react搜索表单组件
DRY (Don’t repeat yourself) is a principle of software development aimed at reducing repetition of software patterns, replacing it with abstractions or using data normalization to avoid redundancy. And while the nature of React development in rich forms application will include a lot of repetition in dealing with the state variables, validations, and rendering fields; I present in this article a way to abstract these operations through an abstract React component.
DRY(不要重复自己)是一种软件开发原则,旨在减少软件模式的重复 ,用抽象代替它或使用数据规范化来避免冗余。 而且尽管以丰富形式进行React开发的本质是应用程序在处理状态变量,验证和呈现字段时将包括很多重复; 我在本文中介绍了一种通过抽象的React组件抽象这些操作的方法。
The first step is to create a React component that will work as the abstract forms component which should be inherited from any view component that needs to access these features. An example of that component would be as next:
第一步是创建一个React组件,该组件将用作抽象表单组件,该组件应从需要访问这些功能的任何视图组件中继承。 该组件的一个示例如下:
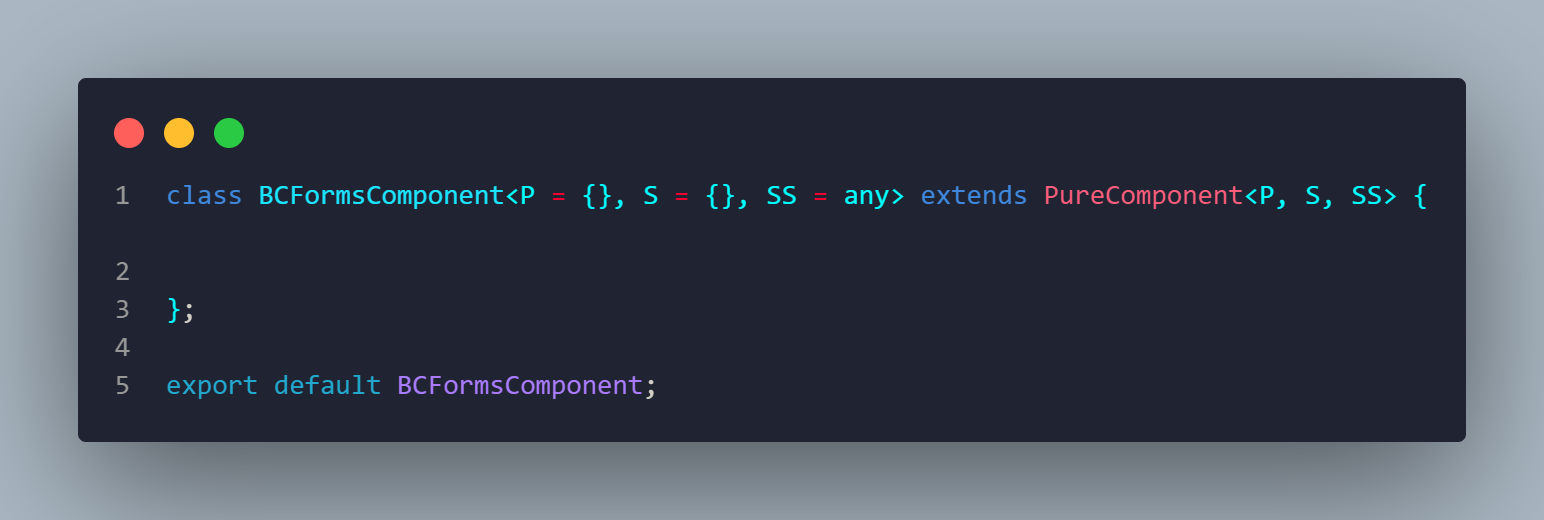
抽象处理字段生成 (Abstracting dealing with fields generation)
Normally we need to have the same way of rendering fields, that contains usually the following steps:
通常,我们需要采用相同的方式呈现字段,通常包含以下步骤:
1- Choosing the input component (Of course, in that case, don’t forget to implement your own low-level input components :))
1-选择输入组件(当然,在那种情况下,请不要忘记实现自己的低级输入组件:))
2- Choosing the structure of the group and the needed CSS classes
2-选择组的结构和所需CSS类
3- Rendering the error message component
3-呈现错误消息组件
4- Rendering the validation classes logic
4-渲染验证类逻辑
Next is an example of how to handle that inside the code:
接下来是如何在代码内部处理该示例:
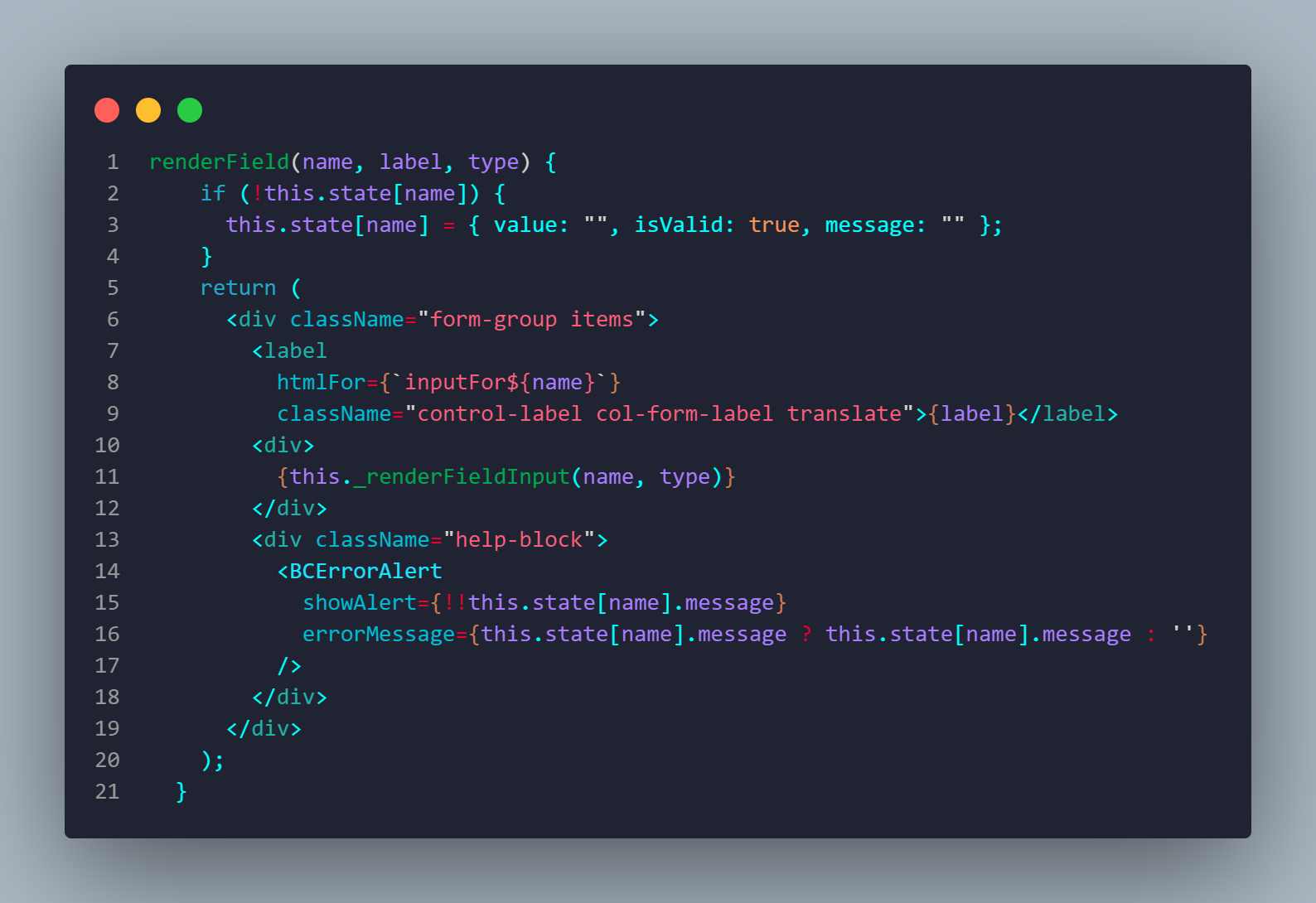
This function will render a form group (Bootstrap form group) that includes a label, a placeholder for the low-level component, a help block to render the errors inside. I am using also a ready component for displaying errors.
此函数将呈现一个表单组(Bootstrap表单组),该表单组包括一个标签,一个低级组件的占位符,一个用于呈现内部错误的帮助块。 我也在使用准备就绪的组件来显示错误。
Please noticed that I made another function to render the low-level input component according to the type of the field, the function code will be as next:
请注意,我做了另一个函数来根据字段的类型来渲染低级输入组件,该函数代码如下:
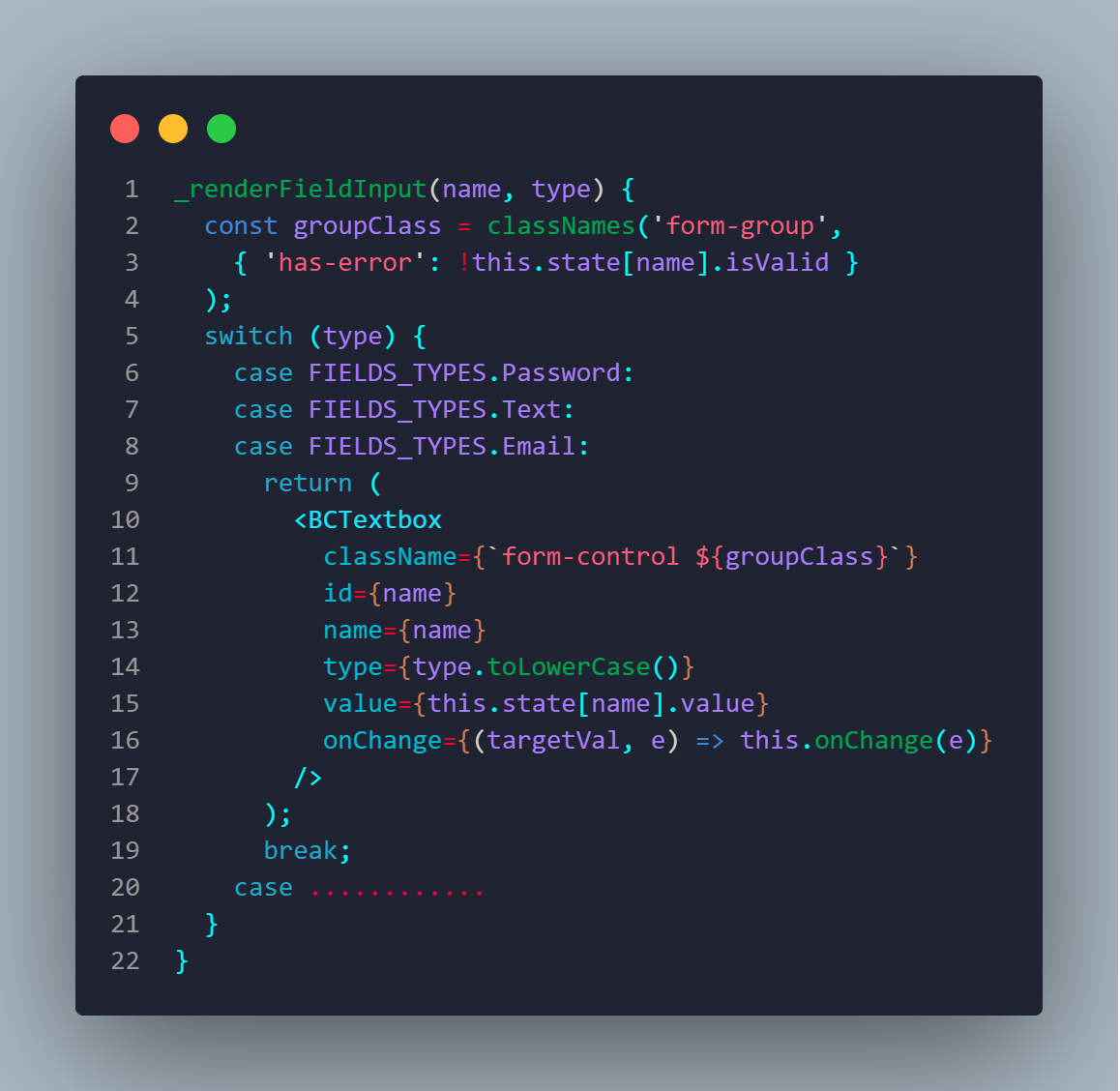
Usually, I prefer starting the name of the function with _ if its a private function.
通常,如果函数名称是私有函数,我更喜欢以_开头。
We can notice that for three types (password, text, and email) we are rendering a Textbox component. And so on for each other type, we will render the low-level component that suits the type. Like file uploaded for image type and date picker for date type…etc.
我们可以注意到,对于三种类型(密码,文本和电子邮件),我们正在渲染文本框组件。 依次类推,我们将呈现适合该类型的低级组件。 如上传的图片类型文件和日期选择器的日期类型…等等。
And we see that in case of error, has-error will be appended to component CSS classes where we can style that as we want to show the error (Like a red border around the input component).
而且我们看到在发生错误的情况下,has-error将被添加到组件CSS类中,在其中我们可以根据需要显示样式(如输入组件周围的红色边框)来设置样式。
So in order to use the “renderField” function in any child component, we need just to call it like next:
因此,为了在任何子组件中使用“ renderField”函数,我们只需要像下一个那样调用它即可:
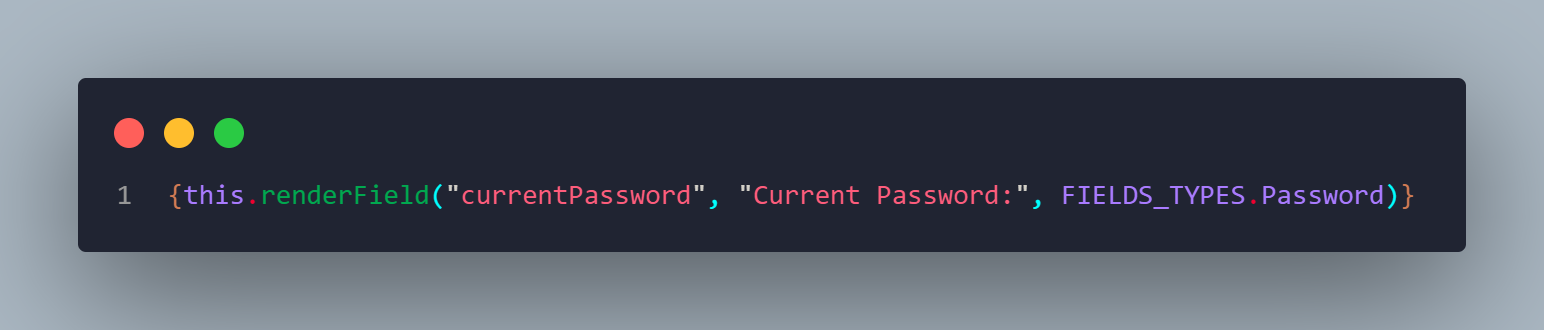
Be aware that inside this function we are initializing the state variable if it's not already initialized, and for each variable (Field), we have three properties:
请注意,如果尚未初始化状态变量,则在该函数内部我们将对其进行初始化,并且对于每个变量(字段),我们具有三个属性:
Value: To store the real value of the field
值 :存储字段的实际值
IsValid: A boolean to know that the field is valid or not
IsValid :知道该字段是否有效的布尔值
Message: The error message in case of invalid
消息 :无效情况下的错误消息
However, if there is a need to initialize the component before rendering it we can implement the next function for that:
但是,如果需要在渲染之前初始化组件,我们可以为此实现下一个功能:
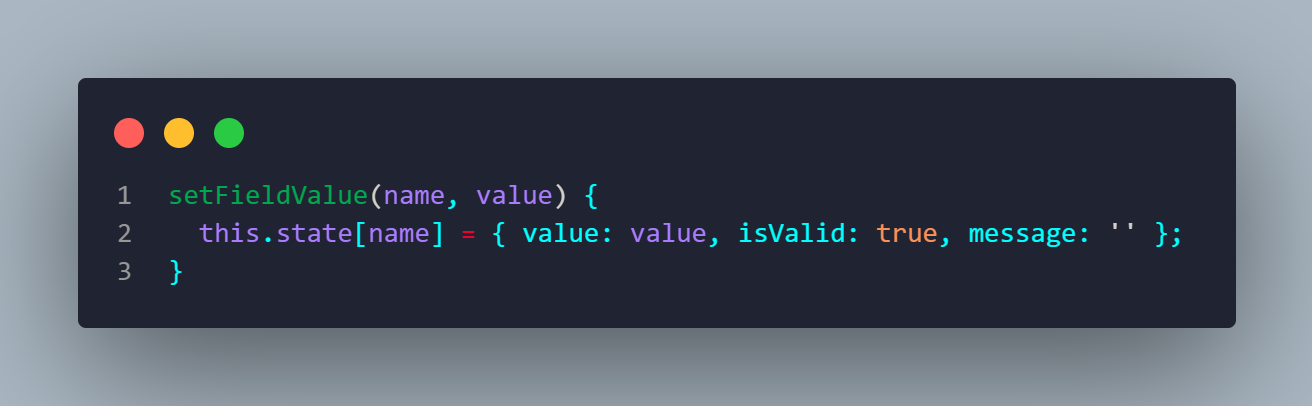
抽象验证操作 (Abstracting validation operations)
Most of the time inside forms, we may need to validate the fields, and it will be a very good idea to have a function that handles validation in a clean way. The next function is taking the field name, the validation type, and additional parameter when needed. And it will check the field with that name if it's following the validation rule related to that type. And then the function will update the IsValid to false and the Message with the error message of the target field if it's not valid; which means the field will re-render again with the error style and message.
大多数时候,在表单中,我们可能需要验证字段,因此,拥有一个以干净的方式处理验证的函数将是一个非常好的主意。 下一个功能是在需要时使用字段名称,验证类型和其他参数。 如果它遵循与该类型相关的验证规则,它将检查具有该名称的字段。 然后,如果无效,该函数会将IsValid更新为false,并使用目标字段的错误消息更新Message; 这意味着该字段将再次使用错误样式和消息重新呈现。
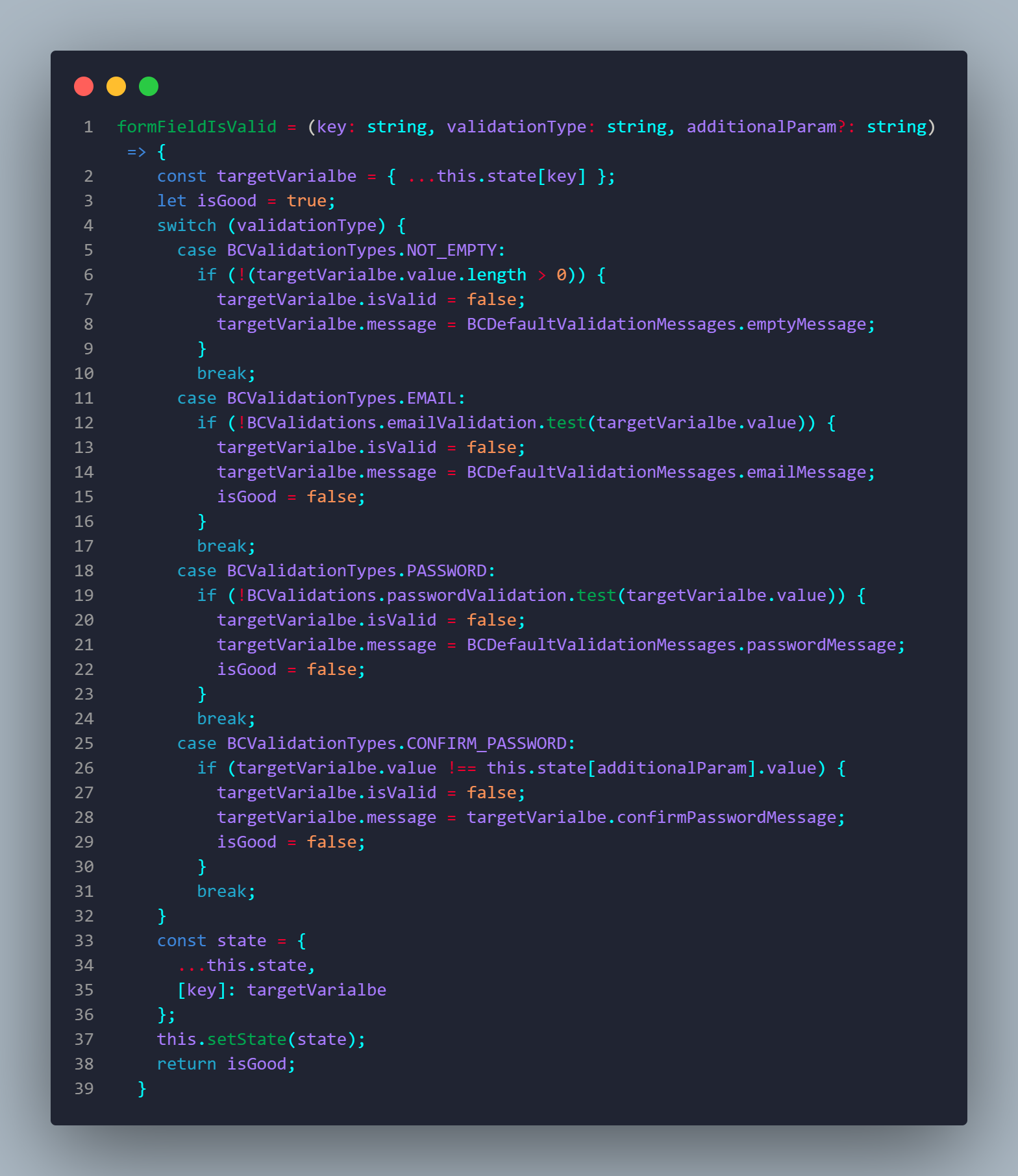
And we can add more validation types as we need, and also we will need a function that will reset the whole fields again so error messages and styles will disappear, the next function can easily iterate on all form fields and reset them:
并且,我们可以根据需要添加更多的验证类型,并且我们将需要一个函数来再次重置整个字段,以便错误消息和样式消失,下一个函数可以轻松地在所有表单字段上进行迭代并重置它们:
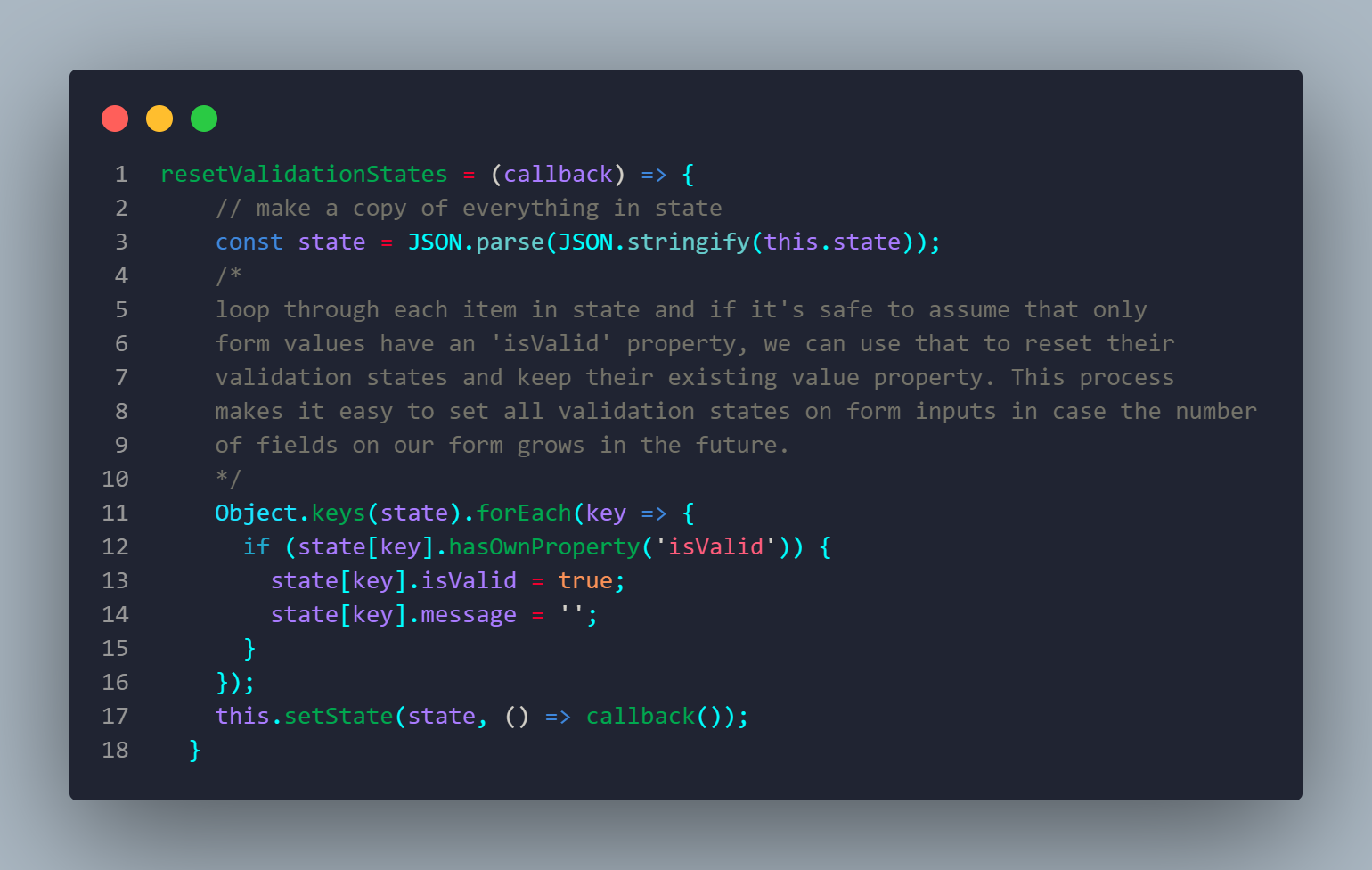
Notice that the “resetValidationStates” function takes one parameter (a callback parameter) which is needed because updating the state in React is not working in a synchronized way, so we need to make sure that all fields are reset before validating again.
注意,“ resetValidationStates”函数采用一个参数(回调参数),这是必需的,因为在React中更新状态不能以同步方式进行,因此我们需要确保在再次验证之前重置所有字段。
So finally, we got a very clean way to validate any form field in just few lines inside the view component, like the next example is showing how I am validating the forget password form before dispatching the action:
因此,最后,我们有了一种非常干净的方法来验证视图组件内仅几行中的任何表单字段,例如下一个示例显示了我如何在分派操作之前验证忘记密码的表单:
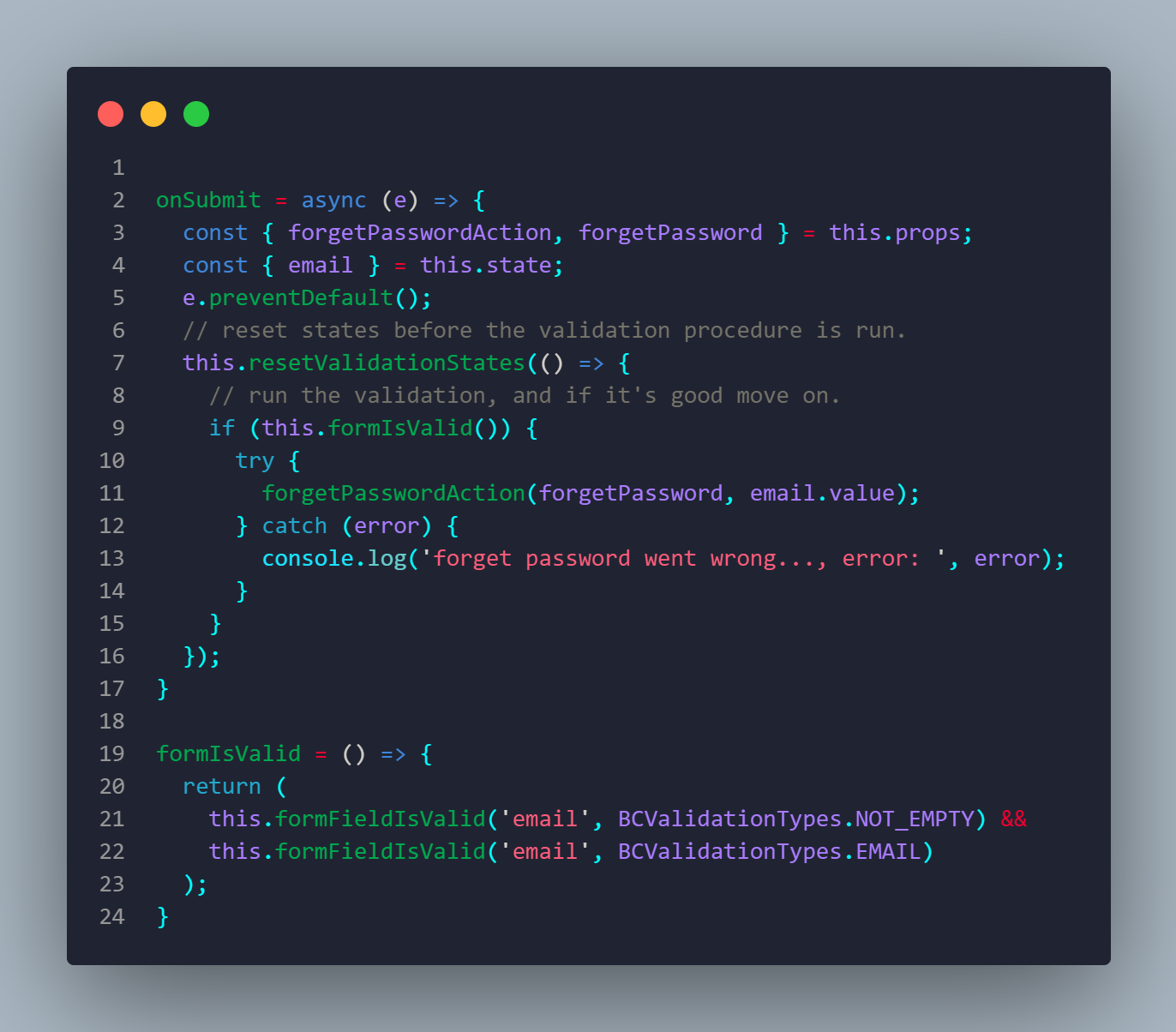
I hope this article will support you in your React project, both for a more organized, shorter, and cleaner code, and one place of modifications when needed to followup DRY principle :)
我希望本文能在您的React项目中为您提供支持,以获得更井井有条,更简短,更简洁的代码,以及在需要时遵循DRY原则进行修改的地方:
普通英语JavaScript (JavaScript In Plain English)
Enjoyed this article? If so, get more similar content by subscribing to Decoded, our YouTube channel!
喜欢这篇文章吗? 如果是这样,请订阅我们的YouTube频道解码,以获得更多类似的内容!
翻译自: https://medium.com/javascript-in-plain-english/abstract-forms-component-in-react-25304681fd55
react搜索表单组件