UPDATE: once you are done with this, read my “extension” tutorial on how to add Google Login to Firebase inside Expo project => https://medium.com/@popeating/react-native-firebase-adding-google-authenticaton-in-an-expo-project-2-ed20cb440732
更新 :完成此操作后,阅读我的“扩展”教程,了解如何在Expo项目中将Google登录添加到Firebase => https://medium.com/@popeating/react-native-firebase-adding-google-authenticaton in-expo-project-2-ed20cb440732
On my journey on learning App development with React Native, i decided to try making a small and contained app that will service a unique task, a self contained login system, that uses Firebase Auth using (at the moment) email/password sign-in method.
在学习使用React Native开发应用程序的过程中,我决定尝试制作一个小型且包含内容的应用程序,该应用程序将为一项独特的任务提供服务,即一个自包含的登录系统,该系统使用Firebase Auth(目前使用)通过电子邮件/密码登录方法。
Even if the app is just a skeleton i tried to make it as complete as possibile in term of interface/usability, using react-native-paper components for interface, enabling dark theme if supported, adding translation and other little touches. I tried to code in a modern way, so i make use of context and state Hooks, of course this code is not perfect and i will keep updating it on its GitHub https://github.com/popeating/FirebaseLogin.
即使该应用只是一个框架,我也试图在界面/可用性方面使其尽可能完整,使用react-native-paper组件作为界面,启用深色主题(如果支持),添加翻译和其他一些修饰。 我尝试以现代方式进行编码,因此我使用了上下文和状态Hooks,当然,这段代码并不完美,我将继续在其GitHub https://github.com/popeating/FirebaseLogin上对其进行更新。
这个应用程式的运作方式 (How this app works)
Basically the app is composed by 3 screens in two navigation stacks. A stack holding the signin and signup (not yet implemented) screens, a stack holding the home screen for logged in user. The app is started with a isLoading state active and a userLogged state to false; it will show a page loader, during this time with useEffect we will check the Firebase userstate:
基本上,该应用程序由两个导航堆栈中的3个屏幕组成。 包含登录和注册(尚未实现)屏幕的堆栈,其中包含登录用户的主屏幕的堆栈。 该应用程序以isLoading状态为active且userLogged状态为false来启动; 它将显示一个页面加载器,在此期间,通过useEffect我们将检查Firebase用户状态:
Firebase.auth().onAuthStateChanged((user) => {…})
if it changes and return a user, then we set the isLoading to false and userLogged to true or false, based on the user object presence. The combination of these two values, allow us to show the correct stack.If the user is logged the stack shows up with the home screen (that actually hold just a button to signout and a button to switch theme), if the user is not logged it shows with a login screen that also have a link to sign up screen.I built this project for future implementation in bigger apps that requires user login. This app also lacks error messages for wrong credentials and other things that could be easily implemented (code is commented)
如果更改并返回用户,则根据用户对象的存在,将isLoading设置为false,将userLogged设置为true或false。 这两个值的组合使我们能够显示正确的堆栈。如果用户已登录,则堆栈将显示在主屏幕上(实际上仅包含一个用于注销的按钮和一个用于切换主题的按钮),如果用户未登录登录后,它会显示一个登录屏幕,并带有一个登录屏幕的链接。我构建了这个项目,以便将来在需要用户登录的大型应用中实施。 这个应用程式也没有错误讯息,指出错误的凭证和其他容易实现的事情(注释了程式码)
先决条件 (Prerequisites)
Before start coding i created a new project on Firebase (https://console.firebase.google.com/) and added Authentication Service, I selected Email/Password as Sign-in method, I also created a test user:
在开始编码之前,我在Firebase( https://console.firebase.google.com/ )上创建了一个新项目,并添加了身份验证服务,我选择了Email / Password作为登录方法,还创建了一个测试用户:
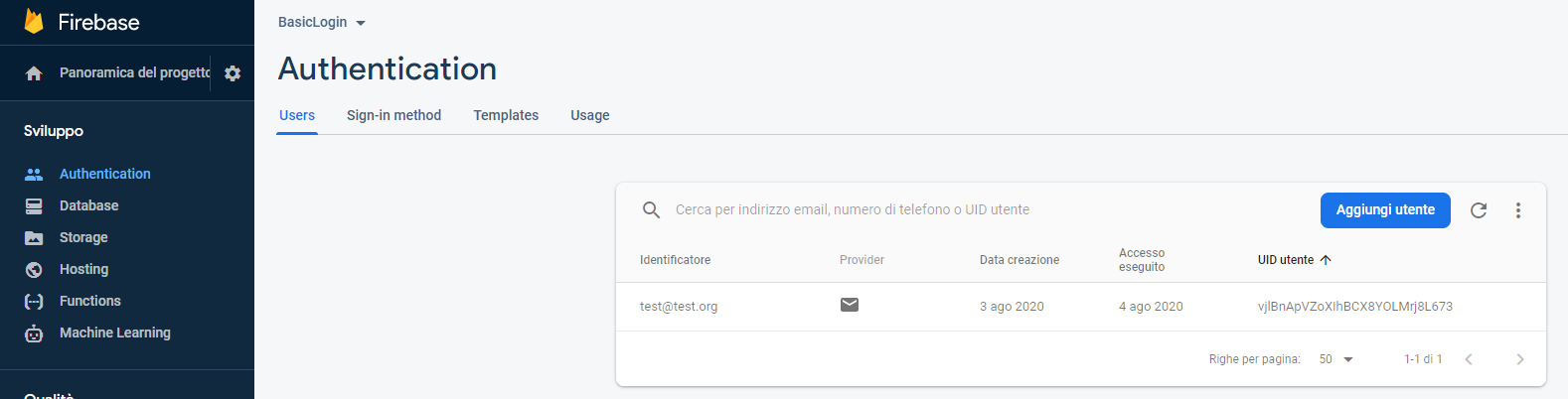
Then i copied the Firebase configuration from the project settings (gear icon on top left)
然后我从项目设置中复制了Firebase配置(左上方的齿轮图标)
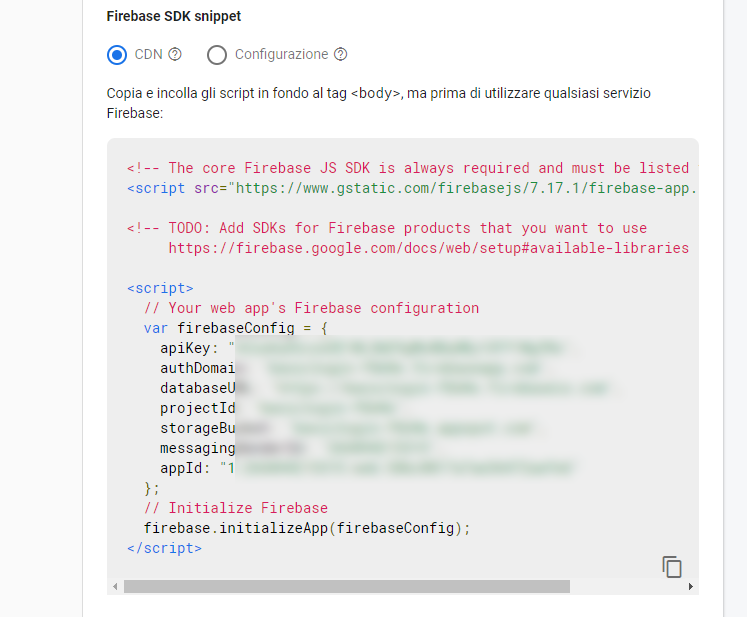
I started a new project in Expo:
我在世博会开始了一个新项目:
expo init FirebaseLogin
and i installed some modules i planned to use (only firebase, navigation and Constants are required for this project, but i also installed localization and react-native-paper)
并且我安装了一些计划使用的模块(此项目仅需要firebase,导航和Constant,但我还安装了本地化和react-native-paper)
expo install firebase
npm install react-native-paper
expo install expo-localization
npm install i18n-js
expo install expo-constants
npm install @react-navigation/native
//navigation dependencies
expo install react-native-gesture-handler react-native-reanimated react-native-screens react-native-safe-area-context @react-native-community/masked-view
Configure app.json to include Firebase conf
配置app.json以包括Firebase conf
Once the app.json contain the Firebase configuration, it can be imported in other files; for example we create a file in the root of the project where we initialize the Firebase App, based on the config, and we can then import this initialization in other screens and components
一旦app.json包含Firebase配置,就可以将其导入其他文件; 例如,我们在项目的根目录下创建一个文件,在该文件的基础上根据配置对Firebase App进行初始化,然后可以将此初始化导入其他屏幕和组件中
Since we are going to use context (to have function and objects available to multiple components, we create a Context that will be available for import later, it will be called mainContext and it will be used as a sourrounding provider :
由于我们将使用上下文(为了使功能和对象可用于多个组件,因此我们创建了一个Context,以后可将其导入,将其称为mainContext并将其用作外围提供程序:
App.js (App.js)
This will be our app entry point, let’s begin by importing some stuff, nothing fancy just the required modules, and some definitions
这将是我们应用程序的切入点,让我们从导入一些东西开始,仅导入所需的模块,再定义一些定义
Next, still in App.js we can go on building the app
接下来,仍然在App.js中,我们可以继续构建应用
At this point the app won’t run because we are still missing the Screens, let’s add them
此时,由于我们仍然缺少屏幕,因此该应用无法运行,让我们添加它们
HomeScreen.js (HomeScreen.js)
This is the screen that user will see once logged, it’s really basic, just a blank page with a Sign Out button and a switch theme button; both onPress action activate a function which is available to the Screen via the contextThe current user come from Firebase.auth() ̶i̶t̶ ̶c̶a̶n̶ ̶a̶l̶s̶o̶ ̶b̶e̶ ̶r̶e̶a̶d̶ ̶f̶r̶o̶m̶ ̶c̶o̶n̶t̶e̶x̶t̶, and i sticked with it, because the user is not always present in context (i think this is due to the fact that everything is async)
这是用户登录后将看到的屏幕,它实际上是基本的,只是一个空白页面,带有“退出”按钮和“切换主题”按钮。 这两个onPress动作均会激活一个可通过上下文在屏幕上使用的功能当前用户来自Firebase.auth()来自上下文的用户一直认为该上下文不存在,因为我始终(这是由于所有内容都是异步的)
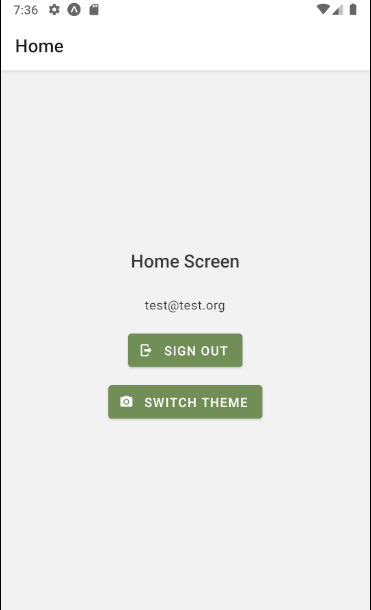
LoginScreen.js (LoginScreen.js)
Nothing fancy here too, a simple form that accept email and password (updated and managed but Hook States) that execute a function ‘grabbed’ from context, it also contain a link to navigate to SignUpScreen
这里也没有什么花哨的,它是一种简单的表单,可以接受从上下文执行“抓取”功能的电子邮件和密码(已更新和托管,但具有挂钩状态),并且还包含一个导航到SignUpScreen的链接
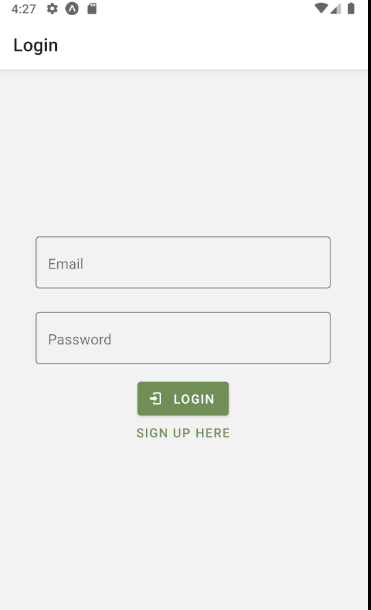
SignUpScreen.js (SignUpScreen.js)
Signup screen simply use Firebase API to create a new user with user/password — name is ignored at the moment — and login the user upon creation
注册屏幕仅使用Firebase API来创建具有用户名/密码的新用户(此刻名称将被忽略),并在创建时登录该用户
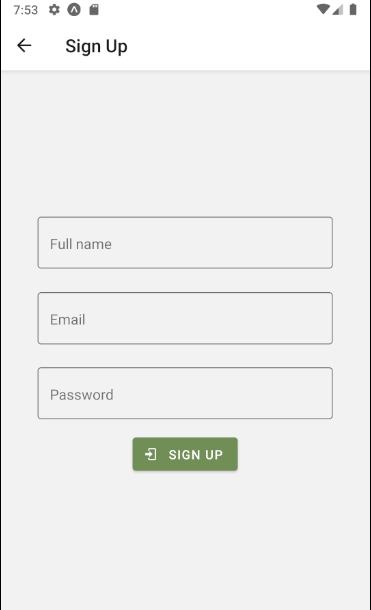
其他有用的东西 (Other useful things)
I added a couple of details that I always struggle to implement, to have them handy.For example the screen that contains a keyboard are enclosed in
为了方便起见,我添加了一些我一直难以实现的细节,例如包含键盘的屏幕
<TouchableWithoutFeedback onPress={() => Keyboard.dismiss()}>//CODE</TouchableWithoutFeedback>
So that if you click outside the text input the keyboard will close
这样,如果您在文本输入之外单击,键盘将关闭
翻译 (Translation)
To have translation working i use the following “skeleton”:
为了使翻译正常工作,我使用以下“骨骼”:
- create a localization utils like this one: 创建一个这样的本地化实用程序:
//utils/localization.js
import * as Localization from 'expo-localization';
import loc from 'i18n-js';//Get the device language
loc.locale = Localization.locale; //Create as many translation you need
import en from '../locale/en';
import it from '../locale/it'; loc.fallbacks = true;
loc.translations = { it, en,}; export default loc;
- create the localization dictionary 创建本地化字典
//locale/en.js
export default {
mappa: 'Map'
clickme: 'Click me',
home: 'Home',
notifiche: 'Notifications',
entra: 'Login',
loginButton: 'Login',
signupButton: 'Sign Up',
noaccount: 'Sign up here',
signup: 'Sign Up',
nome: 'Full name',
signout: 'Sign out',
theme: 'Switch theme',
};
- use the localized string in components 在组件中使用本地化的字符串
//Component.js
import loc from '../utils/localization';...<Text>{loc.t('clickme')}</Text>
去做 (TO DO)
The login page do not show errors if login credentials are wrong or other kind of errors, the login works only if the phone is online (of course) but do not check if internet is available, code could be surely optimized
如果登录凭据错误或其他类型的错误,登录页面不会显示错误,仅当电话处于联机状态(当然)时登录才有效,但是不检查互联网是否可用,可以肯定地优化了代码
需要帮忙? (NEED HELP?)
Need help with this project? Download the full project at https://github.com/popeating/FirebaseLogin
在这个项目上需要帮助吗? 在https://github.com/popeating/FirebaseLogin下载完整项目
Contact me at lennaz@gmail.com
请通过lennaz@gmail.com与我联系