react 使用hook
自从如今切成薄片以来,Spotify似乎是最酷的东西,它是事实上的随时随地听音乐的方式。 (Spotify seems to be the coolest thing since sliced bread nowadays, and the defacto way to listen to music anytime, anywhere.)
While using the Spotify desktop player the other day, I thought that it might be neat to see how difficult it would be to implement some of its user interface in React. Several hours later, and with way less stress than I had imagined, I was able to come up with something pretty nice.
前几天在使用Spotify桌面播放器时,我想看看在React中实现其某些用户界面有多困难可能会很整洁。 几个小时后,而且压力比我想象的要少,我得以提出一些非常不错的东西。
Therefore, this article (and the ones that will follow it) will be geared towards those that want to improve their React skills, and use its newer features to come up with what I think is an elegant implementation of a basic music player.
因此,本文(及其后续文章)将针对那些希望提高自己的React技能,并使用其较新功能提出我认为是基本的音乐播放器的优雅实现的人。
Our audio player will be built to utilize the Web Audio API out of the box, and will include the following functionality:
我们的音频播放器将被构建为可立即使用Web Audio API,并将包括以下功能:
- Creating & removing playlists 创建和删除播放列表
- Viewing & updating playlists 查看和更新播放列表
- Play, pause, fast-forward, rewind etc. 播放,暂停,快进,倒带等
- Adjusting volume 调整音量
- Favoriting songs 喜爱的歌曲
I highly recommend reading through the tutorial first, and then checking out the accompanying video I’ve uploaded for this article on YouTube (which you can find at the end of the article).
我强烈建议您先通读本教程,然后查看我在YouTube上为本文上传的随附视频( 您可以 在本文结尾处 找到 该视频 )。
设计布局 (Designing The Layout)
By dissecting the image featured at the beginning of this article, I’ve come up with a simple diagram to highlight the basic building blocks needed to create our music player.
通过剖析本文开头介绍的图像,我得出了一个简单的图表,突出显示了创建音乐播放器所需的基本构建块。
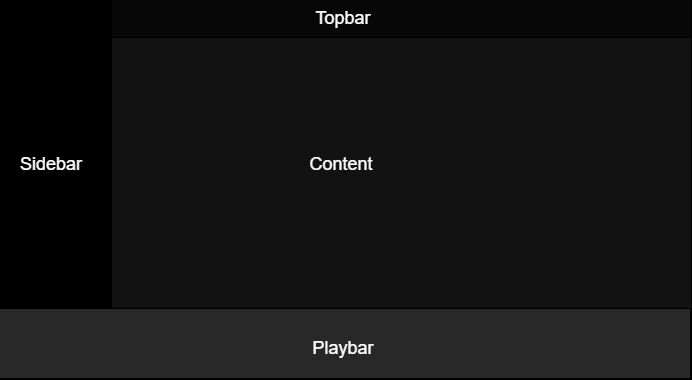
The four components we will use will be named:
我们将使用的四个组件将被命名为:
- Topbar 顶栏
- Sidebar 侧边栏
- Content 内容
- Playbar 播放栏
But before we start their implementation, we also need an entry point to import these components into later on. To keep things simple, let’s create a component named MusicPlayer to serve as the entry point, and then hop right into our first two layout components.
但是在开始实施之前 ,我们还需要一个入口点,以便稍后将这些组件导入。 为简单起见,让我们创建一个名为MusicPlayer的组件作为入口点,然后直接跳入我们的前两个布局组件。
/** @jsx jsx */
import React from 'react'
import { css, jsx } from '@emotion/core'const MusicPlayer = () => (
<div>
{/* ...Content Here */}
</div>
)export default MusicPlayer
内容和侧栏组件 (Content & Sidebar Components)
Per the diagram in the previous section, the Content and Sidebar should sit next to one another, with the Sidebar component being placed on the left hand side. Seeing as items inside of a flex container sit side by side by default, we will use flexbox within MusicPlayer to make this feature a trivial task.
根据上一节中的图表,“内容”和“补充工具栏”应彼此相邻放置,并且“补充工具栏”组件位于左侧。 看到默认情况下,Flex容器中的项并排放置,我们将在MusicPlayer中使用flexbox使此功能变得很简单。
/** @jsx jsx */
import React from 'react'
import { css, jsx } from '@emotion/core'const MusicPlayer = () => (
<div css={CSS}>
{/* ...Content Here */}
</div>
)const CSS = css`
height: 100%;
width: 100%;
display: flex;
position: relative;
color: white;
`
export default MusicPlayer
With that styling in place, we should feel confident that Content and Sidebar will sit side by side when we piece everything together later on.
有了适当的样式后,当我们以后将所有内容组合在一起时,我们应该对Content和Sidebar并排放置充满信心。
内容 (Content)
The Content component isn’t of great importance to us at the moment. For now, let’s give it some very basic styling.
目前,内容组件对我们而言并不重要。 现在,让我们为它提供一些非常基本的样式。
侧边栏 (Sidebar)
Sidebar will be where most of our functionality in regards to playlists will reside. Let’s create it as an unordered list, set it to a fixed width of 200 pixels, and throw in a logo for good measure.
边栏将是我们与播放列表有关的大多数功能所在的位置。 让我们将其创建为无序列表,将其设置为200像素的固定宽度,并放入徽标以作好衡量。
顶栏 (Topbar)
Topbar won’t be providing any functionality in the series, but having it does create an overall nicer visual appeal. We can assign it a height and background color, and then leave it as is for now.
Topbar不会在该系列中提供任何功能,但是拥有它确实可以创造出整体上更好的视觉吸引力。 我们可以为其指定高度和背景颜色,然后将其保留原样。
播放栏 (Playbar)
Playbar is where we will spend a good amount of time later on in the series. But as for it’s current requirements, we only need to position it so that it sits at the bottom of the screen and looks the part.
Playbar是我们在本系列后面将花费大量时间的地方。 但是对于当前的要求,我们只需要对其进行定位,使其位于屏幕底部,即可看到零件。
测试布局 (Testing The Layout)
With our four layout components completed, it’s time to import them into our entry component and see if everything works.
完成了四个布局组件之后,是时候将它们导入到我们的输入组件中,看看是否一切正常。
If we open up the browser and everything has gone according to our plan, then we should be met with our layout working as intended!
如果我们打开浏览器并且一切都按照我们的计划进行,那么我们应该按照预期的方式进行布局!
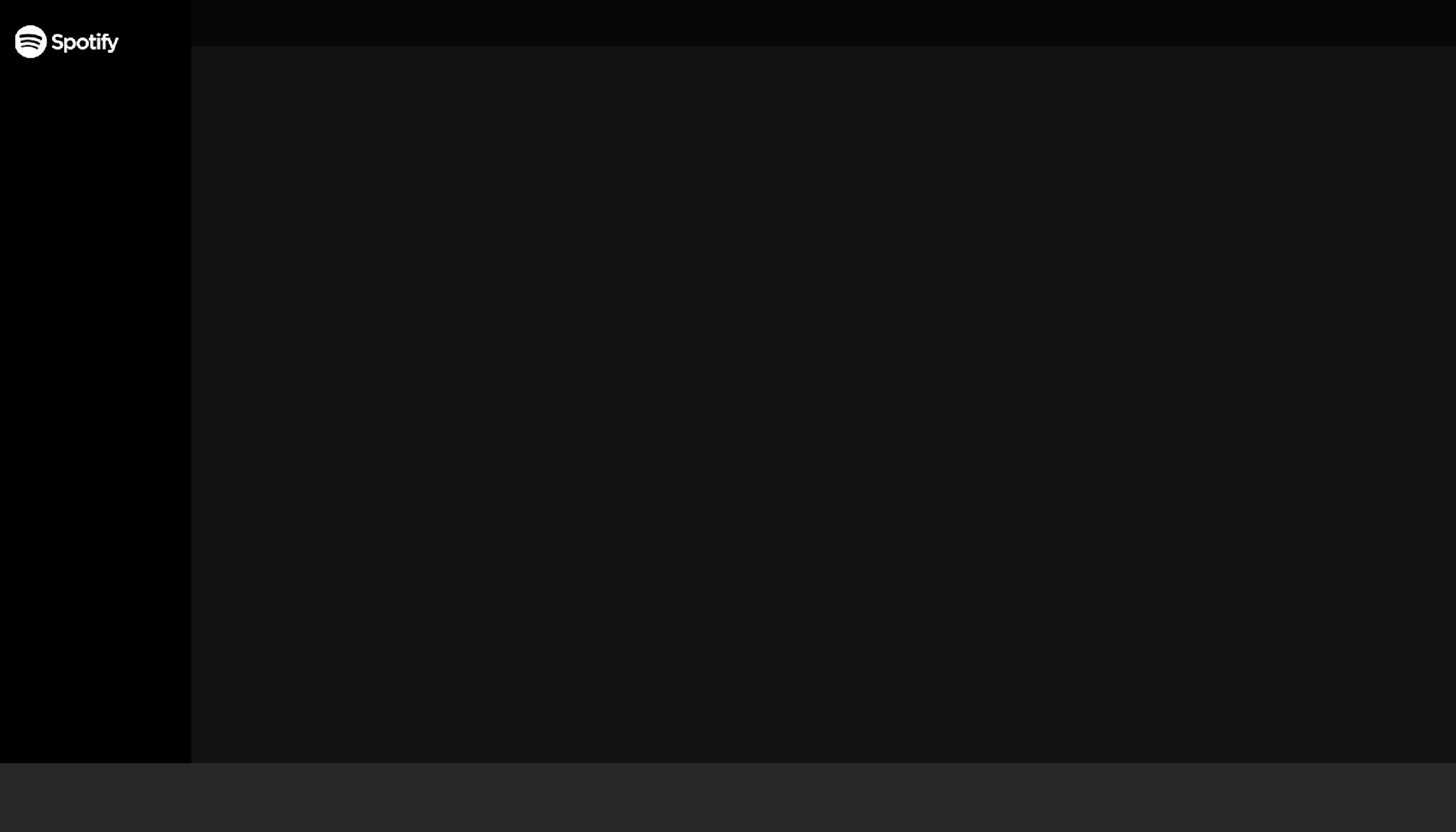
下一个 (Next Up)
So where do we go from here? In part two of this series we will start working within the Sidebar component, and begin implementing some of the state that is required for playlists to work. We will use a “local first” strategy when it comes to state, and will hold off on using Context until the functionality deems it necessary.
那么,我们该何去何从? 在本系列的第二部分中,我们将开始在补充工具栏组件中工作,并开始实现播放列表正常工作所需的某些状态。 当涉及到状态时,我们将使用“本地优先”策略,并将在功能认为必要之前一直使用Context。
As promised, here is the video! The article for part two should be posted shortly, stay tuned!
如所承诺的,这是视频! 第二部分的文章应尽快发布,敬请期待!
react 使用hook