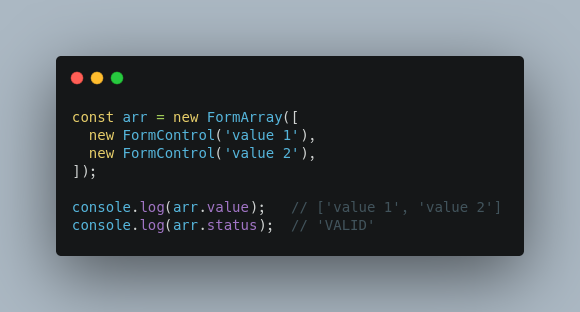
Create a form array with array-level validators
使用数组级验证器创建表单数组
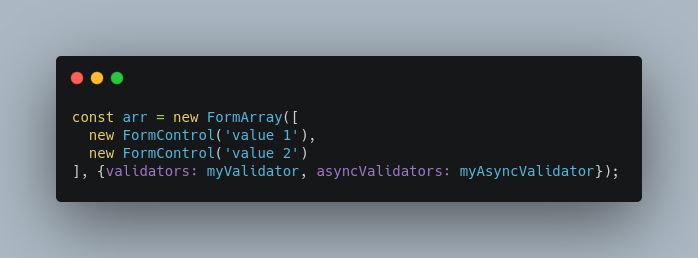
Set the updateOn property for all controls in a form array
为表单数组中的所有控件设置updateOn属性
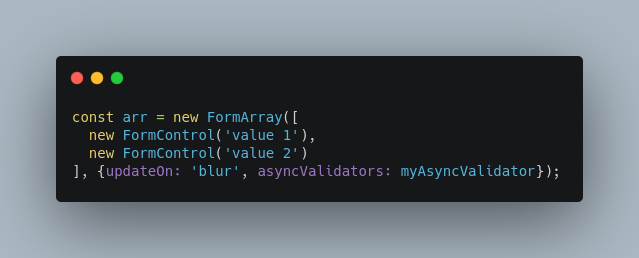
FormArray provides a lot of useful APIs. You can read here.
FormArray提供了许多有用的API。 你可以在这里阅读。
表格生成器 (FormBuilder)
The FormBuilder provides syntactic sugar that shortens creating instances of a FormControl, FormGroup, and FormArray.
FormBuilder提供了语法糖,可以缩短FormControl,FormGroup和FormArray的创建实例。
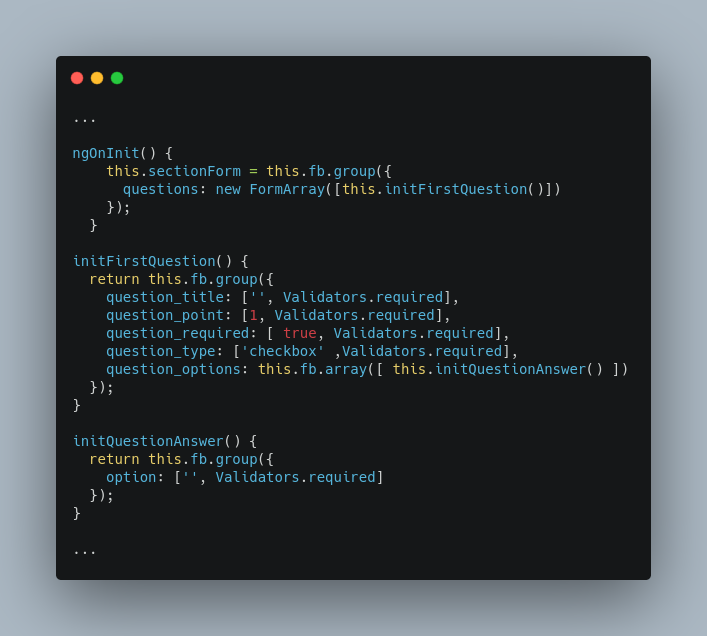
FormBuilder provides us 3 methods:
FormBuilder为我们提供了3种方法:
control
控制
create FormControl
创建FormControl
groupcreate FormGroup
组创建FormGroup
arraycreate FormArray
数组创建FormArray
验证者 (Validators)
Adding validation to the form is very simple by using validators class.
使用验证器类将验证添加到表单非常简单。
分 (min)
Validator that requires the control’s value to be greater than or equal to the provided number.
要求控件的值大于或等于提供的数字的验证器。
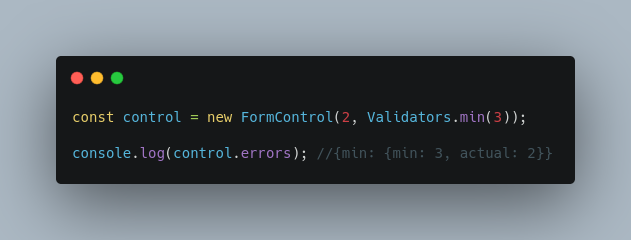
最高 (max)
Validator that requires the control’s value to be less than or equal to the provided number.
要求控件的值小于或等于提供的数字的验证器。
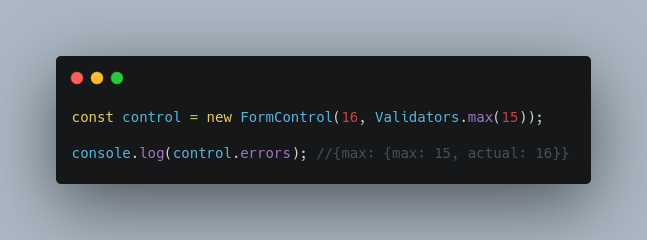
需要 (required)
Validator that requires the control to have a non-empty value.
要求控件具有非空值的验证器。
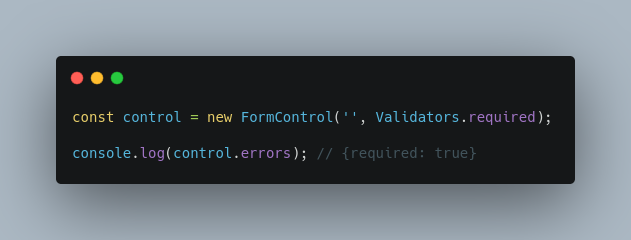
requiredTrue (requiredTrue)
Validator that requires the control’s value be true. This validator is commonly used for required checkboxes.
要求控件值为true的验证器。 该验证器通常用于必需的复选框。
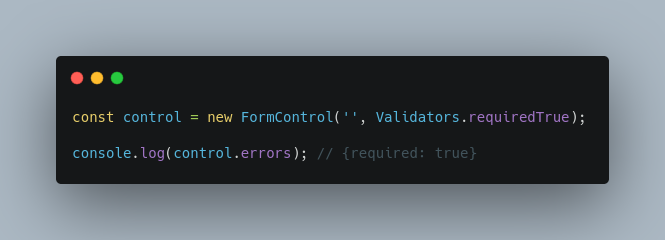
电子邮件 (email)
Validator that requires the control’s value pass an email validation test.
需要控件值的验证器通过电子邮件验证测试。
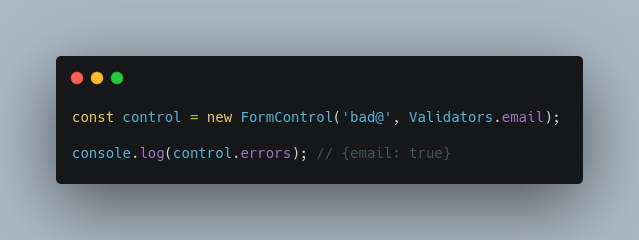
minLength (minLength)
Validator that requires the length of the control’s value to be greater than or equal to the provided minimum length.
验证程序,要求控件值的长度大于或等于提供的最小长度。
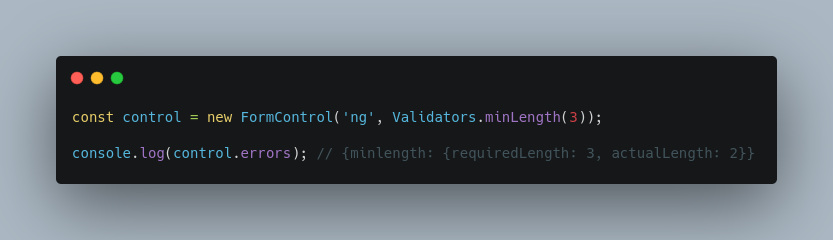
Note: This validator is also provided by default if you use the HTML5 minlength attribute.
注意:如果您使用HTML5 minlength 属性 ,则默认情况下也会提供此验证器 。
最长长度 (maxLength)
Validator that requires the length of the control’s value to be less than or equal to the provided maximum length.
验证程序,要求控件值的长度小于或等于提供的最大长度。
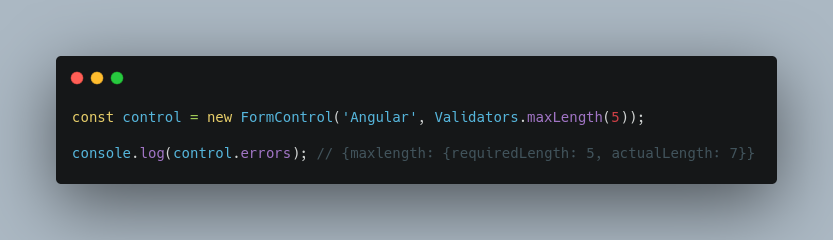
Note: This validator is also provided by default if you use the HTML5 maxlength attribute.
注意:如果您使用HTML5 maxlength 属性 ,则默认情况下也会提供此验证器 。
模式 (pattern)
Validator that requires the control’s value to match a regex pattern.
要求控件值匹配正则表达式模式的验证器。
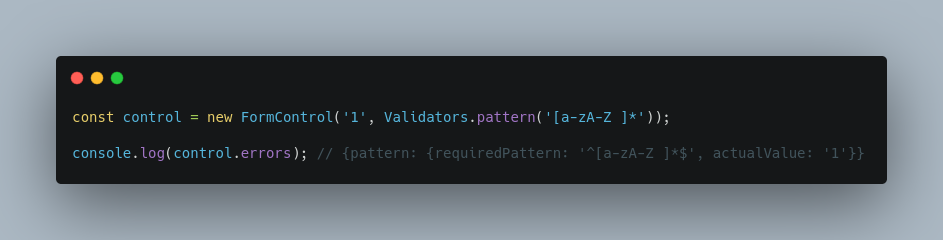
nullValidator
nullValidator
Validator that performs no operation
验证器不执行任何操作
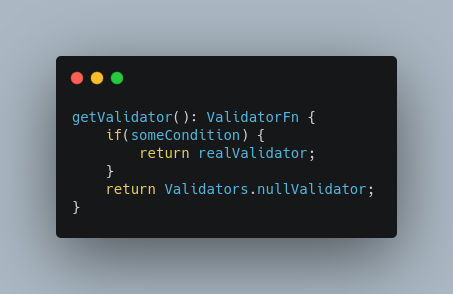
This represents null object pattern which prevents null pointers/null guards in code as always have some validator even when it does nothing.
这表示空对象模式 ,即使在不执行任何操作时,也可以防止代码中的空指针/空防护符始终具有一些验证器。
撰写 (compose)
Compose multiple validators into a single function that returns the union of the individual error maps for the provided control.
将多个验证器组合到一个函数中,该函数返回所提供控件的单个错误映射的并集。
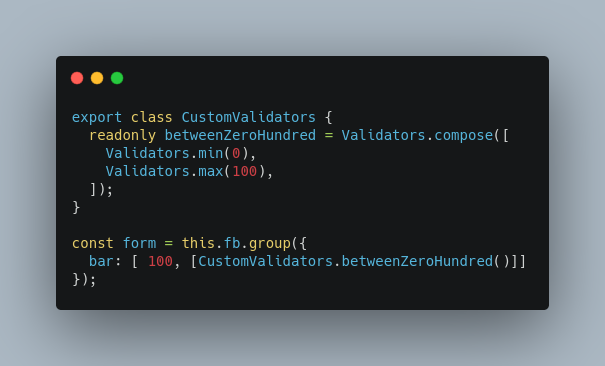
However, we don’t use this method much now. This is a shorter way.
但是,我们现在很少使用此方法。 这是一种较短的方法。
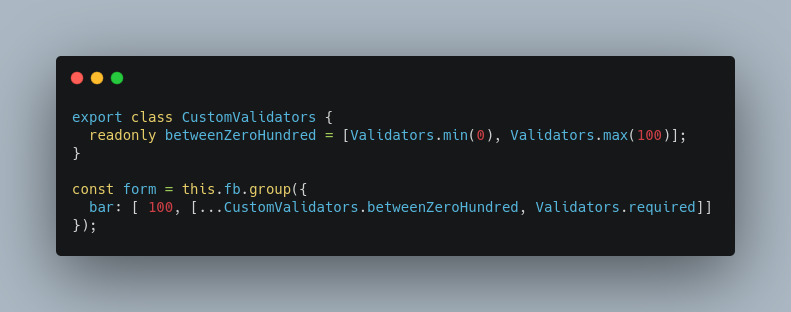
魔术 (The Magic)
What if we have a form with multiple levels?
如果我们有多个级别的表单怎么办?
While it is very painful in React or Vuejs to create a form with 3 levels or 4 levels or more, in Angular we can do it very easily.
虽然在React或Vuejs中创建具有3个级别或4个级别或更多级别的表单非常麻烦,但在Angular中,我们可以很轻松地做到这一点。
Let’s take an example where we need to create a form with a structure like this:
让我们举一个例子,我们需要创建一个具有如下结构的表单:
Questions []
问题[]
- question title
-问题标题
- question point
-问题点
- question type
- 问题类型
- question required
-需要提问
- question options []
-问题选项[]
+ option 1
+选项1
+ option 2
+选项2
+ option 3
+选项3
+ option 4
+选项4
So, we have a simple three level form here with:
因此,我们这里有一个简单的三级表单:
- first level: list of question 第一级:问题清单
- second level: question information 第二级:问题信息
- third level: list of option in one question 第三级:一个问题中的选项列表
In Angular, we create it like this:
在Angular中,我们像这样创建它:
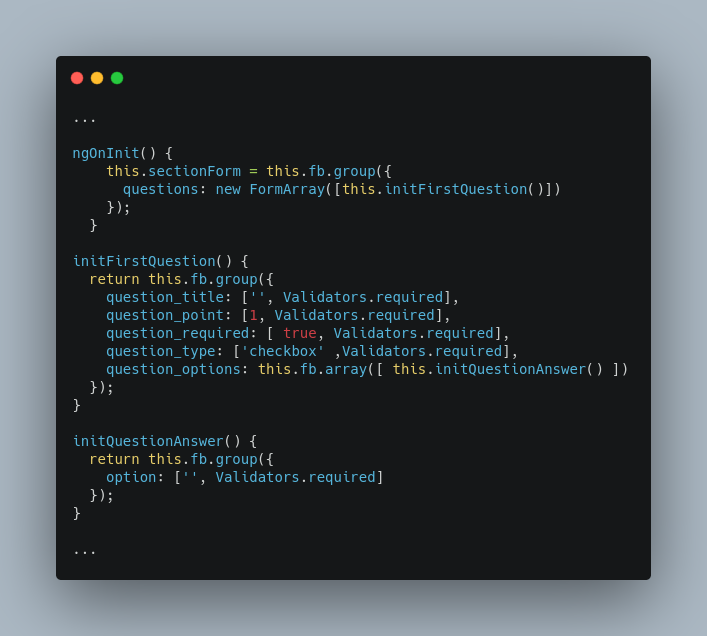
How do we want to add a new question?
我们要如何添加一个新问题?
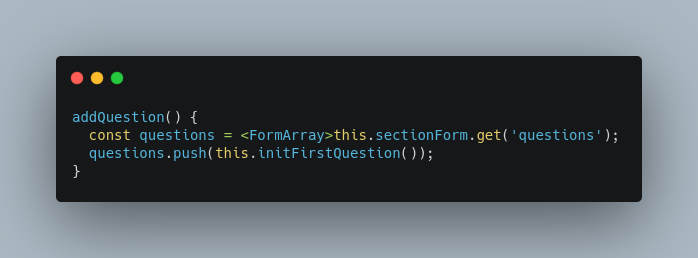
How do we remove a question at index i?
我们如何删除索引i上的问题?
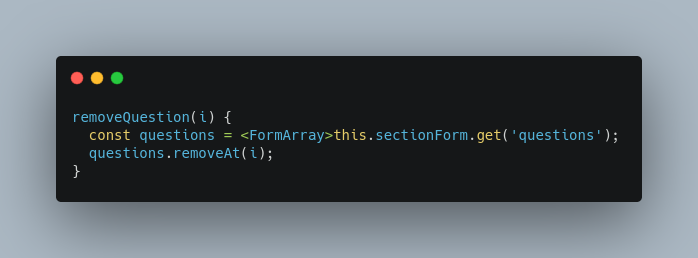
Now, we want to add a new option for question number 10. We just need to do pass the index of question number 10 and do like this:
现在,我们要为问题编号10添加一个新选项。我们只需要传递问题编号10的索引并执行以下操作:
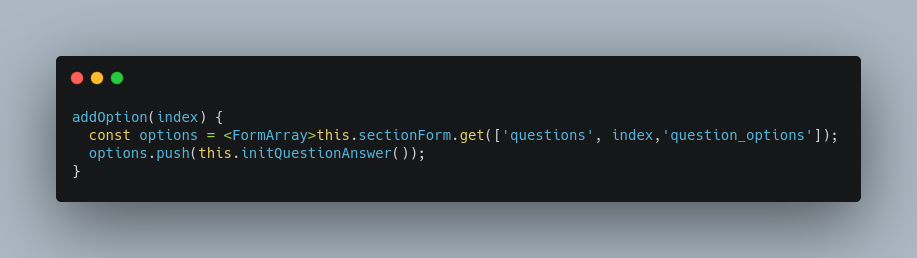
Remove an option j at question i.
删除问题i的选项j。
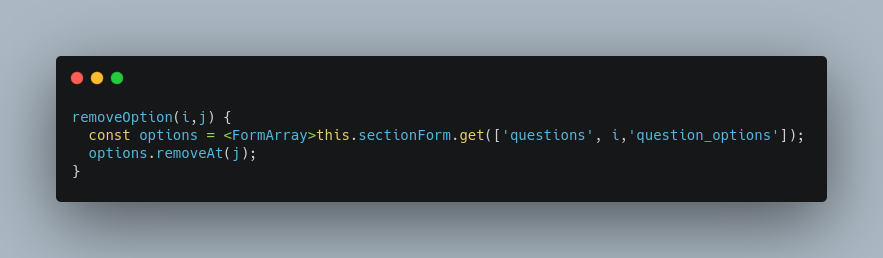
IS IT SIMPLE?
简单吗?
This is the magic that I want to share in this story. The get() in Angular reactive form is so powerful which allows us to work with complicated multiple level forms.
我想在这个故事中分享这魔术。 AngularReact形式的get()功能如此强大,它使我们能够处理复杂的多级形式。
升级编码 (Level Up Coding)
Thanks for being a part of our community! Subscribe to our YouTube channel or join the Skilled.dev coding interview course.
感谢您加入我们的社区! 订阅我们的YouTube频道或参加Skilled.dev编码面试课程 。
翻译自: https://levelup.gitconnected.com/advanced-level-for-angular-reactive-form-6482e19add5c
react高级