react hooks使用
Have you stared at those loading spinners on your screen while waiting for an image to load? Whether they are simple, fancy, or animated, they are still boring and frustrating. 😑
您是否在等待图像加载时盯着屏幕上的加载微调框? 无论是简单,精美还是动画,它们仍然无聊而令人沮丧。 😑
Loading a blurred image first enhances the UX and reduces a website’s loading time significantly. After thorough research, I realized that loading images progressively is easier said than done. However, with the power of React hooks, the process becomes extremely easy and hassle-free. Its reusable functional component can work tricks and can be seamlessly used throughout the project.
首先加载模糊的图像可以增强用户体验,并显着减少网站的加载时间。 经过深入研究,我意识到逐步加载图像说起来容易做起来难。 但是,借助React挂钩的功能,该过程变得非常容易且无麻烦。 它的可重用功能组件可以解决问题,并且可以在整个项目中无缝使用。
核心逻辑 (The Core Logic)
Start off by loading a placeholder image in the browser and start the asynchronous call in order to load the original image. Once the original image is loaded, replace the placeholder image with the original image.
首先在浏览器中加载一个占位符图像,然后启动异步调用以加载原始图像。 加载原始图像后,将占位符图像替换为原始图像。
If you are wondering how to make the placeholder image then begin by resizing the image while maintaining the aspect ratio. Make the image as small as possible. For demo purposes, I have resized the original image of resolution 1920*2876 by reducing its width to 5px and adjusting it’s height accordingly. As a result, you can achieve a drastic 99% reduction, leaving the image size to approximately 4kB! 😄
如果您想知道如何制作占位符图像,请先调整图像的大小,同时保持宽高比。 使图像尽可能小。 出于演示目的,我将分辨率为1920 * 2876的原始图像调整为5px的高度,并相应地调整了其高度。 结果,您可以实现99%的大幅降低,而图像大小大约只有4kB! 😄
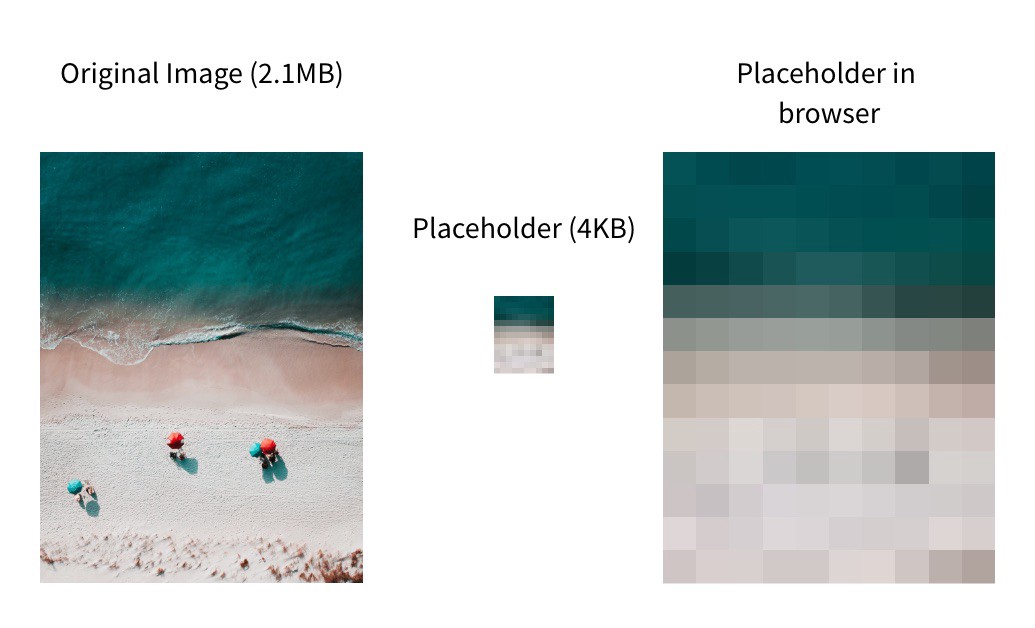
In real-world applications, you don’t want to resize the image manually. Companies like Trello, Slack, Unsplash, etc implement services that will automatically resize the images and then send both the original and placeholder images directly to the frontend for display.
在实际的应用程序中,您不想手动调整图像的大小。 Trello,Slack,Unsplash等公司实施的服务会自动调整图像的大小,然后将原始图像和占位符图像直接发送到前端进行显示。
Since we are already implementing this using React hooks we will have a built-in advantage over class components. Additionally, using React.memo will throw in an added performance boost.
由于我们已经使用React钩子实现了这一点,因此我们将比类组件具有内在的优势。 另外,使用React.memo可以提高性能。
制作可重用的功能组件。 (Making the Reusable Functional Component.)
I have created a reusable functional component named ImageLoad
which takes three arguments namely the original image, the placeholder image, and an alt text.
我创建了一个名为ImageLoad
的可重用功能组件,该组件接受三个参数,即原始图像,占位符图像和alt文本。
The useState
hook returns a tuple where the first parameter is the variable name and the second parameter is the function to update that particular variable. We can destructure the tuple using ES6 syntax. Moreover, useState
also has the ability to take an optional default argument.
useState
挂钩返回一个元组,其中第一个参数是变量名称,第二个参数是更新该特定变量的函数。 我们可以使用ES6语法来破坏元组。 此外, useState
还可以采用可选的默认参数。
In the first line, we are going to implement a
useState
React hook to store and update the loading state. The default value for the loading state is set to true.在第一行中,我们将实现
useState
React钩子以存储和更新加载状态。 加载状态的默认值设置为true。In the second line, the
useState
React hook is implemented to initialize the state with a placeholder image as the default value and to store and update the src of the image.在第二行中,实现了
useState
React钩子,以使用占位符图像作为默认值初始化状态,并存储和更新图像的src。Afterward, we implement the
useEffect
hook to load the image asynchronously and passsrc
as the dependency. This allows it to work similarly to the lifecycle method ofcomponentDidMount
and re-runs only when the value of the src is changed.之后,我们实现
useEffect
钩子以异步加载图像并将src
作为依赖项传递。 这使它的工作方式类似于componentDidMount
的生命周期方法,并且仅在src的值更改时才重新运行。The above
useEffect
will fire only after the code inside the return statement is executed. Similar to the class component, where the render method is executed before running thecomponentDidMount
method. The user will be shown with a blurred placeholder image first.上述
useEffect
仅在执行return语句中的代码后才会触发。 与类组件类似,在运行componentDidMount
方法之前执行render方法。 首先将为用户显示模糊的占位符图像。Finally, once the original image is loaded inside the
useEffect
hook, theupdateSrc
method will update the placeholder image with the original image in the state. As a result, the component is re-rendered and the original image is displayed to the user.最后,将原始图像加载到
useEffect
挂钩中之后,updateSrc
方法将使用状态为的原始图像更新占位符图像。 结果,该组件被重新渲染,并且原始图像显示给用户。
The purpose of using React.memo is to avoid any re-render, provided the props passed, remain unchanged.
使用React.memo的目的是避免重新渲染,只要传递的道具保持不变。
结论 (Conclusion)
Progressive loading is an excellent way to achieve great UX, especially during a slow internet connection. It is not only visually appealing to the users but it also allows them to know what to expect on their screen. React hooks makes the process concise and clear as well as aids in performance improvement.
渐进式加载是获得出色UX的绝佳方法,尤其是在互联网连接速度缓慢的情况下。 它不仅在视觉上吸引了用户,而且还使他们知道在屏幕上期待什么。 React挂钩使过程简洁明了,并有助于提高性能。
For further enhancements, you can also try lazy loading, so that images are loaded only when they are visible in the browser viewport.
为了进一步增强功能,您还可以尝试延迟加载,以便仅在浏览器视口中可见图像时才加载图像。
I hope you find this helpful! Thank you for reading.
我希望你觉得这有用! 感谢您的阅读。
翻译自: https://codeburst.io/how-to-progressively-load-images-in-react-using-hooks-80c50fd447cd
react hooks使用